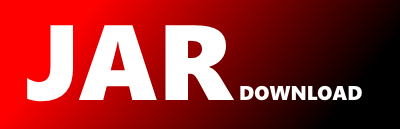
com.formkiq.server.util.Zips Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of formkiq-server Show documentation
Show all versions of formkiq-server Show documentation
Server-side integration for the FormKiQ ios application
package com.formkiq.server.util;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
import java.util.zip.ZipOutputStream;
import org.apache.commons.io.IOUtils;
/**
* Helper method for dealing with Zip Files.
*
*/
public final class Zips {
/**
* Extract Zip file to Map.
* @param bytes byte[]
* @return {@link Map}
* @throws IOException IOException
*/
public static Map extractZipToMap(final byte[] bytes)
throws IOException {
Map map = new HashMap();
ByteArrayInputStream is = new ByteArrayInputStream(bytes);
ZipInputStream zipStream = new ZipInputStream(is);
try {
ZipEntry entry = null;
while ((entry = zipStream.getNextEntry()) != null) {
String filename = entry.getName();
String data = IOUtils.toString(zipStream);
map.put(filename, data);
}
} finally {
IOUtils.closeQuietly(is);
IOUtils.closeQuietly(zipStream);
}
return map;
}
/**
* Create Zip file.
* @param map {@link Map}
* @return byte[] zip file
* @throws IOException IOException
*/
public static byte[] zipFile(final Map map)
throws IOException {
ByteArrayOutputStream bs = new ByteArrayOutputStream();
ZipOutputStream zip = new ZipOutputStream(bs);
for (Map.Entry e : map.entrySet()) {
String name = e.getKey();
byte[] data = e.getValue();
ZipEntry ze = new ZipEntry(name + ".zip");
zip.putNextEntry(ze);
zip.write(data, 0, data.length);
zip.closeEntry();
}
byte[] bytes = bs.toByteArray();
IOUtils.closeQuietly(bs);
IOUtils.closeQuietly(zip);
return bytes;
}
/**
* Zips data.
* @param name {@link String}
* @param data {@link String}
* @return byte[]
* @throws IOException IOException
*/
public static byte[] zipFile(final String name, final String data)
throws IOException {
ByteArrayOutputStream bs = new ByteArrayOutputStream();
ZipOutputStream zip = new ZipOutputStream(bs);
byte[] dataBytes = Strings.getBytes(data);
ZipEntry ze = new ZipEntry(name);
zip.putNextEntry(ze);
zip.write(dataBytes, 0, dataBytes.length);
zip.closeEntry();
zip.finish();
byte[] returnBytes = bs.toByteArray();
IOUtils.closeQuietly(bs);
IOUtils.closeQuietly(zip);
return returnBytes;
}
/**
* private constructor.
*/
private Zips() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy