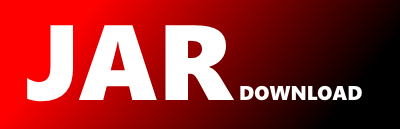
com.formance.formance_sdk.SDKV2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of formance-sdk Show documentation
Show all versions of formance-sdk Show documentation
SDK enabling Java developers to easily integrate with the Formance API.
/*
* Code generated by Speakeasy (https://speakeasy.com). DO NOT EDIT.
*/
package com.formance.formance_sdk;
import com.fasterxml.jackson.core.type.TypeReference;
import com.formance.formance_sdk.models.errors.SDKError;
import com.formance.formance_sdk.models.errors.V2Error;
import com.formance.formance_sdk.models.operations.SDKMethodInterfaces.*;
import com.formance.formance_sdk.models.operations.TestTriggerRequest;
import com.formance.formance_sdk.models.operations.TestTriggerRequestBuilder;
import com.formance.formance_sdk.models.operations.TestTriggerResponse;
import com.formance.formance_sdk.models.operations.V2CancelEventRequest;
import com.formance.formance_sdk.models.operations.V2CancelEventRequestBuilder;
import com.formance.formance_sdk.models.operations.V2CancelEventResponse;
import com.formance.formance_sdk.models.operations.V2CreateTriggerRequestBuilder;
import com.formance.formance_sdk.models.operations.V2CreateTriggerResponse;
import com.formance.formance_sdk.models.operations.V2CreateWorkflowRequestBuilder;
import com.formance.formance_sdk.models.operations.V2CreateWorkflowResponse;
import com.formance.formance_sdk.models.operations.V2DeleteTriggerRequest;
import com.formance.formance_sdk.models.operations.V2DeleteTriggerRequestBuilder;
import com.formance.formance_sdk.models.operations.V2DeleteTriggerResponse;
import com.formance.formance_sdk.models.operations.V2DeleteWorkflowRequest;
import com.formance.formance_sdk.models.operations.V2DeleteWorkflowRequestBuilder;
import com.formance.formance_sdk.models.operations.V2DeleteWorkflowResponse;
import com.formance.formance_sdk.models.operations.V2GetInstanceHistoryRequest;
import com.formance.formance_sdk.models.operations.V2GetInstanceHistoryRequestBuilder;
import com.formance.formance_sdk.models.operations.V2GetInstanceHistoryResponse;
import com.formance.formance_sdk.models.operations.V2GetInstanceRequest;
import com.formance.formance_sdk.models.operations.V2GetInstanceRequestBuilder;
import com.formance.formance_sdk.models.operations.V2GetInstanceResponse;
import com.formance.formance_sdk.models.operations.V2GetInstanceStageHistoryRequest;
import com.formance.formance_sdk.models.operations.V2GetInstanceStageHistoryRequestBuilder;
import com.formance.formance_sdk.models.operations.V2GetInstanceStageHistoryResponse;
import com.formance.formance_sdk.models.operations.V2GetServerInfoRequestBuilder;
import com.formance.formance_sdk.models.operations.V2GetServerInfoResponse;
import com.formance.formance_sdk.models.operations.V2GetWorkflowRequest;
import com.formance.formance_sdk.models.operations.V2GetWorkflowRequestBuilder;
import com.formance.formance_sdk.models.operations.V2GetWorkflowResponse;
import com.formance.formance_sdk.models.operations.V2ListInstancesRequest;
import com.formance.formance_sdk.models.operations.V2ListInstancesRequestBuilder;
import com.formance.formance_sdk.models.operations.V2ListInstancesResponse;
import com.formance.formance_sdk.models.operations.V2ListTriggersOccurrencesRequest;
import com.formance.formance_sdk.models.operations.V2ListTriggersOccurrencesRequestBuilder;
import com.formance.formance_sdk.models.operations.V2ListTriggersOccurrencesResponse;
import com.formance.formance_sdk.models.operations.V2ListTriggersRequest;
import com.formance.formance_sdk.models.operations.V2ListTriggersRequestBuilder;
import com.formance.formance_sdk.models.operations.V2ListTriggersResponse;
import com.formance.formance_sdk.models.operations.V2ListWorkflowsRequest;
import com.formance.formance_sdk.models.operations.V2ListWorkflowsRequestBuilder;
import com.formance.formance_sdk.models.operations.V2ListWorkflowsResponse;
import com.formance.formance_sdk.models.operations.V2ReadTriggerRequest;
import com.formance.formance_sdk.models.operations.V2ReadTriggerRequestBuilder;
import com.formance.formance_sdk.models.operations.V2ReadTriggerResponse;
import com.formance.formance_sdk.models.operations.V2RunWorkflowRequest;
import com.formance.formance_sdk.models.operations.V2RunWorkflowRequestBuilder;
import com.formance.formance_sdk.models.operations.V2RunWorkflowResponse;
import com.formance.formance_sdk.models.operations.V2SendEventRequest;
import com.formance.formance_sdk.models.operations.V2SendEventRequestBuilder;
import com.formance.formance_sdk.models.operations.V2SendEventResponse;
import com.formance.formance_sdk.models.shared.V2CreateWorkflowRequest;
import com.formance.formance_sdk.models.shared.V2GetWorkflowInstanceHistoryResponse;
import com.formance.formance_sdk.models.shared.V2GetWorkflowInstanceHistoryStageResponse;
import com.formance.formance_sdk.models.shared.V2GetWorkflowInstanceResponse;
import com.formance.formance_sdk.models.shared.V2ListRunsResponse;
import com.formance.formance_sdk.models.shared.V2ServerInfo;
import com.formance.formance_sdk.models.shared.V2TestTriggerResponse;
import com.formance.formance_sdk.models.shared.V2TriggerData;
import com.formance.formance_sdk.utils.HTTPClient;
import com.formance.formance_sdk.utils.HTTPRequest;
import com.formance.formance_sdk.utils.Hook.AfterErrorContextImpl;
import com.formance.formance_sdk.utils.Hook.AfterSuccessContextImpl;
import com.formance.formance_sdk.utils.Hook.BeforeRequestContextImpl;
import com.formance.formance_sdk.utils.SerializedBody;
import com.formance.formance_sdk.utils.Utils.JsonShape;
import com.formance.formance_sdk.utils.Utils;
import java.io.InputStream;
import java.lang.Exception;
import java.lang.Object;
import java.lang.String;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.util.List;
import java.util.Optional;
public class SDKV2 implements
MethodCallV2CancelEvent,
MethodCallV2CreateTrigger,
MethodCallV2CreateWorkflow,
MethodCallV2DeleteTrigger,
MethodCallV2DeleteWorkflow,
MethodCallV2GetInstance,
MethodCallV2GetInstanceHistory,
MethodCallV2GetInstanceStageHistory,
MethodCallV2GetServerInfo,
MethodCallV2GetWorkflow,
MethodCallV2ListInstances,
MethodCallV2ListTriggers,
MethodCallV2ListTriggersOccurrences,
MethodCallV2ListWorkflows,
MethodCallV2ReadTrigger,
MethodCallV2RunWorkflow,
MethodCallV2SendEvent,
MethodCallTestTrigger {
private final SDKConfiguration sdkConfiguration;
SDKV2(SDKConfiguration sdkConfiguration) {
this.sdkConfiguration = sdkConfiguration;
}
/**
* Cancel a running workflow
* Cancel a running workflow
* @return The call builder
*/
public V2CancelEventRequestBuilder cancelEvent() {
return new V2CancelEventRequestBuilder(this);
}
/**
* Cancel a running workflow
* Cancel a running workflow
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2CancelEventResponse cancelEvent(
V2CancelEventRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
V2CancelEventRequest.class,
_baseUrl,
"/api/orchestration/v2/instances/{instanceID}/abort",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "PUT");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2CancelEvent",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2CancelEvent",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2CancelEvent",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2CancelEvent",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2CancelEventResponse.Builder _resBuilder =
V2CancelEventResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2CancelEventResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Create trigger
* Create trigger
* @return The call builder
*/
public V2CreateTriggerRequestBuilder createTrigger() {
return new V2CreateTriggerRequestBuilder(this);
}
/**
* Create trigger
* Create trigger
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2CreateTriggerResponse createTriggerDirect() throws Exception {
return createTrigger(Optional.empty());
}
/**
* Create trigger
* Create trigger
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2CreateTriggerResponse createTrigger(
Optional extends V2TriggerData> request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/orchestration/v2/triggers");
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference>() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"request",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2CreateTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2CreateTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2CreateTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2CreateTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2CreateTriggerResponse.Builder _resBuilder =
V2CreateTriggerResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2CreateTriggerResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "201")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.V2CreateTriggerResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2CreateTriggerResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Create workflow
* Create a workflow
* @return The call builder
*/
public V2CreateWorkflowRequestBuilder createWorkflow() {
return new V2CreateWorkflowRequestBuilder(this);
}
/**
* Create workflow
* Create a workflow
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2CreateWorkflowResponse createWorkflowDirect() throws Exception {
return createWorkflow(Optional.empty());
}
/**
* Create workflow
* Create a workflow
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2CreateWorkflowResponse createWorkflow(
Optional extends V2CreateWorkflowRequest> request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/orchestration/v2/workflows");
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference>() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"request",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2CreateWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2CreateWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2CreateWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2CreateWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2CreateWorkflowResponse.Builder _resBuilder =
V2CreateWorkflowResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2CreateWorkflowResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "201")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.V2CreateWorkflowResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2CreateWorkflowResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Delete trigger
* Read trigger
* @return The call builder
*/
public V2DeleteTriggerRequestBuilder deleteTrigger() {
return new V2DeleteTriggerRequestBuilder(this);
}
/**
* Delete trigger
* Read trigger
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2DeleteTriggerResponse deleteTrigger(
V2DeleteTriggerRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
V2DeleteTriggerRequest.class,
_baseUrl,
"/api/orchestration/v2/triggers/{triggerID}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "DELETE");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2DeleteTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2DeleteTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2DeleteTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2DeleteTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2DeleteTriggerResponse.Builder _resBuilder =
V2DeleteTriggerResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2DeleteTriggerResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Delete a flow by id
* Delete a flow by id
* @return The call builder
*/
public V2DeleteWorkflowRequestBuilder deleteWorkflow() {
return new V2DeleteWorkflowRequestBuilder(this);
}
/**
* Delete a flow by id
* Delete a flow by id
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2DeleteWorkflowResponse deleteWorkflow(
V2DeleteWorkflowRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
V2DeleteWorkflowRequest.class,
_baseUrl,
"/api/orchestration/v2/workflows/{flowId}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "DELETE");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2DeleteWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2DeleteWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2DeleteWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2DeleteWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2DeleteWorkflowResponse.Builder _resBuilder =
V2DeleteWorkflowResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2DeleteWorkflowResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get a workflow instance by id
* Get a workflow instance by id
* @return The call builder
*/
public V2GetInstanceRequestBuilder getInstance() {
return new V2GetInstanceRequestBuilder(this);
}
/**
* Get a workflow instance by id
* Get a workflow instance by id
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2GetInstanceResponse getInstance(
V2GetInstanceRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
V2GetInstanceRequest.class,
_baseUrl,
"/api/orchestration/v2/instances/{instanceID}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2GetInstance",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2GetInstance",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2GetInstance",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2GetInstance",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2GetInstanceResponse.Builder _resBuilder =
V2GetInstanceResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2GetInstanceResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2GetWorkflowInstanceResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2GetWorkflowInstanceResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get a workflow instance history by id
* Get a workflow instance history by id
* @return The call builder
*/
public V2GetInstanceHistoryRequestBuilder getInstanceHistory() {
return new V2GetInstanceHistoryRequestBuilder(this);
}
/**
* Get a workflow instance history by id
* Get a workflow instance history by id
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2GetInstanceHistoryResponse getInstanceHistory(
V2GetInstanceHistoryRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
V2GetInstanceHistoryRequest.class,
_baseUrl,
"/api/orchestration/v2/instances/{instanceID}/history",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2GetInstanceHistory",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2GetInstanceHistory",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2GetInstanceHistory",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2GetInstanceHistory",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2GetInstanceHistoryResponse.Builder _resBuilder =
V2GetInstanceHistoryResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2GetInstanceHistoryResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2GetWorkflowInstanceHistoryResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2GetWorkflowInstanceHistoryResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get a workflow instance stage history
* Get a workflow instance stage history
* @return The call builder
*/
public V2GetInstanceStageHistoryRequestBuilder getInstanceStageHistory() {
return new V2GetInstanceStageHistoryRequestBuilder(this);
}
/**
* Get a workflow instance stage history
* Get a workflow instance stage history
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2GetInstanceStageHistoryResponse getInstanceStageHistory(
V2GetInstanceStageHistoryRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
V2GetInstanceStageHistoryRequest.class,
_baseUrl,
"/api/orchestration/v2/instances/{instanceID}/stages/{number}/history",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2GetInstanceStageHistory",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2GetInstanceStageHistory",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2GetInstanceStageHistory",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2GetInstanceStageHistory",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2GetInstanceStageHistoryResponse.Builder _resBuilder =
V2GetInstanceStageHistoryResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2GetInstanceStageHistoryResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2GetWorkflowInstanceHistoryStageResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2GetWorkflowInstanceHistoryStageResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get server info
* @return The call builder
*/
public V2GetServerInfoRequestBuilder getServerInfo() {
return new V2GetServerInfoRequestBuilder(this);
}
/**
* Get server info
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2GetServerInfoResponse getServerInfoDirect() throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/orchestration/v2/_info");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2GetServerInfo",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2GetServerInfo",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2GetServerInfo",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2GetServerInfo",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2GetServerInfoResponse.Builder _resBuilder =
V2GetServerInfoResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2GetServerInfoResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2ServerInfo _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2ServerInfo(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get a flow by id
* Get a flow by id
* @return The call builder
*/
public V2GetWorkflowRequestBuilder getWorkflow() {
return new V2GetWorkflowRequestBuilder(this);
}
/**
* Get a flow by id
* Get a flow by id
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2GetWorkflowResponse getWorkflow(
V2GetWorkflowRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
V2GetWorkflowRequest.class,
_baseUrl,
"/api/orchestration/v2/workflows/{flowId}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2GetWorkflow",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2GetWorkflow",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2GetWorkflow",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2GetWorkflow",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2GetWorkflowResponse.Builder _resBuilder =
V2GetWorkflowResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2GetWorkflowResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.V2GetWorkflowResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2GetWorkflowResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* List instances of a workflow
* List instances of a workflow
* @return The call builder
*/
public V2ListInstancesRequestBuilder listInstances() {
return new V2ListInstancesRequestBuilder(this);
}
/**
* List instances of a workflow
* List instances of a workflow
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2ListInstancesResponse listInstances(
V2ListInstancesRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/orchestration/v2/instances");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addQueryParams(Utils.getQueryParams(
V2ListInstancesRequest.class,
request,
null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2ListInstances",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2ListInstances",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2ListInstances",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2ListInstances",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2ListInstancesResponse.Builder _resBuilder =
V2ListInstancesResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2ListInstancesResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2ListRunsResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2ListRunsResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* List triggers
* List triggers
* @return The call builder
*/
public V2ListTriggersRequestBuilder listTriggers() {
return new V2ListTriggersRequestBuilder(this);
}
/**
* List triggers
* List triggers
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2ListTriggersResponse listTriggers(
V2ListTriggersRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/orchestration/v2/triggers");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addQueryParams(Utils.getQueryParams(
V2ListTriggersRequest.class,
request,
null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2ListTriggers",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2ListTriggers",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2ListTriggers",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2ListTriggers",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2ListTriggersResponse.Builder _resBuilder =
V2ListTriggersResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2ListTriggersResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.V2ListTriggersResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2ListTriggersResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* List triggers occurrences
* List triggers occurrences
* @return The call builder
*/
public V2ListTriggersOccurrencesRequestBuilder listTriggersOccurrences() {
return new V2ListTriggersOccurrencesRequestBuilder(this);
}
/**
* List triggers occurrences
* List triggers occurrences
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2ListTriggersOccurrencesResponse listTriggersOccurrences(
V2ListTriggersOccurrencesRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
V2ListTriggersOccurrencesRequest.class,
_baseUrl,
"/api/orchestration/v2/triggers/{triggerID}/occurrences",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addQueryParams(Utils.getQueryParams(
V2ListTriggersOccurrencesRequest.class,
request,
null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2ListTriggersOccurrences",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2ListTriggersOccurrences",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2ListTriggersOccurrences",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2ListTriggersOccurrences",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2ListTriggersOccurrencesResponse.Builder _resBuilder =
V2ListTriggersOccurrencesResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2ListTriggersOccurrencesResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.V2ListTriggersOccurrencesResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2ListTriggersOccurrencesResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* List registered workflows
* List registered workflows
* @return The call builder
*/
public V2ListWorkflowsRequestBuilder listWorkflows() {
return new V2ListWorkflowsRequestBuilder(this);
}
/**
* List registered workflows
* List registered workflows
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2ListWorkflowsResponse listWorkflows(
V2ListWorkflowsRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/orchestration/v2/workflows");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addQueryParams(Utils.getQueryParams(
V2ListWorkflowsRequest.class,
request,
null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2ListWorkflows",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2ListWorkflows",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2ListWorkflows",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2ListWorkflows",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2ListWorkflowsResponse.Builder _resBuilder =
V2ListWorkflowsResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2ListWorkflowsResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.V2ListWorkflowsResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2ListWorkflowsResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Read trigger
* Read trigger
* @return The call builder
*/
public V2ReadTriggerRequestBuilder readTrigger() {
return new V2ReadTriggerRequestBuilder(this);
}
/**
* Read trigger
* Read trigger
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2ReadTriggerResponse readTrigger(
V2ReadTriggerRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
V2ReadTriggerRequest.class,
_baseUrl,
"/api/orchestration/v2/triggers/{triggerID}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2ReadTrigger",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2ReadTrigger",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2ReadTrigger",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2ReadTrigger",
Optional.of(List.of("auth:read", "orchestration:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2ReadTriggerResponse.Builder _resBuilder =
V2ReadTriggerResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2ReadTriggerResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.V2ReadTriggerResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2ReadTriggerResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Run workflow
* Run workflow
* @return The call builder
*/
public V2RunWorkflowRequestBuilder runWorkflow() {
return new V2RunWorkflowRequestBuilder(this);
}
/**
* Run workflow
* Run workflow
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2RunWorkflowResponse runWorkflow(
V2RunWorkflowRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
V2RunWorkflowRequest.class,
_baseUrl,
"/api/orchestration/v2/workflows/{workflowID}/instances",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"requestBody",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addQueryParams(Utils.getQueryParams(
V2RunWorkflowRequest.class,
request,
null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2RunWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2RunWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2RunWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2RunWorkflow",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2RunWorkflowResponse.Builder _resBuilder =
V2RunWorkflowResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2RunWorkflowResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "201")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.V2RunWorkflowResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2RunWorkflowResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Send an event to a running workflow
* Send an event to a running workflow
* @return The call builder
*/
public V2SendEventRequestBuilder sendEvent() {
return new V2SendEventRequestBuilder(this);
}
/**
* Send an event to a running workflow
* Send an event to a running workflow
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public V2SendEventResponse sendEvent(
V2SendEventRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
V2SendEventRequest.class,
_baseUrl,
"/api/orchestration/v2/instances/{instanceID}/events",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"requestBody",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"v2SendEvent",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2SendEvent",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"v2SendEvent",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"v2SendEvent",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
V2SendEventResponse.Builder _resBuilder =
V2SendEventResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
V2SendEventResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Test trigger
* Test trigger
* @return The call builder
*/
public TestTriggerRequestBuilder testTrigger() {
return new TestTriggerRequestBuilder(this);
}
/**
* Test trigger
* Test trigger
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public TestTriggerResponse testTrigger(
TestTriggerRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
TestTriggerRequest.class,
_baseUrl,
"/api/orchestration/v2/triggers/{triggerID}/test",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"requestBody",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"testTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"testTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"testTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"testTrigger",
Optional.of(List.of("auth:read", "orchestration:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
TestTriggerResponse.Builder _resBuilder =
TestTriggerResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
TestTriggerResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2TestTriggerResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withV2TestTriggerResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
V2Error _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy