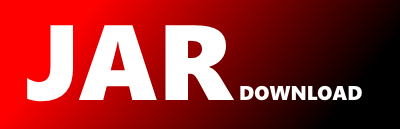
fluximpl.UnzipFileActionImpl Maven / Gradle / Ivy
package fluximpl;
import flux.EngineException;
import flux.FlowContext;
import flux.UnzipFileAction;
import java.io.File;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.util.Enumeration;
import java.util.Set;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
/**
* Implementation for UnzipFileAction.
*
* @author [email protected]
*/
public class UnzipFileActionImpl extends ActionImpl implements UnzipFileAction {
public static String ACTION_VARIABLE = "UNZIP_FILE_ACTION_VARIABLE";
public UnzipFileActionImpl() {
super(new FlowChartImpl(), "Unzip File Action");
}
public UnzipFileActionImpl(FlowChartImpl flowChart, String name) {
super(flowChart, name);
}
public Set getHiddenVariableNames() {
// The returned raw type is a Set of String.
@SuppressWarnings("unchecked")
Set set = super.getHiddenVariableNames();
set.add(ACTION_VARIABLE);
return set;
}
public Object execute(FlowContext flowContext) throws Exception {
UnzipFileActionVariable var = getVariable();
String base = var.getDestination();
if (base == null || base.equalsIgnoreCase(".")) {
base = "";
}
if (!base.equalsIgnoreCase("") && !base.endsWith("/")) {
base += "/";
}
ZipFile zipFile = new ZipFile(new File(var.getZipFile()), ZipFile.OPEN_READ);
final Enumeration extends ZipEntry> entries = zipFile.entries();
byte buffer[] = new byte[51200];
int bytesRead;
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
File fileEntry = new File(base + entry.getName());
if (entry.isDirectory()) {
fileEntry.mkdirs();
} else {
InputStream in = zipFile.getInputStream(entry);
if (!fileEntry.createNewFile()) {
fileEntry.delete();
fileEntry.createNewFile();
}
FileOutputStream out = new FileOutputStream(fileEntry);
int offset = 0;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, offset, bytesRead);
}
}
}
return null;
}
public void verify() throws EngineException {
UnzipFileActionVariable var = getVariable();
if (var.getZipFile() == null || var.getZipFile().equalsIgnoreCase("")) {
throw new EngineException("Expected zip file path to be non-null or not empty, but it was");
}
}
public String getDestination() {
return getVariable().getDestination();
}
public void setDestination(String path) {
UnzipFileActionVariable var = getVariable();
var.setDestination(path);
putVariable(var);
}
public String getZipFile() {
return getVariable().getZipFile();
}
public void setZipFile(String path) {
UnzipFileActionVariable var = getVariable();
var.setZipFile(path);
putVariable(var);
}
private UnzipFileActionVariable getVariable() {
if (!getVariableManager().contains(ACTION_VARIABLE)) {
getVariableManager().put(ACTION_VARIABLE, new UnzipFileActionVariable());
} // if
return (UnzipFileActionVariable) getVariableManager().get(ACTION_VARIABLE);
} // getVariable()
private void putVariable(UnzipFileActionVariable variable) {
getVariableManager().put(ACTION_VARIABLE, variable);
} // putVariable()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy