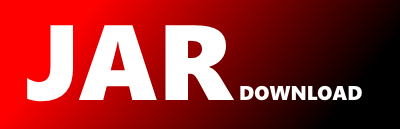
com.formance.formance_sdk.SDKWalletsV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of formance-sdk Show documentation
Show all versions of formance-sdk Show documentation
SDK enabling Java developers to easily integrate with the Formance API.
/*
* Code generated by Speakeasy (https://speakeasy.com). DO NOT EDIT.
*/
package com.formance.formance_sdk;
import com.fasterxml.jackson.core.type.TypeReference;
import com.formance.formance_sdk.models.errors.SDKError;
import com.formance.formance_sdk.models.errors.WalletsErrorResponse;
import com.formance.formance_sdk.models.operations.ConfirmHoldRequest;
import com.formance.formance_sdk.models.operations.ConfirmHoldRequestBuilder;
import com.formance.formance_sdk.models.operations.ConfirmHoldResponse;
import com.formance.formance_sdk.models.operations.CreateBalanceRequest;
import com.formance.formance_sdk.models.operations.CreateBalanceRequestBuilder;
import com.formance.formance_sdk.models.operations.CreateBalanceResponse;
import com.formance.formance_sdk.models.operations.CreateWalletRequest;
import com.formance.formance_sdk.models.operations.CreateWalletRequestBuilder;
import com.formance.formance_sdk.models.operations.CreateWalletResponse;
import com.formance.formance_sdk.models.operations.CreditWalletRequest;
import com.formance.formance_sdk.models.operations.CreditWalletRequestBuilder;
import com.formance.formance_sdk.models.operations.CreditWalletResponse;
import com.formance.formance_sdk.models.operations.DebitWalletRequest;
import com.formance.formance_sdk.models.operations.DebitWalletRequestBuilder;
import com.formance.formance_sdk.models.operations.DebitWalletResponse;
import com.formance.formance_sdk.models.operations.GetBalanceRequest;
import com.formance.formance_sdk.models.operations.GetBalanceRequestBuilder;
import com.formance.formance_sdk.models.operations.GetBalanceResponse;
import com.formance.formance_sdk.models.operations.GetHoldRequest;
import com.formance.formance_sdk.models.operations.GetHoldRequestBuilder;
import com.formance.formance_sdk.models.operations.GetHoldResponse;
import com.formance.formance_sdk.models.operations.GetHoldsRequest;
import com.formance.formance_sdk.models.operations.GetHoldsRequestBuilder;
import com.formance.formance_sdk.models.operations.GetHoldsResponse;
import com.formance.formance_sdk.models.operations.GetTransactionsRequest;
import com.formance.formance_sdk.models.operations.GetTransactionsRequestBuilder;
import com.formance.formance_sdk.models.operations.GetTransactionsResponse;
import com.formance.formance_sdk.models.operations.GetWalletRequest;
import com.formance.formance_sdk.models.operations.GetWalletRequestBuilder;
import com.formance.formance_sdk.models.operations.GetWalletResponse;
import com.formance.formance_sdk.models.operations.GetWalletSummaryRequest;
import com.formance.formance_sdk.models.operations.GetWalletSummaryRequestBuilder;
import com.formance.formance_sdk.models.operations.GetWalletSummaryResponse;
import com.formance.formance_sdk.models.operations.ListBalancesRequest;
import com.formance.formance_sdk.models.operations.ListBalancesRequestBuilder;
import com.formance.formance_sdk.models.operations.ListBalancesResponse;
import com.formance.formance_sdk.models.operations.ListWalletsRequest;
import com.formance.formance_sdk.models.operations.ListWalletsRequestBuilder;
import com.formance.formance_sdk.models.operations.ListWalletsResponse;
import com.formance.formance_sdk.models.operations.SDKMethodInterfaces.*;
import com.formance.formance_sdk.models.operations.UpdateWalletRequest;
import com.formance.formance_sdk.models.operations.UpdateWalletRequestBuilder;
import com.formance.formance_sdk.models.operations.UpdateWalletResponse;
import com.formance.formance_sdk.models.operations.VoidHoldRequest;
import com.formance.formance_sdk.models.operations.VoidHoldRequestBuilder;
import com.formance.formance_sdk.models.operations.VoidHoldResponse;
import com.formance.formance_sdk.models.operations.WalletsgetServerInfoRequestBuilder;
import com.formance.formance_sdk.models.operations.WalletsgetServerInfoResponse;
import com.formance.formance_sdk.models.shared.ServerInfo;
import com.formance.formance_sdk.utils.HTTPClient;
import com.formance.formance_sdk.utils.HTTPRequest;
import com.formance.formance_sdk.utils.Hook.AfterErrorContextImpl;
import com.formance.formance_sdk.utils.Hook.AfterSuccessContextImpl;
import com.formance.formance_sdk.utils.Hook.BeforeRequestContextImpl;
import com.formance.formance_sdk.utils.SerializedBody;
import com.formance.formance_sdk.utils.Utils.JsonShape;
import com.formance.formance_sdk.utils.Utils;
import java.io.InputStream;
import java.lang.Exception;
import java.lang.Object;
import java.lang.String;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.util.List;
import java.util.Optional;
public class SDKWalletsV1 implements
MethodCallConfirmHold,
MethodCallCreateBalance,
MethodCallCreateWallet,
MethodCallCreditWallet,
MethodCallDebitWallet,
MethodCallGetBalance,
MethodCallGetHold,
MethodCallGetHolds,
MethodCallGetTransactions,
MethodCallGetWallet,
MethodCallGetWalletSummary,
MethodCallListBalances,
MethodCallListWallets,
MethodCallUpdateWallet,
MethodCallVoidHold,
MethodCallWalletsgetServerInfo {
private final SDKConfiguration sdkConfiguration;
SDKWalletsV1(SDKConfiguration sdkConfiguration) {
this.sdkConfiguration = sdkConfiguration;
}
/**
* Confirm a hold
* @return The call builder
*/
public ConfirmHoldRequestBuilder confirmHold() {
return new ConfirmHoldRequestBuilder(this);
}
/**
* Confirm a hold
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public ConfirmHoldResponse confirmHold(
ConfirmHoldRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
ConfirmHoldRequest.class,
_baseUrl,
"/api/wallets/holds/{hold_id}/confirm",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"confirmHoldRequest",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addHeaders(Utils.getHeadersFromMetadata(request, null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"confirmHold",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"confirmHold",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"confirmHold",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"confirmHold",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
ConfirmHoldResponse.Builder _resBuilder =
ConfirmHoldResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
ConfirmHoldResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Create a balance
* @return The call builder
*/
public CreateBalanceRequestBuilder createBalance() {
return new CreateBalanceRequestBuilder(this);
}
/**
* Create a balance
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public CreateBalanceResponse createBalance(
CreateBalanceRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
CreateBalanceRequest.class,
_baseUrl,
"/api/wallets/wallets/{id}/balances",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"createBalanceRequest",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addHeaders(Utils.getHeadersFromMetadata(request, null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"createBalance",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"createBalance",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"createBalance",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"createBalance",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
CreateBalanceResponse.Builder _resBuilder =
CreateBalanceResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
CreateBalanceResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "201")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.CreateBalanceResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withCreateBalanceResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Create a new wallet
* @return The call builder
*/
public CreateWalletRequestBuilder createWallet() {
return new CreateWalletRequestBuilder(this);
}
/**
* Create a new wallet
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public CreateWalletResponse createWallet(
CreateWalletRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/wallets/wallets");
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"createWalletRequest",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addHeaders(Utils.getHeadersFromMetadata(request, null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"createWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"createWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"createWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"createWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
CreateWalletResponse.Builder _resBuilder =
CreateWalletResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
CreateWalletResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "201")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.CreateWalletResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withCreateWalletResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Credit a wallet
* @return The call builder
*/
public CreditWalletRequestBuilder creditWallet() {
return new CreditWalletRequestBuilder(this);
}
/**
* Credit a wallet
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public CreditWalletResponse creditWallet(
CreditWalletRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
CreditWalletRequest.class,
_baseUrl,
"/api/wallets/wallets/{id}/credit",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"creditWalletRequest",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addHeaders(Utils.getHeadersFromMetadata(request, null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"creditWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"creditWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"creditWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"creditWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
CreditWalletResponse.Builder _resBuilder =
CreditWalletResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
CreditWalletResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Debit a wallet
* @return The call builder
*/
public DebitWalletRequestBuilder debitWallet() {
return new DebitWalletRequestBuilder(this);
}
/**
* Debit a wallet
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public DebitWalletResponse debitWallet(
DebitWalletRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
DebitWalletRequest.class,
_baseUrl,
"/api/wallets/wallets/{id}/debit",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"debitWalletRequest",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addHeaders(Utils.getHeadersFromMetadata(request, null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"debitWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"debitWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"debitWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"debitWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
DebitWalletResponse.Builder _resBuilder =
DebitWalletResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
DebitWalletResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "201")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.DebitWalletResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withDebitWalletResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get detailed balance
* @return The call builder
*/
public GetBalanceRequestBuilder getBalance() {
return new GetBalanceRequestBuilder(this);
}
/**
* Get detailed balance
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public GetBalanceResponse getBalance(
GetBalanceRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
GetBalanceRequest.class,
_baseUrl,
"/api/wallets/wallets/{id}/balances/{balanceName}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"getBalance",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getBalance",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"getBalance",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getBalance",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
GetBalanceResponse.Builder _resBuilder =
GetBalanceResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
GetBalanceResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.GetBalanceResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withGetBalanceResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get a hold
* @return The call builder
*/
public GetHoldRequestBuilder getHold() {
return new GetHoldRequestBuilder(this);
}
/**
* Get a hold
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public GetHoldResponse getHold(
GetHoldRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
GetHoldRequest.class,
_baseUrl,
"/api/wallets/holds/{holdID}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"getHold",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getHold",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"getHold",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getHold",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
GetHoldResponse.Builder _resBuilder =
GetHoldResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
GetHoldResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.GetHoldResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withGetHoldResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get all holds for a wallet
* @return The call builder
*/
public GetHoldsRequestBuilder getHolds() {
return new GetHoldsRequestBuilder(this);
}
/**
* Get all holds for a wallet
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public GetHoldsResponse getHolds(
GetHoldsRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/wallets/holds");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addQueryParams(Utils.getQueryParams(
GetHoldsRequest.class,
request,
null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"getHolds",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getHolds",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"getHolds",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getHolds",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
GetHoldsResponse.Builder _resBuilder =
GetHoldsResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
GetHoldsResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.GetHoldsResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withGetHoldsResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
public GetTransactionsRequestBuilder getTransactions() {
return new GetTransactionsRequestBuilder(this);
}
public GetTransactionsResponse getTransactions(
GetTransactionsRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/wallets/transactions");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addQueryParams(Utils.getQueryParams(
GetTransactionsRequest.class,
request,
null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"getTransactions",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getTransactions",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"getTransactions",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getTransactions",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
GetTransactionsResponse.Builder _resBuilder =
GetTransactionsResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
GetTransactionsResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.GetTransactionsResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withGetTransactionsResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get a wallet
* @return The call builder
*/
public GetWalletRequestBuilder getWallet() {
return new GetWalletRequestBuilder(this);
}
/**
* Get a wallet
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public GetWalletResponse getWallet(
GetWalletRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
GetWalletRequest.class,
_baseUrl,
"/api/wallets/wallets/{id}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"getWallet",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getWallet",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"getWallet",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getWallet",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
GetWalletResponse.Builder _resBuilder =
GetWalletResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
GetWalletResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.GetWalletResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withGetWalletResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "404")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get wallet summary
* @return The call builder
*/
public GetWalletSummaryRequestBuilder getWalletSummary() {
return new GetWalletSummaryRequestBuilder(this);
}
/**
* Get wallet summary
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public GetWalletSummaryResponse getWalletSummary(
GetWalletSummaryRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
GetWalletSummaryRequest.class,
_baseUrl,
"/api/wallets/wallets/{id}/summary",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"getWalletSummary",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getWalletSummary",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"getWalletSummary",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getWalletSummary",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
GetWalletSummaryResponse.Builder _resBuilder =
GetWalletSummaryResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
GetWalletSummaryResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.GetWalletSummaryResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withGetWalletSummaryResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "404")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* List balances of a wallet
* @return The call builder
*/
public ListBalancesRequestBuilder listBalances() {
return new ListBalancesRequestBuilder(this);
}
/**
* List balances of a wallet
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public ListBalancesResponse listBalances(
ListBalancesRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
ListBalancesRequest.class,
_baseUrl,
"/api/wallets/wallets/{id}/balances",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"listBalances",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"listBalances",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"listBalances",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"listBalances",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
ListBalancesResponse.Builder _resBuilder =
ListBalancesResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
ListBalancesResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.ListBalancesResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withListBalancesResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* List all wallets
* @return The call builder
*/
public ListWalletsRequestBuilder listWallets() {
return new ListWalletsRequestBuilder(this);
}
/**
* List all wallets
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public ListWalletsResponse listWallets(
ListWalletsRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/wallets/wallets");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addQueryParams(Utils.getQueryParams(
ListWalletsRequest.class,
request,
null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"listWallets",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"listWallets",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"listWallets",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"listWallets",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
ListWalletsResponse.Builder _resBuilder =
ListWalletsResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
ListWalletsResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.ListWalletsResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withListWalletsResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Update a wallet
* @return The call builder
*/
public UpdateWalletRequestBuilder updateWallet() {
return new UpdateWalletRequestBuilder(this);
}
/**
* Update a wallet
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public UpdateWalletResponse updateWallet(
UpdateWalletRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
UpdateWalletRequest.class,
_baseUrl,
"/api/wallets/wallets/{id}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "PATCH");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"requestBody",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addHeaders(Utils.getHeadersFromMetadata(request, null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"updateWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"updateWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"updateWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"updateWallet",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
UpdateWalletResponse.Builder _resBuilder =
UpdateWalletResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
UpdateWalletResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Cancel a hold
* @return The call builder
*/
public VoidHoldRequestBuilder voidHold() {
return new VoidHoldRequestBuilder(this);
}
/**
* Cancel a hold
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public VoidHoldResponse voidHold(
VoidHoldRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
VoidHoldRequest.class,
_baseUrl,
"/api/wallets/holds/{hold_id}/void",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "POST");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addHeaders(Utils.getHeadersFromMetadata(request, null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"voidHold",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"voidHold",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"voidHold",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"voidHold",
Optional.of(List.of("auth:read", "wallets:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
VoidHoldResponse.Builder _resBuilder =
VoidHoldResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
VoidHoldResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get server info
* @return The call builder
*/
public WalletsgetServerInfoRequestBuilder walletsgetServerInfo() {
return new WalletsgetServerInfoRequestBuilder(this);
}
/**
* Get server info
* @return The response from the API call
* @throws Exception if the API call fails
*/
public WalletsgetServerInfoResponse walletsgetServerInfoDirect() throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/wallets/_info");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"walletsgetServerInfo",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"walletsgetServerInfo",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"walletsgetServerInfo",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"walletsgetServerInfo",
Optional.of(List.of("auth:read", "wallets:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
WalletsgetServerInfoResponse.Builder _resBuilder =
WalletsgetServerInfoResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
WalletsgetServerInfoResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
ServerInfo _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withServerInfo(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WalletsErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy