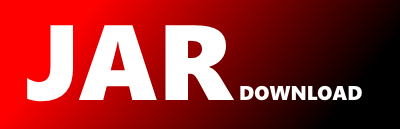
com.fluxtion.ext.streaming.api.stream.Argument Maven / Gradle / Ivy
package com.fluxtion.ext.streaming.api.stream;
import com.fluxtion.api.partition.LambdaReflection.SerializableFunction;
import com.fluxtion.api.partition.LambdaReflection.SerializableSupplier;
import com.fluxtion.ext.streaming.api.Wrapper;
import com.fluxtion.ext.streaming.api.WrapperBase;
import com.fluxtion.ext.streaming.api.numeric.ConstantNumber;
import java.lang.reflect.Method;
import java.util.logging.Level;
import java.util.logging.Logger;
import lombok.Data;
/**
* Representation of a an argument
*
* @author Greg Higgins [email protected]
* @param
*/
@Data
public class Argument {
public Object source;
public Method accessor;
public boolean cast;
public static Argument arg(SerializableFunction supplier) {
final Class containingClass = supplier.getContainingClass();
return new Argument(StreamOperator.service().select(containingClass), supplier.method(), true);
}
public static Argument arg(Double d) {
SerializableFunction s = Number::doubleValue;
return new Argument<>(new ConstantNumber(d), s.method(), true);
}
public static Argument arg(int i) {
SerializableFunction s = Number::intValue;
return new Argument<>(new ConstantNumber(i), s.method(), true);
}
public static Argument arg(long l) {
SerializableFunction s = Number::longValue;
return new Argument<>(new ConstantNumber(l), s.method(), true);
}
public static Argument arg(Wrapper wrapper) {
return arg(wrapper, Number::doubleValue);
}
public static Argument arg(Wrapper wrapper, SerializableFunction supplier) {
return new Argument<>(wrapper, supplier.method(), true);
}
public static Argument arg(WrapperBase wrapper, SerializableFunction supplier) {
return new Argument<>(wrapper, supplier.method(), true);
}
public static Argument arg(SerializableSupplier supplier) {
Class extends Object> aClass = supplier.captured()[0].getClass();
Method method = supplier.method();
try {
method = aClass.getMethod(supplier.method().getName());
} catch (NoSuchMethodException | SecurityException ex) {
Logger.getLogger(Argument.class.getName()).log(Level.SEVERE, null, ex);
}
return new Argument<>(supplier.captured()[0], method, true);
}
public static Argument arg(Class clazz) {
Wrapper select = StreamOperator.service().select(clazz);
return arg(select);
}
public static Argument arg(Object supplier) {
return new Argument(supplier, null, true);
}
public static Argument argInt(T i) {
SerializableFunction s = Number::intValue;
return new Argument<>(i, s.method(), true);
}
public static Argument argInt(Wrapper i) {
SerializableFunction s = Number::intValue;
return new Argument<>(i, s.method(), true);
}
public static Argument argLong(T i) {
SerializableFunction s = Number::longValue;
return new Argument<>(i, s.method(), true);
}
public static Argument argLong(Wrapper i) {
SerializableFunction s = Number::longValue;
return new Argument<>(i, s.method(), true);
}
public static Argument argDouble(T i) {
SerializableFunction s = Number::doubleValue;
return new Argument<>(i, s.method(), true);
}
public static Argument argDouble(Wrapper i) {
SerializableFunction s = Number::doubleValue;
return new Argument<>(i, s.method(), true);
}
public Argument(Object source, Method accessor, boolean cast) {
this.source = source;
this.accessor = accessor;
this.cast = cast;
}
public boolean isWrapper() {
return source instanceof Wrapper;
}
public boolean isWrapperBase() {
return source instanceof WrapperBase;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy