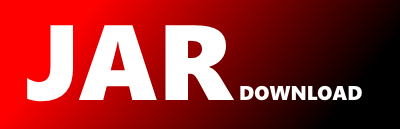
fluximpl.RabbitMQActionImplBeanInfo Maven / Gradle / Ivy
package fluximpl;
import java.awt.*;
import java.beans.BeanDescriptor;
import java.beans.BeanInfo;
import java.beans.PropertyDescriptor;
import java.util.Arrays;
import java.util.Vector;
/**
* BeanInfo for RabbitMQAction.
*
* @author [email protected]
*/
public class RabbitMQActionImplBeanInfo extends ActionImplBeanInfo {
/**
* A small image for this custom action to be displayed in the Flux GUI.
*/
private Image smallImage;
/**
* A large image for this custom action to be displayed in the Flux GUI.
*/
private Image bigImage;
protected BeanDescriptor bd = new BeanDescriptor(RabbitMQActionImpl.class);
public RabbitMQActionImplBeanInfo(BeanDescriptor bd) {
super();
this.bd = bd;
setCategory(bd, ActionImplBeanInfo.MESSAGING);
}
public RabbitMQActionImplBeanInfo() {
super();
bd.setDisplayName("RabbitMQ Action");
bd.setShortDescription("Publishes AMQP message to a RabbitMQ queue.");
setCategory(bd, ActionImplBeanInfo.MESSAGING);
}
public Image getIcon(int type) {
if (type == BeanInfo.ICON_COLOR_16x16) {
return getSmallImage();
} // if
if (type == BeanInfo.ICON_COLOR_32x32) {
return getBigImage();
} // if
return null;
} // getIcon()
/**
* Returns a small image that represents this JavaBean.
*
* @return A small image that represents this JavaBean.
*/
private Image getSmallImage() {
if (smallImage == null) {
smallImage = loadImage("/RabbitMQActionImpl_small.png");
} // if
return smallImage;
} // getSmallImage()
/**
* Returns a large image that represents this JavaBean.
*
* @return A large image that represents this JavaBean.
*/
private Image getBigImage() {
if (bigImage == null) {
bigImage = loadImage("/RabbitMQActionImpl_big.png");
} // if
return bigImage;
} // getBigImage()
public BeanDescriptor getBeanDescriptor() {
return bd;
}
public PropertyDescriptor[] getPropertyDescriptors() {
Vector descriptors = new Vector(Arrays.asList(super.getPropertyDescriptors()));
try {
int order = 10;
descriptors.add(makePropertyDescriptor("exchangeName", "Exchange Name", RabbitMQActionImpl.class.getName(), "getExchangeName", true, null, order++, false, false, false, false));
descriptors.add(makePropertyDescriptor("exchangeType", "Exchange Type", RabbitMQActionImpl.class.getName(), "getExchangeType", true, null, order++, false, false, false, false));
descriptors.add(makePropertyDescriptor("host", "Hostname", RabbitMQActionImpl.class.getName(), "getHost", true, null, order++, false, false, false, false));
descriptors.add(makePropertyDescriptor("message", "Message", RabbitMQActionImpl.class.getName(), "getMessage", true, null, order++, false, false, false, false));
descriptors.add(makePropertyDescriptor("password", "Password", RabbitMQActionImpl.class.getName(), "getPassword", true, null, order++, false, false, false, true));
descriptors.add(makePropertyDescriptor("port", "Port", RabbitMQActionImpl.class.getName(), "getPort", true, null, order++, false, false, false, false));
descriptors.add(makePropertyDescriptor("queueName", "Queue Name", RabbitMQActionImpl.class.getName(), "getQueueName", true, null, order++, false, false, false, false));
descriptors.add(makePropertyDescriptor("queueType", "Queue Type", RabbitMQActionImpl.class.getName(), "getQueueType", true, null, order++, false, false, false, false));
descriptors.add(makePropertyDescriptor("routingKey", "Routing Key", RabbitMQActionImpl.class.getName(), "getRoutingKey", false, null, order++, false, false, false, false));
descriptors.add(makePropertyDescriptor("username", "Username", RabbitMQActionImpl.class.getName(), "getUsername", true, null, order++, false, false, false, false));
descriptors.add(makePropertyDescriptor("virtualHost", "Virtual Host", RabbitMQActionImpl.class.getName(), "getVirtualHost", false, null, order, false, false, false, false));
} catch (Exception e) {
e.printStackTrace();
throw new IllegalStateException("Could not create property descriptor.");
} // catch
return descriptors.toArray(new PropertyDescriptor[descriptors.size()]);
}
public void setCategory(BeanDescriptor beanDescriptor, String category) {
beanDescriptor.setValue("category", category);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy