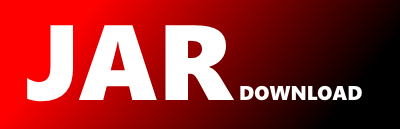
com.fluxtion.compiler.builder.dataflow.AbstractFlowBuilder Maven / Gradle / Ivy
package com.fluxtion.compiler.builder.dataflow;
import com.fluxtion.runtime.EventProcessorBuilderService;
import com.fluxtion.runtime.dataflow.TriggeredFlowFunction;
import com.fluxtion.runtime.dataflow.function.*;
import com.fluxtion.runtime.dataflow.function.MapFlowFunction.MapRef2RefFlowFunction;
import com.fluxtion.runtime.dataflow.function.MapFlowFunction.MapRef2ToDoubleFlowFunction;
import com.fluxtion.runtime.dataflow.function.MapFlowFunction.MapRef2ToIntFlowFunction;
import com.fluxtion.runtime.dataflow.function.MapFlowFunction.MapRef2ToLongFlowFunction;
import com.fluxtion.runtime.dataflow.helpers.InternalEventDispatcher;
import com.fluxtion.runtime.dataflow.helpers.Peekers;
import com.fluxtion.runtime.dataflow.helpers.Predicates.PredicateWrapper;
import com.fluxtion.runtime.output.SinkPublisher;
import com.fluxtion.runtime.partition.LambdaReflection;
import com.fluxtion.runtime.partition.LambdaReflection.SerializableBiFunction;
import com.fluxtion.runtime.partition.LambdaReflection.SerializableConsumer;
import com.fluxtion.runtime.partition.LambdaReflection.SerializableFunction;
import com.fluxtion.runtime.partition.LambdaReflection.SerializableSupplier;
public abstract class AbstractFlowBuilder> {
final TriggeredFlowFunction eventStream;
public AbstractFlowBuilder(TriggeredFlowFunction eventStream) {
this.eventStream = eventStream;
}
protected abstract B connect(TriggeredFlowFunction stream);
public B parallel() {
eventStream.parallel();
return identity();
}
protected abstract AbstractFlowBuilder connectMap(TriggeredFlowFunction stream);
protected abstract B identity();
//TRIGGERS - START
public B updateTrigger(Object updateTrigger) {
eventStream.setUpdateTriggerNode(StreamHelper.getSource(updateTrigger));
return identity();
}
public B updateTrigger(Object... publishTrigger) {
eventStream.setUpdateTriggerNode(PredicateBuilder.anyTriggered(publishTrigger));
return identity();
}
public B publishTrigger(Object publishTrigger) {
eventStream.setPublishTriggerNode(StreamHelper.getSource(publishTrigger));
return identity();
}
public B publishTrigger(Object... publishTrigger) {
eventStream.setPublishTriggerNode(PredicateBuilder.anyTriggered(publishTrigger));
return identity();
}
public B publishTriggerOverride(Object publishTrigger) {
eventStream.setPublishTriggerOverrideNode(StreamHelper.getSource(publishTrigger));
return identity();
}
public B publishTriggerOverride(Object... publishTrigger) {
eventStream.setPublishTriggerOverrideNode(PredicateBuilder.anyTriggered(publishTrigger));
return identity();
}
public B resetTrigger(Object resetTrigger) {
eventStream.setResetTriggerNode(StreamHelper.getSource(resetTrigger));
return identity();
}
public B resetTrigger(Object... publishTrigger) {
eventStream.setResetTriggerNode(PredicateBuilder.anyTriggered(publishTrigger));
return identity();
}
//FILTERS - START
public B filter(SerializableFunction filterFunction) {
return connect(new FilterFlowFunction<>(eventStream, filterFunction));
}
public B filter(SerializableSupplier filterFunction) {
return filter(new PredicateWrapper(filterFunction)::test);
}
public B filterByProperty(SerializableFunction accessor, SerializableFunction filterFunction) {
return connect(new FilterByPropertyFlowFunction<>(eventStream, accessor, filterFunction));
}
public B filter(
SerializableBiFunction predicate,
FlowBuilder secondArgument) {
return connect(
new FilterDynamicFlowFunction<>(eventStream, secondArgument.eventStream, predicate));
}
public B filter(
SerializableBiFunction predicate,
IntFlowBuilder secondArgument) {
return connect(
new FilterDynamicFlowFunction<>(eventStream, secondArgument.eventStream, predicate));
}
public B filter(
SerializableBiFunction predicate,
DoubleFlowBuilder secondArgument) {
return connect(
new FilterDynamicFlowFunction<>(eventStream, secondArgument.eventStream, predicate));
}
public B filter(
SerializableBiFunction predicate,
LongFlowBuilder secondArgument) {
return connect(
new FilterDynamicFlowFunction<>(eventStream, secondArgument.eventStream, predicate));
}
public B filterByProperty(
SerializableBiFunction
predicate,
SerializableFunction accessor,
FlowBuilder secondArgument) {
return connect(
new FilterByPropertyDynamicFlowFunction<>(eventStream, accessor, secondArgument.eventStream, predicate));
}
public B filterByProperty(
SerializableBiFunction
predicate,
SerializableFunction accessor,
IntFlowBuilder secondArgument) {
return connect(
new FilterByPropertyDynamicFlowFunction<>(eventStream, accessor, secondArgument.eventStream, predicate));
}
public B filterByProperty(
SerializableBiFunction
predicate,
SerializableFunction accessor,
DoubleFlowBuilder secondArgument) {
return connect(
new FilterByPropertyDynamicFlowFunction<>(eventStream, accessor, secondArgument.eventStream, predicate));
}
public B filterByProperty(
SerializableBiFunction
predicate,
SerializableFunction accessor,
LongFlowBuilder secondArgument) {
return connect(
new FilterByPropertyDynamicFlowFunction<>(eventStream, accessor, secondArgument.eventStream, predicate));
}
//MAPPING
protected E mapOnNotifyBase(R target) {
return (E) connectMap(new MapOnNotifyFlowFunction<>(eventStream, target));
}
protected E mapBase(SerializableFunction mapFunction) {
return (E) connectMap(new MapRef2RefFlowFunction<>(eventStream, mapFunction));
}
//MAP TO PRIMITIVES
//OUTPUTS - START
@SafeVarargs
public final B push(SerializableConsumer... pushFunctions) {
B target = null;
for (SerializableConsumer pushFunction : pushFunctions) {
target = connect(new PushFlowFunction<>(eventStream, pushFunction));
}
return target;
}
public B sink(String sinkId) {
return push(new SinkPublisher<>(sinkId)::publish);
}
public B notify(Object target) {
EventProcessorBuilderService.service().add(target);
return connect(new NotifyFlowFunction<>(eventStream, target));
}
public B processAsNewGraphEvent() {
return connect(new PeekFlowFunction<>(eventStream, new InternalEventDispatcher()::dispatchToGraph));
}
public B peek(SerializableConsumer peekFunction) {
return connect(new PeekFlowFunction<>(eventStream, peekFunction));
}
public B console(String in, SerializableFunction transformFunction) {
peek(Peekers.console(in, transformFunction));
return identity();
}
public B console(String in) {
return console(in, null);
}
public B console() {
return console("{}");
}
public IntFlowBuilder mapToInt(LambdaReflection.SerializableToIntFunction mapFunction) {
return new IntFlowBuilder(new MapRef2ToIntFlowFunction<>(eventStream, mapFunction));
}
public DoubleFlowBuilder mapToDouble(LambdaReflection.SerializableToDoubleFunction mapFunction) {
return new DoubleFlowBuilder(new MapRef2ToDoubleFlowFunction<>(eventStream, mapFunction));
}
public LongFlowBuilder mapToLong(LambdaReflection.SerializableToLongFunction mapFunction) {
return new LongFlowBuilder(new MapRef2ToLongFlowFunction<>(eventStream, mapFunction));
}
//META-DATA
public B id(String nodeId) {
EventProcessorBuilderService.service().add(eventStream, nodeId);
return identity();
}
}