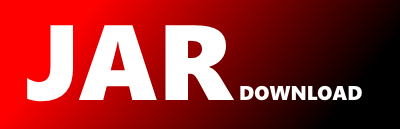
com.formkiq.server.api.UserSettingsController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of formkiq-server Show documentation
Show all versions of formkiq-server Show documentation
Server-side integration for the FormKiQ ios application
/*
* Copyright (C) 2016 FormKiQ Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.formkiq.server.api;
import static javax.servlet.http.HttpServletResponse.SC_FORBIDDEN;
import static javax.servlet.http.HttpServletResponse.SC_INTERNAL_SERVER_ERROR;
import static javax.servlet.http.HttpServletResponse.SC_NOT_FOUND;
import static javax.servlet.http.HttpServletResponse.SC_OK;
import static javax.servlet.http.HttpServletResponse.SC_UNAUTHORIZED;
import static org.springframework.web.bind.annotation.RequestMethod.POST;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.transaction.Transactional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.formkiq.server.domain.type.UserSettings;
import com.formkiq.server.service.SpringSecurityService;
import com.formkiq.server.service.UserService;
import io.swagger.annotations.ApiImplicitParam;
import io.swagger.annotations.ApiImplicitParams;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiResponse;
import io.swagger.annotations.ApiResponses;
/**
* User Settings Rest Service.
*
*/
@RestController
public class UserSettingsController extends AbstractRestController {
/** User Settings Root URL. */
private static final String ROOT = "/api/users/settings";
/** User Settings Save. */
public static final String API_USER_SETTINGS_SAVE = ROOT + "/save";
/** User Settings Delete. */
public static final String API_USER_SETTINGS_DELETE = ROOT + "/delete";
/** SpringSecurityService. */
@Autowired
private SpringSecurityService securityService;
/** UserService. */
@Autowired
private UserService userservice;
/**
* Delete User Settings.
* @param request {@link HttpServletRequest}
* @return {@link ApiMessageResponse}
*/
@ApiOperation(value = "deleteUserSettings", nickname = "deleteUserSettings")
@ApiImplicitParams({
@ApiImplicitParam(name = "email", value = "User's email",
required = false, dataType = "string", paramType = "query"),
@ApiImplicitParam(name = "setting", value = "Setting name",
required = true, dataType = "string", paramType = "query")
})
@ApiResponses(value = {
@ApiResponse(code = SC_OK, message = "Success",
response = ApiMessageResponse.class),
@ApiResponse(code = SC_UNAUTHORIZED, message = "Unauthorized"),
@ApiResponse(code = SC_FORBIDDEN, message = "Forbidden"),
@ApiResponse(code = SC_NOT_FOUND, message = "Not Found"),
@ApiResponse(code = SC_INTERNAL_SERVER_ERROR,
message = "Failure") })
@Transactional
@RequestMapping(value = API_USER_SETTINGS_DELETE, method = POST)
public ApiMessageResponse deleteUserSettings(
final HttpServletRequest request) {
Map map = request.getParameterMap();
String email = getParameter(map, "email", false);
UserSettings setting = getParameter(map, "setting", null,
UserSettings.class);
if (StringUtils.isEmpty(email) || !this.securityService.isAdmin()) {
email = this.securityService.getUsername();
}
this.userservice.deleteUserSettings(email, setting);
return new ApiMessageResponse("Setting Deleted");
}
/**
* Save User Settings.
* @param request {@link HttpServletRequest}
* @return {@link ApiMessageResponse}
*/
@ApiOperation(value = "saveUserSettings", nickname = "saveUserSettings")
@ApiImplicitParams({
@ApiImplicitParam(name = "email", value = "User's email",
required = false, dataType = "string", paramType = "query"),
@ApiImplicitParam(name = "setting", value = "Setting name",
required = true, dataType = "string", paramType = "query"),
@ApiImplicitParam(name = "value", value = "Setting value",
required = true, dataType = "string", paramType = "query")
})
@ApiResponses(value = {
@ApiResponse(code = SC_OK, message = "Success",
response = ApiMessageResponse.class),
@ApiResponse(code = SC_UNAUTHORIZED, message = "Unauthorized"),
@ApiResponse(code = SC_FORBIDDEN, message = "Forbidden"),
@ApiResponse(code = SC_NOT_FOUND, message = "Not Found"),
@ApiResponse(code = SC_INTERNAL_SERVER_ERROR,
message = "Failure") })
@Transactional
@RequestMapping(value = API_USER_SETTINGS_SAVE, method = POST)
public ApiMessageResponse saveUserSettings(
final HttpServletRequest request) {
Map map = request.getParameterMap();
String email = getParameter(map, "email", false);
UserSettings setting = getParameter(map, "setting", null,
UserSettings.class);
String value = getParameter(map, "value", true);
if (StringUtils.isEmpty(email) || !this.securityService.isAdmin()) {
email = this.securityService.getUsername();
}
this.userservice.saveUserSettings(email, setting, value);
return new ApiMessageResponse("Setting Saved");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy