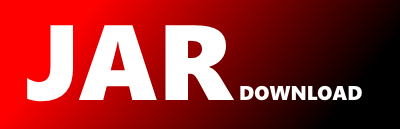
com.formkiq.vision.crafter.DocumentBlockRemoveDuplicate Maven / Gradle / Ivy
/*
* Copyright (C) 2018 FormKiQ Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.formkiq.vision.crafter;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
import java.util.function.Function;
import com.formkiq.vision.document.DocumentBlock;
import com.formkiq.vision.document.DocumentBlockRectangle;
/**
* {@link DocumentBlock} remove Duplicates {@link Function}.
*
*/
public class DocumentBlockRemoveDuplicate
implements Function, List> {
/** int. */
private int delta;
/**
* constructor.
* @param deltarect int
*/
public DocumentBlockRemoveDuplicate(final int deltarect) {
this.delta = deltarect;
}
@Override
public List apply(final List list) {
Set keys = new HashSet<>();
for (Iterator i = list.iterator(); i.hasNext();) {
DocumentBlockRectangle rect = i.next();
Collection removeKeys = generateRemoveKeys(rect);
if (removeKeys.stream().anyMatch(keys::contains)) {
i.remove();
}
keys.addAll(removeKeys);
}
return list;
}
/**
* Generate Remove Keys with delta.
*
* @param r {@link DocumentBlock}
* @return {@link Collection} of remove Key {@link String}.
*/
Collection generateRemoveKeys(final DocumentBlockRectangle r) {
int x1 = (int) r.getLowerLeftX();
int y1 = (int) r.getLowerLeftY();
int x2 = (int) r.getUpperRightX();
int y2 = (int) r.getUpperRightY();
Collection list = new HashSet<>();
for (int i = -this.delta; i <= this.delta; i++) {
for (int j = -this.delta; j <= this.delta; j++) {
for (int k = -this.delta; k <= this.delta; k++) {
for (int l = -this.delta; l <= this.delta; l++) {
list.add((x1 + i) + "_" + (y1 + j) + "_" + (x2 + k)
+ "_" + (y2 + l));
}
}
}
}
return list;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy