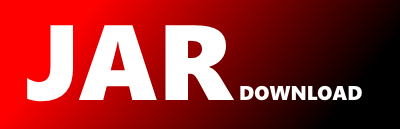
com.formance.formance_sdk.V1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of formance-sdk Show documentation
Show all versions of formance-sdk Show documentation
SDK enabling Java developers to easily integrate with the Formance API.
/*
* Code generated by Speakeasy (https://speakeasy.com). DO NOT EDIT.
*/
package com.formance.formance_sdk;
import com.fasterxml.jackson.core.type.TypeReference;
import com.formance.formance_sdk.models.errors.SDKError;
import com.formance.formance_sdk.models.operations.CreateClientRequestBuilder;
import com.formance.formance_sdk.models.operations.CreateClientResponse;
import com.formance.formance_sdk.models.operations.CreateSecretRequest;
import com.formance.formance_sdk.models.operations.CreateSecretRequestBuilder;
import com.formance.formance_sdk.models.operations.CreateSecretResponse;
import com.formance.formance_sdk.models.operations.DeleteClientRequest;
import com.formance.formance_sdk.models.operations.DeleteClientRequestBuilder;
import com.formance.formance_sdk.models.operations.DeleteClientResponse;
import com.formance.formance_sdk.models.operations.DeleteSecretRequest;
import com.formance.formance_sdk.models.operations.DeleteSecretRequestBuilder;
import com.formance.formance_sdk.models.operations.DeleteSecretResponse;
import com.formance.formance_sdk.models.operations.GetOIDCWellKnownsRequestBuilder;
import com.formance.formance_sdk.models.operations.GetOIDCWellKnownsResponse;
import com.formance.formance_sdk.models.operations.GetServerInfoRequestBuilder;
import com.formance.formance_sdk.models.operations.GetServerInfoResponse;
import com.formance.formance_sdk.models.operations.ListClientsRequestBuilder;
import com.formance.formance_sdk.models.operations.ListClientsResponse;
import com.formance.formance_sdk.models.operations.ListUsersRequestBuilder;
import com.formance.formance_sdk.models.operations.ListUsersResponse;
import com.formance.formance_sdk.models.operations.ReadClientRequest;
import com.formance.formance_sdk.models.operations.ReadClientRequestBuilder;
import com.formance.formance_sdk.models.operations.ReadClientResponse;
import com.formance.formance_sdk.models.operations.ReadUserRequest;
import com.formance.formance_sdk.models.operations.ReadUserRequestBuilder;
import com.formance.formance_sdk.models.operations.ReadUserResponse;
import com.formance.formance_sdk.models.operations.SDKMethodInterfaces.*;
import com.formance.formance_sdk.models.operations.UpdateClientRequest;
import com.formance.formance_sdk.models.operations.UpdateClientRequestBuilder;
import com.formance.formance_sdk.models.operations.UpdateClientResponse;
import com.formance.formance_sdk.models.shared.CreateClientRequest;
import com.formance.formance_sdk.models.shared.ServerInfo;
import com.formance.formance_sdk.utils.HTTPClient;
import com.formance.formance_sdk.utils.HTTPRequest;
import com.formance.formance_sdk.utils.Hook.AfterErrorContextImpl;
import com.formance.formance_sdk.utils.Hook.AfterSuccessContextImpl;
import com.formance.formance_sdk.utils.Hook.BeforeRequestContextImpl;
import com.formance.formance_sdk.utils.SerializedBody;
import com.formance.formance_sdk.utils.Utils.JsonShape;
import com.formance.formance_sdk.utils.Utils;
import java.io.InputStream;
import java.lang.Exception;
import java.lang.Object;
import java.lang.String;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.util.List;
import java.util.Optional;
public class V1 implements
MethodCallCreateClient,
MethodCallCreateSecret,
MethodCallDeleteClient,
MethodCallDeleteSecret,
MethodCallGetOIDCWellKnowns,
MethodCallGetServerInfo,
MethodCallListClients,
MethodCallListUsers,
MethodCallReadClient,
MethodCallReadUser,
MethodCallUpdateClient {
private final SDKConfiguration sdkConfiguration;
V1(SDKConfiguration sdkConfiguration) {
this.sdkConfiguration = sdkConfiguration;
}
/**
* Create client
* @return The call builder
*/
public CreateClientRequestBuilder createClient() {
return new CreateClientRequestBuilder(this);
}
/**
* Create client
* @return The response from the API call
* @throws Exception if the API call fails
*/
public CreateClientResponse createClientDirect() throws Exception {
return createClient(Optional.empty());
}
/**
* Create client
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public CreateClientResponse createClient(
Optional extends CreateClientRequest> request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/auth/clients");
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference>() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"request",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"createClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"createClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"createClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"createClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
CreateClientResponse.Builder _resBuilder =
CreateClientResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
CreateClientResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "201")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.CreateClientResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withCreateClientResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Add a secret to a client
* @return The call builder
*/
public CreateSecretRequestBuilder createSecret() {
return new CreateSecretRequestBuilder(this);
}
/**
* Add a secret to a client
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public CreateSecretResponse createSecret(
CreateSecretRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
CreateSecretRequest.class,
_baseUrl,
"/api/auth/clients/{clientId}/secrets",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"createSecretRequest",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"createSecret",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"createSecret",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"createSecret",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"createSecret",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
CreateSecretResponse.Builder _resBuilder =
CreateSecretResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
CreateSecretResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.CreateSecretResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withCreateSecretResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Delete client
* @return The call builder
*/
public DeleteClientRequestBuilder deleteClient() {
return new DeleteClientRequestBuilder(this);
}
/**
* Delete client
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public DeleteClientResponse deleteClient(
DeleteClientRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
DeleteClientRequest.class,
_baseUrl,
"/api/auth/clients/{clientId}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "DELETE");
_req.addHeader("Accept", "*/*")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"deleteClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"deleteClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"deleteClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"deleteClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
DeleteClientResponse.Builder _resBuilder =
DeleteClientResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
DeleteClientResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Delete a secret from a client
* @return The call builder
*/
public DeleteSecretRequestBuilder deleteSecret() {
return new DeleteSecretRequestBuilder(this);
}
/**
* Delete a secret from a client
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public DeleteSecretResponse deleteSecret(
DeleteSecretRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
DeleteSecretRequest.class,
_baseUrl,
"/api/auth/clients/{clientId}/secrets/{secretId}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "DELETE");
_req.addHeader("Accept", "*/*")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"deleteSecret",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"deleteSecret",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"deleteSecret",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"deleteSecret",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
DeleteSecretResponse.Builder _resBuilder =
DeleteSecretResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
DeleteSecretResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "204")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Retrieve OpenID connect well-knowns.
* @return The call builder
*/
public GetOIDCWellKnownsRequestBuilder getOIDCWellKnowns() {
return new GetOIDCWellKnownsRequestBuilder(this);
}
/**
* Retrieve OpenID connect well-knowns.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public GetOIDCWellKnownsResponse getOIDCWellKnownsDirect() throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/auth/.well-known/openid-configuration");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "*/*")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"getOIDCWellKnowns",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getOIDCWellKnowns",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"getOIDCWellKnowns",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getOIDCWellKnowns",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
GetOIDCWellKnownsResponse.Builder _resBuilder =
GetOIDCWellKnownsResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
GetOIDCWellKnownsResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
// no content
return _res;
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Get server info
* @return The call builder
*/
public GetServerInfoRequestBuilder getServerInfo() {
return new GetServerInfoRequestBuilder(this);
}
/**
* Get server info
* @return The response from the API call
* @throws Exception if the API call fails
*/
public GetServerInfoResponse getServerInfoDirect() throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/auth/_info");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"getServerInfo",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getServerInfo",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"getServerInfo",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"getServerInfo",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
GetServerInfoResponse.Builder _resBuilder =
GetServerInfoResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
GetServerInfoResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
ServerInfo _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withServerInfo(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* List clients
* @return The call builder
*/
public ListClientsRequestBuilder listClients() {
return new ListClientsRequestBuilder(this);
}
/**
* List clients
* @return The response from the API call
* @throws Exception if the API call fails
*/
public ListClientsResponse listClientsDirect() throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/auth/clients");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"listClients",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"listClients",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"listClients",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"listClients",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
ListClientsResponse.Builder _resBuilder =
ListClientsResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
ListClientsResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.ListClientsResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withListClientsResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* List users
* List users
* @return The call builder
*/
public ListUsersRequestBuilder listUsers() {
return new ListUsersRequestBuilder(this);
}
/**
* List users
* List users
* @return The response from the API call
* @throws Exception if the API call fails
*/
public ListUsersResponse listUsersDirect() throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
_baseUrl,
"/api/auth/users");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"listUsers",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"listUsers",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"listUsers",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"listUsers",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
ListUsersResponse.Builder _resBuilder =
ListUsersResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
ListUsersResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.ListUsersResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withListUsersResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Read client
* @return The call builder
*/
public ReadClientRequestBuilder readClient() {
return new ReadClientRequestBuilder(this);
}
/**
* Read client
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public ReadClientResponse readClient(
ReadClientRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
ReadClientRequest.class,
_baseUrl,
"/api/auth/clients/{clientId}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"readClient",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"readClient",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"readClient",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"readClient",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
ReadClientResponse.Builder _resBuilder =
ReadClientResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
ReadClientResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.ReadClientResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withReadClientResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Read user
* Read user
* @return The call builder
*/
public ReadUserRequestBuilder readUser() {
return new ReadUserRequestBuilder(this);
}
/**
* Read user
* Read user
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public ReadUserResponse readUser(
ReadUserRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
ReadUserRequest.class,
_baseUrl,
"/api/auth/users/{userId}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"readUser",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"readUser",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"readUser",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"readUser",
Optional.of(List.of("auth:read", "auth:read")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
ReadUserResponse.Builder _resBuilder =
ReadUserResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
ReadUserResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.ReadUserResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withReadUserResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
/**
* Update client
* @return The call builder
*/
public UpdateClientRequestBuilder updateClient() {
return new UpdateClientRequestBuilder(this);
}
/**
* Update client
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public UpdateClientResponse updateClient(
UpdateClientRequest request) throws Exception {
String _baseUrl = Utils.templateUrl(
this.sdkConfiguration.serverUrl, this.sdkConfiguration.getServerVariableDefaults());
String _url = Utils.generateURL(
UpdateClientRequest.class,
_baseUrl,
"/api/auth/clients/{clientId}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "PUT");
Object _convertedRequest = Utils.convertToShape(
request,
JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest,
"updateClientRequest",
"json",
false);
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
SDKConfiguration.USER_AGENT);
Utils.configureSecurity(_req,
this.sdkConfiguration.securitySource.getSecurity());
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl(
"updateClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"updateClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl(
"updateClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl(
"updateClient",
Optional.of(List.of("auth:read", "auth:write")),
sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
UpdateClientResponse.Builder _resBuilder =
UpdateClientResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
UpdateClientResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.formance.formance_sdk.models.shared.UpdateClientResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withUpdateClientResponse(Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.extractByteArrayFromBody(_httpRes));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "default")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.extractByteArrayFromBody(_httpRes));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.extractByteArrayFromBody(_httpRes));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy