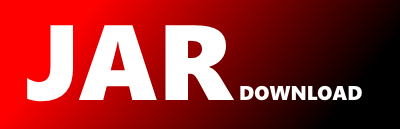
com.formance.formance_sdk.SDK Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of formance-sdk Show documentation
Show all versions of formance-sdk Show documentation
SDK enabling Java developers to easily integrate with the Formance API.
/*
* Code generated by Speakeasy (https://speakeasy.com). DO NOT EDIT.
*/
package com.formance.formance_sdk;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.core.type.TypeReference;
import com.formance.formance_sdk.models.errors.SDKError;
import com.formance.formance_sdk.models.operations.GetVersionsRequestBuilder;
import com.formance.formance_sdk.models.operations.GetVersionsResponse;
import com.formance.formance_sdk.models.operations.SDKMethodInterfaces.*;
import com.formance.formance_sdk.utils.HTTPClient;
import com.formance.formance_sdk.utils.HTTPRequest;
import com.formance.formance_sdk.utils.Hook.AfterErrorContextImpl;
import com.formance.formance_sdk.utils.Hook.AfterSuccessContextImpl;
import com.formance.formance_sdk.utils.Hook.BeforeRequestContextImpl;
import com.formance.formance_sdk.utils.RetryConfig;
import com.formance.formance_sdk.utils.SpeakeasyHTTPClient;
import com.formance.formance_sdk.utils.Utils;
import java.io.InputStream;
import java.lang.Exception;
import java.lang.String;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.util.List;
import java.util.Map;
import java.util.Optional;
/**
* Formance Stack API: Open, modular foundation for unique payments flows
*
* # Introduction
* This API is documented in **OpenAPI format**.
*
* # Authentication
* Formance Stack offers one forms of authentication:
* - OAuth2
* OAuth2 - an open protocol to allow secure authorization in a simple
* and standard method from web, mobile and desktop applications.
* <SecurityDefinitions />
*
*/
public class SDK implements
MethodCallGetVersions {
/**
* SERVERS contains the list of server urls available to the SDK.
*/
public static final String[] SERVERS = {
/**
* local server
*/
"http://localhost",
/**
* A per-organization and per-environment API
*/
"https://{organization}.{environment}.formance.cloud",
};
private final Auth auth;
private final Ledger ledger;
private final Orchestration orchestration;
private final Payments payments;
private final Reconciliation reconciliation;
private final Search search;
private final Wallets wallets;
private final Webhooks webhooks;
public Auth auth() {
return auth;
}
public Ledger ledger() {
return ledger;
}
public Orchestration orchestration() {
return orchestration;
}
public Payments payments() {
return payments;
}
public Reconciliation reconciliation() {
return reconciliation;
}
public Search search() {
return search;
}
public Wallets wallets() {
return wallets;
}
public Webhooks webhooks() {
return webhooks;
}
private final SDKConfiguration sdkConfiguration;
/**
* The Builder class allows the configuration of a new instance of the SDK.
*/
public static class Builder {
private final SDKConfiguration sdkConfiguration = new SDKConfiguration();
private Builder() {
}
/**
* Allows the default HTTP client to be overridden with a custom implementation.
*
* @param client The HTTP client to use for all requests.
* @return The builder instance.
*/
public Builder client(HTTPClient client) {
this.sdkConfiguration.defaultClient = client;
return this;
}
/**
* Configures the SDK to use the provided security details.
*
* @param security The security details to use for all requests.
* @return The builder instance.
*/
public Builder security(com.formance.formance_sdk.models.shared.Security security) {
this.sdkConfiguration.securitySource = SecuritySource.of(security);
return this;
}
/**
* Configures the SDK to use a custom security source.
* @param securitySource The security source to use for all requests.
* @return The builder instance.
*/
public Builder securitySource(SecuritySource securitySource) {
this.sdkConfiguration.securitySource = securitySource;
return this;
}
/**
* Overrides the default server URL.
*
* @param serverUrl The server URL to use for all requests.
* @return The builder instance.
*/
public Builder serverURL(String serverUrl) {
this.sdkConfiguration.serverUrl = serverUrl;
return this;
}
/**
* Overrides the default server URL with a templated URL populated with the provided parameters.
*
* @param serverUrl The server URL to use for all requests.
* @param params The parameters to use when templating the URL.
* @return The builder instance.
*/
public Builder serverURL(String serverUrl, Map params) {
this.sdkConfiguration.serverUrl = Utils.templateUrl(serverUrl, params);
return this;
}
/**
* Overrides the default server by index.
*
* @param serverIdx The server to use for all requests.
* @return The builder instance.
*/
public Builder serverIndex(int serverIdx) {
this.sdkConfiguration.serverIdx = serverIdx;
this.sdkConfiguration.serverUrl = SERVERS[serverIdx];
return this;
}
/**
* Overrides the default configuration for retries
*
* @param retryConfig The retry configuration to use for all requests.
* @return The builder instance.
*/
public Builder retryConfig(RetryConfig retryConfig) {
this.sdkConfiguration.retryConfig = Optional.of(retryConfig);
return this;
}
/**
* ServerEnvironment - The environment name. Defaults to the production environment.
*/
public enum ServerEnvironment {
SANDBOX("sandbox"),
EU_WEST1("eu-west-1"),
US_EAST1("us-east-1");
@JsonValue
private final String value;
private ServerEnvironment(String value) {
this.value = value;
}
public String value() {
return value;
}
}
/**
* Sets the environment variable for url substitution.
*
* @param environment The value to set.
* @return The builder instance.
*/
public Builder environment(ServerEnvironment environment) {
for (Map server : this.sdkConfiguration.serverDefaults) {
if (!server.containsKey("environment")) {
continue;
}
server.put("environment", environment.toString());
}
return this;
}
/**
* Sets the organization variable for url substitution.
*
* @param organization The value to set.
* @return The builder instance.
*/
public Builder organization(String organization) {
for (Map server : this.sdkConfiguration.serverDefaults) {
if (!server.containsKey("organization")) {
continue;
}
server.put("organization", organization.toString());
}
return this;
}
// Visible for testing, will be accessed via reflection
void _hooks(com.formance.formance_sdk.utils.Hooks hooks) {
sdkConfiguration.setHooks(hooks);
}
/**
* Builds a new instance of the SDK.
* @return The SDK instance.
*/
public SDK build() {
if (sdkConfiguration.defaultClient == null) {
sdkConfiguration.defaultClient = new SpeakeasyHTTPClient();
}
if (sdkConfiguration.securitySource == null) {
sdkConfiguration.securitySource = SecuritySource.of(null);
}
if (sdkConfiguration.serverUrl == null || sdkConfiguration.serverUrl.isBlank()) {
sdkConfiguration.serverUrl = SERVERS[0];
sdkConfiguration.serverIdx = 0;
}
if (sdkConfiguration.serverUrl.endsWith("/")) {
sdkConfiguration.serverUrl = sdkConfiguration.serverUrl.substring(0, sdkConfiguration.serverUrl.length() - 1);
}
return new SDK(sdkConfiguration);
}
}
/**
* Get a new instance of the SDK builder to configure a new instance of the SDK.
* @return The SDK builder instance.
*/
public static Builder builder() {
return new Builder();
}
private SDK(SDKConfiguration sdkConfiguration) {
this.sdkConfiguration = sdkConfiguration;
this.auth = new Auth(sdkConfiguration);
this.ledger = new Ledger(sdkConfiguration);
this.orchestration = new Orchestration(sdkConfiguration);
this.payments = new Payments(sdkConfiguration);
this.reconciliation = new Reconciliation(sdkConfiguration);
this.search = new Search(sdkConfiguration);
this.wallets = new Wallets(sdkConfiguration);
this.webhooks = new Webhooks(sdkConfiguration);
this.sdkConfiguration.initialize();
}
/**
* Show stack version information
* @return The call builder
*/
public GetVersionsRequestBuilder getVersions() {
return new GetVersionsRequestBuilder(this);
}
/**
* Show stack version information
* @return The response from the API call
* @throws Exception if the API call fails
*/
public GetVersionsResponse getVersionsDirect() throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/versions");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"getVersions",
Optional.of(List.of("auth:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getVersions",
Optional.of(List.of("auth:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"getVersions",
Optional.of(List.of("auth:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getVersions",
Optional.of(List.of("auth:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
GetVersionsResponse.Builder _resBuilder =
GetVersionsResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
GetVersionsResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.GetVersionsResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withGetVersionsResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy