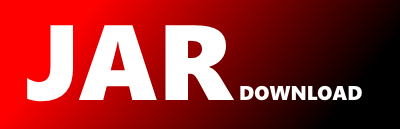
com.foreach.common.web.util.Log4jWebUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common-web Show documentation
Show all versions of common-web Show documentation
A number of general purpose libraries for Java. Dependencies are kept minimal but Spring framework
is considered a base requirement for most libraries.
/*
* Copyright 2014 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.foreach.common.web.util;
import com.foreach.common.spring.logging.Log4JUtils;
import org.apache.log4j.Level;
import org.apache.log4j.LogManager;
import org.apache.log4j.Logger;
import javax.servlet.http.HttpServletRequest;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
/**
* This class contains methods to display and change the log levels of registered Log4j loggers dynamically with an http client.
*
* To configure log levels dynamically, do the following:
*
* - In your controller, define a get method that will serve the update form to the client.
* Use the method {@link #getLoggersHtmlContent} to obtain the html content to be served.
* - Define a post method to process the client response. From this routine you need only call
* {@link #setLoggerLevels} to update the log levels to the user provided values.
*
*
* @version 1.0
*/
public class Log4jWebUtils
{
protected Log4jWebUtils() {
// protected constructor, so that this class can be extended.
}
/**
* This method generates the html content for a web page containing a form to change the log level of registered log4j loggers.
* The html body can be directly sent to the browser by defining spring annotation @ResponseBody on a controller method.
*
* Eg. HttpServletResponse.getWriter().write( "html content" );
*
* Use this method to display the registered loggers and their current log level.
*
* @param applicationName a label to be used in the html body, so the user knows which application's loggers are shown.
* @param formAction the url that maps to the controller method that calls
* {@link #setLoggerLevels}
* @param includePageTags true to include html start/end tags (eg. ''), false to generate only html form content
* @return a string with html content.
*/
public static String getLoggersHtmlContent( String applicationName, String formAction, boolean includePageTags ) {
List loggers = Log4JUtils.getClassLoggers();
List levels =
Arrays.asList( Level.OFF, Level.FATAL, Level.ERROR, Level.WARN, Level.INFO, Level.DEBUG );
StringBuffer output = new StringBuffer();
if ( includePageTags ) {
output.append( "Manage Loggers - " ).append( applicationName ).append(
" " );
}
output.append( "Manage Loggers - " ).append( applicationName ).append( "
" );
output.append( "" );
if ( includePageTags ) {
output.append( "" );
}
return output.toString();
}
/**
* This method will update the levels of registered Loggers to the new levels
* retrieved from the given HttpServletRequest object.
*
* You should call this method from within the controller method that maps to
* the formAction parameter you provided to {@link #getLoggersHtmlContent}.
*
* @param request the http request object containing the updated log levels.
*/
public static void setLoggerLevels( HttpServletRequest request ) {
Map params = request.getParameterMap();
for ( Map.Entry entry : params.entrySet() ) {
String name = entry.getKey();
if ( LogManager.exists( name ) != null ) {
String[] levels = entry.getValue();
Level level = Level.toLevel( levels[0] );
Logger.getLogger( name ).setLevel( level );
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy