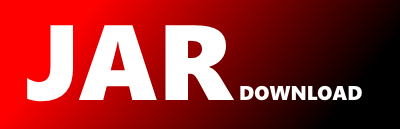
com.formance.formance_sdk.SDKWebhooksV1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of formance-sdk Show documentation
Show all versions of formance-sdk Show documentation
SDK enabling Java developers to easily integrate with the Formance API.
/*
* Code generated by Speakeasy (https://speakeasy.com). DO NOT EDIT.
*/
package com.formance.formance_sdk;
import com.fasterxml.jackson.core.type.TypeReference;
import com.formance.formance_sdk.models.errors.SDKError;
import com.formance.formance_sdk.models.errors.WebhooksErrorResponse;
import com.formance.formance_sdk.models.operations.ActivateConfigRequest;
import com.formance.formance_sdk.models.operations.ActivateConfigRequestBuilder;
import com.formance.formance_sdk.models.operations.ActivateConfigResponse;
import com.formance.formance_sdk.models.operations.ChangeConfigSecretRequest;
import com.formance.formance_sdk.models.operations.ChangeConfigSecretRequestBuilder;
import com.formance.formance_sdk.models.operations.ChangeConfigSecretResponse;
import com.formance.formance_sdk.models.operations.DeactivateConfigRequest;
import com.formance.formance_sdk.models.operations.DeactivateConfigRequestBuilder;
import com.formance.formance_sdk.models.operations.DeactivateConfigResponse;
import com.formance.formance_sdk.models.operations.DeleteConfigRequest;
import com.formance.formance_sdk.models.operations.DeleteConfigRequestBuilder;
import com.formance.formance_sdk.models.operations.DeleteConfigResponse;
import com.formance.formance_sdk.models.operations.GetManyConfigsRequest;
import com.formance.formance_sdk.models.operations.GetManyConfigsRequestBuilder;
import com.formance.formance_sdk.models.operations.GetManyConfigsResponse;
import com.formance.formance_sdk.models.operations.InsertConfigRequestBuilder;
import com.formance.formance_sdk.models.operations.InsertConfigResponse;
import com.formance.formance_sdk.models.operations.SDKMethodInterfaces.*;
import com.formance.formance_sdk.models.operations.TestConfigRequest;
import com.formance.formance_sdk.models.operations.TestConfigRequestBuilder;
import com.formance.formance_sdk.models.operations.TestConfigResponse;
import com.formance.formance_sdk.models.shared.AttemptResponse;
import com.formance.formance_sdk.models.shared.ConfigResponse;
import com.formance.formance_sdk.models.shared.ConfigUser;
import com.formance.formance_sdk.models.shared.ConfigsResponse;
import com.formance.formance_sdk.utils.HTTPClient;
import com.formance.formance_sdk.utils.HTTPRequest;
import com.formance.formance_sdk.utils.Hook.AfterErrorContextImpl;
import com.formance.formance_sdk.utils.Hook.AfterSuccessContextImpl;
import com.formance.formance_sdk.utils.Hook.BeforeRequestContextImpl;
import com.formance.formance_sdk.utils.SerializedBody;
import com.formance.formance_sdk.utils.Utils.JsonShape;
import com.formance.formance_sdk.utils.Utils;
import java.io.InputStream;
import java.lang.Exception;
import java.lang.Object;
import java.lang.String;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.util.List;
import java.util.Optional;
public class SDKWebhooksV1 implements
MethodCallActivateConfig,
MethodCallChangeConfigSecret,
MethodCallDeactivateConfig,
MethodCallDeleteConfig,
MethodCallGetManyConfigs,
MethodCallInsertConfig,
MethodCallTestConfig {
private final SDKConfiguration sdkConfiguration;
SDKWebhooksV1(SDKConfiguration sdkConfiguration) {
this.sdkConfiguration = sdkConfiguration;
}
/**
* Activate one config
* Activate a webhooks config by ID, to start receiving webhooks to its endpoint.
* @return The call builder
*/
public ActivateConfigRequestBuilder activateConfig() {
return new ActivateConfigRequestBuilder(this);
}
/**
* Activate one config
* Activate a webhooks config by ID, to start receiving webhooks to its endpoint.
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public ActivateConfigResponse activateConfig(
ActivateConfigRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
ActivateConfigRequest.class,
_baseUrl,
"/api/webhooks/configs/{id}/activate",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "PUT");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"activateConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"activateConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"activateConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"activateConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
ActivateConfigResponse.Builder _resBuilder =
ActivateConfigResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
ActivateConfigResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
ConfigResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withConfigResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WebhooksErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Change the signing secret of a config
* Change the signing secret of the endpoint of a webhooks config.
*
* If not passed or empty, a secret is automatically generated.
* The format is a random string of bytes of size 24, base64 encoded. (larger size after encoding)
*
* @return The call builder
*/
public ChangeConfigSecretRequestBuilder changeConfigSecret() {
return new ChangeConfigSecretRequestBuilder(this);
}
/**
* Change the signing secret of a config
* Change the signing secret of the endpoint of a webhooks config.
*
* If not passed or empty, a secret is automatically generated.
* The format is a random string of bytes of size 24, base64 encoded. (larger size after encoding)
*
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public ChangeConfigSecretResponse changeConfigSecret(
ChangeConfigSecretRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
ChangeConfigSecretRequest.class,
_baseUrl,
"/api/webhooks/configs/{id}/secret/change",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "PUT");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"configChangeSecret",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"changeConfigSecret",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"changeConfigSecret",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"changeConfigSecret",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"changeConfigSecret",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
ChangeConfigSecretResponse.Builder _resBuilder =
ChangeConfigSecretResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
ChangeConfigSecretResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
ConfigResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withConfigResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WebhooksErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Deactivate one config
* Deactivate a webhooks config by ID, to stop receiving webhooks to its endpoint.
* @return The call builder
*/
public DeactivateConfigRequestBuilder deactivateConfig() {
return new DeactivateConfigRequestBuilder(this);
}
/**
* Deactivate one config
* Deactivate a webhooks config by ID, to stop receiving webhooks to its endpoint.
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public DeactivateConfigResponse deactivateConfig(
DeactivateConfigRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
DeactivateConfigRequest.class,
_baseUrl,
"/api/webhooks/configs/{id}/deactivate",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "PUT");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"deactivateConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"deactivateConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"deactivateConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"deactivateConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
DeactivateConfigResponse.Builder _resBuilder =
DeactivateConfigResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
DeactivateConfigResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
ConfigResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withConfigResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WebhooksErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Delete one config
* Delete a webhooks config by ID.
* @return The call builder
*/
public DeleteConfigRequestBuilder deleteConfig() {
return new DeleteConfigRequestBuilder(this);
}
/**
* Delete one config
* Delete a webhooks config by ID.
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public DeleteConfigResponse deleteConfig(
DeleteConfigRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
DeleteConfigRequest.class,
_baseUrl,
"/api/webhooks/configs/{id}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "DELETE");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"deleteConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"deleteConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"deleteConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"deleteConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
DeleteConfigResponse.Builder _resBuilder =
DeleteConfigResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
DeleteConfigResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WebhooksErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get many configs
* Sorted by updated date descending
* @return The call builder
*/
public GetManyConfigsRequestBuilder getManyConfigs() {
return new GetManyConfigsRequestBuilder(this);
}
/**
* Get many configs
* Sorted by updated date descending
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public GetManyConfigsResponse getManyConfigs(
GetManyConfigsRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/webhooks/configs");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
_req.addQueryParams(Utils.getQueryParams(
GetManyConfigsRequest.class,
request,
null));
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"getManyConfigs",
Optional.of(List.of("auth:read", "webhooks:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getManyConfigs",
Optional.of(List.of("auth:read", "webhooks:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"getManyConfigs",
Optional.of(List.of("auth:read", "webhooks:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getManyConfigs",
Optional.of(List.of("auth:read", "webhooks:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
GetManyConfigsResponse.Builder _resBuilder =
GetManyConfigsResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
GetManyConfigsResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
ConfigsResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withConfigsResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WebhooksErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Insert a new config
* Insert a new webhooks config.
*
* The endpoint should be a valid https URL and be unique.
*
* The secret is the endpoint's verification secret.
* If not passed or empty, a secret is automatically generated.
* The format is a random string of bytes of size 24, base64 encoded. (larger size after encoding)
*
* All eventTypes are converted to lower-case when inserted.
*
* @return The call builder
*/
public InsertConfigRequestBuilder insertConfig() {
return new InsertConfigRequestBuilder(this);
}
/**
* Insert a new config
* Insert a new webhooks config.
*
* The endpoint should be a valid https URL and be unique.
*
* The secret is the endpoint's verification secret.
* If not passed or empty, a secret is automatically generated.
* The format is a random string of bytes of size 24, base64 encoded. (larger size after encoding)
*
* All eventTypes are converted to lower-case when inserted.
*
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public InsertConfigResponse insertConfig(
ConfigUser request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/webhooks/configs");
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"request",
"json",
false);
if (_serializedRequestBody == null) {
throw new Exception("Request body is required");
}
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"insertConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"insertConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"insertConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"insertConfig",
Optional.of(List.of("auth:read", "webhooks:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
InsertConfigResponse.Builder _resBuilder =
InsertConfigResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
InsertConfigResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
ConfigResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withConfigResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WebhooksErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Test one config
* Test a config by sending a webhook to its endpoint.
* @return The call builder
*/
public TestConfigRequestBuilder testConfig() {
return new TestConfigRequestBuilder(this);
}
/**
* Test one config
* Test a config by sending a webhook to its endpoint.
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public TestConfigResponse testConfig(
TestConfigRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
TestConfigRequest.class,
_baseUrl,
"/api/webhooks/configs/{id}/test",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"testConfig",
Optional.of(List.of("auth:read", "webhooks:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"testConfig",
Optional.of(List.of("auth:read", "webhooks:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"testConfig",
Optional.of(List.of("auth:read", "webhooks:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"testConfig",
Optional.of(List.of("auth:read", "webhooks:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
TestConfigResponse.Builder _resBuilder =
TestConfigResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
TestConfigResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
AttemptResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withAttemptResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
WebhooksErrorResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy