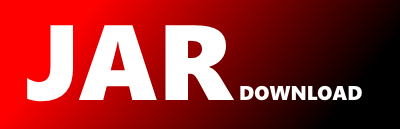
com.fluxtion.ext.streaming.api.group.GroupByTargetMap Maven / Gradle / Ivy
/*
* Copyright (C) 2018 V12 Technology Ltd.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Server Side Public License, version 1,
* as published by MongoDB, Inc.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Server Side Public License for more details.
*
* You should have received a copy of the Server Side Public License
* along with this program. If not, see
* .
*/
package com.fluxtion.ext.streaming.api.group;
import com.fluxtion.ext.streaming.api.Wrapper;
import com.fluxtion.ext.streaming.api.numeric.BufferValue;
import java.lang.reflect.InvocationTargetException;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.function.Supplier;
/**
* A map holding the instances in the group by
*
* @param The underlying instance type of the group by
* @param The underlying instance type of the key of the group by
* @param The wrapper for elements in the map
* @author Greg Higgins
*/
public class GroupByTargetMap> {
private Supplier supplier;
private final Class targetClass;
private final HashMap map = new HashMap<>();
private final Map immutableMap = Collections.unmodifiableMap(map);
public GroupByTargetMap(Class targetClass) {
this.targetClass = targetClass;
}
public GroupByTargetMap(Supplier targetClass) {
this.supplier = targetClass;
this.targetClass = null;
}
//TODO add methods for Numeric value, and primitive types
public T getOrCreateInstance(Object key, GroupByIniitialiser initialiser, K source) {
T instance = map.get(key);
if (instance == null) {
try {
instance = newTargetInstance();
initialiser.apply(source, instance.event());
map.put((K) key, instance);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
return instance;
}
public T getOrCreateInstance(Object key) {
T instance = map.get(key);
if (instance == null) {
try {
instance = newTargetInstance();
map.put((K) key, instance);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
return instance;
}
return instance;
}
//use charsequence key!!
public T getOrCreateInstance(CharSequence key) {
String keyString = key.toString();
T instance = map.get(keyString);
if (instance == null) {
try {
instance = newTargetInstance();
map.put((K) keyString, instance);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
return instance;
}
public T getOrCreateInstance(BufferValue key) {
T instance = map.get(key.asString());
if (instance == null) {
try {
instance = newTargetInstance();
map.put((K) key.asString(), instance);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
return instance;
}
public T getOrCreateInstance(CharSequence key, GroupByIniitialiser initialiser, K source) {
String keyString = key.toString();
T instance = map.get(keyString);
if (instance == null) {
try {
instance = newTargetInstance();
initialiser.apply(source, instance.event());
map.put((K) keyString, instance);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
return instance;
}
public T getOrCreateInstance(BufferValue key, GroupByIniitialiser initialiser, K source) {
T instance = map.get(key.asString());
if (instance == null) {
try {
instance = newTargetInstance();
initialiser.apply(source, instance.event());
map.put((K) key.asString(), instance);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
return instance;
}
public T getOrCreateInstance(MultiKey key, GroupByIniitialiser initialiser, K source) {
T instance = map.get(key);
if (instance == null) {
try {
instance = newTargetInstance();
initialiser.apply(source, instance.event());
map.put(key.copyKey(), instance);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
return instance;
}
public T getOrCreateInstance(MultiKey key) {
T instance = map.get(key);
if (instance == null) {
try {
instance = newTargetInstance();
map.put(key.copyKey(), instance);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
return instance;
}
private T newTargetInstance() throws InstantiationException, IllegalAccessException, NoSuchMethodException, IllegalArgumentException, InvocationTargetException{
if(supplier!=null){
return supplier.get();
}
return targetClass.getDeclaredConstructor().newInstance();
}
public T getInstance(K key) {
return map.get(key);
}
public Map getInstanceMap() {
return immutableMap;
}
public T expireInstance(K key){
return map.remove(key);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy