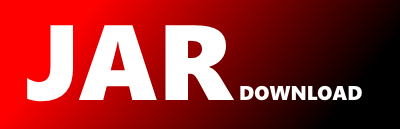
com.sforce.soap.metadata.CustomApplicationComponent Maven / Gradle / Ivy
package com.sforce.soap.metadata;
/**
* This is a generated class for the SObject Enterprise API.
* Do not edit this file, as your changes will be lost.
*/
public class CustomApplicationComponent extends com.sforce.soap.metadata.Metadata {
/**
* Constructor
*/
public CustomApplicationComponent() {}
/* Cache the typeInfo instead of declaring static fields throughout*/
private transient java.util.Map typeInfoCache = new java.util.HashMap();
private com.sforce.ws.bind.TypeInfo _lookupTypeInfo(String fieldName, String namespace, String name, String typeNS, String type, int minOcc, int maxOcc, boolean elementForm) {
com.sforce.ws.bind.TypeInfo typeInfo = typeInfoCache.get(fieldName);
if (typeInfo == null) {
typeInfo = new com.sforce.ws.bind.TypeInfo(namespace, name, typeNS, type, minOcc, maxOcc, elementForm);
typeInfoCache.put(fieldName, typeInfo);
}
return typeInfo;
}
/**
* element : buttonIconUrl of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean buttonIconUrl__is_set = false;
private java.lang.String buttonIconUrl;
public java.lang.String getButtonIconUrl() {
return buttonIconUrl;
}
public void setButtonIconUrl(java.lang.String buttonIconUrl) {
this.buttonIconUrl = buttonIconUrl;
buttonIconUrl__is_set = true;
}
protected void setButtonIconUrl(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("buttonIconUrl", "http://soap.sforce.com/2006/04/metadata","buttonIconUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setButtonIconUrl(__typeMapper.readString(__in, _lookupTypeInfo("buttonIconUrl", "http://soap.sforce.com/2006/04/metadata","buttonIconUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldButtonIconUrl(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("buttonIconUrl", "http://soap.sforce.com/2006/04/metadata","buttonIconUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), buttonIconUrl, buttonIconUrl__is_set);
}
/**
* element : buttonStyle of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean buttonStyle__is_set = false;
private java.lang.String buttonStyle;
public java.lang.String getButtonStyle() {
return buttonStyle;
}
public void setButtonStyle(java.lang.String buttonStyle) {
this.buttonStyle = buttonStyle;
buttonStyle__is_set = true;
}
protected void setButtonStyle(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("buttonStyle", "http://soap.sforce.com/2006/04/metadata","buttonStyle","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setButtonStyle(__typeMapper.readString(__in, _lookupTypeInfo("buttonStyle", "http://soap.sforce.com/2006/04/metadata","buttonStyle","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldButtonStyle(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("buttonStyle", "http://soap.sforce.com/2006/04/metadata","buttonStyle","http://www.w3.org/2001/XMLSchema","string",0,1,true), buttonStyle, buttonStyle__is_set);
}
/**
* element : buttonText of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean buttonText__is_set = false;
private java.lang.String buttonText;
public java.lang.String getButtonText() {
return buttonText;
}
public void setButtonText(java.lang.String buttonText) {
this.buttonText = buttonText;
buttonText__is_set = true;
}
protected void setButtonText(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("buttonText", "http://soap.sforce.com/2006/04/metadata","buttonText","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setButtonText(__typeMapper.readString(__in, _lookupTypeInfo("buttonText", "http://soap.sforce.com/2006/04/metadata","buttonText","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldButtonText(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("buttonText", "http://soap.sforce.com/2006/04/metadata","buttonText","http://www.w3.org/2001/XMLSchema","string",0,1,true), buttonText, buttonText__is_set);
}
/**
* element : buttonWidth of type {http://www.w3.org/2001/XMLSchema}int
* java type: int
*/
private boolean buttonWidth__is_set = false;
private int buttonWidth;
public int getButtonWidth() {
return buttonWidth;
}
public void setButtonWidth(int buttonWidth) {
this.buttonWidth = buttonWidth;
buttonWidth__is_set = true;
}
protected void setButtonWidth(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("buttonWidth", "http://soap.sforce.com/2006/04/metadata","buttonWidth","http://www.w3.org/2001/XMLSchema","int",0,1,true))) {
setButtonWidth((int)__typeMapper.readInt(__in, _lookupTypeInfo("buttonWidth", "http://soap.sforce.com/2006/04/metadata","buttonWidth","http://www.w3.org/2001/XMLSchema","int",0,1,true), int.class));
}
}
private void writeFieldButtonWidth(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("buttonWidth", "http://soap.sforce.com/2006/04/metadata","buttonWidth","http://www.w3.org/2001/XMLSchema","int",0,1,true), buttonWidth, buttonWidth__is_set);
}
/**
* element : height of type {http://www.w3.org/2001/XMLSchema}int
* java type: int
*/
private boolean height__is_set = false;
private int height;
public int getHeight() {
return height;
}
public void setHeight(int height) {
this.height = height;
height__is_set = true;
}
protected void setHeight(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("height", "http://soap.sforce.com/2006/04/metadata","height","http://www.w3.org/2001/XMLSchema","int",0,1,true))) {
setHeight((int)__typeMapper.readInt(__in, _lookupTypeInfo("height", "http://soap.sforce.com/2006/04/metadata","height","http://www.w3.org/2001/XMLSchema","int",0,1,true), int.class));
}
}
private void writeFieldHeight(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("height", "http://soap.sforce.com/2006/04/metadata","height","http://www.w3.org/2001/XMLSchema","int",0,1,true), height, height__is_set);
}
/**
* element : isHeightFixed of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean isHeightFixed__is_set = false;
private boolean isHeightFixed;
public boolean getIsHeightFixed() {
return isHeightFixed;
}
public boolean isIsHeightFixed() {
return isHeightFixed;
}
public void setIsHeightFixed(boolean isHeightFixed) {
this.isHeightFixed = isHeightFixed;
isHeightFixed__is_set = true;
}
protected void setIsHeightFixed(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("isHeightFixed", "http://soap.sforce.com/2006/04/metadata","isHeightFixed","http://www.w3.org/2001/XMLSchema","boolean",1,1,true))) {
setIsHeightFixed(__typeMapper.readBoolean(__in, _lookupTypeInfo("isHeightFixed", "http://soap.sforce.com/2006/04/metadata","isHeightFixed","http://www.w3.org/2001/XMLSchema","boolean",1,1,true), boolean.class));
}
}
private void writeFieldIsHeightFixed(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("isHeightFixed", "http://soap.sforce.com/2006/04/metadata","isHeightFixed","http://www.w3.org/2001/XMLSchema","boolean",1,1,true), isHeightFixed, isHeightFixed__is_set);
}
/**
* element : isHidden of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean isHidden__is_set = false;
private boolean isHidden;
public boolean getIsHidden() {
return isHidden;
}
public boolean isIsHidden() {
return isHidden;
}
public void setIsHidden(boolean isHidden) {
this.isHidden = isHidden;
isHidden__is_set = true;
}
protected void setIsHidden(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("isHidden", "http://soap.sforce.com/2006/04/metadata","isHidden","http://www.w3.org/2001/XMLSchema","boolean",1,1,true))) {
setIsHidden(__typeMapper.readBoolean(__in, _lookupTypeInfo("isHidden", "http://soap.sforce.com/2006/04/metadata","isHidden","http://www.w3.org/2001/XMLSchema","boolean",1,1,true), boolean.class));
}
}
private void writeFieldIsHidden(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("isHidden", "http://soap.sforce.com/2006/04/metadata","isHidden","http://www.w3.org/2001/XMLSchema","boolean",1,1,true), isHidden, isHidden__is_set);
}
/**
* element : isWidthFixed of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean isWidthFixed__is_set = false;
private boolean isWidthFixed;
public boolean getIsWidthFixed() {
return isWidthFixed;
}
public boolean isIsWidthFixed() {
return isWidthFixed;
}
public void setIsWidthFixed(boolean isWidthFixed) {
this.isWidthFixed = isWidthFixed;
isWidthFixed__is_set = true;
}
protected void setIsWidthFixed(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("isWidthFixed", "http://soap.sforce.com/2006/04/metadata","isWidthFixed","http://www.w3.org/2001/XMLSchema","boolean",1,1,true))) {
setIsWidthFixed(__typeMapper.readBoolean(__in, _lookupTypeInfo("isWidthFixed", "http://soap.sforce.com/2006/04/metadata","isWidthFixed","http://www.w3.org/2001/XMLSchema","boolean",1,1,true), boolean.class));
}
}
private void writeFieldIsWidthFixed(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("isWidthFixed", "http://soap.sforce.com/2006/04/metadata","isWidthFixed","http://www.w3.org/2001/XMLSchema","boolean",1,1,true), isWidthFixed, isWidthFixed__is_set);
}
/**
* element : visualforcePage of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean visualforcePage__is_set = false;
private java.lang.String visualforcePage;
public java.lang.String getVisualforcePage() {
return visualforcePage;
}
public void setVisualforcePage(java.lang.String visualforcePage) {
this.visualforcePage = visualforcePage;
visualforcePage__is_set = true;
}
protected void setVisualforcePage(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("visualforcePage", "http://soap.sforce.com/2006/04/metadata","visualforcePage","http://www.w3.org/2001/XMLSchema","string",1,1,true))) {
setVisualforcePage(__typeMapper.readString(__in, _lookupTypeInfo("visualforcePage", "http://soap.sforce.com/2006/04/metadata","visualforcePage","http://www.w3.org/2001/XMLSchema","string",1,1,true), java.lang.String.class));
}
}
private void writeFieldVisualforcePage(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("visualforcePage", "http://soap.sforce.com/2006/04/metadata","visualforcePage","http://www.w3.org/2001/XMLSchema","string",1,1,true), visualforcePage, visualforcePage__is_set);
}
/**
* element : width of type {http://www.w3.org/2001/XMLSchema}int
* java type: int
*/
private boolean width__is_set = false;
private int width;
public int getWidth() {
return width;
}
public void setWidth(int width) {
this.width = width;
width__is_set = true;
}
protected void setWidth(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("width", "http://soap.sforce.com/2006/04/metadata","width","http://www.w3.org/2001/XMLSchema","int",0,1,true))) {
setWidth((int)__typeMapper.readInt(__in, _lookupTypeInfo("width", "http://soap.sforce.com/2006/04/metadata","width","http://www.w3.org/2001/XMLSchema","int",0,1,true), int.class));
}
}
private void writeFieldWidth(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("width", "http://soap.sforce.com/2006/04/metadata","width","http://www.w3.org/2001/XMLSchema","int",0,1,true), width, width__is_set);
}
/**
*/
@Override
public void write(javax.xml.namespace.QName __element,
com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
__out.writeStartTag(__element.getNamespaceURI(), __element.getLocalPart());
__typeMapper.writeXsiType(__out, "http://soap.sforce.com/2006/04/metadata", "CustomApplicationComponent");
writeFields(__out, __typeMapper);
__out.writeEndTag(__element.getNamespaceURI(), __element.getLocalPart());
}
protected void writeFields(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
super.writeFields(__out, __typeMapper);
writeFields1(__out, __typeMapper);
}
@Override
public void load(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__typeMapper.consumeStartTag(__in);
loadFields(__in, __typeMapper);
__typeMapper.consumeEndTag(__in);
}
protected void loadFields(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
super.loadFields(__in, __typeMapper);
loadFields1(__in, __typeMapper);
}
@Override
public String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("[CustomApplicationComponent ");
sb.append(super.toString());
toString1(sb);
sb.append("]\n");
return sb.toString();
}
private void toStringHelper(StringBuilder sb, String name, Object value) {
sb.append(' ').append(name).append("='").append(com.sforce.ws.util.Verbose.toString(value)).append("'\n");
}
private void writeFields1(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
writeFieldButtonIconUrl(__out, __typeMapper);
writeFieldButtonStyle(__out, __typeMapper);
writeFieldButtonText(__out, __typeMapper);
writeFieldButtonWidth(__out, __typeMapper);
writeFieldHeight(__out, __typeMapper);
writeFieldIsHeightFixed(__out, __typeMapper);
writeFieldIsHidden(__out, __typeMapper);
writeFieldIsWidthFixed(__out, __typeMapper);
writeFieldVisualforcePage(__out, __typeMapper);
writeFieldWidth(__out, __typeMapper);
}
private void loadFields1(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
setButtonIconUrl(__in, __typeMapper);
setButtonStyle(__in, __typeMapper);
setButtonText(__in, __typeMapper);
setButtonWidth(__in, __typeMapper);
setHeight(__in, __typeMapper);
setIsHeightFixed(__in, __typeMapper);
setIsHidden(__in, __typeMapper);
setIsWidthFixed(__in, __typeMapper);
setVisualforcePage(__in, __typeMapper);
setWidth(__in, __typeMapper);
}
private void toString1(StringBuilder sb) {
toStringHelper(sb, "buttonIconUrl", buttonIconUrl);
toStringHelper(sb, "buttonStyle", buttonStyle);
toStringHelper(sb, "buttonText", buttonText);
toStringHelper(sb, "buttonWidth", buttonWidth);
toStringHelper(sb, "height", height);
toStringHelper(sb, "isHeightFixed", isHeightFixed);
toStringHelper(sb, "isHidden", isHidden);
toStringHelper(sb, "isWidthFixed", isWidthFixed);
toStringHelper(sb, "visualforcePage", visualforcePage);
toStringHelper(sb, "width", width);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy