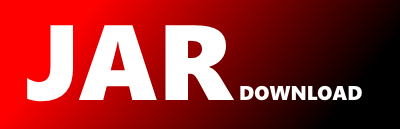
fluximpl.UnzipFileActionImplBeanInfo Maven / Gradle / Ivy
package fluximpl;
import java.awt.*;
import java.beans.BeanDescriptor;
import java.beans.BeanInfo;
import java.beans.IntrospectionException;
import java.beans.PropertyDescriptor;
import java.util.Arrays;
import java.util.Vector;
/**
* BeanInfo for UnzipFileAction.
*
* @author [email protected]
*/
public class UnzipFileActionImplBeanInfo extends ActionImplBeanInfo {
/**
* A small image for this custom action to be displayed in the Flux GUI.
*/
private Image smallImage;
/**
* A large image for this custom action to be displayed in the Flux GUI.
*/
private Image bigImage;
/**
* This BeanInfo needs a BeanDescriptor.
*/
protected BeanDescriptor bd = new BeanDescriptor(UnzipFileActionImpl.class);
/**
* This BeanInfo needs a BeanDescriptor.
*/
public UnzipFileActionImplBeanInfo(BeanDescriptor bd) {
super();
this.bd = bd;
setCategory(bd, ActionImplBeanInfo.FILE);
} // constructor
/**
* This BeanInfo needs a BeanDescriptor.
*/
public UnzipFileActionImplBeanInfo() {
// setup bean descriptor in constructor
bd.setDisplayName("Unzip File Action");
bd.setShortDescription("Unzips a specified zip archive file.");
setCategory(bd, ActionImplBeanInfo.FILE);
} // constructor
/**
* Returns this BeanInfo's BeanDescriptor.
*
* @return This BeanInfo's BeanDescriptor.
*/
public BeanDescriptor getBeanDescriptor() {
return bd;
} // getBeanDescriptor()
/**
* You can change the icon associated with your custom action/trigger here.
*/
public Image getIcon(int type) {
if (type == BeanInfo.ICON_COLOR_16x16) {
return getSmallImage();
} // if
if (type == BeanInfo.ICON_COLOR_32x32) {
return getBigImage();
} // if
return null;
} // getIcon()
/**
* Returns all JavaBean descriptors described by this BeanInfo.
*
* @return All JavaBean descriptors described by this BeanInfo.
*/
public PropertyDescriptor[] getPropertyDescriptors() {
Vector descriptors = new Vector(Arrays.asList(super.getPropertyDescriptors()));
PropertyDescriptor nameDescriptor = null, contentDescriptor = null;
try {
nameDescriptor = new PropertyDescriptor("zipFile", UnzipFileActionImpl.class);
nameDescriptor.setDisplayName("Zip File");
nameDescriptor.setShortDescription("Zip file path");
descriptors.add(nameDescriptor);
contentDescriptor = new PropertyDescriptor("destination", UnzipFileActionImpl.class);
contentDescriptor.setDisplayName("Destination");
contentDescriptor.setShortDescription("Destination directory path");
descriptors.add(contentDescriptor);
} // try
catch (IntrospectionException e) {
e.printStackTrace();
throw new IllegalStateException("could not create property descriptor");
} // catch
return (PropertyDescriptor[]) descriptors.toArray(new PropertyDescriptor[descriptors.size()]);
} // getPropertyDescriptors()
/**
* Returns a small image that represents this JavaBean.
*
* @return A small image that represents this JavaBean.
*/
private Image getSmallImage() {
if (smallImage == null) {
smallImage = loadImage("/UnzipFileActionImpl_small.png");
} // if
return smallImage;
} // getSmallImage()
/**
* Returns a large image that represents this JavaBean.
*
* @return A large image that represents this JavaBean.
*/
private Image getBigImage() {
if (bigImage == null) {
bigImage = loadImage("/UnzipFileActionImpl_big.png");
} // if
return bigImage;
} // getBigImage()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy