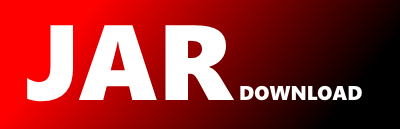
com.fluxtion.ext.streaming.api.stream.StreamOperator Maven / Gradle / Ivy
/*
* Copyright (C) 2019 V12 Technology Ltd.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Server Side Public License, version 1,
* as published by MongoDB, Inc.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Server Side License for more details.
*
* You should have received a copy of the Server Side Public License
* along with this program. If not, see
* .
*/
package com.fluxtion.ext.streaming.api.stream;
import com.fluxtion.api.partition.LambdaReflection;
import com.fluxtion.api.partition.LambdaReflection.SerializableBiFunction;
import com.fluxtion.api.partition.LambdaReflection.SerializableConsumer;
import com.fluxtion.api.partition.LambdaReflection.SerializableFunction;
import com.fluxtion.ext.streaming.api.FilterWrapper;
import com.fluxtion.ext.streaming.api.Wrapper;
import com.fluxtion.ext.streaming.api.WrapperBase;
import com.fluxtion.ext.streaming.api.group.GroupBy;
import java.lang.reflect.Method;
import java.util.Comparator;
import java.util.ServiceLoader;
/**
* An interface defining stream operations on a node in a SEP graph. The
* {@link ServiceLoader} mechanism is used to load an implementation at runtime
* for the StreamOperator interface.
*
* @author V12 Technology Ltd.
*/
public interface StreamOperator {
default Wrapper select(Class eventClazz) {
return null;
}
default FilterWrapper filter(SerializableFunction filter,
Wrapper source, Method accessor, boolean cast) {
return (FilterWrapper) source;
}
default FilterWrapper filter(SerializableFunction extends T, Boolean> filter,
Wrapper source, boolean cast) {
return (FilterWrapper) source;
}
default GroupBy group(Wrapper source,
SerializableFunction key, SerializableBiFunction super R, ? super R, ? extends R> calcFunctionClass) {
return null;
}
default GroupBy group(Wrapper source,
SerializableFunction key,
SerializableFunction supplier,
SerializableBiFunction super R, ? super R, ? extends R> functionClass) {
return null;
}
default Wrapper streamInstance(LambdaReflection.SerializableSupplier source) {
return null;
}
/**
* Streams the return of a method as a wrapped instance.
*
* @param
* @param
* @param mapper
* @param source
* @return
*/
default Wrapper get(SerializableFunction mapper, Wrapper source) {
return null;
}
default Wrapper map(SerializableFunction mapper, Wrapper source, boolean cast) {
return null;
}
default Wrapper map(SerializableFunction mapper, Wrapper source, Method accessor, boolean cast) {
return null;
}
default Wrapper map(SerializableBiFunction extends U, ? extends S, R> mapper,
Argument extends U> arg1,
Argument extends S> arg2) {
return null;
}
default Wrapper map(F mapper, Method mappingMethod, Argument... args) {
return null;
}
/**
* push data from the wrapper to the consumer
*
* @param
* @param
* @param source
* @param accessor
* @param consumer
*/
default void push(Wrapper source, Method accessor, SerializableConsumer consumer) {
}
/**
* Supply the wrapper to a consumer when the wrapper is on the execution
* path
*
* @param
* @param
* @param consumer
* @param source
* @param consumerId - id for node in SEP, can be null for autonaming
* @return
*/
default Wrapper forEach(SerializableConsumer consumer, Wrapper source, String consumerId) {
return source;
}
default Wrapper log(Wrapper source, String message, SerializableFunction... supplier) {
return source;
}
default WrapperBase log(WrapperBase source, String message, SerializableFunction... supplier) {
return source;
}
/**
* Attaches an event notification instance to the current stream node and
* merges notifications from stream and the added notifier.When the notifier
* updates all the child nodes of this stream node will be on the execution
* path and invoked following normal SEP rules.The existing execution path
* will be unaltered if either the parent wrapped node or the eventNotifier
* updates then the execution path will progress.
*
* @param
* @param source
* @param notifier
* @return
*/
default Wrapper notiferMerge(Wrapper source, Object notifier) {
return source;
}
/**
* Attaches an event notification instance to the current stream node,
* overriding the execution path of the current stream.Only when the
* notifier updates will the child nodes of this stream node be on the
* execution path.
*
* @param
* @param source
* @param notifier external event notifier
* @return
*/
default Wrapper notifierOverride(Wrapper source, Object notifier) {
return source;
}
/**
* name a StreamOperator node in the generated SEP.
*
* @param
* @param node
* @param name
* @return
*/
default T nodeId(T node, String name) {
return node;
}
default Comparator comparing(SerializableBiFunction func) {
return null;
}
default Wrapper defaultVal(Wrapper source, T defaultValue){
return null;
}
static StreamOperator service() {
ServiceLoader load = ServiceLoader.load(StreamOperator.class);
if (load.iterator().hasNext()) {
return load.iterator().next();
} else {
load = ServiceLoader.load(StreamOperator.class, StreamOperator.class.getClassLoader());
if (load.iterator().hasNext()) {
return load.iterator().next();
} else {
return new StreamOperator() {
};
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy