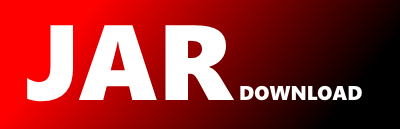
com.fluxtion.ext.streaming.api.stream.CollectionFunctions Maven / Gradle / Ivy
/*
* Copyright (c) 2020, V12 Technology Ltd.
* All rights reserved.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Server Side Public License, version 1,
* as published by MongoDB, Inc.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Server Side Public License for more details.
*
* You should have received a copy of the Server Side Public License
* along with this program. If not, see
* .
*/
package com.fluxtion.ext.streaming.api.stream;
import com.fluxtion.api.partition.LambdaReflection.SerializableFunction;
import com.fluxtion.ext.streaming.api.WrappedList;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
/**
* Functions to use with WrappedList
*
* @author Greg Higgins [email protected]
*/
public class CollectionFunctions {
public static List toList(Collection collection) {
return new ArrayList<>(collection);
}
public static SerializableFunction, Number> listSum() {
return CollectionFunctions::sumList;
}
public static SerializableFunction, Number> listMin() {
return CollectionFunctions::minList;
}
public static SerializableFunction, Number> listMax() {
return CollectionFunctions::maxList;
}
public static SerializableFunction, Number> listAvg() {
return CollectionFunctions::avgList;
}
public static SerializableFunction, Number> wrappedAvg() {
return CollectionFunctions::avgWrappedList;
}
public static SerializableFunction, Number> wrappedMax() {
return CollectionFunctions::maxWrappedList;
}
public static SerializableFunction, Number> wrappedMin() {
return CollectionFunctions::minWrappedList;
}
public static SerializableFunction, Number> wrappedSum() {
return CollectionFunctions::sumWrappedList;
}
public static double sumList(final List collection) {
if (collection.isEmpty()) {
return Double.NaN;
}
int size = collection.size();
double ans = 0;
for (int j = 0; j < size; j++) {
ans += collection.get(j).doubleValue();
}
return ans;
}
public static double minList(final List collection) {
if (collection.isEmpty()) {
return Double.NaN;
}
int size = collection.size();
double ans = 0;
for (int j = 0; j < size; j++) {
ans = Math.min(ans, collection.get(j).doubleValue());
}
return ans;
}
public static double maxList(final List collection) {
if (collection.isEmpty()) {
return Double.NaN;
}
int size = collection.size();
double ans = 0;
for (int j = 0; j < size; j++) {
ans = Math.max(ans, collection.get(j).doubleValue());
}
return ans;
}
public static double avgList(final List collection) {
return sumList(collection) / collection.size();
}
public static double sumWrappedList(WrappedList list) {
return sumList(list.collection());
}
public static double minWrappedList(WrappedList list) {
return minList(list.collection());
}
public static double maxWrappedList(WrappedList list) {
return maxList(list.collection());
}
public static double avgWrappedList(WrappedList list) {
return sumWrappedList(list) / list.size();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy