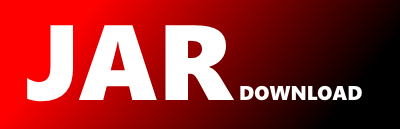
com.formkiq.server.api.ClientsController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of formkiq-server Show documentation
Show all versions of formkiq-server Show documentation
Server-side integration for the FormKiQ ios application
/*
* Copyright (C) 2016 FormKiQ Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.formkiq.server.api;
import static javax.servlet.http.HttpServletResponse.SC_FORBIDDEN;
import static javax.servlet.http.HttpServletResponse.SC_INTERNAL_SERVER_ERROR;
import static javax.servlet.http.HttpServletResponse.SC_NOT_FOUND;
import static javax.servlet.http.HttpServletResponse.SC_OK;
import static javax.servlet.http.HttpServletResponse.SC_UNAUTHORIZED;
import static org.springframework.web.bind.annotation.RequestMethod.GET;
import static org.springframework.web.bind.annotation.RequestMethod.POST;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.transaction.Transactional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.access.annotation.Secured;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.formkiq.server.domain.type.ClientDTO;
import com.formkiq.server.domain.type.ClientListDTO;
import com.formkiq.server.domain.type.OAuthGrantTypes;
import com.formkiq.server.service.OAuthService;
import com.formkiq.server.service.SpringSecurityService;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiResponse;
import io.swagger.annotations.ApiResponses;
/**
* Form Group Rest Services.
*
*/
@RestController
public class ClientsController extends AbstractRestController {
/** Client Create URL. */
public static final String API_CLIENT = "/api/clients";
/** Client GET URL. */
public static final String API_CLIENT_GET = API_CLIENT + "/get";
/** Client Save URL. */
public static final String API_CLIENT_SAVE = API_CLIENT + "/save";
/** Client Create URL. */
public static final String API_CLIENT_LIST = API_CLIENT + "/list";
/** Client Delete URL. */
public static final String API_CLIENT_DELETE = API_CLIENT + "/delete";
/** OAuthService. */
@Autowired
private OAuthService oauthservice;
/** SpringSecurityService. */
@Autowired
private SpringSecurityService securityService;
/**
* Gets a Client.
* @param request {@link HttpServletRequest}
* @param response {@link HttpServletResponse}
* @param client {@link String}
* @throws IOException IOException
* @return {@link ClientDTO}
*/
@ApiOperation(value = "get", nickname = "Client Get")
@ApiResponses(value = {
@ApiResponse(code = SC_OK, message = "Success",
response = ClientDTO.class),
@ApiResponse(code = SC_UNAUTHORIZED, message = "Unauthorized"),
@ApiResponse(code = SC_FORBIDDEN, message = "Forbidden"),
@ApiResponse(code = SC_NOT_FOUND, message = "Not Found"),
@ApiResponse(code = SC_INTERNAL_SERVER_ERROR,
message = "Failure") })
@Transactional
@RequestMapping(value = API_CLIENT_GET, method = GET)
public ClientDTO get(
final HttpServletRequest request,
final HttpServletResponse response,
@RequestParam(value = "client", required = true)
final String client) throws IOException {
getApiVersion(request);
this.securityService.verifyUserHasAccessToClient(client);
UserDetails user = this.securityService.getUserDetails();
return this.oauthservice.findClient(user, client);
}
/**
* Creates Form Group.
* @param request {@link HttpServletRequest}
* @param name {@link String}
* @param client {@link String}
* @param secret {@link String}
* @param granttypes {@link String[]}
* @return ApiStringResponse
*/
@ApiOperation(value = "get", nickname = "Client Save")
@ApiResponses(value = {
@ApiResponse(code = SC_OK, message = "Success",
response = ApiMessageResponse.class),
@ApiResponse(code = SC_UNAUTHORIZED, message = "Unauthorized"),
@ApiResponse(code = SC_FORBIDDEN, message = "Forbidden"),
@ApiResponse(code = SC_NOT_FOUND, message = "Not Found"),
@ApiResponse(code = SC_INTERNAL_SERVER_ERROR,
message = "Failure") })
@Transactional
@Secured({ "ROLE_ADMIN" })
@RequestMapping(value = API_CLIENT_SAVE, method = POST)
public ApiMessageResponse save(
final HttpServletRequest request,
@RequestParam(value = "clientname", required = false)
final String name,
@RequestParam(value = "client", required = true)
final String client,
@RequestParam(value = "clientsecret", required = false)
final String secret,
@RequestParam(value = "granttype", required = true)
final String[] granttypes) {
List types = Arrays.asList(granttypes).stream()
.map(s -> OAuthGrantTypes.valueOf(s.toUpperCase()))
.collect(Collectors.toList());
this.oauthservice.save(name, client, secret, types);
return new ApiMessageResponse("Client saved");
}
/**
* List Apps.
* @param request {@link HttpServletRequest}
* @param token {@link String}
* @return ClientListDTO
*/
@ApiOperation(value = "list", nickname = "Client List")
@ApiResponses(value = {
@ApiResponse(code = SC_OK, message = "Success",
response = ClientListDTO.class),
@ApiResponse(code = SC_UNAUTHORIZED, message = "Unauthorized"),
@ApiResponse(code = SC_FORBIDDEN, message = "Forbidden"),
@ApiResponse(code = SC_NOT_FOUND, message = "Not Found"),
@ApiResponse(code = SC_INTERNAL_SERVER_ERROR,
message = "Failure") })
@Transactional
@Secured({ "ROLE_ADMIN" })
@RequestMapping(value = API_CLIENT_LIST, method = GET)
public ClientListDTO list(final HttpServletRequest request,
@RequestParam(value = "token", required = false)
final String token) {
return this.oauthservice.list(token);
}
/**
* Delete Client.
* @param request {@link HttpServletRequest}
* @param client {@link String}
* @return ApiStringResponse
*/
@ApiOperation(value = "delete", nickname = "Client Delete")
@ApiResponses(value = {
@ApiResponse(code = SC_OK, message = "Success",
response = ApiMessageResponse.class),
@ApiResponse(code = SC_UNAUTHORIZED, message = "Unauthorized"),
@ApiResponse(code = SC_FORBIDDEN, message = "Forbidden"),
@ApiResponse(code = SC_NOT_FOUND, message = "Not Found"),
@ApiResponse(code = SC_INTERNAL_SERVER_ERROR,
message = "Failure") })
@Transactional
@Secured({ "ROLE_ADMIN" })
@RequestMapping(value = API_CLIENT_DELETE, method = POST)
public ApiMessageResponse delete(final HttpServletRequest request,
@RequestParam(value = "client", required = true)
final String client) {
this.oauthservice.deleteClient(client);
return new ApiMessageResponse("Client deleted");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy