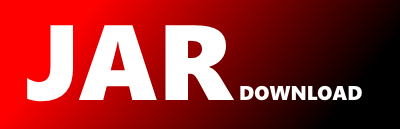
com.sforce.soap.metadata.EACSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of force-metadata-api Show documentation
Show all versions of force-metadata-api Show documentation
Force.com Web Service Connector
The newest version!
package com.sforce.soap.metadata;
/**
* This is a generated class for the SObject Enterprise API.
* Do not edit this file, as your changes will be lost.
*/
public class EACSettings extends com.sforce.soap.metadata.Metadata {
/**
* Constructor
*/
public EACSettings() {}
/* Cache the typeInfo instead of declaring static fields throughout*/
private transient java.util.Map typeInfoCache = new java.util.HashMap();
private com.sforce.ws.bind.TypeInfo _lookupTypeInfo(String fieldName, String namespace, String name, String typeNS, String type, int minOcc, int maxOcc, boolean elementForm) {
com.sforce.ws.bind.TypeInfo typeInfo = typeInfoCache.get(fieldName);
if (typeInfo == null) {
typeInfo = new com.sforce.ws.bind.TypeInfo(namespace, name, typeNS, type, minOcc, maxOcc, elementForm);
typeInfoCache.put(fieldName, typeInfo);
}
return typeInfo;
}
/**
* element : addRcCompToFlexiPages of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean addRcCompToFlexiPages__is_set = false;
private boolean addRcCompToFlexiPages;
public boolean getAddRcCompToFlexiPages() {
return addRcCompToFlexiPages;
}
public boolean isAddRcCompToFlexiPages() {
return addRcCompToFlexiPages;
}
public void setAddRcCompToFlexiPages(boolean addRcCompToFlexiPages) {
this.addRcCompToFlexiPages = addRcCompToFlexiPages;
addRcCompToFlexiPages__is_set = true;
}
protected void setAddRcCompToFlexiPages(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("addRcCompToFlexiPages", "http://soap.sforce.com/2006/04/metadata","addRcCompToFlexiPages","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setAddRcCompToFlexiPages(__typeMapper.readBoolean(__in, _lookupTypeInfo("addRcCompToFlexiPages", "http://soap.sforce.com/2006/04/metadata","addRcCompToFlexiPages","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldAddRcCompToFlexiPages(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("addRcCompToFlexiPages", "http://soap.sforce.com/2006/04/metadata","addRcCompToFlexiPages","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), addRcCompToFlexiPages, addRcCompToFlexiPages__is_set);
}
/**
* element : autoContactCreationPref of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean autoContactCreationPref__is_set = false;
private boolean autoContactCreationPref;
public boolean getAutoContactCreationPref() {
return autoContactCreationPref;
}
public boolean isAutoContactCreationPref() {
return autoContactCreationPref;
}
public void setAutoContactCreationPref(boolean autoContactCreationPref) {
this.autoContactCreationPref = autoContactCreationPref;
autoContactCreationPref__is_set = true;
}
protected void setAutoContactCreationPref(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("autoContactCreationPref", "http://soap.sforce.com/2006/04/metadata","autoContactCreationPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setAutoContactCreationPref(__typeMapper.readBoolean(__in, _lookupTypeInfo("autoContactCreationPref", "http://soap.sforce.com/2006/04/metadata","autoContactCreationPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldAutoContactCreationPref(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("autoContactCreationPref", "http://soap.sforce.com/2006/04/metadata","autoContactCreationPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), autoContactCreationPref, autoContactCreationPref__is_set);
}
/**
* element : autoContactEnrichmentPref of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean autoContactEnrichmentPref__is_set = false;
private boolean autoContactEnrichmentPref;
public boolean getAutoContactEnrichmentPref() {
return autoContactEnrichmentPref;
}
public boolean isAutoContactEnrichmentPref() {
return autoContactEnrichmentPref;
}
public void setAutoContactEnrichmentPref(boolean autoContactEnrichmentPref) {
this.autoContactEnrichmentPref = autoContactEnrichmentPref;
autoContactEnrichmentPref__is_set = true;
}
protected void setAutoContactEnrichmentPref(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("autoContactEnrichmentPref", "http://soap.sforce.com/2006/04/metadata","autoContactEnrichmentPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setAutoContactEnrichmentPref(__typeMapper.readBoolean(__in, _lookupTypeInfo("autoContactEnrichmentPref", "http://soap.sforce.com/2006/04/metadata","autoContactEnrichmentPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldAutoContactEnrichmentPref(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("autoContactEnrichmentPref", "http://soap.sforce.com/2006/04/metadata","autoContactEnrichmentPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), autoContactEnrichmentPref, autoContactEnrichmentPref__is_set);
}
/**
* element : autoPopulateGoogleMeetLinks of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean autoPopulateGoogleMeetLinks__is_set = false;
private boolean autoPopulateGoogleMeetLinks;
public boolean getAutoPopulateGoogleMeetLinks() {
return autoPopulateGoogleMeetLinks;
}
public boolean isAutoPopulateGoogleMeetLinks() {
return autoPopulateGoogleMeetLinks;
}
public void setAutoPopulateGoogleMeetLinks(boolean autoPopulateGoogleMeetLinks) {
this.autoPopulateGoogleMeetLinks = autoPopulateGoogleMeetLinks;
autoPopulateGoogleMeetLinks__is_set = true;
}
protected void setAutoPopulateGoogleMeetLinks(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("autoPopulateGoogleMeetLinks", "http://soap.sforce.com/2006/04/metadata","autoPopulateGoogleMeetLinks","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setAutoPopulateGoogleMeetLinks(__typeMapper.readBoolean(__in, _lookupTypeInfo("autoPopulateGoogleMeetLinks", "http://soap.sforce.com/2006/04/metadata","autoPopulateGoogleMeetLinks","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldAutoPopulateGoogleMeetLinks(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("autoPopulateGoogleMeetLinks", "http://soap.sforce.com/2006/04/metadata","autoPopulateGoogleMeetLinks","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), autoPopulateGoogleMeetLinks, autoPopulateGoogleMeetLinks__is_set);
}
/**
* element : automatedEmailFilter of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean automatedEmailFilter__is_set = false;
private boolean automatedEmailFilter;
public boolean getAutomatedEmailFilter() {
return automatedEmailFilter;
}
public boolean isAutomatedEmailFilter() {
return automatedEmailFilter;
}
public void setAutomatedEmailFilter(boolean automatedEmailFilter) {
this.automatedEmailFilter = automatedEmailFilter;
automatedEmailFilter__is_set = true;
}
protected void setAutomatedEmailFilter(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("automatedEmailFilter", "http://soap.sforce.com/2006/04/metadata","automatedEmailFilter","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setAutomatedEmailFilter(__typeMapper.readBoolean(__in, _lookupTypeInfo("automatedEmailFilter", "http://soap.sforce.com/2006/04/metadata","automatedEmailFilter","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldAutomatedEmailFilter(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("automatedEmailFilter", "http://soap.sforce.com/2006/04/metadata","automatedEmailFilter","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), automatedEmailFilter, automatedEmailFilter__is_set);
}
/**
* element : dSThresholdNotification of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean dSThresholdNotification__is_set = false;
private boolean dSThresholdNotification;
public boolean getDSThresholdNotification() {
return dSThresholdNotification;
}
public boolean isDSThresholdNotification() {
return dSThresholdNotification;
}
public void setDSThresholdNotification(boolean dSThresholdNotification) {
this.dSThresholdNotification = dSThresholdNotification;
dSThresholdNotification__is_set = true;
}
protected void setDSThresholdNotification(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("dSThresholdNotification", "http://soap.sforce.com/2006/04/metadata","dSThresholdNotification","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setDSThresholdNotification(__typeMapper.readBoolean(__in, _lookupTypeInfo("dSThresholdNotification", "http://soap.sforce.com/2006/04/metadata","dSThresholdNotification","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldDSThresholdNotification(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("dSThresholdNotification", "http://soap.sforce.com/2006/04/metadata","dSThresholdNotification","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), dSThresholdNotification, dSThresholdNotification__is_set);
}
/**
* element : enableActivityAnalyticsPref of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableActivityAnalyticsPref__is_set = false;
private boolean enableActivityAnalyticsPref;
public boolean getEnableActivityAnalyticsPref() {
return enableActivityAnalyticsPref;
}
public boolean isEnableActivityAnalyticsPref() {
return enableActivityAnalyticsPref;
}
public void setEnableActivityAnalyticsPref(boolean enableActivityAnalyticsPref) {
this.enableActivityAnalyticsPref = enableActivityAnalyticsPref;
enableActivityAnalyticsPref__is_set = true;
}
protected void setEnableActivityAnalyticsPref(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableActivityAnalyticsPref", "http://soap.sforce.com/2006/04/metadata","enableActivityAnalyticsPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableActivityAnalyticsPref(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableActivityAnalyticsPref", "http://soap.sforce.com/2006/04/metadata","enableActivityAnalyticsPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableActivityAnalyticsPref(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableActivityAnalyticsPref", "http://soap.sforce.com/2006/04/metadata","enableActivityAnalyticsPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableActivityAnalyticsPref, enableActivityAnalyticsPref__is_set);
}
/**
* element : enableActivityCapture of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableActivityCapture__is_set = false;
private boolean enableActivityCapture;
public boolean getEnableActivityCapture() {
return enableActivityCapture;
}
public boolean isEnableActivityCapture() {
return enableActivityCapture;
}
public void setEnableActivityCapture(boolean enableActivityCapture) {
this.enableActivityCapture = enableActivityCapture;
enableActivityCapture__is_set = true;
}
protected void setEnableActivityCapture(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableActivityCapture", "http://soap.sforce.com/2006/04/metadata","enableActivityCapture","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableActivityCapture(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableActivityCapture", "http://soap.sforce.com/2006/04/metadata","enableActivityCapture","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableActivityCapture(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableActivityCapture", "http://soap.sforce.com/2006/04/metadata","enableActivityCapture","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableActivityCapture, enableActivityCapture__is_set);
}
/**
* element : enableActivityMetrics of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableActivityMetrics__is_set = false;
private boolean enableActivityMetrics;
public boolean getEnableActivityMetrics() {
return enableActivityMetrics;
}
public boolean isEnableActivityMetrics() {
return enableActivityMetrics;
}
public void setEnableActivityMetrics(boolean enableActivityMetrics) {
this.enableActivityMetrics = enableActivityMetrics;
enableActivityMetrics__is_set = true;
}
protected void setEnableActivityMetrics(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableActivityMetrics", "http://soap.sforce.com/2006/04/metadata","enableActivityMetrics","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableActivityMetrics(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableActivityMetrics", "http://soap.sforce.com/2006/04/metadata","enableActivityMetrics","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableActivityMetrics(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableActivityMetrics", "http://soap.sforce.com/2006/04/metadata","enableActivityMetrics","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableActivityMetrics, enableActivityMetrics__is_set);
}
/**
* element : enableActivitySyncEngine of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableActivitySyncEngine__is_set = false;
private boolean enableActivitySyncEngine;
public boolean getEnableActivitySyncEngine() {
return enableActivitySyncEngine;
}
public boolean isEnableActivitySyncEngine() {
return enableActivitySyncEngine;
}
public void setEnableActivitySyncEngine(boolean enableActivitySyncEngine) {
this.enableActivitySyncEngine = enableActivitySyncEngine;
enableActivitySyncEngine__is_set = true;
}
protected void setEnableActivitySyncEngine(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableActivitySyncEngine", "http://soap.sforce.com/2006/04/metadata","enableActivitySyncEngine","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableActivitySyncEngine(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableActivitySyncEngine", "http://soap.sforce.com/2006/04/metadata","enableActivitySyncEngine","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableActivitySyncEngine(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableActivitySyncEngine", "http://soap.sforce.com/2006/04/metadata","enableActivitySyncEngine","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableActivitySyncEngine, enableActivitySyncEngine__is_set);
}
/**
* element : enableEACForEveryonePref of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableEACForEveryonePref__is_set = false;
private boolean enableEACForEveryonePref;
public boolean getEnableEACForEveryonePref() {
return enableEACForEveryonePref;
}
public boolean isEnableEACForEveryonePref() {
return enableEACForEveryonePref;
}
public void setEnableEACForEveryonePref(boolean enableEACForEveryonePref) {
this.enableEACForEveryonePref = enableEACForEveryonePref;
enableEACForEveryonePref__is_set = true;
}
protected void setEnableEACForEveryonePref(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableEACForEveryonePref", "http://soap.sforce.com/2006/04/metadata","enableEACForEveryonePref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableEACForEveryonePref(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableEACForEveryonePref", "http://soap.sforce.com/2006/04/metadata","enableEACForEveryonePref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableEACForEveryonePref(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableEACForEveryonePref", "http://soap.sforce.com/2006/04/metadata","enableEACForEveryonePref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableEACForEveryonePref, enableEACForEveryonePref__is_set);
}
/**
* element : enableEnforceEacSharingPref of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableEnforceEacSharingPref__is_set = false;
private boolean enableEnforceEacSharingPref;
public boolean getEnableEnforceEacSharingPref() {
return enableEnforceEacSharingPref;
}
public boolean isEnableEnforceEacSharingPref() {
return enableEnforceEacSharingPref;
}
public void setEnableEnforceEacSharingPref(boolean enableEnforceEacSharingPref) {
this.enableEnforceEacSharingPref = enableEnforceEacSharingPref;
enableEnforceEacSharingPref__is_set = true;
}
protected void setEnableEnforceEacSharingPref(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableEnforceEacSharingPref", "http://soap.sforce.com/2006/04/metadata","enableEnforceEacSharingPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableEnforceEacSharingPref(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableEnforceEacSharingPref", "http://soap.sforce.com/2006/04/metadata","enableEnforceEacSharingPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableEnforceEacSharingPref(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableEnforceEacSharingPref", "http://soap.sforce.com/2006/04/metadata","enableEnforceEacSharingPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableEnforceEacSharingPref, enableEnforceEacSharingPref__is_set);
}
/**
* element : enableInboxActivitySharing of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableInboxActivitySharing__is_set = false;
private boolean enableInboxActivitySharing;
public boolean getEnableInboxActivitySharing() {
return enableInboxActivitySharing;
}
public boolean isEnableInboxActivitySharing() {
return enableInboxActivitySharing;
}
public void setEnableInboxActivitySharing(boolean enableInboxActivitySharing) {
this.enableInboxActivitySharing = enableInboxActivitySharing;
enableInboxActivitySharing__is_set = true;
}
protected void setEnableInboxActivitySharing(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableInboxActivitySharing", "http://soap.sforce.com/2006/04/metadata","enableInboxActivitySharing","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableInboxActivitySharing(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableInboxActivitySharing", "http://soap.sforce.com/2006/04/metadata","enableInboxActivitySharing","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableInboxActivitySharing(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableInboxActivitySharing", "http://soap.sforce.com/2006/04/metadata","enableInboxActivitySharing","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableInboxActivitySharing, enableInboxActivitySharing__is_set);
}
/**
* element : enableInsightsInTimeline of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableInsightsInTimeline__is_set = false;
private boolean enableInsightsInTimeline;
public boolean getEnableInsightsInTimeline() {
return enableInsightsInTimeline;
}
public boolean isEnableInsightsInTimeline() {
return enableInsightsInTimeline;
}
public void setEnableInsightsInTimeline(boolean enableInsightsInTimeline) {
this.enableInsightsInTimeline = enableInsightsInTimeline;
enableInsightsInTimeline__is_set = true;
}
protected void setEnableInsightsInTimeline(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableInsightsInTimeline", "http://soap.sforce.com/2006/04/metadata","enableInsightsInTimeline","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableInsightsInTimeline(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableInsightsInTimeline", "http://soap.sforce.com/2006/04/metadata","enableInsightsInTimeline","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableInsightsInTimeline(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableInsightsInTimeline", "http://soap.sforce.com/2006/04/metadata","enableInsightsInTimeline","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableInsightsInTimeline, enableInsightsInTimeline__is_set);
}
/**
* element : enableInsightsInTimelineEacStd of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableInsightsInTimelineEacStd__is_set = false;
private boolean enableInsightsInTimelineEacStd;
public boolean getEnableInsightsInTimelineEacStd() {
return enableInsightsInTimelineEacStd;
}
public boolean isEnableInsightsInTimelineEacStd() {
return enableInsightsInTimelineEacStd;
}
public void setEnableInsightsInTimelineEacStd(boolean enableInsightsInTimelineEacStd) {
this.enableInsightsInTimelineEacStd = enableInsightsInTimelineEacStd;
enableInsightsInTimelineEacStd__is_set = true;
}
protected void setEnableInsightsInTimelineEacStd(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableInsightsInTimelineEacStd", "http://soap.sforce.com/2006/04/metadata","enableInsightsInTimelineEacStd","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableInsightsInTimelineEacStd(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableInsightsInTimelineEacStd", "http://soap.sforce.com/2006/04/metadata","enableInsightsInTimelineEacStd","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableInsightsInTimelineEacStd(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableInsightsInTimelineEacStd", "http://soap.sforce.com/2006/04/metadata","enableInsightsInTimelineEacStd","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableInsightsInTimelineEacStd, enableInsightsInTimelineEacStd__is_set);
}
/**
* element : enableUnifiedActivitiesPref of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableUnifiedActivitiesPref__is_set = false;
private boolean enableUnifiedActivitiesPref;
public boolean getEnableUnifiedActivitiesPref() {
return enableUnifiedActivitiesPref;
}
public boolean isEnableUnifiedActivitiesPref() {
return enableUnifiedActivitiesPref;
}
public void setEnableUnifiedActivitiesPref(boolean enableUnifiedActivitiesPref) {
this.enableUnifiedActivitiesPref = enableUnifiedActivitiesPref;
enableUnifiedActivitiesPref__is_set = true;
}
protected void setEnableUnifiedActivitiesPref(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableUnifiedActivitiesPref", "http://soap.sforce.com/2006/04/metadata","enableUnifiedActivitiesPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableUnifiedActivitiesPref(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableUnifiedActivitiesPref", "http://soap.sforce.com/2006/04/metadata","enableUnifiedActivitiesPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableUnifiedActivitiesPref(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableUnifiedActivitiesPref", "http://soap.sforce.com/2006/04/metadata","enableUnifiedActivitiesPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableUnifiedActivitiesPref, enableUnifiedActivitiesPref__is_set);
}
/**
* element : provisionProductivityFeatures of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean provisionProductivityFeatures__is_set = false;
private boolean provisionProductivityFeatures;
public boolean getProvisionProductivityFeatures() {
return provisionProductivityFeatures;
}
public boolean isProvisionProductivityFeatures() {
return provisionProductivityFeatures;
}
public void setProvisionProductivityFeatures(boolean provisionProductivityFeatures) {
this.provisionProductivityFeatures = provisionProductivityFeatures;
provisionProductivityFeatures__is_set = true;
}
protected void setProvisionProductivityFeatures(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("provisionProductivityFeatures", "http://soap.sforce.com/2006/04/metadata","provisionProductivityFeatures","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setProvisionProductivityFeatures(__typeMapper.readBoolean(__in, _lookupTypeInfo("provisionProductivityFeatures", "http://soap.sforce.com/2006/04/metadata","provisionProductivityFeatures","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldProvisionProductivityFeatures(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("provisionProductivityFeatures", "http://soap.sforce.com/2006/04/metadata","provisionProductivityFeatures","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), provisionProductivityFeatures, provisionProductivityFeatures__is_set);
}
/**
* element : relationshipGraphPref of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean relationshipGraphPref__is_set = false;
private boolean relationshipGraphPref;
public boolean getRelationshipGraphPref() {
return relationshipGraphPref;
}
public boolean isRelationshipGraphPref() {
return relationshipGraphPref;
}
public void setRelationshipGraphPref(boolean relationshipGraphPref) {
this.relationshipGraphPref = relationshipGraphPref;
relationshipGraphPref__is_set = true;
}
protected void setRelationshipGraphPref(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("relationshipGraphPref", "http://soap.sforce.com/2006/04/metadata","relationshipGraphPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setRelationshipGraphPref(__typeMapper.readBoolean(__in, _lookupTypeInfo("relationshipGraphPref", "http://soap.sforce.com/2006/04/metadata","relationshipGraphPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldRelationshipGraphPref(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("relationshipGraphPref", "http://soap.sforce.com/2006/04/metadata","relationshipGraphPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), relationshipGraphPref, relationshipGraphPref__is_set);
}
/**
* element : s2XSvcAccEmail of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean s2XSvcAccEmail__is_set = false;
private boolean s2XSvcAccEmail;
public boolean getS2XSvcAccEmail() {
return s2XSvcAccEmail;
}
public boolean isS2XSvcAccEmail() {
return s2XSvcAccEmail;
}
public void setS2XSvcAccEmail(boolean s2XSvcAccEmail) {
this.s2XSvcAccEmail = s2XSvcAccEmail;
s2XSvcAccEmail__is_set = true;
}
protected void setS2XSvcAccEmail(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("s2XSvcAccEmail", "http://soap.sforce.com/2006/04/metadata","s2XSvcAccEmail","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setS2XSvcAccEmail(__typeMapper.readBoolean(__in, _lookupTypeInfo("s2XSvcAccEmail", "http://soap.sforce.com/2006/04/metadata","s2XSvcAccEmail","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldS2XSvcAccEmail(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("s2XSvcAccEmail", "http://soap.sforce.com/2006/04/metadata","s2XSvcAccEmail","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), s2XSvcAccEmail, s2XSvcAccEmail__is_set);
}
/**
* element : salesforceEventsOnlyPref of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean salesforceEventsOnlyPref__is_set = false;
private boolean salesforceEventsOnlyPref;
public boolean getSalesforceEventsOnlyPref() {
return salesforceEventsOnlyPref;
}
public boolean isSalesforceEventsOnlyPref() {
return salesforceEventsOnlyPref;
}
public void setSalesforceEventsOnlyPref(boolean salesforceEventsOnlyPref) {
this.salesforceEventsOnlyPref = salesforceEventsOnlyPref;
salesforceEventsOnlyPref__is_set = true;
}
protected void setSalesforceEventsOnlyPref(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("salesforceEventsOnlyPref", "http://soap.sforce.com/2006/04/metadata","salesforceEventsOnlyPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setSalesforceEventsOnlyPref(__typeMapper.readBoolean(__in, _lookupTypeInfo("salesforceEventsOnlyPref", "http://soap.sforce.com/2006/04/metadata","salesforceEventsOnlyPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldSalesforceEventsOnlyPref(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("salesforceEventsOnlyPref", "http://soap.sforce.com/2006/04/metadata","salesforceEventsOnlyPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), salesforceEventsOnlyPref, salesforceEventsOnlyPref__is_set);
}
/**
* element : sensitiveEmailFilter of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean sensitiveEmailFilter__is_set = false;
private boolean sensitiveEmailFilter;
public boolean getSensitiveEmailFilter() {
return sensitiveEmailFilter;
}
public boolean isSensitiveEmailFilter() {
return sensitiveEmailFilter;
}
public void setSensitiveEmailFilter(boolean sensitiveEmailFilter) {
this.sensitiveEmailFilter = sensitiveEmailFilter;
sensitiveEmailFilter__is_set = true;
}
protected void setSensitiveEmailFilter(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("sensitiveEmailFilter", "http://soap.sforce.com/2006/04/metadata","sensitiveEmailFilter","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setSensitiveEmailFilter(__typeMapper.readBoolean(__in, _lookupTypeInfo("sensitiveEmailFilter", "http://soap.sforce.com/2006/04/metadata","sensitiveEmailFilter","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldSensitiveEmailFilter(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("sensitiveEmailFilter", "http://soap.sforce.com/2006/04/metadata","sensitiveEmailFilter","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), sensitiveEmailFilter, sensitiveEmailFilter__is_set);
}
/**
* element : showEACCalendarPref of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean showEACCalendarPref__is_set = false;
private boolean showEACCalendarPref;
public boolean getShowEACCalendarPref() {
return showEACCalendarPref;
}
public boolean isShowEACCalendarPref() {
return showEACCalendarPref;
}
public void setShowEACCalendarPref(boolean showEACCalendarPref) {
this.showEACCalendarPref = showEACCalendarPref;
showEACCalendarPref__is_set = true;
}
protected void setShowEACCalendarPref(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("showEACCalendarPref", "http://soap.sforce.com/2006/04/metadata","showEACCalendarPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setShowEACCalendarPref(__typeMapper.readBoolean(__in, _lookupTypeInfo("showEACCalendarPref", "http://soap.sforce.com/2006/04/metadata","showEACCalendarPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldShowEACCalendarPref(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("showEACCalendarPref", "http://soap.sforce.com/2006/04/metadata","showEACCalendarPref","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), showEACCalendarPref, showEACCalendarPref__is_set);
}
/**
* element : syncEmailToCoreActivity of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean syncEmailToCoreActivity__is_set = false;
private boolean syncEmailToCoreActivity;
public boolean getSyncEmailToCoreActivity() {
return syncEmailToCoreActivity;
}
public boolean isSyncEmailToCoreActivity() {
return syncEmailToCoreActivity;
}
public void setSyncEmailToCoreActivity(boolean syncEmailToCoreActivity) {
this.syncEmailToCoreActivity = syncEmailToCoreActivity;
syncEmailToCoreActivity__is_set = true;
}
protected void setSyncEmailToCoreActivity(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("syncEmailToCoreActivity", "http://soap.sforce.com/2006/04/metadata","syncEmailToCoreActivity","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setSyncEmailToCoreActivity(__typeMapper.readBoolean(__in, _lookupTypeInfo("syncEmailToCoreActivity", "http://soap.sforce.com/2006/04/metadata","syncEmailToCoreActivity","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldSyncEmailToCoreActivity(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("syncEmailToCoreActivity", "http://soap.sforce.com/2006/04/metadata","syncEmailToCoreActivity","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), syncEmailToCoreActivity, syncEmailToCoreActivity__is_set);
}
/**
* element : syncInternalEvents of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean syncInternalEvents__is_set = false;
private boolean syncInternalEvents;
public boolean getSyncInternalEvents() {
return syncInternalEvents;
}
public boolean isSyncInternalEvents() {
return syncInternalEvents;
}
public void setSyncInternalEvents(boolean syncInternalEvents) {
this.syncInternalEvents = syncInternalEvents;
syncInternalEvents__is_set = true;
}
protected void setSyncInternalEvents(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("syncInternalEvents", "http://soap.sforce.com/2006/04/metadata","syncInternalEvents","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setSyncInternalEvents(__typeMapper.readBoolean(__in, _lookupTypeInfo("syncInternalEvents", "http://soap.sforce.com/2006/04/metadata","syncInternalEvents","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldSyncInternalEvents(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("syncInternalEvents", "http://soap.sforce.com/2006/04/metadata","syncInternalEvents","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), syncInternalEvents, syncInternalEvents__is_set);
}
/**
*/
@Override
public void write(javax.xml.namespace.QName __element,
com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
__out.writeStartTag(__element.getNamespaceURI(), __element.getLocalPart());
__typeMapper.writeXsiType(__out, "http://soap.sforce.com/2006/04/metadata", "EACSettings");
writeFields(__out, __typeMapper);
__out.writeEndTag(__element.getNamespaceURI(), __element.getLocalPart());
}
protected void writeFields(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
super.writeFields(__out, __typeMapper);
writeFields1(__out, __typeMapper);
}
@Override
public void load(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__typeMapper.consumeStartTag(__in);
loadFields(__in, __typeMapper);
__typeMapper.consumeEndTag(__in);
}
protected void loadFields(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
super.loadFields(__in, __typeMapper);
loadFields1(__in, __typeMapper);
}
@Override
public String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("[EACSettings ");
sb.append(super.toString());
toString1(sb);
sb.append("]\n");
return sb.toString();
}
private void toStringHelper(StringBuilder sb, String name, Object value) {
sb.append(' ').append(name).append("='").append(com.sforce.ws.util.Verbose.toString(value)).append("'\n");
}
private void writeFields1(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
writeFieldAddRcCompToFlexiPages(__out, __typeMapper);
writeFieldAutoContactCreationPref(__out, __typeMapper);
writeFieldAutoContactEnrichmentPref(__out, __typeMapper);
writeFieldAutoPopulateGoogleMeetLinks(__out, __typeMapper);
writeFieldAutomatedEmailFilter(__out, __typeMapper);
writeFieldDSThresholdNotification(__out, __typeMapper);
writeFieldEnableActivityAnalyticsPref(__out, __typeMapper);
writeFieldEnableActivityCapture(__out, __typeMapper);
writeFieldEnableActivityMetrics(__out, __typeMapper);
writeFieldEnableActivitySyncEngine(__out, __typeMapper);
writeFieldEnableEACForEveryonePref(__out, __typeMapper);
writeFieldEnableEnforceEacSharingPref(__out, __typeMapper);
writeFieldEnableInboxActivitySharing(__out, __typeMapper);
writeFieldEnableInsightsInTimeline(__out, __typeMapper);
writeFieldEnableInsightsInTimelineEacStd(__out, __typeMapper);
writeFieldEnableUnifiedActivitiesPref(__out, __typeMapper);
writeFieldProvisionProductivityFeatures(__out, __typeMapper);
writeFieldRelationshipGraphPref(__out, __typeMapper);
writeFieldS2XSvcAccEmail(__out, __typeMapper);
writeFieldSalesforceEventsOnlyPref(__out, __typeMapper);
writeFieldSensitiveEmailFilter(__out, __typeMapper);
writeFieldShowEACCalendarPref(__out, __typeMapper);
writeFieldSyncEmailToCoreActivity(__out, __typeMapper);
writeFieldSyncInternalEvents(__out, __typeMapper);
}
private void loadFields1(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
setAddRcCompToFlexiPages(__in, __typeMapper);
setAutoContactCreationPref(__in, __typeMapper);
setAutoContactEnrichmentPref(__in, __typeMapper);
setAutoPopulateGoogleMeetLinks(__in, __typeMapper);
setAutomatedEmailFilter(__in, __typeMapper);
setDSThresholdNotification(__in, __typeMapper);
setEnableActivityAnalyticsPref(__in, __typeMapper);
setEnableActivityCapture(__in, __typeMapper);
setEnableActivityMetrics(__in, __typeMapper);
setEnableActivitySyncEngine(__in, __typeMapper);
setEnableEACForEveryonePref(__in, __typeMapper);
setEnableEnforceEacSharingPref(__in, __typeMapper);
setEnableInboxActivitySharing(__in, __typeMapper);
setEnableInsightsInTimeline(__in, __typeMapper);
setEnableInsightsInTimelineEacStd(__in, __typeMapper);
setEnableUnifiedActivitiesPref(__in, __typeMapper);
setProvisionProductivityFeatures(__in, __typeMapper);
setRelationshipGraphPref(__in, __typeMapper);
setS2XSvcAccEmail(__in, __typeMapper);
setSalesforceEventsOnlyPref(__in, __typeMapper);
setSensitiveEmailFilter(__in, __typeMapper);
setShowEACCalendarPref(__in, __typeMapper);
setSyncEmailToCoreActivity(__in, __typeMapper);
setSyncInternalEvents(__in, __typeMapper);
}
private void toString1(StringBuilder sb) {
toStringHelper(sb, "addRcCompToFlexiPages", addRcCompToFlexiPages);
toStringHelper(sb, "autoContactCreationPref", autoContactCreationPref);
toStringHelper(sb, "autoContactEnrichmentPref", autoContactEnrichmentPref);
toStringHelper(sb, "autoPopulateGoogleMeetLinks", autoPopulateGoogleMeetLinks);
toStringHelper(sb, "automatedEmailFilter", automatedEmailFilter);
toStringHelper(sb, "dSThresholdNotification", dSThresholdNotification);
toStringHelper(sb, "enableActivityAnalyticsPref", enableActivityAnalyticsPref);
toStringHelper(sb, "enableActivityCapture", enableActivityCapture);
toStringHelper(sb, "enableActivityMetrics", enableActivityMetrics);
toStringHelper(sb, "enableActivitySyncEngine", enableActivitySyncEngine);
toStringHelper(sb, "enableEACForEveryonePref", enableEACForEveryonePref);
toStringHelper(sb, "enableEnforceEacSharingPref", enableEnforceEacSharingPref);
toStringHelper(sb, "enableInboxActivitySharing", enableInboxActivitySharing);
toStringHelper(sb, "enableInsightsInTimeline", enableInsightsInTimeline);
toStringHelper(sb, "enableInsightsInTimelineEacStd", enableInsightsInTimelineEacStd);
toStringHelper(sb, "enableUnifiedActivitiesPref", enableUnifiedActivitiesPref);
toStringHelper(sb, "provisionProductivityFeatures", provisionProductivityFeatures);
toStringHelper(sb, "relationshipGraphPref", relationshipGraphPref);
toStringHelper(sb, "s2XSvcAccEmail", s2XSvcAccEmail);
toStringHelper(sb, "salesforceEventsOnlyPref", salesforceEventsOnlyPref);
toStringHelper(sb, "sensitiveEmailFilter", sensitiveEmailFilter);
toStringHelper(sb, "showEACCalendarPref", showEACCalendarPref);
toStringHelper(sb, "syncEmailToCoreActivity", syncEmailToCoreActivity);
toStringHelper(sb, "syncInternalEvents", syncInternalEvents);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy