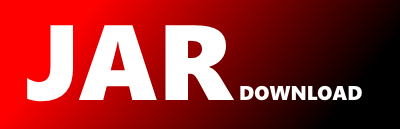
com.sforce.soap.metadata.FlowSettings Maven / Gradle / Ivy
package com.sforce.soap.metadata;
/**
* This is a generated class for the SObject Enterprise API.
* Do not edit this file, as your changes will be lost.
*/
public class FlowSettings extends com.sforce.soap.metadata.Metadata {
/**
* Constructor
*/
public FlowSettings() {}
/* Cache the typeInfo instead of declaring static fields throughout*/
private transient java.util.Map typeInfoCache = new java.util.HashMap();
private com.sforce.ws.bind.TypeInfo _lookupTypeInfo(String fieldName, String namespace, String name, String typeNS, String type, int minOcc, int maxOcc, boolean elementForm) {
com.sforce.ws.bind.TypeInfo typeInfo = typeInfoCache.get(fieldName);
if (typeInfo == null) {
typeInfo = new com.sforce.ws.bind.TypeInfo(namespace, name, typeNS, type, minOcc, maxOcc, elementForm);
typeInfoCache.put(fieldName, typeInfo);
}
return typeInfo;
}
/**
* element : canDebugFlowAsAnotherUser of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean canDebugFlowAsAnotherUser__is_set = false;
private boolean canDebugFlowAsAnotherUser;
public boolean getCanDebugFlowAsAnotherUser() {
return canDebugFlowAsAnotherUser;
}
public boolean isCanDebugFlowAsAnotherUser() {
return canDebugFlowAsAnotherUser;
}
public void setCanDebugFlowAsAnotherUser(boolean canDebugFlowAsAnotherUser) {
this.canDebugFlowAsAnotherUser = canDebugFlowAsAnotherUser;
canDebugFlowAsAnotherUser__is_set = true;
}
protected void setCanDebugFlowAsAnotherUser(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("canDebugFlowAsAnotherUser", "http://soap.sforce.com/2006/04/metadata","canDebugFlowAsAnotherUser","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setCanDebugFlowAsAnotherUser(__typeMapper.readBoolean(__in, _lookupTypeInfo("canDebugFlowAsAnotherUser", "http://soap.sforce.com/2006/04/metadata","canDebugFlowAsAnotherUser","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldCanDebugFlowAsAnotherUser(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("canDebugFlowAsAnotherUser", "http://soap.sforce.com/2006/04/metadata","canDebugFlowAsAnotherUser","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), canDebugFlowAsAnotherUser, canDebugFlowAsAnotherUser__is_set);
}
/**
* element : doesEnforceApexCpuTimeLimit of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean doesEnforceApexCpuTimeLimit__is_set = false;
private boolean doesEnforceApexCpuTimeLimit;
public boolean getDoesEnforceApexCpuTimeLimit() {
return doesEnforceApexCpuTimeLimit;
}
public boolean isDoesEnforceApexCpuTimeLimit() {
return doesEnforceApexCpuTimeLimit;
}
public void setDoesEnforceApexCpuTimeLimit(boolean doesEnforceApexCpuTimeLimit) {
this.doesEnforceApexCpuTimeLimit = doesEnforceApexCpuTimeLimit;
doesEnforceApexCpuTimeLimit__is_set = true;
}
protected void setDoesEnforceApexCpuTimeLimit(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("doesEnforceApexCpuTimeLimit", "http://soap.sforce.com/2006/04/metadata","doesEnforceApexCpuTimeLimit","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setDoesEnforceApexCpuTimeLimit(__typeMapper.readBoolean(__in, _lookupTypeInfo("doesEnforceApexCpuTimeLimit", "http://soap.sforce.com/2006/04/metadata","doesEnforceApexCpuTimeLimit","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldDoesEnforceApexCpuTimeLimit(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("doesEnforceApexCpuTimeLimit", "http://soap.sforce.com/2006/04/metadata","doesEnforceApexCpuTimeLimit","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), doesEnforceApexCpuTimeLimit, doesEnforceApexCpuTimeLimit__is_set);
}
/**
* element : doesFormulaEnforceDataAccess of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean doesFormulaEnforceDataAccess__is_set = false;
private boolean doesFormulaEnforceDataAccess;
public boolean getDoesFormulaEnforceDataAccess() {
return doesFormulaEnforceDataAccess;
}
public boolean isDoesFormulaEnforceDataAccess() {
return doesFormulaEnforceDataAccess;
}
public void setDoesFormulaEnforceDataAccess(boolean doesFormulaEnforceDataAccess) {
this.doesFormulaEnforceDataAccess = doesFormulaEnforceDataAccess;
doesFormulaEnforceDataAccess__is_set = true;
}
protected void setDoesFormulaEnforceDataAccess(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("doesFormulaEnforceDataAccess", "http://soap.sforce.com/2006/04/metadata","doesFormulaEnforceDataAccess","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setDoesFormulaEnforceDataAccess(__typeMapper.readBoolean(__in, _lookupTypeInfo("doesFormulaEnforceDataAccess", "http://soap.sforce.com/2006/04/metadata","doesFormulaEnforceDataAccess","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldDoesFormulaEnforceDataAccess(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("doesFormulaEnforceDataAccess", "http://soap.sforce.com/2006/04/metadata","doesFormulaEnforceDataAccess","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), doesFormulaEnforceDataAccess, doesFormulaEnforceDataAccess__is_set);
}
/**
* element : doesFormulaGenerateHtmlOutput of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean doesFormulaGenerateHtmlOutput__is_set = false;
private boolean doesFormulaGenerateHtmlOutput;
public boolean getDoesFormulaGenerateHtmlOutput() {
return doesFormulaGenerateHtmlOutput;
}
public boolean isDoesFormulaGenerateHtmlOutput() {
return doesFormulaGenerateHtmlOutput;
}
public void setDoesFormulaGenerateHtmlOutput(boolean doesFormulaGenerateHtmlOutput) {
this.doesFormulaGenerateHtmlOutput = doesFormulaGenerateHtmlOutput;
doesFormulaGenerateHtmlOutput__is_set = true;
}
protected void setDoesFormulaGenerateHtmlOutput(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("doesFormulaGenerateHtmlOutput", "http://soap.sforce.com/2006/04/metadata","doesFormulaGenerateHtmlOutput","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setDoesFormulaGenerateHtmlOutput(__typeMapper.readBoolean(__in, _lookupTypeInfo("doesFormulaGenerateHtmlOutput", "http://soap.sforce.com/2006/04/metadata","doesFormulaGenerateHtmlOutput","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldDoesFormulaGenerateHtmlOutput(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("doesFormulaGenerateHtmlOutput", "http://soap.sforce.com/2006/04/metadata","doesFormulaGenerateHtmlOutput","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), doesFormulaGenerateHtmlOutput, doesFormulaGenerateHtmlOutput__is_set);
}
/**
* element : enableEmailSimpleRespectProfiles of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableEmailSimpleRespectProfiles__is_set = false;
private boolean enableEmailSimpleRespectProfiles;
public boolean getEnableEmailSimpleRespectProfiles() {
return enableEmailSimpleRespectProfiles;
}
public boolean isEnableEmailSimpleRespectProfiles() {
return enableEmailSimpleRespectProfiles;
}
public void setEnableEmailSimpleRespectProfiles(boolean enableEmailSimpleRespectProfiles) {
this.enableEmailSimpleRespectProfiles = enableEmailSimpleRespectProfiles;
enableEmailSimpleRespectProfiles__is_set = true;
}
protected void setEnableEmailSimpleRespectProfiles(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableEmailSimpleRespectProfiles", "http://soap.sforce.com/2006/04/metadata","enableEmailSimpleRespectProfiles","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableEmailSimpleRespectProfiles(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableEmailSimpleRespectProfiles", "http://soap.sforce.com/2006/04/metadata","enableEmailSimpleRespectProfiles","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableEmailSimpleRespectProfiles(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableEmailSimpleRespectProfiles", "http://soap.sforce.com/2006/04/metadata","enableEmailSimpleRespectProfiles","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableEmailSimpleRespectProfiles, enableEmailSimpleRespectProfiles__is_set);
}
/**
* element : enableEmailsimpleSecureProfiles of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableEmailsimpleSecureProfiles__is_set = false;
private boolean enableEmailsimpleSecureProfiles;
public boolean getEnableEmailsimpleSecureProfiles() {
return enableEmailsimpleSecureProfiles;
}
public boolean isEnableEmailsimpleSecureProfiles() {
return enableEmailsimpleSecureProfiles;
}
public void setEnableEmailsimpleSecureProfiles(boolean enableEmailsimpleSecureProfiles) {
this.enableEmailsimpleSecureProfiles = enableEmailsimpleSecureProfiles;
enableEmailsimpleSecureProfiles__is_set = true;
}
protected void setEnableEmailsimpleSecureProfiles(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableEmailsimpleSecureProfiles", "http://soap.sforce.com/2006/04/metadata","enableEmailsimpleSecureProfiles","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableEmailsimpleSecureProfiles(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableEmailsimpleSecureProfiles", "http://soap.sforce.com/2006/04/metadata","enableEmailsimpleSecureProfiles","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableEmailsimpleSecureProfiles(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableEmailsimpleSecureProfiles", "http://soap.sforce.com/2006/04/metadata","enableEmailsimpleSecureProfiles","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableEmailsimpleSecureProfiles, enableEmailsimpleSecureProfiles__is_set);
}
/**
* element : enableFlowBREncodedFixEnabled of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowBREncodedFixEnabled__is_set = false;
private boolean enableFlowBREncodedFixEnabled;
public boolean getEnableFlowBREncodedFixEnabled() {
return enableFlowBREncodedFixEnabled;
}
public boolean isEnableFlowBREncodedFixEnabled() {
return enableFlowBREncodedFixEnabled;
}
public void setEnableFlowBREncodedFixEnabled(boolean enableFlowBREncodedFixEnabled) {
this.enableFlowBREncodedFixEnabled = enableFlowBREncodedFixEnabled;
enableFlowBREncodedFixEnabled__is_set = true;
}
protected void setEnableFlowBREncodedFixEnabled(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowBREncodedFixEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowBREncodedFixEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowBREncodedFixEnabled(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowBREncodedFixEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowBREncodedFixEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowBREncodedFixEnabled(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowBREncodedFixEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowBREncodedFixEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowBREncodedFixEnabled, enableFlowBREncodedFixEnabled__is_set);
}
/**
* element : enableFlowCustomPropertyEditor of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowCustomPropertyEditor__is_set = false;
private boolean enableFlowCustomPropertyEditor;
public boolean getEnableFlowCustomPropertyEditor() {
return enableFlowCustomPropertyEditor;
}
public boolean isEnableFlowCustomPropertyEditor() {
return enableFlowCustomPropertyEditor;
}
public void setEnableFlowCustomPropertyEditor(boolean enableFlowCustomPropertyEditor) {
this.enableFlowCustomPropertyEditor = enableFlowCustomPropertyEditor;
enableFlowCustomPropertyEditor__is_set = true;
}
protected void setEnableFlowCustomPropertyEditor(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowCustomPropertyEditor", "http://soap.sforce.com/2006/04/metadata","enableFlowCustomPropertyEditor","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowCustomPropertyEditor(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowCustomPropertyEditor", "http://soap.sforce.com/2006/04/metadata","enableFlowCustomPropertyEditor","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowCustomPropertyEditor(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowCustomPropertyEditor", "http://soap.sforce.com/2006/04/metadata","enableFlowCustomPropertyEditor","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowCustomPropertyEditor, enableFlowCustomPropertyEditor__is_set);
}
/**
* element : enableFlowDeployAsActiveEnabled of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowDeployAsActiveEnabled__is_set = false;
private boolean enableFlowDeployAsActiveEnabled;
public boolean getEnableFlowDeployAsActiveEnabled() {
return enableFlowDeployAsActiveEnabled;
}
public boolean isEnableFlowDeployAsActiveEnabled() {
return enableFlowDeployAsActiveEnabled;
}
public void setEnableFlowDeployAsActiveEnabled(boolean enableFlowDeployAsActiveEnabled) {
this.enableFlowDeployAsActiveEnabled = enableFlowDeployAsActiveEnabled;
enableFlowDeployAsActiveEnabled__is_set = true;
}
protected void setEnableFlowDeployAsActiveEnabled(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowDeployAsActiveEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowDeployAsActiveEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowDeployAsActiveEnabled(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowDeployAsActiveEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowDeployAsActiveEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowDeployAsActiveEnabled(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowDeployAsActiveEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowDeployAsActiveEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowDeployAsActiveEnabled, enableFlowDeployAsActiveEnabled__is_set);
}
/**
* element : enableFlowFieldFilterEnabled of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowFieldFilterEnabled__is_set = false;
private boolean enableFlowFieldFilterEnabled;
public boolean getEnableFlowFieldFilterEnabled() {
return enableFlowFieldFilterEnabled;
}
public boolean isEnableFlowFieldFilterEnabled() {
return enableFlowFieldFilterEnabled;
}
public void setEnableFlowFieldFilterEnabled(boolean enableFlowFieldFilterEnabled) {
this.enableFlowFieldFilterEnabled = enableFlowFieldFilterEnabled;
enableFlowFieldFilterEnabled__is_set = true;
}
protected void setEnableFlowFieldFilterEnabled(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowFieldFilterEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowFieldFilterEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowFieldFilterEnabled(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowFieldFilterEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowFieldFilterEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowFieldFilterEnabled(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowFieldFilterEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowFieldFilterEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowFieldFilterEnabled, enableFlowFieldFilterEnabled__is_set);
}
/**
* element : enableFlowFormulasFixEnabled of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowFormulasFixEnabled__is_set = false;
private boolean enableFlowFormulasFixEnabled;
public boolean getEnableFlowFormulasFixEnabled() {
return enableFlowFormulasFixEnabled;
}
public boolean isEnableFlowFormulasFixEnabled() {
return enableFlowFormulasFixEnabled;
}
public void setEnableFlowFormulasFixEnabled(boolean enableFlowFormulasFixEnabled) {
this.enableFlowFormulasFixEnabled = enableFlowFormulasFixEnabled;
enableFlowFormulasFixEnabled__is_set = true;
}
protected void setEnableFlowFormulasFixEnabled(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowFormulasFixEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowFormulasFixEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowFormulasFixEnabled(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowFormulasFixEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowFormulasFixEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowFormulasFixEnabled(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowFormulasFixEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowFormulasFixEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowFormulasFixEnabled, enableFlowFormulasFixEnabled__is_set);
}
/**
* element : enableFlowInterviewSharingEnabled of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowInterviewSharingEnabled__is_set = false;
private boolean enableFlowInterviewSharingEnabled;
public boolean getEnableFlowInterviewSharingEnabled() {
return enableFlowInterviewSharingEnabled;
}
public boolean isEnableFlowInterviewSharingEnabled() {
return enableFlowInterviewSharingEnabled;
}
public void setEnableFlowInterviewSharingEnabled(boolean enableFlowInterviewSharingEnabled) {
this.enableFlowInterviewSharingEnabled = enableFlowInterviewSharingEnabled;
enableFlowInterviewSharingEnabled__is_set = true;
}
protected void setEnableFlowInterviewSharingEnabled(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowInterviewSharingEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowInterviewSharingEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowInterviewSharingEnabled(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowInterviewSharingEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowInterviewSharingEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowInterviewSharingEnabled(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowInterviewSharingEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowInterviewSharingEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowInterviewSharingEnabled, enableFlowInterviewSharingEnabled__is_set);
}
/**
* element : enableFlowNullPreviousValueFix of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowNullPreviousValueFix__is_set = false;
private boolean enableFlowNullPreviousValueFix;
public boolean getEnableFlowNullPreviousValueFix() {
return enableFlowNullPreviousValueFix;
}
public boolean isEnableFlowNullPreviousValueFix() {
return enableFlowNullPreviousValueFix;
}
public void setEnableFlowNullPreviousValueFix(boolean enableFlowNullPreviousValueFix) {
this.enableFlowNullPreviousValueFix = enableFlowNullPreviousValueFix;
enableFlowNullPreviousValueFix__is_set = true;
}
protected void setEnableFlowNullPreviousValueFix(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowNullPreviousValueFix", "http://soap.sforce.com/2006/04/metadata","enableFlowNullPreviousValueFix","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowNullPreviousValueFix(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowNullPreviousValueFix", "http://soap.sforce.com/2006/04/metadata","enableFlowNullPreviousValueFix","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowNullPreviousValueFix(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowNullPreviousValueFix", "http://soap.sforce.com/2006/04/metadata","enableFlowNullPreviousValueFix","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowNullPreviousValueFix, enableFlowNullPreviousValueFix__is_set);
}
/**
* element : enableFlowPauseEnabled of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowPauseEnabled__is_set = false;
private boolean enableFlowPauseEnabled;
public boolean getEnableFlowPauseEnabled() {
return enableFlowPauseEnabled;
}
public boolean isEnableFlowPauseEnabled() {
return enableFlowPauseEnabled;
}
public void setEnableFlowPauseEnabled(boolean enableFlowPauseEnabled) {
this.enableFlowPauseEnabled = enableFlowPauseEnabled;
enableFlowPauseEnabled__is_set = true;
}
protected void setEnableFlowPauseEnabled(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowPauseEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowPauseEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowPauseEnabled(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowPauseEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowPauseEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowPauseEnabled(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowPauseEnabled", "http://soap.sforce.com/2006/04/metadata","enableFlowPauseEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowPauseEnabled, enableFlowPauseEnabled__is_set);
}
/**
* element : enableFlowReactiveChoiceOptions of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowReactiveChoiceOptions__is_set = false;
private boolean enableFlowReactiveChoiceOptions;
public boolean getEnableFlowReactiveChoiceOptions() {
return enableFlowReactiveChoiceOptions;
}
public boolean isEnableFlowReactiveChoiceOptions() {
return enableFlowReactiveChoiceOptions;
}
public void setEnableFlowReactiveChoiceOptions(boolean enableFlowReactiveChoiceOptions) {
this.enableFlowReactiveChoiceOptions = enableFlowReactiveChoiceOptions;
enableFlowReactiveChoiceOptions__is_set = true;
}
protected void setEnableFlowReactiveChoiceOptions(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowReactiveChoiceOptions", "http://soap.sforce.com/2006/04/metadata","enableFlowReactiveChoiceOptions","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowReactiveChoiceOptions(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowReactiveChoiceOptions", "http://soap.sforce.com/2006/04/metadata","enableFlowReactiveChoiceOptions","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowReactiveChoiceOptions(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowReactiveChoiceOptions", "http://soap.sforce.com/2006/04/metadata","enableFlowReactiveChoiceOptions","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowReactiveChoiceOptions, enableFlowReactiveChoiceOptions__is_set);
}
/**
* element : enableFlowReactiveScreens of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowReactiveScreens__is_set = false;
private boolean enableFlowReactiveScreens;
public boolean getEnableFlowReactiveScreens() {
return enableFlowReactiveScreens;
}
public boolean isEnableFlowReactiveScreens() {
return enableFlowReactiveScreens;
}
public void setEnableFlowReactiveScreens(boolean enableFlowReactiveScreens) {
this.enableFlowReactiveScreens = enableFlowReactiveScreens;
enableFlowReactiveScreens__is_set = true;
}
protected void setEnableFlowReactiveScreens(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowReactiveScreens", "http://soap.sforce.com/2006/04/metadata","enableFlowReactiveScreens","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowReactiveScreens(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowReactiveScreens", "http://soap.sforce.com/2006/04/metadata","enableFlowReactiveScreens","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowReactiveScreens(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowReactiveScreens", "http://soap.sforce.com/2006/04/metadata","enableFlowReactiveScreens","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowReactiveScreens, enableFlowReactiveScreens__is_set);
}
/**
* element : enableFlowUseApexExceptionEmail of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowUseApexExceptionEmail__is_set = false;
private boolean enableFlowUseApexExceptionEmail;
public boolean getEnableFlowUseApexExceptionEmail() {
return enableFlowUseApexExceptionEmail;
}
public boolean isEnableFlowUseApexExceptionEmail() {
return enableFlowUseApexExceptionEmail;
}
public void setEnableFlowUseApexExceptionEmail(boolean enableFlowUseApexExceptionEmail) {
this.enableFlowUseApexExceptionEmail = enableFlowUseApexExceptionEmail;
enableFlowUseApexExceptionEmail__is_set = true;
}
protected void setEnableFlowUseApexExceptionEmail(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowUseApexExceptionEmail", "http://soap.sforce.com/2006/04/metadata","enableFlowUseApexExceptionEmail","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowUseApexExceptionEmail(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowUseApexExceptionEmail", "http://soap.sforce.com/2006/04/metadata","enableFlowUseApexExceptionEmail","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowUseApexExceptionEmail(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowUseApexExceptionEmail", "http://soap.sforce.com/2006/04/metadata","enableFlowUseApexExceptionEmail","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowUseApexExceptionEmail, enableFlowUseApexExceptionEmail__is_set);
}
/**
* element : enableFlowViaRestUsesUserCtxt of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableFlowViaRestUsesUserCtxt__is_set = false;
private boolean enableFlowViaRestUsesUserCtxt;
public boolean getEnableFlowViaRestUsesUserCtxt() {
return enableFlowViaRestUsesUserCtxt;
}
public boolean isEnableFlowViaRestUsesUserCtxt() {
return enableFlowViaRestUsesUserCtxt;
}
public void setEnableFlowViaRestUsesUserCtxt(boolean enableFlowViaRestUsesUserCtxt) {
this.enableFlowViaRestUsesUserCtxt = enableFlowViaRestUsesUserCtxt;
enableFlowViaRestUsesUserCtxt__is_set = true;
}
protected void setEnableFlowViaRestUsesUserCtxt(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableFlowViaRestUsesUserCtxt", "http://soap.sforce.com/2006/04/metadata","enableFlowViaRestUsesUserCtxt","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableFlowViaRestUsesUserCtxt(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableFlowViaRestUsesUserCtxt", "http://soap.sforce.com/2006/04/metadata","enableFlowViaRestUsesUserCtxt","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableFlowViaRestUsesUserCtxt(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableFlowViaRestUsesUserCtxt", "http://soap.sforce.com/2006/04/metadata","enableFlowViaRestUsesUserCtxt","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableFlowViaRestUsesUserCtxt, enableFlowViaRestUsesUserCtxt__is_set);
}
/**
* element : enableLightningRuntimeEnabled of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableLightningRuntimeEnabled__is_set = false;
private boolean enableLightningRuntimeEnabled;
public boolean getEnableLightningRuntimeEnabled() {
return enableLightningRuntimeEnabled;
}
public boolean isEnableLightningRuntimeEnabled() {
return enableLightningRuntimeEnabled;
}
public void setEnableLightningRuntimeEnabled(boolean enableLightningRuntimeEnabled) {
this.enableLightningRuntimeEnabled = enableLightningRuntimeEnabled;
enableLightningRuntimeEnabled__is_set = true;
}
protected void setEnableLightningRuntimeEnabled(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableLightningRuntimeEnabled", "http://soap.sforce.com/2006/04/metadata","enableLightningRuntimeEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableLightningRuntimeEnabled(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableLightningRuntimeEnabled", "http://soap.sforce.com/2006/04/metadata","enableLightningRuntimeEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableLightningRuntimeEnabled(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableLightningRuntimeEnabled", "http://soap.sforce.com/2006/04/metadata","enableLightningRuntimeEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableLightningRuntimeEnabled, enableLightningRuntimeEnabled__is_set);
}
/**
* element : isApexPluginAccessModifierRespected of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean isApexPluginAccessModifierRespected__is_set = false;
private boolean isApexPluginAccessModifierRespected;
public boolean getIsApexPluginAccessModifierRespected() {
return isApexPluginAccessModifierRespected;
}
public boolean isIsApexPluginAccessModifierRespected() {
return isApexPluginAccessModifierRespected;
}
public void setIsApexPluginAccessModifierRespected(boolean isApexPluginAccessModifierRespected) {
this.isApexPluginAccessModifierRespected = isApexPluginAccessModifierRespected;
isApexPluginAccessModifierRespected__is_set = true;
}
protected void setIsApexPluginAccessModifierRespected(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("isApexPluginAccessModifierRespected", "http://soap.sforce.com/2006/04/metadata","isApexPluginAccessModifierRespected","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setIsApexPluginAccessModifierRespected(__typeMapper.readBoolean(__in, _lookupTypeInfo("isApexPluginAccessModifierRespected", "http://soap.sforce.com/2006/04/metadata","isApexPluginAccessModifierRespected","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldIsApexPluginAccessModifierRespected(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("isApexPluginAccessModifierRespected", "http://soap.sforce.com/2006/04/metadata","isApexPluginAccessModifierRespected","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), isApexPluginAccessModifierRespected, isApexPluginAccessModifierRespected__is_set);
}
/**
* element : isEnhancedFlowListViewVisible of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean isEnhancedFlowListViewVisible__is_set = false;
private boolean isEnhancedFlowListViewVisible;
public boolean getIsEnhancedFlowListViewVisible() {
return isEnhancedFlowListViewVisible;
}
public boolean isIsEnhancedFlowListViewVisible() {
return isEnhancedFlowListViewVisible;
}
public void setIsEnhancedFlowListViewVisible(boolean isEnhancedFlowListViewVisible) {
this.isEnhancedFlowListViewVisible = isEnhancedFlowListViewVisible;
isEnhancedFlowListViewVisible__is_set = true;
}
protected void setIsEnhancedFlowListViewVisible(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("isEnhancedFlowListViewVisible", "http://soap.sforce.com/2006/04/metadata","isEnhancedFlowListViewVisible","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setIsEnhancedFlowListViewVisible(__typeMapper.readBoolean(__in, _lookupTypeInfo("isEnhancedFlowListViewVisible", "http://soap.sforce.com/2006/04/metadata","isEnhancedFlowListViewVisible","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldIsEnhancedFlowListViewVisible(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("isEnhancedFlowListViewVisible", "http://soap.sforce.com/2006/04/metadata","isEnhancedFlowListViewVisible","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), isEnhancedFlowListViewVisible, isEnhancedFlowListViewVisible__is_set);
}
/**
* element : isFlowBlockAccessToSessionIDEnabled of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean isFlowBlockAccessToSessionIDEnabled__is_set = false;
private boolean isFlowBlockAccessToSessionIDEnabled;
public boolean getIsFlowBlockAccessToSessionIDEnabled() {
return isFlowBlockAccessToSessionIDEnabled;
}
public boolean isIsFlowBlockAccessToSessionIDEnabled() {
return isFlowBlockAccessToSessionIDEnabled;
}
public void setIsFlowBlockAccessToSessionIDEnabled(boolean isFlowBlockAccessToSessionIDEnabled) {
this.isFlowBlockAccessToSessionIDEnabled = isFlowBlockAccessToSessionIDEnabled;
isFlowBlockAccessToSessionIDEnabled__is_set = true;
}
protected void setIsFlowBlockAccessToSessionIDEnabled(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("isFlowBlockAccessToSessionIDEnabled", "http://soap.sforce.com/2006/04/metadata","isFlowBlockAccessToSessionIDEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setIsFlowBlockAccessToSessionIDEnabled(__typeMapper.readBoolean(__in, _lookupTypeInfo("isFlowBlockAccessToSessionIDEnabled", "http://soap.sforce.com/2006/04/metadata","isFlowBlockAccessToSessionIDEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldIsFlowBlockAccessToSessionIDEnabled(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("isFlowBlockAccessToSessionIDEnabled", "http://soap.sforce.com/2006/04/metadata","isFlowBlockAccessToSessionIDEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), isFlowBlockAccessToSessionIDEnabled, isFlowBlockAccessToSessionIDEnabled__is_set);
}
/**
* element : isManageFlowRequiredForAutomationCharts of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean isManageFlowRequiredForAutomationCharts__is_set = false;
private boolean isManageFlowRequiredForAutomationCharts;
public boolean getIsManageFlowRequiredForAutomationCharts() {
return isManageFlowRequiredForAutomationCharts;
}
public boolean isIsManageFlowRequiredForAutomationCharts() {
return isManageFlowRequiredForAutomationCharts;
}
public void setIsManageFlowRequiredForAutomationCharts(boolean isManageFlowRequiredForAutomationCharts) {
this.isManageFlowRequiredForAutomationCharts = isManageFlowRequiredForAutomationCharts;
isManageFlowRequiredForAutomationCharts__is_set = true;
}
protected void setIsManageFlowRequiredForAutomationCharts(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("isManageFlowRequiredForAutomationCharts", "http://soap.sforce.com/2006/04/metadata","isManageFlowRequiredForAutomationCharts","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setIsManageFlowRequiredForAutomationCharts(__typeMapper.readBoolean(__in, _lookupTypeInfo("isManageFlowRequiredForAutomationCharts", "http://soap.sforce.com/2006/04/metadata","isManageFlowRequiredForAutomationCharts","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldIsManageFlowRequiredForAutomationCharts(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("isManageFlowRequiredForAutomationCharts", "http://soap.sforce.com/2006/04/metadata","isManageFlowRequiredForAutomationCharts","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), isManageFlowRequiredForAutomationCharts, isManageFlowRequiredForAutomationCharts__is_set);
}
/**
* element : isSupportRollbackOnErrorForApexInvocableActionsEnabled of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean isSupportRollbackOnErrorForApexInvocableActionsEnabled__is_set = false;
private boolean isSupportRollbackOnErrorForApexInvocableActionsEnabled;
public boolean getIsSupportRollbackOnErrorForApexInvocableActionsEnabled() {
return isSupportRollbackOnErrorForApexInvocableActionsEnabled;
}
public boolean isIsSupportRollbackOnErrorForApexInvocableActionsEnabled() {
return isSupportRollbackOnErrorForApexInvocableActionsEnabled;
}
public void setIsSupportRollbackOnErrorForApexInvocableActionsEnabled(boolean isSupportRollbackOnErrorForApexInvocableActionsEnabled) {
this.isSupportRollbackOnErrorForApexInvocableActionsEnabled = isSupportRollbackOnErrorForApexInvocableActionsEnabled;
isSupportRollbackOnErrorForApexInvocableActionsEnabled__is_set = true;
}
protected void setIsSupportRollbackOnErrorForApexInvocableActionsEnabled(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("isSupportRollbackOnErrorForApexInvocableActionsEnabled", "http://soap.sforce.com/2006/04/metadata","isSupportRollbackOnErrorForApexInvocableActionsEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setIsSupportRollbackOnErrorForApexInvocableActionsEnabled(__typeMapper.readBoolean(__in, _lookupTypeInfo("isSupportRollbackOnErrorForApexInvocableActionsEnabled", "http://soap.sforce.com/2006/04/metadata","isSupportRollbackOnErrorForApexInvocableActionsEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldIsSupportRollbackOnErrorForApexInvocableActionsEnabled(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("isSupportRollbackOnErrorForApexInvocableActionsEnabled", "http://soap.sforce.com/2006/04/metadata","isSupportRollbackOnErrorForApexInvocableActionsEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), isSupportRollbackOnErrorForApexInvocableActionsEnabled, isSupportRollbackOnErrorForApexInvocableActionsEnabled__is_set);
}
/**
*/
@Override
public void write(javax.xml.namespace.QName __element,
com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
__out.writeStartTag(__element.getNamespaceURI(), __element.getLocalPart());
__typeMapper.writeXsiType(__out, "http://soap.sforce.com/2006/04/metadata", "FlowSettings");
writeFields(__out, __typeMapper);
__out.writeEndTag(__element.getNamespaceURI(), __element.getLocalPart());
}
protected void writeFields(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
super.writeFields(__out, __typeMapper);
writeFields1(__out, __typeMapper);
}
@Override
public void load(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__typeMapper.consumeStartTag(__in);
loadFields(__in, __typeMapper);
__typeMapper.consumeEndTag(__in);
}
protected void loadFields(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
super.loadFields(__in, __typeMapper);
loadFields1(__in, __typeMapper);
}
@Override
public String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("[FlowSettings ");
sb.append(super.toString());
toString1(sb);
sb.append("]\n");
return sb.toString();
}
private void toStringHelper(StringBuilder sb, String name, Object value) {
sb.append(' ').append(name).append("='").append(com.sforce.ws.util.Verbose.toString(value)).append("'\n");
}
private void writeFields1(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
writeFieldCanDebugFlowAsAnotherUser(__out, __typeMapper);
writeFieldDoesEnforceApexCpuTimeLimit(__out, __typeMapper);
writeFieldDoesFormulaEnforceDataAccess(__out, __typeMapper);
writeFieldDoesFormulaGenerateHtmlOutput(__out, __typeMapper);
writeFieldEnableEmailSimpleRespectProfiles(__out, __typeMapper);
writeFieldEnableEmailsimpleSecureProfiles(__out, __typeMapper);
writeFieldEnableFlowBREncodedFixEnabled(__out, __typeMapper);
writeFieldEnableFlowCustomPropertyEditor(__out, __typeMapper);
writeFieldEnableFlowDeployAsActiveEnabled(__out, __typeMapper);
writeFieldEnableFlowFieldFilterEnabled(__out, __typeMapper);
writeFieldEnableFlowFormulasFixEnabled(__out, __typeMapper);
writeFieldEnableFlowInterviewSharingEnabled(__out, __typeMapper);
writeFieldEnableFlowNullPreviousValueFix(__out, __typeMapper);
writeFieldEnableFlowPauseEnabled(__out, __typeMapper);
writeFieldEnableFlowReactiveChoiceOptions(__out, __typeMapper);
writeFieldEnableFlowReactiveScreens(__out, __typeMapper);
writeFieldEnableFlowUseApexExceptionEmail(__out, __typeMapper);
writeFieldEnableFlowViaRestUsesUserCtxt(__out, __typeMapper);
writeFieldEnableLightningRuntimeEnabled(__out, __typeMapper);
writeFieldIsApexPluginAccessModifierRespected(__out, __typeMapper);
writeFieldIsEnhancedFlowListViewVisible(__out, __typeMapper);
writeFieldIsFlowBlockAccessToSessionIDEnabled(__out, __typeMapper);
writeFieldIsManageFlowRequiredForAutomationCharts(__out, __typeMapper);
writeFieldIsSupportRollbackOnErrorForApexInvocableActionsEnabled(__out, __typeMapper);
}
private void loadFields1(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
setCanDebugFlowAsAnotherUser(__in, __typeMapper);
setDoesEnforceApexCpuTimeLimit(__in, __typeMapper);
setDoesFormulaEnforceDataAccess(__in, __typeMapper);
setDoesFormulaGenerateHtmlOutput(__in, __typeMapper);
setEnableEmailSimpleRespectProfiles(__in, __typeMapper);
setEnableEmailsimpleSecureProfiles(__in, __typeMapper);
setEnableFlowBREncodedFixEnabled(__in, __typeMapper);
setEnableFlowCustomPropertyEditor(__in, __typeMapper);
setEnableFlowDeployAsActiveEnabled(__in, __typeMapper);
setEnableFlowFieldFilterEnabled(__in, __typeMapper);
setEnableFlowFormulasFixEnabled(__in, __typeMapper);
setEnableFlowInterviewSharingEnabled(__in, __typeMapper);
setEnableFlowNullPreviousValueFix(__in, __typeMapper);
setEnableFlowPauseEnabled(__in, __typeMapper);
setEnableFlowReactiveChoiceOptions(__in, __typeMapper);
setEnableFlowReactiveScreens(__in, __typeMapper);
setEnableFlowUseApexExceptionEmail(__in, __typeMapper);
setEnableFlowViaRestUsesUserCtxt(__in, __typeMapper);
setEnableLightningRuntimeEnabled(__in, __typeMapper);
setIsApexPluginAccessModifierRespected(__in, __typeMapper);
setIsEnhancedFlowListViewVisible(__in, __typeMapper);
setIsFlowBlockAccessToSessionIDEnabled(__in, __typeMapper);
setIsManageFlowRequiredForAutomationCharts(__in, __typeMapper);
setIsSupportRollbackOnErrorForApexInvocableActionsEnabled(__in, __typeMapper);
}
private void toString1(StringBuilder sb) {
toStringHelper(sb, "canDebugFlowAsAnotherUser", canDebugFlowAsAnotherUser);
toStringHelper(sb, "doesEnforceApexCpuTimeLimit", doesEnforceApexCpuTimeLimit);
toStringHelper(sb, "doesFormulaEnforceDataAccess", doesFormulaEnforceDataAccess);
toStringHelper(sb, "doesFormulaGenerateHtmlOutput", doesFormulaGenerateHtmlOutput);
toStringHelper(sb, "enableEmailSimpleRespectProfiles", enableEmailSimpleRespectProfiles);
toStringHelper(sb, "enableEmailsimpleSecureProfiles", enableEmailsimpleSecureProfiles);
toStringHelper(sb, "enableFlowBREncodedFixEnabled", enableFlowBREncodedFixEnabled);
toStringHelper(sb, "enableFlowCustomPropertyEditor", enableFlowCustomPropertyEditor);
toStringHelper(sb, "enableFlowDeployAsActiveEnabled", enableFlowDeployAsActiveEnabled);
toStringHelper(sb, "enableFlowFieldFilterEnabled", enableFlowFieldFilterEnabled);
toStringHelper(sb, "enableFlowFormulasFixEnabled", enableFlowFormulasFixEnabled);
toStringHelper(sb, "enableFlowInterviewSharingEnabled", enableFlowInterviewSharingEnabled);
toStringHelper(sb, "enableFlowNullPreviousValueFix", enableFlowNullPreviousValueFix);
toStringHelper(sb, "enableFlowPauseEnabled", enableFlowPauseEnabled);
toStringHelper(sb, "enableFlowReactiveChoiceOptions", enableFlowReactiveChoiceOptions);
toStringHelper(sb, "enableFlowReactiveScreens", enableFlowReactiveScreens);
toStringHelper(sb, "enableFlowUseApexExceptionEmail", enableFlowUseApexExceptionEmail);
toStringHelper(sb, "enableFlowViaRestUsesUserCtxt", enableFlowViaRestUsesUserCtxt);
toStringHelper(sb, "enableLightningRuntimeEnabled", enableLightningRuntimeEnabled);
toStringHelper(sb, "isApexPluginAccessModifierRespected", isApexPluginAccessModifierRespected);
toStringHelper(sb, "isEnhancedFlowListViewVisible", isEnhancedFlowListViewVisible);
toStringHelper(sb, "isFlowBlockAccessToSessionIDEnabled", isFlowBlockAccessToSessionIDEnabled);
toStringHelper(sb, "isManageFlowRequiredForAutomationCharts", isManageFlowRequiredForAutomationCharts);
toStringHelper(sb, "isSupportRollbackOnErrorForApexInvocableActionsEnabled", isSupportRollbackOnErrorForApexInvocableActionsEnabled);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy