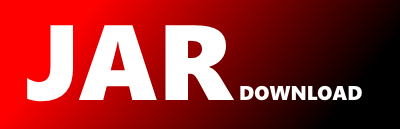
com.sforce.soap.metadata.LiveChatButton Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of force-metadata-api Show documentation
Show all versions of force-metadata-api Show documentation
Force.com Web Service Connector
The newest version!
package com.sforce.soap.metadata;
/**
* This is a generated class for the SObject Enterprise API.
* Do not edit this file, as your changes will be lost.
*/
public class LiveChatButton extends com.sforce.soap.metadata.Metadata {
/**
* Constructor
*/
public LiveChatButton() {}
/* Cache the typeInfo instead of declaring static fields throughout*/
private transient java.util.Map typeInfoCache = new java.util.HashMap();
private com.sforce.ws.bind.TypeInfo _lookupTypeInfo(String fieldName, String namespace, String name, String typeNS, String type, int minOcc, int maxOcc, boolean elementForm) {
com.sforce.ws.bind.TypeInfo typeInfo = typeInfoCache.get(fieldName);
if (typeInfo == null) {
typeInfo = new com.sforce.ws.bind.TypeInfo(namespace, name, typeNS, type, minOcc, maxOcc, elementForm);
typeInfoCache.put(fieldName, typeInfo);
}
return typeInfo;
}
/**
* element : animation of type {http://soap.sforce.com/2006/04/metadata}LiveChatButtonPresentation
* java type: com.sforce.soap.metadata.LiveChatButtonPresentation
*/
private boolean animation__is_set = false;
private com.sforce.soap.metadata.LiveChatButtonPresentation animation;
public com.sforce.soap.metadata.LiveChatButtonPresentation getAnimation() {
return animation;
}
public void setAnimation(com.sforce.soap.metadata.LiveChatButtonPresentation animation) {
this.animation = animation;
animation__is_set = true;
}
protected void setAnimation(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("animation", "http://soap.sforce.com/2006/04/metadata","animation","http://soap.sforce.com/2006/04/metadata","LiveChatButtonPresentation",0,1,true))) {
setAnimation((com.sforce.soap.metadata.LiveChatButtonPresentation)__typeMapper.readObject(__in, _lookupTypeInfo("animation", "http://soap.sforce.com/2006/04/metadata","animation","http://soap.sforce.com/2006/04/metadata","LiveChatButtonPresentation",0,1,true), com.sforce.soap.metadata.LiveChatButtonPresentation.class));
}
}
private void writeFieldAnimation(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("animation", "http://soap.sforce.com/2006/04/metadata","animation","http://soap.sforce.com/2006/04/metadata","LiveChatButtonPresentation",0,1,true), animation, animation__is_set);
}
/**
* element : autoGreeting of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean autoGreeting__is_set = false;
private java.lang.String autoGreeting;
public java.lang.String getAutoGreeting() {
return autoGreeting;
}
public void setAutoGreeting(java.lang.String autoGreeting) {
this.autoGreeting = autoGreeting;
autoGreeting__is_set = true;
}
protected void setAutoGreeting(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("autoGreeting", "http://soap.sforce.com/2006/04/metadata","autoGreeting","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setAutoGreeting(__typeMapper.readString(__in, _lookupTypeInfo("autoGreeting", "http://soap.sforce.com/2006/04/metadata","autoGreeting","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldAutoGreeting(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("autoGreeting", "http://soap.sforce.com/2006/04/metadata","autoGreeting","http://www.w3.org/2001/XMLSchema","string",0,1,true), autoGreeting, autoGreeting__is_set);
}
/**
* element : chasitorIdleTimeout of type {http://www.w3.org/2001/XMLSchema}int
* java type: int
*/
private boolean chasitorIdleTimeout__is_set = false;
private int chasitorIdleTimeout;
public int getChasitorIdleTimeout() {
return chasitorIdleTimeout;
}
public void setChasitorIdleTimeout(int chasitorIdleTimeout) {
this.chasitorIdleTimeout = chasitorIdleTimeout;
chasitorIdleTimeout__is_set = true;
}
protected void setChasitorIdleTimeout(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("chasitorIdleTimeout", "http://soap.sforce.com/2006/04/metadata","chasitorIdleTimeout","http://www.w3.org/2001/XMLSchema","int",0,1,true))) {
setChasitorIdleTimeout((int)__typeMapper.readInt(__in, _lookupTypeInfo("chasitorIdleTimeout", "http://soap.sforce.com/2006/04/metadata","chasitorIdleTimeout","http://www.w3.org/2001/XMLSchema","int",0,1,true), int.class));
}
}
private void writeFieldChasitorIdleTimeout(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("chasitorIdleTimeout", "http://soap.sforce.com/2006/04/metadata","chasitorIdleTimeout","http://www.w3.org/2001/XMLSchema","int",0,1,true), chasitorIdleTimeout, chasitorIdleTimeout__is_set);
}
/**
* element : chasitorIdleTimeoutWarning of type {http://www.w3.org/2001/XMLSchema}int
* java type: int
*/
private boolean chasitorIdleTimeoutWarning__is_set = false;
private int chasitorIdleTimeoutWarning;
public int getChasitorIdleTimeoutWarning() {
return chasitorIdleTimeoutWarning;
}
public void setChasitorIdleTimeoutWarning(int chasitorIdleTimeoutWarning) {
this.chasitorIdleTimeoutWarning = chasitorIdleTimeoutWarning;
chasitorIdleTimeoutWarning__is_set = true;
}
protected void setChasitorIdleTimeoutWarning(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("chasitorIdleTimeoutWarning", "http://soap.sforce.com/2006/04/metadata","chasitorIdleTimeoutWarning","http://www.w3.org/2001/XMLSchema","int",0,1,true))) {
setChasitorIdleTimeoutWarning((int)__typeMapper.readInt(__in, _lookupTypeInfo("chasitorIdleTimeoutWarning", "http://soap.sforce.com/2006/04/metadata","chasitorIdleTimeoutWarning","http://www.w3.org/2001/XMLSchema","int",0,1,true), int.class));
}
}
private void writeFieldChasitorIdleTimeoutWarning(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("chasitorIdleTimeoutWarning", "http://soap.sforce.com/2006/04/metadata","chasitorIdleTimeoutWarning","http://www.w3.org/2001/XMLSchema","int",0,1,true), chasitorIdleTimeoutWarning, chasitorIdleTimeoutWarning__is_set);
}
/**
* element : chatPage of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean chatPage__is_set = false;
private java.lang.String chatPage;
public java.lang.String getChatPage() {
return chatPage;
}
public void setChatPage(java.lang.String chatPage) {
this.chatPage = chatPage;
chatPage__is_set = true;
}
protected void setChatPage(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("chatPage", "http://soap.sforce.com/2006/04/metadata","chatPage","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setChatPage(__typeMapper.readString(__in, _lookupTypeInfo("chatPage", "http://soap.sforce.com/2006/04/metadata","chatPage","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldChatPage(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("chatPage", "http://soap.sforce.com/2006/04/metadata","chatPage","http://www.w3.org/2001/XMLSchema","string",0,1,true), chatPage, chatPage__is_set);
}
/**
* element : customAgentName of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean customAgentName__is_set = false;
private java.lang.String customAgentName;
public java.lang.String getCustomAgentName() {
return customAgentName;
}
public void setCustomAgentName(java.lang.String customAgentName) {
this.customAgentName = customAgentName;
customAgentName__is_set = true;
}
protected void setCustomAgentName(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("customAgentName", "http://soap.sforce.com/2006/04/metadata","customAgentName","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setCustomAgentName(__typeMapper.readString(__in, _lookupTypeInfo("customAgentName", "http://soap.sforce.com/2006/04/metadata","customAgentName","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldCustomAgentName(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("customAgentName", "http://soap.sforce.com/2006/04/metadata","customAgentName","http://www.w3.org/2001/XMLSchema","string",0,1,true), customAgentName, customAgentName__is_set);
}
/**
* element : deployments of type {http://soap.sforce.com/2006/04/metadata}LiveChatButtonDeployments
* java type: com.sforce.soap.metadata.LiveChatButtonDeployments
*/
private boolean deployments__is_set = false;
private com.sforce.soap.metadata.LiveChatButtonDeployments deployments;
public com.sforce.soap.metadata.LiveChatButtonDeployments getDeployments() {
return deployments;
}
public void setDeployments(com.sforce.soap.metadata.LiveChatButtonDeployments deployments) {
this.deployments = deployments;
deployments__is_set = true;
}
protected void setDeployments(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("deployments", "http://soap.sforce.com/2006/04/metadata","deployments","http://soap.sforce.com/2006/04/metadata","LiveChatButtonDeployments",0,1,true))) {
setDeployments((com.sforce.soap.metadata.LiveChatButtonDeployments)__typeMapper.readObject(__in, _lookupTypeInfo("deployments", "http://soap.sforce.com/2006/04/metadata","deployments","http://soap.sforce.com/2006/04/metadata","LiveChatButtonDeployments",0,1,true), com.sforce.soap.metadata.LiveChatButtonDeployments.class));
}
}
private void writeFieldDeployments(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("deployments", "http://soap.sforce.com/2006/04/metadata","deployments","http://soap.sforce.com/2006/04/metadata","LiveChatButtonDeployments",0,1,true), deployments, deployments__is_set);
}
/**
* element : enableQueue of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean enableQueue__is_set = false;
private boolean enableQueue;
public boolean getEnableQueue() {
return enableQueue;
}
public boolean isEnableQueue() {
return enableQueue;
}
public void setEnableQueue(boolean enableQueue) {
this.enableQueue = enableQueue;
enableQueue__is_set = true;
}
protected void setEnableQueue(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("enableQueue", "http://soap.sforce.com/2006/04/metadata","enableQueue","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setEnableQueue(__typeMapper.readBoolean(__in, _lookupTypeInfo("enableQueue", "http://soap.sforce.com/2006/04/metadata","enableQueue","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldEnableQueue(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("enableQueue", "http://soap.sforce.com/2006/04/metadata","enableQueue","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), enableQueue, enableQueue__is_set);
}
/**
* element : inviteEndPosition of type {http://soap.sforce.com/2006/04/metadata}LiveChatButtonInviteEndPosition
* java type: com.sforce.soap.metadata.LiveChatButtonInviteEndPosition
*/
private boolean inviteEndPosition__is_set = false;
private com.sforce.soap.metadata.LiveChatButtonInviteEndPosition inviteEndPosition;
public com.sforce.soap.metadata.LiveChatButtonInviteEndPosition getInviteEndPosition() {
return inviteEndPosition;
}
public void setInviteEndPosition(com.sforce.soap.metadata.LiveChatButtonInviteEndPosition inviteEndPosition) {
this.inviteEndPosition = inviteEndPosition;
inviteEndPosition__is_set = true;
}
protected void setInviteEndPosition(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("inviteEndPosition", "http://soap.sforce.com/2006/04/metadata","inviteEndPosition","http://soap.sforce.com/2006/04/metadata","LiveChatButtonInviteEndPosition",0,1,true))) {
setInviteEndPosition((com.sforce.soap.metadata.LiveChatButtonInviteEndPosition)__typeMapper.readObject(__in, _lookupTypeInfo("inviteEndPosition", "http://soap.sforce.com/2006/04/metadata","inviteEndPosition","http://soap.sforce.com/2006/04/metadata","LiveChatButtonInviteEndPosition",0,1,true), com.sforce.soap.metadata.LiveChatButtonInviteEndPosition.class));
}
}
private void writeFieldInviteEndPosition(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("inviteEndPosition", "http://soap.sforce.com/2006/04/metadata","inviteEndPosition","http://soap.sforce.com/2006/04/metadata","LiveChatButtonInviteEndPosition",0,1,true), inviteEndPosition, inviteEndPosition__is_set);
}
/**
* element : inviteImage of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean inviteImage__is_set = false;
private java.lang.String inviteImage;
public java.lang.String getInviteImage() {
return inviteImage;
}
public void setInviteImage(java.lang.String inviteImage) {
this.inviteImage = inviteImage;
inviteImage__is_set = true;
}
protected void setInviteImage(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("inviteImage", "http://soap.sforce.com/2006/04/metadata","inviteImage","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setInviteImage(__typeMapper.readString(__in, _lookupTypeInfo("inviteImage", "http://soap.sforce.com/2006/04/metadata","inviteImage","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldInviteImage(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("inviteImage", "http://soap.sforce.com/2006/04/metadata","inviteImage","http://www.w3.org/2001/XMLSchema","string",0,1,true), inviteImage, inviteImage__is_set);
}
/**
* element : inviteStartPosition of type {http://soap.sforce.com/2006/04/metadata}LiveChatButtonInviteStartPosition
* java type: com.sforce.soap.metadata.LiveChatButtonInviteStartPosition
*/
private boolean inviteStartPosition__is_set = false;
private com.sforce.soap.metadata.LiveChatButtonInviteStartPosition inviteStartPosition;
public com.sforce.soap.metadata.LiveChatButtonInviteStartPosition getInviteStartPosition() {
return inviteStartPosition;
}
public void setInviteStartPosition(com.sforce.soap.metadata.LiveChatButtonInviteStartPosition inviteStartPosition) {
this.inviteStartPosition = inviteStartPosition;
inviteStartPosition__is_set = true;
}
protected void setInviteStartPosition(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("inviteStartPosition", "http://soap.sforce.com/2006/04/metadata","inviteStartPosition","http://soap.sforce.com/2006/04/metadata","LiveChatButtonInviteStartPosition",0,1,true))) {
setInviteStartPosition((com.sforce.soap.metadata.LiveChatButtonInviteStartPosition)__typeMapper.readObject(__in, _lookupTypeInfo("inviteStartPosition", "http://soap.sforce.com/2006/04/metadata","inviteStartPosition","http://soap.sforce.com/2006/04/metadata","LiveChatButtonInviteStartPosition",0,1,true), com.sforce.soap.metadata.LiveChatButtonInviteStartPosition.class));
}
}
private void writeFieldInviteStartPosition(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("inviteStartPosition", "http://soap.sforce.com/2006/04/metadata","inviteStartPosition","http://soap.sforce.com/2006/04/metadata","LiveChatButtonInviteStartPosition",0,1,true), inviteStartPosition, inviteStartPosition__is_set);
}
/**
* element : isActive of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean isActive__is_set = false;
private boolean isActive;
public boolean getIsActive() {
return isActive;
}
public boolean isIsActive() {
return isActive;
}
public void setIsActive(boolean isActive) {
this.isActive = isActive;
isActive__is_set = true;
}
protected void setIsActive(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("isActive", "http://soap.sforce.com/2006/04/metadata","isActive","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setIsActive(__typeMapper.readBoolean(__in, _lookupTypeInfo("isActive", "http://soap.sforce.com/2006/04/metadata","isActive","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldIsActive(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("isActive", "http://soap.sforce.com/2006/04/metadata","isActive","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), isActive, isActive__is_set);
}
/**
* element : label of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean label__is_set = false;
private java.lang.String label;
public java.lang.String getLabel() {
return label;
}
public void setLabel(java.lang.String label) {
this.label = label;
label__is_set = true;
}
protected void setLabel(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("label", "http://soap.sforce.com/2006/04/metadata","label","http://www.w3.org/2001/XMLSchema","string",1,1,true))) {
setLabel(__typeMapper.readString(__in, _lookupTypeInfo("label", "http://soap.sforce.com/2006/04/metadata","label","http://www.w3.org/2001/XMLSchema","string",1,1,true), java.lang.String.class));
}
}
private void writeFieldLabel(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("label", "http://soap.sforce.com/2006/04/metadata","label","http://www.w3.org/2001/XMLSchema","string",1,1,true), label, label__is_set);
}
/**
* element : numberOfReroutingAttempts of type {http://www.w3.org/2001/XMLSchema}int
* java type: int
*/
private boolean numberOfReroutingAttempts__is_set = false;
private int numberOfReroutingAttempts;
public int getNumberOfReroutingAttempts() {
return numberOfReroutingAttempts;
}
public void setNumberOfReroutingAttempts(int numberOfReroutingAttempts) {
this.numberOfReroutingAttempts = numberOfReroutingAttempts;
numberOfReroutingAttempts__is_set = true;
}
protected void setNumberOfReroutingAttempts(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("numberOfReroutingAttempts", "http://soap.sforce.com/2006/04/metadata","numberOfReroutingAttempts","http://www.w3.org/2001/XMLSchema","int",0,1,true))) {
setNumberOfReroutingAttempts((int)__typeMapper.readInt(__in, _lookupTypeInfo("numberOfReroutingAttempts", "http://soap.sforce.com/2006/04/metadata","numberOfReroutingAttempts","http://www.w3.org/2001/XMLSchema","int",0,1,true), int.class));
}
}
private void writeFieldNumberOfReroutingAttempts(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("numberOfReroutingAttempts", "http://soap.sforce.com/2006/04/metadata","numberOfReroutingAttempts","http://www.w3.org/2001/XMLSchema","int",0,1,true), numberOfReroutingAttempts, numberOfReroutingAttempts__is_set);
}
/**
* element : offlineImage of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean offlineImage__is_set = false;
private java.lang.String offlineImage;
public java.lang.String getOfflineImage() {
return offlineImage;
}
public void setOfflineImage(java.lang.String offlineImage) {
this.offlineImage = offlineImage;
offlineImage__is_set = true;
}
protected void setOfflineImage(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("offlineImage", "http://soap.sforce.com/2006/04/metadata","offlineImage","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setOfflineImage(__typeMapper.readString(__in, _lookupTypeInfo("offlineImage", "http://soap.sforce.com/2006/04/metadata","offlineImage","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldOfflineImage(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("offlineImage", "http://soap.sforce.com/2006/04/metadata","offlineImage","http://www.w3.org/2001/XMLSchema","string",0,1,true), offlineImage, offlineImage__is_set);
}
/**
* element : onlineImage of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean onlineImage__is_set = false;
private java.lang.String onlineImage;
public java.lang.String getOnlineImage() {
return onlineImage;
}
public void setOnlineImage(java.lang.String onlineImage) {
this.onlineImage = onlineImage;
onlineImage__is_set = true;
}
protected void setOnlineImage(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("onlineImage", "http://soap.sforce.com/2006/04/metadata","onlineImage","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setOnlineImage(__typeMapper.readString(__in, _lookupTypeInfo("onlineImage", "http://soap.sforce.com/2006/04/metadata","onlineImage","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldOnlineImage(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("onlineImage", "http://soap.sforce.com/2006/04/metadata","onlineImage","http://www.w3.org/2001/XMLSchema","string",0,1,true), onlineImage, onlineImage__is_set);
}
/**
* element : optionsCustomRoutingIsEnabled of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean optionsCustomRoutingIsEnabled__is_set = false;
private boolean optionsCustomRoutingIsEnabled;
public boolean getOptionsCustomRoutingIsEnabled() {
return optionsCustomRoutingIsEnabled;
}
public boolean isOptionsCustomRoutingIsEnabled() {
return optionsCustomRoutingIsEnabled;
}
public void setOptionsCustomRoutingIsEnabled(boolean optionsCustomRoutingIsEnabled) {
this.optionsCustomRoutingIsEnabled = optionsCustomRoutingIsEnabled;
optionsCustomRoutingIsEnabled__is_set = true;
}
protected void setOptionsCustomRoutingIsEnabled(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("optionsCustomRoutingIsEnabled", "http://soap.sforce.com/2006/04/metadata","optionsCustomRoutingIsEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setOptionsCustomRoutingIsEnabled(__typeMapper.readBoolean(__in, _lookupTypeInfo("optionsCustomRoutingIsEnabled", "http://soap.sforce.com/2006/04/metadata","optionsCustomRoutingIsEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldOptionsCustomRoutingIsEnabled(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("optionsCustomRoutingIsEnabled", "http://soap.sforce.com/2006/04/metadata","optionsCustomRoutingIsEnabled","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), optionsCustomRoutingIsEnabled, optionsCustomRoutingIsEnabled__is_set);
}
/**
* element : optionsHasChasitorIdleTimeout of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean optionsHasChasitorIdleTimeout__is_set = false;
private boolean optionsHasChasitorIdleTimeout;
public boolean getOptionsHasChasitorIdleTimeout() {
return optionsHasChasitorIdleTimeout;
}
public boolean isOptionsHasChasitorIdleTimeout() {
return optionsHasChasitorIdleTimeout;
}
public void setOptionsHasChasitorIdleTimeout(boolean optionsHasChasitorIdleTimeout) {
this.optionsHasChasitorIdleTimeout = optionsHasChasitorIdleTimeout;
optionsHasChasitorIdleTimeout__is_set = true;
}
protected void setOptionsHasChasitorIdleTimeout(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("optionsHasChasitorIdleTimeout", "http://soap.sforce.com/2006/04/metadata","optionsHasChasitorIdleTimeout","http://www.w3.org/2001/XMLSchema","boolean",1,1,true))) {
setOptionsHasChasitorIdleTimeout(__typeMapper.readBoolean(__in, _lookupTypeInfo("optionsHasChasitorIdleTimeout", "http://soap.sforce.com/2006/04/metadata","optionsHasChasitorIdleTimeout","http://www.w3.org/2001/XMLSchema","boolean",1,1,true), boolean.class));
}
}
private void writeFieldOptionsHasChasitorIdleTimeout(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("optionsHasChasitorIdleTimeout", "http://soap.sforce.com/2006/04/metadata","optionsHasChasitorIdleTimeout","http://www.w3.org/2001/XMLSchema","boolean",1,1,true), optionsHasChasitorIdleTimeout, optionsHasChasitorIdleTimeout__is_set);
}
/**
* element : optionsHasInviteAfterAccept of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean optionsHasInviteAfterAccept__is_set = false;
private boolean optionsHasInviteAfterAccept;
public boolean getOptionsHasInviteAfterAccept() {
return optionsHasInviteAfterAccept;
}
public boolean isOptionsHasInviteAfterAccept() {
return optionsHasInviteAfterAccept;
}
public void setOptionsHasInviteAfterAccept(boolean optionsHasInviteAfterAccept) {
this.optionsHasInviteAfterAccept = optionsHasInviteAfterAccept;
optionsHasInviteAfterAccept__is_set = true;
}
protected void setOptionsHasInviteAfterAccept(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("optionsHasInviteAfterAccept", "http://soap.sforce.com/2006/04/metadata","optionsHasInviteAfterAccept","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setOptionsHasInviteAfterAccept(__typeMapper.readBoolean(__in, _lookupTypeInfo("optionsHasInviteAfterAccept", "http://soap.sforce.com/2006/04/metadata","optionsHasInviteAfterAccept","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldOptionsHasInviteAfterAccept(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("optionsHasInviteAfterAccept", "http://soap.sforce.com/2006/04/metadata","optionsHasInviteAfterAccept","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), optionsHasInviteAfterAccept, optionsHasInviteAfterAccept__is_set);
}
/**
* element : optionsHasInviteAfterReject of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean optionsHasInviteAfterReject__is_set = false;
private boolean optionsHasInviteAfterReject;
public boolean getOptionsHasInviteAfterReject() {
return optionsHasInviteAfterReject;
}
public boolean isOptionsHasInviteAfterReject() {
return optionsHasInviteAfterReject;
}
public void setOptionsHasInviteAfterReject(boolean optionsHasInviteAfterReject) {
this.optionsHasInviteAfterReject = optionsHasInviteAfterReject;
optionsHasInviteAfterReject__is_set = true;
}
protected void setOptionsHasInviteAfterReject(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("optionsHasInviteAfterReject", "http://soap.sforce.com/2006/04/metadata","optionsHasInviteAfterReject","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setOptionsHasInviteAfterReject(__typeMapper.readBoolean(__in, _lookupTypeInfo("optionsHasInviteAfterReject", "http://soap.sforce.com/2006/04/metadata","optionsHasInviteAfterReject","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldOptionsHasInviteAfterReject(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("optionsHasInviteAfterReject", "http://soap.sforce.com/2006/04/metadata","optionsHasInviteAfterReject","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), optionsHasInviteAfterReject, optionsHasInviteAfterReject__is_set);
}
/**
* element : optionsHasRerouteDeclinedRequest of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean optionsHasRerouteDeclinedRequest__is_set = false;
private boolean optionsHasRerouteDeclinedRequest;
public boolean getOptionsHasRerouteDeclinedRequest() {
return optionsHasRerouteDeclinedRequest;
}
public boolean isOptionsHasRerouteDeclinedRequest() {
return optionsHasRerouteDeclinedRequest;
}
public void setOptionsHasRerouteDeclinedRequest(boolean optionsHasRerouteDeclinedRequest) {
this.optionsHasRerouteDeclinedRequest = optionsHasRerouteDeclinedRequest;
optionsHasRerouteDeclinedRequest__is_set = true;
}
protected void setOptionsHasRerouteDeclinedRequest(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("optionsHasRerouteDeclinedRequest", "http://soap.sforce.com/2006/04/metadata","optionsHasRerouteDeclinedRequest","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setOptionsHasRerouteDeclinedRequest(__typeMapper.readBoolean(__in, _lookupTypeInfo("optionsHasRerouteDeclinedRequest", "http://soap.sforce.com/2006/04/metadata","optionsHasRerouteDeclinedRequest","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldOptionsHasRerouteDeclinedRequest(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("optionsHasRerouteDeclinedRequest", "http://soap.sforce.com/2006/04/metadata","optionsHasRerouteDeclinedRequest","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), optionsHasRerouteDeclinedRequest, optionsHasRerouteDeclinedRequest__is_set);
}
/**
* element : optionsIsAutoAccept of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean optionsIsAutoAccept__is_set = false;
private boolean optionsIsAutoAccept;
public boolean getOptionsIsAutoAccept() {
return optionsIsAutoAccept;
}
public boolean isOptionsIsAutoAccept() {
return optionsIsAutoAccept;
}
public void setOptionsIsAutoAccept(boolean optionsIsAutoAccept) {
this.optionsIsAutoAccept = optionsIsAutoAccept;
optionsIsAutoAccept__is_set = true;
}
protected void setOptionsIsAutoAccept(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("optionsIsAutoAccept", "http://soap.sforce.com/2006/04/metadata","optionsIsAutoAccept","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setOptionsIsAutoAccept(__typeMapper.readBoolean(__in, _lookupTypeInfo("optionsIsAutoAccept", "http://soap.sforce.com/2006/04/metadata","optionsIsAutoAccept","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldOptionsIsAutoAccept(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("optionsIsAutoAccept", "http://soap.sforce.com/2006/04/metadata","optionsIsAutoAccept","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), optionsIsAutoAccept, optionsIsAutoAccept__is_set);
}
/**
* element : optionsIsInviteAutoRemove of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean optionsIsInviteAutoRemove__is_set = false;
private boolean optionsIsInviteAutoRemove;
public boolean getOptionsIsInviteAutoRemove() {
return optionsIsInviteAutoRemove;
}
public boolean isOptionsIsInviteAutoRemove() {
return optionsIsInviteAutoRemove;
}
public void setOptionsIsInviteAutoRemove(boolean optionsIsInviteAutoRemove) {
this.optionsIsInviteAutoRemove = optionsIsInviteAutoRemove;
optionsIsInviteAutoRemove__is_set = true;
}
protected void setOptionsIsInviteAutoRemove(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("optionsIsInviteAutoRemove", "http://soap.sforce.com/2006/04/metadata","optionsIsInviteAutoRemove","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setOptionsIsInviteAutoRemove(__typeMapper.readBoolean(__in, _lookupTypeInfo("optionsIsInviteAutoRemove", "http://soap.sforce.com/2006/04/metadata","optionsIsInviteAutoRemove","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldOptionsIsInviteAutoRemove(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("optionsIsInviteAutoRemove", "http://soap.sforce.com/2006/04/metadata","optionsIsInviteAutoRemove","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), optionsIsInviteAutoRemove, optionsIsInviteAutoRemove__is_set);
}
/**
* element : overallQueueLength of type {http://www.w3.org/2001/XMLSchema}int
* java type: int
*/
private boolean overallQueueLength__is_set = false;
private int overallQueueLength;
public int getOverallQueueLength() {
return overallQueueLength;
}
public void setOverallQueueLength(int overallQueueLength) {
this.overallQueueLength = overallQueueLength;
overallQueueLength__is_set = true;
}
protected void setOverallQueueLength(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("overallQueueLength", "http://soap.sforce.com/2006/04/metadata","overallQueueLength","http://www.w3.org/2001/XMLSchema","int",0,1,true))) {
setOverallQueueLength((int)__typeMapper.readInt(__in, _lookupTypeInfo("overallQueueLength", "http://soap.sforce.com/2006/04/metadata","overallQueueLength","http://www.w3.org/2001/XMLSchema","int",0,1,true), int.class));
}
}
private void writeFieldOverallQueueLength(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("overallQueueLength", "http://soap.sforce.com/2006/04/metadata","overallQueueLength","http://www.w3.org/2001/XMLSchema","int",0,1,true), overallQueueLength, overallQueueLength__is_set);
}
/**
* element : perAgentQueueLength of type {http://www.w3.org/2001/XMLSchema}int
* java type: int
*/
private boolean perAgentQueueLength__is_set = false;
private int perAgentQueueLength;
public int getPerAgentQueueLength() {
return perAgentQueueLength;
}
public void setPerAgentQueueLength(int perAgentQueueLength) {
this.perAgentQueueLength = perAgentQueueLength;
perAgentQueueLength__is_set = true;
}
protected void setPerAgentQueueLength(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("perAgentQueueLength", "http://soap.sforce.com/2006/04/metadata","perAgentQueueLength","http://www.w3.org/2001/XMLSchema","int",0,1,true))) {
setPerAgentQueueLength((int)__typeMapper.readInt(__in, _lookupTypeInfo("perAgentQueueLength", "http://soap.sforce.com/2006/04/metadata","perAgentQueueLength","http://www.w3.org/2001/XMLSchema","int",0,1,true), int.class));
}
}
private void writeFieldPerAgentQueueLength(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("perAgentQueueLength", "http://soap.sforce.com/2006/04/metadata","perAgentQueueLength","http://www.w3.org/2001/XMLSchema","int",0,1,true), perAgentQueueLength, perAgentQueueLength__is_set);
}
/**
* element : postChatPage of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean postChatPage__is_set = false;
private java.lang.String postChatPage;
public java.lang.String getPostChatPage() {
return postChatPage;
}
public void setPostChatPage(java.lang.String postChatPage) {
this.postChatPage = postChatPage;
postChatPage__is_set = true;
}
protected void setPostChatPage(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("postChatPage", "http://soap.sforce.com/2006/04/metadata","postChatPage","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setPostChatPage(__typeMapper.readString(__in, _lookupTypeInfo("postChatPage", "http://soap.sforce.com/2006/04/metadata","postChatPage","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldPostChatPage(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("postChatPage", "http://soap.sforce.com/2006/04/metadata","postChatPage","http://www.w3.org/2001/XMLSchema","string",0,1,true), postChatPage, postChatPage__is_set);
}
/**
* element : postChatUrl of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean postChatUrl__is_set = false;
private java.lang.String postChatUrl;
public java.lang.String getPostChatUrl() {
return postChatUrl;
}
public void setPostChatUrl(java.lang.String postChatUrl) {
this.postChatUrl = postChatUrl;
postChatUrl__is_set = true;
}
protected void setPostChatUrl(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("postChatUrl", "http://soap.sforce.com/2006/04/metadata","postChatUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setPostChatUrl(__typeMapper.readString(__in, _lookupTypeInfo("postChatUrl", "http://soap.sforce.com/2006/04/metadata","postChatUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldPostChatUrl(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("postChatUrl", "http://soap.sforce.com/2006/04/metadata","postChatUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), postChatUrl, postChatUrl__is_set);
}
/**
* element : preChatFormPage of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean preChatFormPage__is_set = false;
private java.lang.String preChatFormPage;
public java.lang.String getPreChatFormPage() {
return preChatFormPage;
}
public void setPreChatFormPage(java.lang.String preChatFormPage) {
this.preChatFormPage = preChatFormPage;
preChatFormPage__is_set = true;
}
protected void setPreChatFormPage(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("preChatFormPage", "http://soap.sforce.com/2006/04/metadata","preChatFormPage","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setPreChatFormPage(__typeMapper.readString(__in, _lookupTypeInfo("preChatFormPage", "http://soap.sforce.com/2006/04/metadata","preChatFormPage","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldPreChatFormPage(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("preChatFormPage", "http://soap.sforce.com/2006/04/metadata","preChatFormPage","http://www.w3.org/2001/XMLSchema","string",0,1,true), preChatFormPage, preChatFormPage__is_set);
}
/**
* element : preChatFormUrl of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean preChatFormUrl__is_set = false;
private java.lang.String preChatFormUrl;
public java.lang.String getPreChatFormUrl() {
return preChatFormUrl;
}
public void setPreChatFormUrl(java.lang.String preChatFormUrl) {
this.preChatFormUrl = preChatFormUrl;
preChatFormUrl__is_set = true;
}
protected void setPreChatFormUrl(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("preChatFormUrl", "http://soap.sforce.com/2006/04/metadata","preChatFormUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setPreChatFormUrl(__typeMapper.readString(__in, _lookupTypeInfo("preChatFormUrl", "http://soap.sforce.com/2006/04/metadata","preChatFormUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldPreChatFormUrl(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("preChatFormUrl", "http://soap.sforce.com/2006/04/metadata","preChatFormUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), preChatFormUrl, preChatFormUrl__is_set);
}
/**
* element : pushTimeOut of type {http://www.w3.org/2001/XMLSchema}int
* java type: int
*/
private boolean pushTimeOut__is_set = false;
private int pushTimeOut;
public int getPushTimeOut() {
return pushTimeOut;
}
public void setPushTimeOut(int pushTimeOut) {
this.pushTimeOut = pushTimeOut;
pushTimeOut__is_set = true;
}
protected void setPushTimeOut(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("pushTimeOut", "http://soap.sforce.com/2006/04/metadata","pushTimeOut","http://www.w3.org/2001/XMLSchema","int",0,1,true))) {
setPushTimeOut((int)__typeMapper.readInt(__in, _lookupTypeInfo("pushTimeOut", "http://soap.sforce.com/2006/04/metadata","pushTimeOut","http://www.w3.org/2001/XMLSchema","int",0,1,true), int.class));
}
}
private void writeFieldPushTimeOut(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("pushTimeOut", "http://soap.sforce.com/2006/04/metadata","pushTimeOut","http://www.w3.org/2001/XMLSchema","int",0,1,true), pushTimeOut, pushTimeOut__is_set);
}
/**
* element : routingType of type {http://soap.sforce.com/2006/04/metadata}LiveChatButtonRoutingType
* java type: com.sforce.soap.metadata.LiveChatButtonRoutingType
*/
private boolean routingType__is_set = false;
private com.sforce.soap.metadata.LiveChatButtonRoutingType routingType;
public com.sforce.soap.metadata.LiveChatButtonRoutingType getRoutingType() {
return routingType;
}
public void setRoutingType(com.sforce.soap.metadata.LiveChatButtonRoutingType routingType) {
this.routingType = routingType;
routingType__is_set = true;
}
protected void setRoutingType(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("routingType", "http://soap.sforce.com/2006/04/metadata","routingType","http://soap.sforce.com/2006/04/metadata","LiveChatButtonRoutingType",1,1,true))) {
setRoutingType((com.sforce.soap.metadata.LiveChatButtonRoutingType)__typeMapper.readObject(__in, _lookupTypeInfo("routingType", "http://soap.sforce.com/2006/04/metadata","routingType","http://soap.sforce.com/2006/04/metadata","LiveChatButtonRoutingType",1,1,true), com.sforce.soap.metadata.LiveChatButtonRoutingType.class));
}
}
private void writeFieldRoutingType(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("routingType", "http://soap.sforce.com/2006/04/metadata","routingType","http://soap.sforce.com/2006/04/metadata","LiveChatButtonRoutingType",1,1,true), routingType, routingType__is_set);
}
/**
* element : site of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean site__is_set = false;
private java.lang.String site;
public java.lang.String getSite() {
return site;
}
public void setSite(java.lang.String site) {
this.site = site;
site__is_set = true;
}
protected void setSite(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("site", "http://soap.sforce.com/2006/04/metadata","site","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setSite(__typeMapper.readString(__in, _lookupTypeInfo("site", "http://soap.sforce.com/2006/04/metadata","site","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldSite(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("site", "http://soap.sforce.com/2006/04/metadata","site","http://www.w3.org/2001/XMLSchema","string",0,1,true), site, site__is_set);
}
/**
* element : skills of type {http://soap.sforce.com/2006/04/metadata}LiveChatButtonSkills
* java type: com.sforce.soap.metadata.LiveChatButtonSkills
*/
private boolean skills__is_set = false;
private com.sforce.soap.metadata.LiveChatButtonSkills skills;
public com.sforce.soap.metadata.LiveChatButtonSkills getSkills() {
return skills;
}
public void setSkills(com.sforce.soap.metadata.LiveChatButtonSkills skills) {
this.skills = skills;
skills__is_set = true;
}
protected void setSkills(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("skills", "http://soap.sforce.com/2006/04/metadata","skills","http://soap.sforce.com/2006/04/metadata","LiveChatButtonSkills",0,1,true))) {
setSkills((com.sforce.soap.metadata.LiveChatButtonSkills)__typeMapper.readObject(__in, _lookupTypeInfo("skills", "http://soap.sforce.com/2006/04/metadata","skills","http://soap.sforce.com/2006/04/metadata","LiveChatButtonSkills",0,1,true), com.sforce.soap.metadata.LiveChatButtonSkills.class));
}
}
private void writeFieldSkills(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("skills", "http://soap.sforce.com/2006/04/metadata","skills","http://soap.sforce.com/2006/04/metadata","LiveChatButtonSkills",0,1,true), skills, skills__is_set);
}
/**
* element : timeToRemoveInvite of type {http://www.w3.org/2001/XMLSchema}int
* java type: int
*/
private boolean timeToRemoveInvite__is_set = false;
private int timeToRemoveInvite;
public int getTimeToRemoveInvite() {
return timeToRemoveInvite;
}
public void setTimeToRemoveInvite(int timeToRemoveInvite) {
this.timeToRemoveInvite = timeToRemoveInvite;
timeToRemoveInvite__is_set = true;
}
protected void setTimeToRemoveInvite(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("timeToRemoveInvite", "http://soap.sforce.com/2006/04/metadata","timeToRemoveInvite","http://www.w3.org/2001/XMLSchema","int",0,1,true))) {
setTimeToRemoveInvite((int)__typeMapper.readInt(__in, _lookupTypeInfo("timeToRemoveInvite", "http://soap.sforce.com/2006/04/metadata","timeToRemoveInvite","http://www.w3.org/2001/XMLSchema","int",0,1,true), int.class));
}
}
private void writeFieldTimeToRemoveInvite(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("timeToRemoveInvite", "http://soap.sforce.com/2006/04/metadata","timeToRemoveInvite","http://www.w3.org/2001/XMLSchema","int",0,1,true), timeToRemoveInvite, timeToRemoveInvite__is_set);
}
/**
* element : type of type {http://soap.sforce.com/2006/04/metadata}LiveChatButtonType
* java type: com.sforce.soap.metadata.LiveChatButtonType
*/
private boolean type__is_set = false;
private com.sforce.soap.metadata.LiveChatButtonType type;
public com.sforce.soap.metadata.LiveChatButtonType getType() {
return type;
}
public void setType(com.sforce.soap.metadata.LiveChatButtonType type) {
this.type = type;
type__is_set = true;
}
protected void setType(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("type", "http://soap.sforce.com/2006/04/metadata","type","http://soap.sforce.com/2006/04/metadata","LiveChatButtonType",1,1,true))) {
setType((com.sforce.soap.metadata.LiveChatButtonType)__typeMapper.readObject(__in, _lookupTypeInfo("type", "http://soap.sforce.com/2006/04/metadata","type","http://soap.sforce.com/2006/04/metadata","LiveChatButtonType",1,1,true), com.sforce.soap.metadata.LiveChatButtonType.class));
}
}
private void writeFieldType(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("type", "http://soap.sforce.com/2006/04/metadata","type","http://soap.sforce.com/2006/04/metadata","LiveChatButtonType",1,1,true), type, type__is_set);
}
/**
* element : windowLanguage of type {http://soap.sforce.com/2006/04/metadata}Language
* java type: com.sforce.soap.metadata.Language
*/
private boolean windowLanguage__is_set = false;
private com.sforce.soap.metadata.Language windowLanguage;
public com.sforce.soap.metadata.Language getWindowLanguage() {
return windowLanguage;
}
public void setWindowLanguage(com.sforce.soap.metadata.Language windowLanguage) {
this.windowLanguage = windowLanguage;
windowLanguage__is_set = true;
}
protected void setWindowLanguage(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("windowLanguage", "http://soap.sforce.com/2006/04/metadata","windowLanguage","http://soap.sforce.com/2006/04/metadata","Language",0,1,true))) {
setWindowLanguage((com.sforce.soap.metadata.Language)__typeMapper.readObject(__in, _lookupTypeInfo("windowLanguage", "http://soap.sforce.com/2006/04/metadata","windowLanguage","http://soap.sforce.com/2006/04/metadata","Language",0,1,true), com.sforce.soap.metadata.Language.class));
}
}
private void writeFieldWindowLanguage(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("windowLanguage", "http://soap.sforce.com/2006/04/metadata","windowLanguage","http://soap.sforce.com/2006/04/metadata","Language",0,1,true), windowLanguage, windowLanguage__is_set);
}
/**
*/
@Override
public void write(javax.xml.namespace.QName __element,
com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
__out.writeStartTag(__element.getNamespaceURI(), __element.getLocalPart());
__typeMapper.writeXsiType(__out, "http://soap.sforce.com/2006/04/metadata", "LiveChatButton");
writeFields(__out, __typeMapper);
__out.writeEndTag(__element.getNamespaceURI(), __element.getLocalPart());
}
protected void writeFields(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
super.writeFields(__out, __typeMapper);
writeFields1(__out, __typeMapper);
}
@Override
public void load(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__typeMapper.consumeStartTag(__in);
loadFields(__in, __typeMapper);
__typeMapper.consumeEndTag(__in);
}
protected void loadFields(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
super.loadFields(__in, __typeMapper);
loadFields1(__in, __typeMapper);
}
@Override
public String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("[LiveChatButton ");
sb.append(super.toString());
toString1(sb);
sb.append("]\n");
return sb.toString();
}
private void toStringHelper(StringBuilder sb, String name, Object value) {
sb.append(' ').append(name).append("='").append(com.sforce.ws.util.Verbose.toString(value)).append("'\n");
}
private void writeFields1(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
writeFieldAnimation(__out, __typeMapper);
writeFieldAutoGreeting(__out, __typeMapper);
writeFieldChasitorIdleTimeout(__out, __typeMapper);
writeFieldChasitorIdleTimeoutWarning(__out, __typeMapper);
writeFieldChatPage(__out, __typeMapper);
writeFieldCustomAgentName(__out, __typeMapper);
writeFieldDeployments(__out, __typeMapper);
writeFieldEnableQueue(__out, __typeMapper);
writeFieldInviteEndPosition(__out, __typeMapper);
writeFieldInviteImage(__out, __typeMapper);
writeFieldInviteStartPosition(__out, __typeMapper);
writeFieldIsActive(__out, __typeMapper);
writeFieldLabel(__out, __typeMapper);
writeFieldNumberOfReroutingAttempts(__out, __typeMapper);
writeFieldOfflineImage(__out, __typeMapper);
writeFieldOnlineImage(__out, __typeMapper);
writeFieldOptionsCustomRoutingIsEnabled(__out, __typeMapper);
writeFieldOptionsHasChasitorIdleTimeout(__out, __typeMapper);
writeFieldOptionsHasInviteAfterAccept(__out, __typeMapper);
writeFieldOptionsHasInviteAfterReject(__out, __typeMapper);
writeFieldOptionsHasRerouteDeclinedRequest(__out, __typeMapper);
writeFieldOptionsIsAutoAccept(__out, __typeMapper);
writeFieldOptionsIsInviteAutoRemove(__out, __typeMapper);
writeFieldOverallQueueLength(__out, __typeMapper);
writeFieldPerAgentQueueLength(__out, __typeMapper);
writeFieldPostChatPage(__out, __typeMapper);
writeFieldPostChatUrl(__out, __typeMapper);
writeFieldPreChatFormPage(__out, __typeMapper);
writeFieldPreChatFormUrl(__out, __typeMapper);
writeFieldPushTimeOut(__out, __typeMapper);
writeFieldRoutingType(__out, __typeMapper);
writeFieldSite(__out, __typeMapper);
writeFieldSkills(__out, __typeMapper);
writeFieldTimeToRemoveInvite(__out, __typeMapper);
writeFieldType(__out, __typeMapper);
writeFieldWindowLanguage(__out, __typeMapper);
}
private void loadFields1(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
setAnimation(__in, __typeMapper);
setAutoGreeting(__in, __typeMapper);
setChasitorIdleTimeout(__in, __typeMapper);
setChasitorIdleTimeoutWarning(__in, __typeMapper);
setChatPage(__in, __typeMapper);
setCustomAgentName(__in, __typeMapper);
setDeployments(__in, __typeMapper);
setEnableQueue(__in, __typeMapper);
setInviteEndPosition(__in, __typeMapper);
setInviteImage(__in, __typeMapper);
setInviteStartPosition(__in, __typeMapper);
setIsActive(__in, __typeMapper);
setLabel(__in, __typeMapper);
setNumberOfReroutingAttempts(__in, __typeMapper);
setOfflineImage(__in, __typeMapper);
setOnlineImage(__in, __typeMapper);
setOptionsCustomRoutingIsEnabled(__in, __typeMapper);
setOptionsHasChasitorIdleTimeout(__in, __typeMapper);
setOptionsHasInviteAfterAccept(__in, __typeMapper);
setOptionsHasInviteAfterReject(__in, __typeMapper);
setOptionsHasRerouteDeclinedRequest(__in, __typeMapper);
setOptionsIsAutoAccept(__in, __typeMapper);
setOptionsIsInviteAutoRemove(__in, __typeMapper);
setOverallQueueLength(__in, __typeMapper);
setPerAgentQueueLength(__in, __typeMapper);
setPostChatPage(__in, __typeMapper);
setPostChatUrl(__in, __typeMapper);
setPreChatFormPage(__in, __typeMapper);
setPreChatFormUrl(__in, __typeMapper);
setPushTimeOut(__in, __typeMapper);
setRoutingType(__in, __typeMapper);
setSite(__in, __typeMapper);
setSkills(__in, __typeMapper);
setTimeToRemoveInvite(__in, __typeMapper);
setType(__in, __typeMapper);
setWindowLanguage(__in, __typeMapper);
}
private void toString1(StringBuilder sb) {
toStringHelper(sb, "animation", animation);
toStringHelper(sb, "autoGreeting", autoGreeting);
toStringHelper(sb, "chasitorIdleTimeout", chasitorIdleTimeout);
toStringHelper(sb, "chasitorIdleTimeoutWarning", chasitorIdleTimeoutWarning);
toStringHelper(sb, "chatPage", chatPage);
toStringHelper(sb, "customAgentName", customAgentName);
toStringHelper(sb, "deployments", deployments);
toStringHelper(sb, "enableQueue", enableQueue);
toStringHelper(sb, "inviteEndPosition", inviteEndPosition);
toStringHelper(sb, "inviteImage", inviteImage);
toStringHelper(sb, "inviteStartPosition", inviteStartPosition);
toStringHelper(sb, "isActive", isActive);
toStringHelper(sb, "label", label);
toStringHelper(sb, "numberOfReroutingAttempts", numberOfReroutingAttempts);
toStringHelper(sb, "offlineImage", offlineImage);
toStringHelper(sb, "onlineImage", onlineImage);
toStringHelper(sb, "optionsCustomRoutingIsEnabled", optionsCustomRoutingIsEnabled);
toStringHelper(sb, "optionsHasChasitorIdleTimeout", optionsHasChasitorIdleTimeout);
toStringHelper(sb, "optionsHasInviteAfterAccept", optionsHasInviteAfterAccept);
toStringHelper(sb, "optionsHasInviteAfterReject", optionsHasInviteAfterReject);
toStringHelper(sb, "optionsHasRerouteDeclinedRequest", optionsHasRerouteDeclinedRequest);
toStringHelper(sb, "optionsIsAutoAccept", optionsIsAutoAccept);
toStringHelper(sb, "optionsIsInviteAutoRemove", optionsIsInviteAutoRemove);
toStringHelper(sb, "overallQueueLength", overallQueueLength);
toStringHelper(sb, "perAgentQueueLength", perAgentQueueLength);
toStringHelper(sb, "postChatPage", postChatPage);
toStringHelper(sb, "postChatUrl", postChatUrl);
toStringHelper(sb, "preChatFormPage", preChatFormPage);
toStringHelper(sb, "preChatFormUrl", preChatFormUrl);
toStringHelper(sb, "pushTimeOut", pushTimeOut);
toStringHelper(sb, "routingType", routingType);
toStringHelper(sb, "site", site);
toStringHelper(sb, "skills", skills);
toStringHelper(sb, "timeToRemoveInvite", timeToRemoveInvite);
toStringHelper(sb, "type", type);
toStringHelper(sb, "windowLanguage", windowLanguage);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy