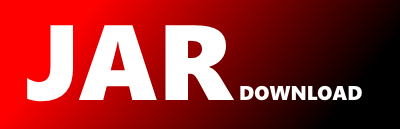
com.sforce.soap.metadata.SamlSsoConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of force-metadata-api Show documentation
Show all versions of force-metadata-api Show documentation
Force.com Web Service Connector
The newest version!
package com.sforce.soap.metadata;
/**
* This is a generated class for the SObject Enterprise API.
* Do not edit this file, as your changes will be lost.
*/
public class SamlSsoConfig extends com.sforce.soap.metadata.Metadata {
/**
* Constructor
*/
public SamlSsoConfig() {}
/* Cache the typeInfo instead of declaring static fields throughout*/
private transient java.util.Map typeInfoCache = new java.util.HashMap();
private com.sforce.ws.bind.TypeInfo _lookupTypeInfo(String fieldName, String namespace, String name, String typeNS, String type, int minOcc, int maxOcc, boolean elementForm) {
com.sforce.ws.bind.TypeInfo typeInfo = typeInfoCache.get(fieldName);
if (typeInfo == null) {
typeInfo = new com.sforce.ws.bind.TypeInfo(namespace, name, typeNS, type, minOcc, maxOcc, elementForm);
typeInfoCache.put(fieldName, typeInfo);
}
return typeInfo;
}
/**
* element : attributeName of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean attributeName__is_set = false;
private java.lang.String attributeName;
public java.lang.String getAttributeName() {
return attributeName;
}
public void setAttributeName(java.lang.String attributeName) {
this.attributeName = attributeName;
attributeName__is_set = true;
}
protected void setAttributeName(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("attributeName", "http://soap.sforce.com/2006/04/metadata","attributeName","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setAttributeName(__typeMapper.readString(__in, _lookupTypeInfo("attributeName", "http://soap.sforce.com/2006/04/metadata","attributeName","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldAttributeName(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("attributeName", "http://soap.sforce.com/2006/04/metadata","attributeName","http://www.w3.org/2001/XMLSchema","string",0,1,true), attributeName, attributeName__is_set);
}
/**
* element : attributeNameIdFormat of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean attributeNameIdFormat__is_set = false;
private java.lang.String attributeNameIdFormat;
public java.lang.String getAttributeNameIdFormat() {
return attributeNameIdFormat;
}
public void setAttributeNameIdFormat(java.lang.String attributeNameIdFormat) {
this.attributeNameIdFormat = attributeNameIdFormat;
attributeNameIdFormat__is_set = true;
}
protected void setAttributeNameIdFormat(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("attributeNameIdFormat", "http://soap.sforce.com/2006/04/metadata","attributeNameIdFormat","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setAttributeNameIdFormat(__typeMapper.readString(__in, _lookupTypeInfo("attributeNameIdFormat", "http://soap.sforce.com/2006/04/metadata","attributeNameIdFormat","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldAttributeNameIdFormat(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("attributeNameIdFormat", "http://soap.sforce.com/2006/04/metadata","attributeNameIdFormat","http://www.w3.org/2001/XMLSchema","string",0,1,true), attributeNameIdFormat, attributeNameIdFormat__is_set);
}
/**
* element : decryptionCertificate of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean decryptionCertificate__is_set = false;
private java.lang.String decryptionCertificate;
public java.lang.String getDecryptionCertificate() {
return decryptionCertificate;
}
public void setDecryptionCertificate(java.lang.String decryptionCertificate) {
this.decryptionCertificate = decryptionCertificate;
decryptionCertificate__is_set = true;
}
protected void setDecryptionCertificate(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("decryptionCertificate", "http://soap.sforce.com/2006/04/metadata","decryptionCertificate","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setDecryptionCertificate(__typeMapper.readString(__in, _lookupTypeInfo("decryptionCertificate", "http://soap.sforce.com/2006/04/metadata","decryptionCertificate","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldDecryptionCertificate(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("decryptionCertificate", "http://soap.sforce.com/2006/04/metadata","decryptionCertificate","http://www.w3.org/2001/XMLSchema","string",0,1,true), decryptionCertificate, decryptionCertificate__is_set);
}
/**
* element : errorUrl of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean errorUrl__is_set = false;
private java.lang.String errorUrl;
public java.lang.String getErrorUrl() {
return errorUrl;
}
public void setErrorUrl(java.lang.String errorUrl) {
this.errorUrl = errorUrl;
errorUrl__is_set = true;
}
protected void setErrorUrl(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("errorUrl", "http://soap.sforce.com/2006/04/metadata","errorUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setErrorUrl(__typeMapper.readString(__in, _lookupTypeInfo("errorUrl", "http://soap.sforce.com/2006/04/metadata","errorUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldErrorUrl(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("errorUrl", "http://soap.sforce.com/2006/04/metadata","errorUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), errorUrl, errorUrl__is_set);
}
/**
* element : executionUserId of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean executionUserId__is_set = false;
private java.lang.String executionUserId;
public java.lang.String getExecutionUserId() {
return executionUserId;
}
public void setExecutionUserId(java.lang.String executionUserId) {
this.executionUserId = executionUserId;
executionUserId__is_set = true;
}
protected void setExecutionUserId(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("executionUserId", "http://soap.sforce.com/2006/04/metadata","executionUserId","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setExecutionUserId(__typeMapper.readString(__in, _lookupTypeInfo("executionUserId", "http://soap.sforce.com/2006/04/metadata","executionUserId","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldExecutionUserId(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("executionUserId", "http://soap.sforce.com/2006/04/metadata","executionUserId","http://www.w3.org/2001/XMLSchema","string",0,1,true), executionUserId, executionUserId__is_set);
}
/**
* element : identityLocation of type {http://soap.sforce.com/2006/04/metadata}SamlIdentityLocationType
* java type: com.sforce.soap.metadata.SamlIdentityLocationType
*/
private boolean identityLocation__is_set = false;
private com.sforce.soap.metadata.SamlIdentityLocationType identityLocation;
public com.sforce.soap.metadata.SamlIdentityLocationType getIdentityLocation() {
return identityLocation;
}
public void setIdentityLocation(com.sforce.soap.metadata.SamlIdentityLocationType identityLocation) {
this.identityLocation = identityLocation;
identityLocation__is_set = true;
}
protected void setIdentityLocation(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("identityLocation", "http://soap.sforce.com/2006/04/metadata","identityLocation","http://soap.sforce.com/2006/04/metadata","SamlIdentityLocationType",1,1,true))) {
setIdentityLocation((com.sforce.soap.metadata.SamlIdentityLocationType)__typeMapper.readObject(__in, _lookupTypeInfo("identityLocation", "http://soap.sforce.com/2006/04/metadata","identityLocation","http://soap.sforce.com/2006/04/metadata","SamlIdentityLocationType",1,1,true), com.sforce.soap.metadata.SamlIdentityLocationType.class));
}
}
private void writeFieldIdentityLocation(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("identityLocation", "http://soap.sforce.com/2006/04/metadata","identityLocation","http://soap.sforce.com/2006/04/metadata","SamlIdentityLocationType",1,1,true), identityLocation, identityLocation__is_set);
}
/**
* element : identityMapping of type {http://soap.sforce.com/2006/04/metadata}SamlIdentityType
* java type: com.sforce.soap.metadata.SamlIdentityType
*/
private boolean identityMapping__is_set = false;
private com.sforce.soap.metadata.SamlIdentityType identityMapping;
public com.sforce.soap.metadata.SamlIdentityType getIdentityMapping() {
return identityMapping;
}
public void setIdentityMapping(com.sforce.soap.metadata.SamlIdentityType identityMapping) {
this.identityMapping = identityMapping;
identityMapping__is_set = true;
}
protected void setIdentityMapping(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("identityMapping", "http://soap.sforce.com/2006/04/metadata","identityMapping","http://soap.sforce.com/2006/04/metadata","SamlIdentityType",1,1,true))) {
setIdentityMapping((com.sforce.soap.metadata.SamlIdentityType)__typeMapper.readObject(__in, _lookupTypeInfo("identityMapping", "http://soap.sforce.com/2006/04/metadata","identityMapping","http://soap.sforce.com/2006/04/metadata","SamlIdentityType",1,1,true), com.sforce.soap.metadata.SamlIdentityType.class));
}
}
private void writeFieldIdentityMapping(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("identityMapping", "http://soap.sforce.com/2006/04/metadata","identityMapping","http://soap.sforce.com/2006/04/metadata","SamlIdentityType",1,1,true), identityMapping, identityMapping__is_set);
}
/**
* element : issuer of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean issuer__is_set = false;
private java.lang.String issuer;
public java.lang.String getIssuer() {
return issuer;
}
public void setIssuer(java.lang.String issuer) {
this.issuer = issuer;
issuer__is_set = true;
}
protected void setIssuer(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("issuer", "http://soap.sforce.com/2006/04/metadata","issuer","http://www.w3.org/2001/XMLSchema","string",1,1,true))) {
setIssuer(__typeMapper.readString(__in, _lookupTypeInfo("issuer", "http://soap.sforce.com/2006/04/metadata","issuer","http://www.w3.org/2001/XMLSchema","string",1,1,true), java.lang.String.class));
}
}
private void writeFieldIssuer(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("issuer", "http://soap.sforce.com/2006/04/metadata","issuer","http://www.w3.org/2001/XMLSchema","string",1,1,true), issuer, issuer__is_set);
}
/**
* element : loginUrl of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean loginUrl__is_set = false;
private java.lang.String loginUrl;
public java.lang.String getLoginUrl() {
return loginUrl;
}
public void setLoginUrl(java.lang.String loginUrl) {
this.loginUrl = loginUrl;
loginUrl__is_set = true;
}
protected void setLoginUrl(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("loginUrl", "http://soap.sforce.com/2006/04/metadata","loginUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setLoginUrl(__typeMapper.readString(__in, _lookupTypeInfo("loginUrl", "http://soap.sforce.com/2006/04/metadata","loginUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldLoginUrl(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("loginUrl", "http://soap.sforce.com/2006/04/metadata","loginUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), loginUrl, loginUrl__is_set);
}
/**
* element : logoutUrl of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean logoutUrl__is_set = false;
private java.lang.String logoutUrl;
public java.lang.String getLogoutUrl() {
return logoutUrl;
}
public void setLogoutUrl(java.lang.String logoutUrl) {
this.logoutUrl = logoutUrl;
logoutUrl__is_set = true;
}
protected void setLogoutUrl(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("logoutUrl", "http://soap.sforce.com/2006/04/metadata","logoutUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setLogoutUrl(__typeMapper.readString(__in, _lookupTypeInfo("logoutUrl", "http://soap.sforce.com/2006/04/metadata","logoutUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldLogoutUrl(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("logoutUrl", "http://soap.sforce.com/2006/04/metadata","logoutUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), logoutUrl, logoutUrl__is_set);
}
/**
* element : name of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean name__is_set = false;
private java.lang.String name;
public java.lang.String getName() {
return name;
}
public void setName(java.lang.String name) {
this.name = name;
name__is_set = true;
}
protected void setName(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("name", "http://soap.sforce.com/2006/04/metadata","name","http://www.w3.org/2001/XMLSchema","string",1,1,true))) {
setName(__typeMapper.readString(__in, _lookupTypeInfo("name", "http://soap.sforce.com/2006/04/metadata","name","http://www.w3.org/2001/XMLSchema","string",1,1,true), java.lang.String.class));
}
}
private void writeFieldName(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("name", "http://soap.sforce.com/2006/04/metadata","name","http://www.w3.org/2001/XMLSchema","string",1,1,true), name, name__is_set);
}
/**
* element : oauthTokenEndpoint of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean oauthTokenEndpoint__is_set = false;
private java.lang.String oauthTokenEndpoint;
public java.lang.String getOauthTokenEndpoint() {
return oauthTokenEndpoint;
}
public void setOauthTokenEndpoint(java.lang.String oauthTokenEndpoint) {
this.oauthTokenEndpoint = oauthTokenEndpoint;
oauthTokenEndpoint__is_set = true;
}
protected void setOauthTokenEndpoint(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("oauthTokenEndpoint", "http://soap.sforce.com/2006/04/metadata","oauthTokenEndpoint","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setOauthTokenEndpoint(__typeMapper.readString(__in, _lookupTypeInfo("oauthTokenEndpoint", "http://soap.sforce.com/2006/04/metadata","oauthTokenEndpoint","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldOauthTokenEndpoint(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("oauthTokenEndpoint", "http://soap.sforce.com/2006/04/metadata","oauthTokenEndpoint","http://www.w3.org/2001/XMLSchema","string",0,1,true), oauthTokenEndpoint, oauthTokenEndpoint__is_set);
}
/**
* element : redirectBinding of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean redirectBinding__is_set = false;
private boolean redirectBinding;
public boolean getRedirectBinding() {
return redirectBinding;
}
public boolean isRedirectBinding() {
return redirectBinding;
}
public void setRedirectBinding(boolean redirectBinding) {
this.redirectBinding = redirectBinding;
redirectBinding__is_set = true;
}
protected void setRedirectBinding(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("redirectBinding", "http://soap.sforce.com/2006/04/metadata","redirectBinding","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setRedirectBinding(__typeMapper.readBoolean(__in, _lookupTypeInfo("redirectBinding", "http://soap.sforce.com/2006/04/metadata","redirectBinding","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldRedirectBinding(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("redirectBinding", "http://soap.sforce.com/2006/04/metadata","redirectBinding","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), redirectBinding, redirectBinding__is_set);
}
/**
* element : requestSignatureMethod of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean requestSignatureMethod__is_set = false;
private java.lang.String requestSignatureMethod;
public java.lang.String getRequestSignatureMethod() {
return requestSignatureMethod;
}
public void setRequestSignatureMethod(java.lang.String requestSignatureMethod) {
this.requestSignatureMethod = requestSignatureMethod;
requestSignatureMethod__is_set = true;
}
protected void setRequestSignatureMethod(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("requestSignatureMethod", "http://soap.sforce.com/2006/04/metadata","requestSignatureMethod","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setRequestSignatureMethod(__typeMapper.readString(__in, _lookupTypeInfo("requestSignatureMethod", "http://soap.sforce.com/2006/04/metadata","requestSignatureMethod","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldRequestSignatureMethod(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("requestSignatureMethod", "http://soap.sforce.com/2006/04/metadata","requestSignatureMethod","http://www.w3.org/2001/XMLSchema","string",0,1,true), requestSignatureMethod, requestSignatureMethod__is_set);
}
/**
* element : requestSigningCertId of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean requestSigningCertId__is_set = false;
private java.lang.String requestSigningCertId;
public java.lang.String getRequestSigningCertId() {
return requestSigningCertId;
}
public void setRequestSigningCertId(java.lang.String requestSigningCertId) {
this.requestSigningCertId = requestSigningCertId;
requestSigningCertId__is_set = true;
}
protected void setRequestSigningCertId(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("requestSigningCertId", "http://soap.sforce.com/2006/04/metadata","requestSigningCertId","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setRequestSigningCertId(__typeMapper.readString(__in, _lookupTypeInfo("requestSigningCertId", "http://soap.sforce.com/2006/04/metadata","requestSigningCertId","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldRequestSigningCertId(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("requestSigningCertId", "http://soap.sforce.com/2006/04/metadata","requestSigningCertId","http://www.w3.org/2001/XMLSchema","string",0,1,true), requestSigningCertId, requestSigningCertId__is_set);
}
/**
* element : salesforceLoginUrl of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean salesforceLoginUrl__is_set = false;
private java.lang.String salesforceLoginUrl;
public java.lang.String getSalesforceLoginUrl() {
return salesforceLoginUrl;
}
public void setSalesforceLoginUrl(java.lang.String salesforceLoginUrl) {
this.salesforceLoginUrl = salesforceLoginUrl;
salesforceLoginUrl__is_set = true;
}
protected void setSalesforceLoginUrl(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("salesforceLoginUrl", "http://soap.sforce.com/2006/04/metadata","salesforceLoginUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setSalesforceLoginUrl(__typeMapper.readString(__in, _lookupTypeInfo("salesforceLoginUrl", "http://soap.sforce.com/2006/04/metadata","salesforceLoginUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldSalesforceLoginUrl(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("salesforceLoginUrl", "http://soap.sforce.com/2006/04/metadata","salesforceLoginUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), salesforceLoginUrl, salesforceLoginUrl__is_set);
}
/**
* element : samlEntityId of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean samlEntityId__is_set = false;
private java.lang.String samlEntityId;
public java.lang.String getSamlEntityId() {
return samlEntityId;
}
public void setSamlEntityId(java.lang.String samlEntityId) {
this.samlEntityId = samlEntityId;
samlEntityId__is_set = true;
}
protected void setSamlEntityId(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("samlEntityId", "http://soap.sforce.com/2006/04/metadata","samlEntityId","http://www.w3.org/2001/XMLSchema","string",1,1,true))) {
setSamlEntityId(__typeMapper.readString(__in, _lookupTypeInfo("samlEntityId", "http://soap.sforce.com/2006/04/metadata","samlEntityId","http://www.w3.org/2001/XMLSchema","string",1,1,true), java.lang.String.class));
}
}
private void writeFieldSamlEntityId(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("samlEntityId", "http://soap.sforce.com/2006/04/metadata","samlEntityId","http://www.w3.org/2001/XMLSchema","string",1,1,true), samlEntityId, samlEntityId__is_set);
}
/**
* element : samlJitHandlerId of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean samlJitHandlerId__is_set = false;
private java.lang.String samlJitHandlerId;
public java.lang.String getSamlJitHandlerId() {
return samlJitHandlerId;
}
public void setSamlJitHandlerId(java.lang.String samlJitHandlerId) {
this.samlJitHandlerId = samlJitHandlerId;
samlJitHandlerId__is_set = true;
}
protected void setSamlJitHandlerId(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("samlJitHandlerId", "http://soap.sforce.com/2006/04/metadata","samlJitHandlerId","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setSamlJitHandlerId(__typeMapper.readString(__in, _lookupTypeInfo("samlJitHandlerId", "http://soap.sforce.com/2006/04/metadata","samlJitHandlerId","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldSamlJitHandlerId(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("samlJitHandlerId", "http://soap.sforce.com/2006/04/metadata","samlJitHandlerId","http://www.w3.org/2001/XMLSchema","string",0,1,true), samlJitHandlerId, samlJitHandlerId__is_set);
}
/**
* element : samlVersion of type {http://soap.sforce.com/2006/04/metadata}SamlType
* java type: com.sforce.soap.metadata.SamlType
*/
private boolean samlVersion__is_set = false;
private com.sforce.soap.metadata.SamlType samlVersion;
public com.sforce.soap.metadata.SamlType getSamlVersion() {
return samlVersion;
}
public void setSamlVersion(com.sforce.soap.metadata.SamlType samlVersion) {
this.samlVersion = samlVersion;
samlVersion__is_set = true;
}
protected void setSamlVersion(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("samlVersion", "http://soap.sforce.com/2006/04/metadata","samlVersion","http://soap.sforce.com/2006/04/metadata","SamlType",1,1,true))) {
setSamlVersion((com.sforce.soap.metadata.SamlType)__typeMapper.readObject(__in, _lookupTypeInfo("samlVersion", "http://soap.sforce.com/2006/04/metadata","samlVersion","http://soap.sforce.com/2006/04/metadata","SamlType",1,1,true), com.sforce.soap.metadata.SamlType.class));
}
}
private void writeFieldSamlVersion(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("samlVersion", "http://soap.sforce.com/2006/04/metadata","samlVersion","http://soap.sforce.com/2006/04/metadata","SamlType",1,1,true), samlVersion, samlVersion__is_set);
}
/**
* element : singleLogoutBinding of type {http://soap.sforce.com/2006/04/metadata}SamlSpSLOBinding
* java type: com.sforce.soap.metadata.SamlSpSLOBinding
*/
private boolean singleLogoutBinding__is_set = false;
private com.sforce.soap.metadata.SamlSpSLOBinding singleLogoutBinding;
public com.sforce.soap.metadata.SamlSpSLOBinding getSingleLogoutBinding() {
return singleLogoutBinding;
}
public void setSingleLogoutBinding(com.sforce.soap.metadata.SamlSpSLOBinding singleLogoutBinding) {
this.singleLogoutBinding = singleLogoutBinding;
singleLogoutBinding__is_set = true;
}
protected void setSingleLogoutBinding(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("singleLogoutBinding", "http://soap.sforce.com/2006/04/metadata","singleLogoutBinding","http://soap.sforce.com/2006/04/metadata","SamlSpSLOBinding",0,1,true))) {
setSingleLogoutBinding((com.sforce.soap.metadata.SamlSpSLOBinding)__typeMapper.readObject(__in, _lookupTypeInfo("singleLogoutBinding", "http://soap.sforce.com/2006/04/metadata","singleLogoutBinding","http://soap.sforce.com/2006/04/metadata","SamlSpSLOBinding",0,1,true), com.sforce.soap.metadata.SamlSpSLOBinding.class));
}
}
private void writeFieldSingleLogoutBinding(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("singleLogoutBinding", "http://soap.sforce.com/2006/04/metadata","singleLogoutBinding","http://soap.sforce.com/2006/04/metadata","SamlSpSLOBinding",0,1,true), singleLogoutBinding, singleLogoutBinding__is_set);
}
/**
* element : singleLogoutUrl of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean singleLogoutUrl__is_set = false;
private java.lang.String singleLogoutUrl;
public java.lang.String getSingleLogoutUrl() {
return singleLogoutUrl;
}
public void setSingleLogoutUrl(java.lang.String singleLogoutUrl) {
this.singleLogoutUrl = singleLogoutUrl;
singleLogoutUrl__is_set = true;
}
protected void setSingleLogoutUrl(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("singleLogoutUrl", "http://soap.sforce.com/2006/04/metadata","singleLogoutUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true))) {
setSingleLogoutUrl(__typeMapper.readString(__in, _lookupTypeInfo("singleLogoutUrl", "http://soap.sforce.com/2006/04/metadata","singleLogoutUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), java.lang.String.class));
}
}
private void writeFieldSingleLogoutUrl(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("singleLogoutUrl", "http://soap.sforce.com/2006/04/metadata","singleLogoutUrl","http://www.w3.org/2001/XMLSchema","string",0,1,true), singleLogoutUrl, singleLogoutUrl__is_set);
}
/**
* element : useConfigRequestMethod of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean useConfigRequestMethod__is_set = false;
private boolean useConfigRequestMethod;
public boolean getUseConfigRequestMethod() {
return useConfigRequestMethod;
}
public boolean isUseConfigRequestMethod() {
return useConfigRequestMethod;
}
public void setUseConfigRequestMethod(boolean useConfigRequestMethod) {
this.useConfigRequestMethod = useConfigRequestMethod;
useConfigRequestMethod__is_set = true;
}
protected void setUseConfigRequestMethod(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("useConfigRequestMethod", "http://soap.sforce.com/2006/04/metadata","useConfigRequestMethod","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setUseConfigRequestMethod(__typeMapper.readBoolean(__in, _lookupTypeInfo("useConfigRequestMethod", "http://soap.sforce.com/2006/04/metadata","useConfigRequestMethod","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldUseConfigRequestMethod(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("useConfigRequestMethod", "http://soap.sforce.com/2006/04/metadata","useConfigRequestMethod","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), useConfigRequestMethod, useConfigRequestMethod__is_set);
}
/**
* element : useSameDigestAlgoForSigning of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean useSameDigestAlgoForSigning__is_set = false;
private boolean useSameDigestAlgoForSigning;
public boolean getUseSameDigestAlgoForSigning() {
return useSameDigestAlgoForSigning;
}
public boolean isUseSameDigestAlgoForSigning() {
return useSameDigestAlgoForSigning;
}
public void setUseSameDigestAlgoForSigning(boolean useSameDigestAlgoForSigning) {
this.useSameDigestAlgoForSigning = useSameDigestAlgoForSigning;
useSameDigestAlgoForSigning__is_set = true;
}
protected void setUseSameDigestAlgoForSigning(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("useSameDigestAlgoForSigning", "http://soap.sforce.com/2006/04/metadata","useSameDigestAlgoForSigning","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setUseSameDigestAlgoForSigning(__typeMapper.readBoolean(__in, _lookupTypeInfo("useSameDigestAlgoForSigning", "http://soap.sforce.com/2006/04/metadata","useSameDigestAlgoForSigning","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldUseSameDigestAlgoForSigning(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("useSameDigestAlgoForSigning", "http://soap.sforce.com/2006/04/metadata","useSameDigestAlgoForSigning","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), useSameDigestAlgoForSigning, useSameDigestAlgoForSigning__is_set);
}
/**
* element : userProvisioning of type {http://www.w3.org/2001/XMLSchema}boolean
* java type: boolean
*/
private boolean userProvisioning__is_set = false;
private boolean userProvisioning;
public boolean getUserProvisioning() {
return userProvisioning;
}
public boolean isUserProvisioning() {
return userProvisioning;
}
public void setUserProvisioning(boolean userProvisioning) {
this.userProvisioning = userProvisioning;
userProvisioning__is_set = true;
}
protected void setUserProvisioning(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.isElement(__in, _lookupTypeInfo("userProvisioning", "http://soap.sforce.com/2006/04/metadata","userProvisioning","http://www.w3.org/2001/XMLSchema","boolean",0,1,true))) {
setUserProvisioning(__typeMapper.readBoolean(__in, _lookupTypeInfo("userProvisioning", "http://soap.sforce.com/2006/04/metadata","userProvisioning","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), boolean.class));
}
}
private void writeFieldUserProvisioning(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("userProvisioning", "http://soap.sforce.com/2006/04/metadata","userProvisioning","http://www.w3.org/2001/XMLSchema","boolean",0,1,true), userProvisioning, userProvisioning__is_set);
}
/**
* element : validationCert of type {http://www.w3.org/2001/XMLSchema}string
* java type: java.lang.String
*/
private boolean validationCert__is_set = false;
private java.lang.String validationCert;
public java.lang.String getValidationCert() {
return validationCert;
}
public void setValidationCert(java.lang.String validationCert) {
this.validationCert = validationCert;
validationCert__is_set = true;
}
protected void setValidationCert(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__in.peekTag();
if (__typeMapper.verifyElement(__in, _lookupTypeInfo("validationCert", "http://soap.sforce.com/2006/04/metadata","validationCert","http://www.w3.org/2001/XMLSchema","string",1,1,true))) {
setValidationCert(__typeMapper.readString(__in, _lookupTypeInfo("validationCert", "http://soap.sforce.com/2006/04/metadata","validationCert","http://www.w3.org/2001/XMLSchema","string",1,1,true), java.lang.String.class));
}
}
private void writeFieldValidationCert(com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
__typeMapper.writeObject(__out, _lookupTypeInfo("validationCert", "http://soap.sforce.com/2006/04/metadata","validationCert","http://www.w3.org/2001/XMLSchema","string",1,1,true), validationCert, validationCert__is_set);
}
/**
*/
@Override
public void write(javax.xml.namespace.QName __element,
com.sforce.ws.parser.XmlOutputStream __out, com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
__out.writeStartTag(__element.getNamespaceURI(), __element.getLocalPart());
__typeMapper.writeXsiType(__out, "http://soap.sforce.com/2006/04/metadata", "SamlSsoConfig");
writeFields(__out, __typeMapper);
__out.writeEndTag(__element.getNamespaceURI(), __element.getLocalPart());
}
protected void writeFields(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper)
throws java.io.IOException {
super.writeFields(__out, __typeMapper);
writeFields1(__out, __typeMapper);
}
@Override
public void load(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
__typeMapper.consumeStartTag(__in);
loadFields(__in, __typeMapper);
__typeMapper.consumeEndTag(__in);
}
protected void loadFields(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
super.loadFields(__in, __typeMapper);
loadFields1(__in, __typeMapper);
}
@Override
public String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("[SamlSsoConfig ");
sb.append(super.toString());
toString1(sb);
sb.append("]\n");
return sb.toString();
}
private void toStringHelper(StringBuilder sb, String name, Object value) {
sb.append(' ').append(name).append("='").append(com.sforce.ws.util.Verbose.toString(value)).append("'\n");
}
private void writeFields1(com.sforce.ws.parser.XmlOutputStream __out,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException {
writeFieldAttributeName(__out, __typeMapper);
writeFieldAttributeNameIdFormat(__out, __typeMapper);
writeFieldDecryptionCertificate(__out, __typeMapper);
writeFieldErrorUrl(__out, __typeMapper);
writeFieldExecutionUserId(__out, __typeMapper);
writeFieldIdentityLocation(__out, __typeMapper);
writeFieldIdentityMapping(__out, __typeMapper);
writeFieldIssuer(__out, __typeMapper);
writeFieldLoginUrl(__out, __typeMapper);
writeFieldLogoutUrl(__out, __typeMapper);
writeFieldName(__out, __typeMapper);
writeFieldOauthTokenEndpoint(__out, __typeMapper);
writeFieldRedirectBinding(__out, __typeMapper);
writeFieldRequestSignatureMethod(__out, __typeMapper);
writeFieldRequestSigningCertId(__out, __typeMapper);
writeFieldSalesforceLoginUrl(__out, __typeMapper);
writeFieldSamlEntityId(__out, __typeMapper);
writeFieldSamlJitHandlerId(__out, __typeMapper);
writeFieldSamlVersion(__out, __typeMapper);
writeFieldSingleLogoutBinding(__out, __typeMapper);
writeFieldSingleLogoutUrl(__out, __typeMapper);
writeFieldUseConfigRequestMethod(__out, __typeMapper);
writeFieldUseSameDigestAlgoForSigning(__out, __typeMapper);
writeFieldUserProvisioning(__out, __typeMapper);
writeFieldValidationCert(__out, __typeMapper);
}
private void loadFields1(com.sforce.ws.parser.XmlInputStream __in,
com.sforce.ws.bind.TypeMapper __typeMapper) throws java.io.IOException, com.sforce.ws.ConnectionException {
setAttributeName(__in, __typeMapper);
setAttributeNameIdFormat(__in, __typeMapper);
setDecryptionCertificate(__in, __typeMapper);
setErrorUrl(__in, __typeMapper);
setExecutionUserId(__in, __typeMapper);
setIdentityLocation(__in, __typeMapper);
setIdentityMapping(__in, __typeMapper);
setIssuer(__in, __typeMapper);
setLoginUrl(__in, __typeMapper);
setLogoutUrl(__in, __typeMapper);
setName(__in, __typeMapper);
setOauthTokenEndpoint(__in, __typeMapper);
setRedirectBinding(__in, __typeMapper);
setRequestSignatureMethod(__in, __typeMapper);
setRequestSigningCertId(__in, __typeMapper);
setSalesforceLoginUrl(__in, __typeMapper);
setSamlEntityId(__in, __typeMapper);
setSamlJitHandlerId(__in, __typeMapper);
setSamlVersion(__in, __typeMapper);
setSingleLogoutBinding(__in, __typeMapper);
setSingleLogoutUrl(__in, __typeMapper);
setUseConfigRequestMethod(__in, __typeMapper);
setUseSameDigestAlgoForSigning(__in, __typeMapper);
setUserProvisioning(__in, __typeMapper);
setValidationCert(__in, __typeMapper);
}
private void toString1(StringBuilder sb) {
toStringHelper(sb, "attributeName", attributeName);
toStringHelper(sb, "attributeNameIdFormat", attributeNameIdFormat);
toStringHelper(sb, "decryptionCertificate", decryptionCertificate);
toStringHelper(sb, "errorUrl", errorUrl);
toStringHelper(sb, "executionUserId", executionUserId);
toStringHelper(sb, "identityLocation", identityLocation);
toStringHelper(sb, "identityMapping", identityMapping);
toStringHelper(sb, "issuer", issuer);
toStringHelper(sb, "loginUrl", loginUrl);
toStringHelper(sb, "logoutUrl", logoutUrl);
toStringHelper(sb, "name", name);
toStringHelper(sb, "oauthTokenEndpoint", oauthTokenEndpoint);
toStringHelper(sb, "redirectBinding", redirectBinding);
toStringHelper(sb, "requestSignatureMethod", requestSignatureMethod);
toStringHelper(sb, "requestSigningCertId", requestSigningCertId);
toStringHelper(sb, "salesforceLoginUrl", salesforceLoginUrl);
toStringHelper(sb, "samlEntityId", samlEntityId);
toStringHelper(sb, "samlJitHandlerId", samlJitHandlerId);
toStringHelper(sb, "samlVersion", samlVersion);
toStringHelper(sb, "singleLogoutBinding", singleLogoutBinding);
toStringHelper(sb, "singleLogoutUrl", singleLogoutUrl);
toStringHelper(sb, "useConfigRequestMethod", useConfigRequestMethod);
toStringHelper(sb, "useSameDigestAlgoForSigning", useSameDigestAlgoForSigning);
toStringHelper(sb, "userProvisioning", userProvisioning);
toStringHelper(sb, "validationCert", validationCert);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy