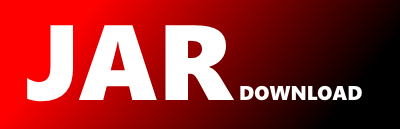
com.fluxtion.ext.streaming.api.WrappedCollection Maven / Gradle / Ivy
/*
* Copyright (c) 2020, V12 Technology Ltd.
* All rights reserved.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Server Side Public License, version 1,
* as published by MongoDB, Inc.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Server Side Public License for more details.
*
* You should have received a copy of the Server Side Public License
* along with this program. If not, see
* .
*/
package com.fluxtion.ext.streaming.api;
import com.fluxtion.api.partition.LambdaReflection.SerializableFunction;
import com.fluxtion.ext.streaming.api.stream.StreamOperator;
import java.util.Collection;
import java.util.Comparator;
import java.util.Iterator;
import java.util.function.Consumer;
import java.util.stream.Stream;
/**
*
* @author Greg Higgins [email protected]
* @param The type held in this collection
* @param The underlying collection type
* @param The type of the subclass of WrappedCollection
*/
public interface WrappedCollection, C extends WrappedCollection>
extends Stateful, WrapperBase, C> {
default WrappedList comparing(SerializableFunction in) {
throw new UnsupportedOperationException("comparing not implemented in WrappedCollection");
}
default WrappedList comparator(Comparator comparator) {
return null;
}
U collection();
default WrappedCollection sliding(int itemsPerBucket, int numberOfBuckets){
return null;
}
default WrappedCollection sliding(Duration timePerBucket, int numberOfBuckets){
return null;
}
/**
* Collects the events into a WrappedList using a time based tumbling window strategy.
* @param time duration of the tumbling window
* @return The collection of events in sliding window
*/
default WrappedCollection tumbling(Duration time){
return null;
}
/**
* Collects the events into a WrappedList using a time based tumbling window strategy.
* @param itemCount number of items in the tumbling window
* @return The collection of events in sliding window
*/
default WrappedCollection tumbling(int itemCount){
return null;
}
default Wrapper map(SerializableFunction mapper) {
return StreamOperator.service().map(mapper, asWrapper(), true);
}
default Wrapper asWrapper() {
return StreamOperator.service().streamInstance(this::collection);
}
WrappedCollection resetNotifier(Object resetNotifier);
@Override
default U event() {
return collection();
}
@Override
default Class> eventClass() {
return (Class>) collection().getClass();
}
default int size() {
return collection().size();
}
default boolean isEmpty() {
return collection().isEmpty();
}
default boolean contains(T o) {
return collection().contains(o);
}
default Iterator iterator() {
return collection().iterator();
}
default Stream stream() {
return collection().stream();
}
default void forEach(Consumer super T> action) {
collection().forEach(action);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy