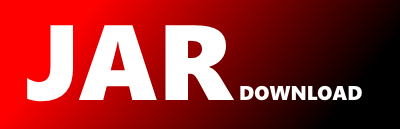
com.fluxtion.ext.streaming.builder.util.FunctionArg Maven / Gradle / Ivy
/*
* Copyright (C) 2019 V12 Technology Ltd.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Server Side Public License, version 1,
* as published by MongoDB, Inc.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Server Side License for more details.
*
* You should have received a copy of the Server Side Public License
* along with this program. If not, see
* .
*/
package com.fluxtion.ext.streaming.builder.util;
import com.fluxtion.api.event.Event;
import com.fluxtion.api.partition.LambdaReflection.SerializableFunction;
import com.fluxtion.api.partition.LambdaReflection.SerializableSupplier;
import com.fluxtion.ext.streaming.api.Wrapper;
import com.fluxtion.ext.streaming.api.numeric.ConstantNumber;
import com.fluxtion.ext.streaming.api.stream.Argument;
import static com.fluxtion.ext.streaming.builder.event.EventSelect.select;
import java.lang.reflect.Method;
/**
* Represents an argument for use with a function
*
* @author V12 Technology Ltd.
*/
public class FunctionArg extends Argument {
public static FunctionArg arg(SerializableFunction supplier) {
final Class containingClass = supplier.getContainingClass();
if (Event.class.isAssignableFrom(containingClass)) {
return new FunctionArg(select(containingClass), supplier.method(), true);
} else {
try {
return new FunctionArg(containingClass.newInstance(), supplier.method(), true);
} catch (InstantiationException | IllegalAccessException ex) {
throw new RuntimeException("default constructor missing for class:'" + containingClass + "'");
}
}
}
public static FunctionArg arg(Double d) {
SerializableFunction s = Number::doubleValue;
return new FunctionArg(new ConstantNumber(d), s.method(), true);
}
public static FunctionArg arg(int d) {
SerializableFunction s = Number::intValue;
return new FunctionArg(new ConstantNumber(d), s.method(), true);
}
public static FunctionArg arg(long d) {
SerializableFunction s = Number::longValue;
return new FunctionArg(new ConstantNumber(d), s.method(), true);
}
public static FunctionArg arg(Wrapper wrapper) {
return arg(wrapper, Number::doubleValue);
}
public static FunctionArg arg(Wrapper wrapper, SerializableFunction supplier) {
return new FunctionArg(wrapper, supplier.method(), true);
}
public static FunctionArg arg(SerializableSupplier supplier) {
return new FunctionArg(supplier.captured()[0], supplier.method(), true);
}
public static FunctionArg arg(Object supplier) {
return new FunctionArg(supplier, null, true);
}
public FunctionArg(Object source, Method accessor, boolean cast) {
super(source, accessor, cast);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy