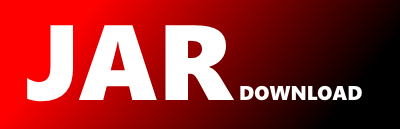
com.fluidbpm.ws.client.v1.form.FormContainerClient Maven / Gradle / Ivy
/*
* Koekiebox CONFIDENTIAL
*
* [2012] - [2017] Koekiebox (Pty) Ltd
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of Koekiebox and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Koekiebox
* and its suppliers and may be covered by South African and Foreign Patents,
* patents in process, and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material is strictly
* forbidden unless prior written permission is obtained from Koekiebox.
*/
package com.fluidbpm.ws.client.v1.form;
import com.fluidbpm.program.api.vo.attachment.Attachment;
import com.fluidbpm.program.api.vo.field.Field;
import com.fluidbpm.program.api.vo.flow.JobView;
import com.fluidbpm.program.api.vo.form.Form;
import com.fluidbpm.program.api.vo.form.FormListing;
import com.fluidbpm.program.api.vo.form.TableRecord;
import com.fluidbpm.program.api.vo.historic.FormFlowHistoricData;
import com.fluidbpm.program.api.vo.historic.FormFlowHistoricDataListing;
import com.fluidbpm.program.api.vo.historic.FormHistoricData;
import com.fluidbpm.program.api.vo.historic.FormHistoricDataListing;
import com.fluidbpm.program.api.vo.item.CustomWebAction;
import com.fluidbpm.program.api.vo.user.User;
import com.fluidbpm.program.api.vo.ws.WS;
import com.fluidbpm.ws.client.FluidClientException;
import com.fluidbpm.ws.client.v1.ABaseClientWS;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.List;
/**
* Java Web Service Client for Electronic Form related actions.
*
* @author jasonbruwer
* @since v1.0
*
* @see JSONObject
* @see com.fluidbpm.program.api.vo.ws.WS.Path.FormContainer
* @see Form
*/
public class FormContainerClient extends ABaseClientWS {
/**
* Constructor that sets the Service Ticket from authentication.
*
* @param endpointBaseUrlParam URL to base endpoint.
* @param serviceTicketParam The Server issued Service Ticket.
*/
public FormContainerClient(
String endpointBaseUrlParam, String serviceTicketParam
) {
super(endpointBaseUrlParam);
this.setServiceTicket(serviceTicketParam);
}
/**
* Create a new Form Container / Electronic Forms.
*
* @param form The Form to create.
* @param addToPersonalInventory Should the form be added to the users P/I after creation.
*
* @return Created Form Container / Electronic Form.
*
* @see Field
*/
public Form createFormContainer(
Form form,
boolean addToPersonalInventory
) {
if (form != null) form.setServiceTicket(this.serviceTicket);
return new Form(this.putJson(
form, WS.Path.FormContainer.Version1.formContainerCreate(addToPersonalInventory)));
}
/**
* Create a new Form Container / Electronic Forms.
* The Form will not be added to the P/I.
*
* @param form The Form to create.
* @return Created Form Container / Electronic Form.
*
* @see Field
*/
public Form createFormContainer(Form form) {
return this.createFormContainer(form, false);
}
/**
* Create a new history record for a {@code Form}.
*
* @param formHistory The FormHistoricData to create.
* @return Created Form Historic Data.
*
* @see FormHistoricData
*/
public FormHistoricData createFormHistoricData(FormHistoricData formHistory) {
if (formHistory != null) formHistory.setServiceTicket(this.serviceTicket);
return new FormHistoricData(this.putJson(formHistory, WS.Path.FormHistory.Version1.formHistoryCreate()));
}
/**
* Create a new Table Record.
*
* @param tableRecordParam The Table Record to create.
* @return Created Table Record.
*
* @see TableRecord
*/
public TableRecord createTableRecord(TableRecord tableRecordParam) {
if (tableRecordParam != null) tableRecordParam.setServiceTicket(this.serviceTicket);
return new TableRecord(this.putJson(
tableRecordParam,
WS.Path.FormContainerTableRecord.Version1.formContainerTableRecordCreate()));
}
/**
* Update a Form Container / Electronic Form.
* The table record forms may also be updated with
*
* @param formParam The Form to update.
* @return Updated Form Container / Electronic Form.
*
* @see Field
* @see TableRecord
* @see Form
*/
public Form updateFormContainer(Form formParam) {
if (formParam != null) formParam.setServiceTicket(this.serviceTicket);
return new Form(this.postJson(formParam, WS.Path.FormContainer.Version1.formContainerUpdate()));
}
/**
* Execute the Custom Program with action alias {@code customWebActionParam}.
* This method may be used for Form and Table Records.
*
* @param customWebActionParam The custom web action name. Action identifier.
* @param formParam The Form to send for 3rd Party execution.
*
* @return Result after the 3rd Party Custom Web Action completed.
*
* @see Field
* @see Form
* @see com.fluidbpm.program.api.vo.thirdpartylib.ThirdPartyLibrary
*/
public CustomWebAction executeCustomWebAction(String customWebActionParam, Form formParam) {
return this.executeCustomWebAction(
customWebActionParam,
false,
null,
formParam);
}
/**
* Execute the Custom Program with action alias {@code customWebActionParam}.
* This method may be used for Form and Table Records.
*
* @param customWebActionParam The custom web action name. Action identifier.
* @param isTableRecordParam Is the form a table record form.
* @param formContainerTableRecordBelongsToParam The parent form container if table record.
* @param formParam The Form to send for 3rd Party execution.
*
* @return Result after the 3rd Party Custom Web Action completed.
*
* @see Field
* @see Form
* @see TableRecord
* @see com.fluidbpm.program.api.vo.thirdpartylib.ThirdPartyLibrary
*/
public CustomWebAction executeCustomWebAction(
String customWebActionParam,
boolean isTableRecordParam,
Long formContainerTableRecordBelongsToParam,
Form formParam
) {
if (customWebActionParam == null || customWebActionParam.trim().isEmpty()) throw new FluidClientException(
"Custom Web Action is mandatory.", FluidClientException.ErrorCode.FIELD_VALIDATE);
CustomWebAction action = new CustomWebAction();
action.setTaskIdentifier(customWebActionParam);
action.setServiceTicket(this.serviceTicket);
action.setForm(formParam);
action.setIsTableRecord(isTableRecordParam);
action.setFormTableRecordBelongsTo(formContainerTableRecordBelongsToParam);
return new CustomWebAction(this.postJson(action, WS.Path.FormContainer.Version1.executeCustomWebAction()));
}
/**
* Deletes the Form Container provided.
* Id must be set on the Form Container.
*
* @param formContainerParam The Form Container to Delete.
* @return The deleted Form Container.
*/
public Form deleteFormContainer(Form formContainerParam) {
if (formContainerParam != null) formContainerParam.setServiceTicket(this.serviceTicket);
return new Form(this.postJson(formContainerParam, WS.Path.FormContainer.Version1.formContainerDelete()));
}
/**
* Retrieves Electronic Form Workflow historic information.
*
* The Form Id must be provided.
*
* @param form The form to retrieve historic data for.
* @return Electronic Form Workflow historic data.
*/
public List getFormFlowHistoricData(Form form) {
Form formToSend = new Form(form.getId());
formToSend.setFormType(form.getFormType());
formToSend.setFormTypeId(form.getFormTypeId());
formToSend.setServiceTicket(this.serviceTicket);
return new FormFlowHistoricDataListing(this.postJson(
formToSend, WS.Path.FlowItemHistory.Version1.getByFormContainer())).getListing();
}
/**
* Retrieves Electronic Form and Field historic information.
*
* The Form Id must be provided.
*
* @param includeCurrent Whether the current values should be included.
*
* @param form The form to retrieve historic data for.
* @return Electronic Form and Field historic data.
*/
public List getFormAndFieldHistoricData(
Form form,
boolean includeCurrent
) {
return this.getFormAndFieldHistoricData(form, includeCurrent, false);
}
/**
* Retrieves Electronic Form and Field historic information.
*
* The Form Id must be provided.
*
* @param includeCurrent Whether the current values should be included.
* @param labelFieldName Should the label field name be used instead of the system name.
*
* @param form The form to retrieve historic data for.
* @return Electronic Form and Field historic data.
*/
public List getFormAndFieldHistoricData(
Form form,
boolean includeCurrent,
boolean labelFieldName
) {
Form formToSend = new Form(form.getId());
formToSend.setFormType(form.getFormType());
formToSend.setFormTypeId(form.getFormTypeId());
formToSend.setServiceTicket(this.serviceTicket);
return new FormHistoricDataListing(this.postJson(
formToSend, WS.Path.FormHistory.Version1.getByFormContainer(
includeCurrent, labelFieldName))).getListing();
}
/**
* Retrieves Electronic Form and Field historic information for
* the most recent modification.
*
* The Form Id must be provided.
*
* @param formParam The form to retrieve historic data for.
*
* @return Electronic Form and Field historic data.
*/
public FormHistoricData getMostRecentFormAndFieldHistoricData(Form formParam) {
if (formParam != null) formParam.setServiceTicket(this.serviceTicket);
return new FormHistoricData(this.postJson(
formParam, WS.Path.FormHistory.Version1.getByMostRecentByFormContainer()));
}
/**
* Retrieves the Form Container by Primary key.
*
* @param formContainerId The Form Container primary key.
* @return Form by Primary key.
*/
public Form getFormContainerById(Long formContainerId) {
return this.getFormContainerById(formContainerId, false);
}
/**
* Retrieves the Form Container by Primary key.
*
* @param formContainerId The Form Container primary key.
* @param executeCalculatedLabels Should calculated labels be executed.
* @return Form by Primary key.
*/
public Form getFormContainerById(Long formContainerId, boolean executeCalculatedLabels) {
Form form = new Form(formContainerId);
form.setServiceTicket(this.serviceTicket);
return new Form(this.postJson(
form, WS.Path.FormContainer.Version1.getById(executeCalculatedLabels)));
}
/**
* Fetch the parent/ancestor form for {@code form}
*
* @param form The child form.
*
* @return List of forms where child is {@code form}
*
* @see Form
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy