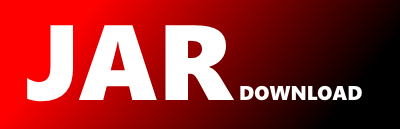
com.sforce.soap.metadata.FlowTranslation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of force-metadata-api Show documentation
Show all versions of force-metadata-api Show documentation
Force.com Web Service Connector
The newest version!
package com.sforce.soap.metadata;
/**
* This is a generated class for the SObject Enterprise API.
* Do not edit this file, as your changes will be lost.
*/
public class FlowTranslation implements com.sforce.ws.bind.XMLizable {
/**
* Constructor
*/
public FlowTranslation() {}
/* Cache the typeInfo instead of declaring static fields throughout*/
private transient java.util.Map typeInfoCache = new java.util.HashMap();
private com.sforce.ws.bind.TypeInfo _lookupTypeInfo(String fieldName, String namespace, String name, String typeNS, String type, int minOcc, int maxOcc, boolean elementForm) {
com.sforce.ws.bind.TypeInfo typeInfo = typeInfoCache.get(fieldName);
if (typeInfo == null) {
typeInfo = new com.sforce.ws.bind.TypeInfo(namespace, name, typeNS, type, minOcc, maxOcc, elementForm);
typeInfoCache.put(fieldName, typeInfo);
}
return typeInfo;
}
/**
* element : choices of type {http://soap.sforce.com/2006/04/metadata}FlowChoiceTranslation
* java type: com.sforce.soap.metadata.FlowChoiceTranslation[]
*/
private boolean choices__is_set = false;
private com.sforce.soap.metadata.FlowChoiceTranslation[] choices = new com.sforce.soap.metadata.FlowChoiceTranslation[0];
public com.sforce.soap.metadata.FlowChoiceTranslation[] getChoices() {
return choices;
}
public void setChoices(com.sforce.soap.metadata.FlowChoiceTranslation[] choices) {
this.choices = choices;
choices__is_set = true;
}
protected void setChoices(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("choices", "http://soap.sforce.com/2006/04/metadata","choices","http://soap.sforce.com/2006/04/metadata","FlowChoiceTranslation",0,-1,true))) {
setChoices((com.sforce.soap.metadata.FlowChoiceTranslation[])__typeMapper.readObject(__in, _lookupTypeInfo("choices", "http://soap.sforce.com/2006/04/metadata","choices","http://soap.sforce.com/2006/04/metadata","FlowChoiceTranslation",0,-1,true), com.sforce.soap.metadata.FlowChoiceTranslation[].class));
}
}
private void writeFieldChoices(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("choices", "http://soap.sforce.com/2006/04/metadata","choices","http://soap.sforce.com/2006/04/metadata","FlowChoiceTranslation",0,-1,true), choices, choices__is_set);
}
/**
* element : customErrorMessages of type {http://soap.sforce.com/2006/04/metadata}FlowCustomErrorMessageTranslation
* java type: com.sforce.soap.metadata.FlowCustomErrorMessageTranslation[]
*/
private boolean customErrorMessages__is_set = false;
private com.sforce.soap.metadata.FlowCustomErrorMessageTranslation[] customErrorMessages = new com.sforce.soap.metadata.FlowCustomErrorMessageTranslation[0];
public com.sforce.soap.metadata.FlowCustomErrorMessageTranslation[] getCustomErrorMessages() {
return customErrorMessages;
}
public void setCustomErrorMessages(com.sforce.soap.metadata.FlowCustomErrorMessageTranslation[] customErrorMessages) {
this.customErrorMessages = customErrorMessages;
customErrorMessages__is_set = true;
}
protected void setCustomErrorMessages(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("customErrorMessages", "http://soap.sforce.com/2006/04/metadata","customErrorMessages","http://soap.sforce.com/2006/04/metadata","FlowCustomErrorMessageTranslation",0,-1,true))) {
setCustomErrorMessages((com.sforce.soap.metadata.FlowCustomErrorMessageTranslation[])__typeMapper.readObject(__in, _lookupTypeInfo("customErrorMessages", "http://soap.sforce.com/2006/04/metadata","customErrorMessages","http://soap.sforce.com/2006/04/metadata","FlowCustomErrorMessageTranslation",0,-1,true), com.sforce.soap.metadata.FlowCustomErrorMessageTranslation[].class));
}
}
private void writeFieldCustomErrorMessages(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("customErrorMessages", "http://soap.sforce.com/2006/04/metadata","customErrorMessages","http://soap.sforce.com/2006/04/metadata","FlowCustomErrorMessageTranslation",0,-1,true), customErrorMessages, customErrorMessages__is_set);
}
/**
* element : fullName of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean fullName__is_set = false;
private java.lang.String fullName;
public java.lang.String getFullName() {
return fullName;
}
public void setFullName(java.lang.String fullName) {
this.fullName = fullName;
fullName__is_set = true;
}
protected void setFullName(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("fullName", "http://soap.sforce.com/2006/04/metadata","fullName","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setFullName(__typeMapper.readString(__in, _lookupTypeInfo("fullName", "http://soap.sforce.com/2006/04/metadata","fullName","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldFullName(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("fullName", "http://soap.sforce.com/2006/04/metadata","fullName","http://www.w3.org/2001/XMLSchema","string",0,1,true), fullName, fullName__is_set);
}
/**
* element : label of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean label__is_set = false;
private java.lang.String label;
public java.lang.String getLabel() {
return label;
}
public void setLabel(java.lang.String label) {
this.label = label;
label__is_set = true;
}
protected void setLabel(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("label", "http://soap.sforce.com/2006/04/metadata","label","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setLabel(__typeMapper.readString(__in, _lookupTypeInfo("label", "http://soap.sforce.com/2006/04/metadata","label","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldLabel(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("label", "http://soap.sforce.com/2006/04/metadata","label","http://www.w3.org/2001/XMLSchema","string",0,1,true), label, label__is_set);
}
/**
* element : orchestrationSteps of type {http://soap.sforce.com/2006/04/metadata}FlowOrchestrationStepTranslation
* java type: com.sforce.soap.metadata.FlowOrchestrationStepTranslation[]
*/
private boolean orchestrationSteps__is_set = false;
private com.sforce.soap.metadata.FlowOrchestrationStepTranslation[] orchestrationSteps = new com.sforce.soap.metadata.FlowOrchestrationStepTranslation[0];
public com.sforce.soap.metadata.FlowOrchestrationStepTranslation[] getOrchestrationSteps() {
return orchestrationSteps;
}
public void setOrchestrationSteps(com.sforce.soap.metadata.FlowOrchestrationStepTranslation[] orchestrationSteps) {
this.orchestrationSteps = orchestrationSteps;
orchestrationSteps__is_set = true;
}
protected void setOrchestrationSteps(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("orchestrationSteps", "http://soap.sforce.com/2006/04/metadata","orchestrationSteps","http://soap.sforce.com/2006/04/metadata","FlowOrchestrationStepTranslation",0,-1,true))) {
setOrchestrationSteps((com.sforce.soap.metadata.FlowOrchestrationStepTranslation[])__typeMapper.readObject(__in, _lookupTypeInfo("orchestrationSteps", "http://soap.sforce.com/2006/04/metadata","orchestrationSteps","http://soap.sforce.com/2006/04/metadata","FlowOrchestrationStepTranslation",0,-1,true), com.sforce.soap.metadata.FlowOrchestrationStepTranslation[].class));
}
}
private void writeFieldOrchestrationSteps(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("orchestrationSteps", "http://soap.sforce.com/2006/04/metadata","orchestrationSteps","http://soap.sforce.com/2006/04/metadata","FlowOrchestrationStepTranslation",0,-1,true), orchestrationSteps, orchestrationSteps__is_set);
}
/**
* element : screens of type {http://soap.sforce.com/2006/04/metadata}FlowScreenTranslation
* java type: com.sforce.soap.metadata.FlowScreenTranslation[]
*/
private boolean screens__is_set = false;
private com.sforce.soap.metadata.FlowScreenTranslation[] screens = new com.sforce.soap.metadata.FlowScreenTranslation[0];
public com.sforce.soap.metadata.FlowScreenTranslation[] getScreens() {
return screens;
}
public void setScreens(com.sforce.soap.metadata.FlowScreenTranslation[] screens) {
this.screens = screens;
screens__is_set = true;
}
protected void setScreens(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("screens", "http://soap.sforce.com/2006/04/metadata","screens","http://soap.sforce.com/2006/04/metadata","FlowScreenTranslation",0,-1,true))) {
setScreens((com.sforce.soap.metadata.FlowScreenTranslation[])__typeMapper.readObject(__in, _lookupTypeInfo("screens", "http://soap.sforce.com/2006/04/metadata","screens","http://soap.sforce.com/2006/04/metadata","FlowScreenTranslation",0,-1,true), com.sforce.soap.metadata.FlowScreenTranslation[].class));
}
}
private void writeFieldScreens(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("screens", "http://soap.sforce.com/2006/04/metadata","screens","http://soap.sforce.com/2006/04/metadata","FlowScreenTranslation",0,-1,true), screens, screens__is_set);
}
/**
* element : stages of type {http://soap.sforce.com/2006/04/metadata}FlowStageTranslation
* java type: com.sforce.soap.metadata.FlowStageTranslation[]
*/
private boolean stages__is_set = false;
private com.sforce.soap.metadata.FlowStageTranslation[] stages = new com.sforce.soap.metadata.FlowStageTranslation[0];
public com.sforce.soap.metadata.FlowStageTranslation[] getStages() {
return stages;
}
public void setStages(com.sforce.soap.metadata.FlowStageTranslation[] stages) {
this.stages = stages;
stages__is_set = true;
}
protected void setStages(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("stages", "http://soap.sforce.com/2006/04/metadata","stages","http://soap.sforce.com/2006/04/metadata","FlowStageTranslation",0,-1,true))) {
setStages((com.sforce.soap.metadata.FlowStageTranslation[])__typeMapper.readObject(__in, _lookupTypeInfo("stages", "http://soap.sforce.com/2006/04/metadata","stages","http://soap.sforce.com/2006/04/metadata","FlowStageTranslation",0,-1,true), com.sforce.soap.metadata.FlowStageTranslation[].class));
}
}
private void writeFieldStages(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("stages", "http://soap.sforce.com/2006/04/metadata","stages","http://soap.sforce.com/2006/04/metadata","FlowStageTranslation",0,-1,true), stages, stages__is_set);
}
/**
* element : textTemplates of type {http://soap.sforce.com/2006/04/metadata}FlowTextTemplateTranslation
* java type: com.sforce.soap.metadata.FlowTextTemplateTranslation[]
*/
private boolean textTemplates__is_set = false;
private com.sforce.soap.metadata.FlowTextTemplateTranslation[] textTemplates = new com.sforce.soap.metadata.FlowTextTemplateTranslation[0];
public com.sforce.soap.metadata.FlowTextTemplateTranslation[] getTextTemplates() {
return textTemplates;
}
public void setTextTemplates(com.sforce.soap.metadata.FlowTextTemplateTranslation[] textTemplates) {
this.textTemplates = textTemplates;
textTemplates__is_set = true;
}
protected void setTextTemplates(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("textTemplates", "http://soap.sforce.com/2006/04/metadata","textTemplates","http://soap.sforce.com/2006/04/metadata","FlowTextTemplateTranslation",0,-1,true))) {
setTextTemplates((com.sforce.soap.metadata.FlowTextTemplateTranslation[])__typeMapper.readObject(__in, _lookupTypeInfo("textTemplates", "http://soap.sforce.com/2006/04/metadata","textTemplates","http://soap.sforce.com/2006/04/metadata","FlowTextTemplateTranslation",0,-1,true), com.sforce.soap.metadata.FlowTextTemplateTranslation[].class));
}
}
private void writeFieldTextTemplates(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("textTemplates", "http://soap.sforce.com/2006/04/metadata","textTemplates","http://soap.sforce.com/2006/04/metadata","FlowTextTemplateTranslation",0,-1,true), textTemplates, textTemplates__is_set);
}
/**
*/
@Override
public void write(javax.xml.namespace.QName __element,
com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
__out.writeStartTag(__element.getNamespaceURI(), __element.getLocalPart());
writeFields(__out, __typeMapper);
__out.writeEndTag(__element.getNamespaceURI(), __element.getLocalPart());
}
protected void writeFields(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
writeFields1(__out, __typeMapper);
}
@Override
public void load(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__typeMapper.consumeStartTag(__in);
loadFields(__in, __typeMapper);
__typeMapper.consumeEndTag(__in);
}
protected void loadFields(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
loadFields1(__in, __typeMapper);
}
@Override
public String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("[FlowTranslation ");
toString1(sb);
sb.append("]\n");
return sb.toString();
}
private void toStringHelper(StringBuilder sb, String name, Object value) {
sb.append(' ').append(name).append("='").append(com.sforce.ws.util.Verbose.toString(value)).append("'\n");
}
private void writeFields1(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
writeFieldChoices(__out, __typeMapper);
writeFieldCustomErrorMessages(__out, __typeMapper);
writeFieldFullName(__out, __typeMapper);
writeFieldLabel(__out, __typeMapper);
writeFieldOrchestrationSteps(__out, __typeMapper);
writeFieldScreens(__out, __typeMapper);
writeFieldStages(__out, __typeMapper);
writeFieldTextTemplates(__out, __typeMapper);
}
private void loadFields1(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
setChoices(__in, __typeMapper);
setCustomErrorMessages(__in, __typeMapper);
setFullName(__in, __typeMapper);
setLabel(__in, __typeMapper);
setOrchestrationSteps(__in, __typeMapper);
setScreens(__in, __typeMapper);
setStages(__in, __typeMapper);
setTextTemplates(__in, __typeMapper);
}
private void toString1(StringBuilder sb) {
toStringHelper(sb, "choices", choices);
toStringHelper(sb, "customErrorMessages", customErrorMessages);
toStringHelper(sb, "fullName", fullName);
toStringHelper(sb, "label", label);
toStringHelper(sb, "orchestrationSteps", orchestrationSteps);
toStringHelper(sb, "screens", screens);
toStringHelper(sb, "stages", stages);
toStringHelper(sb, "textTemplates", textTemplates);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy