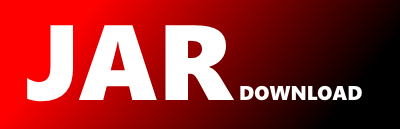
com.formkiq.vision.crafter.DocumentRowLayout Maven / Gradle / Ivy
/*
* Copyright (C) 2018 FormKiQ Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.formkiq.vision.crafter;
import java.io.Serializable;
import java.util.Collection;
import java.util.Collections;
import com.formkiq.vision.document.DocumentBlock;
import com.formkiq.vision.document.DocumentBlockRectangle;
import com.formkiq.vision.predicate.DocumentBlockHorizontalLinePredicate;
import com.formkiq.vision.predicate.DocumentBlockVerticalLinePredicate;
/**
* Document Row built from {@link Collection} of {@link DocumentBlockRectangle}. Also
* holds nestblocks which are {@link DocumentBlockRectangle} that are contained by one of
* the blocks.
*
*/
public class DocumentRowLayout implements DocumentBlockRectangle, Serializable {
/** serialVersionUID. */
private static final long serialVersionUID = 4266273797244939183L;
/** {@link DocumentBlock}. */
private DocumentBlockRectangle block;
/** {@link Collection} {@link DocumentBlock}. */
private Collection blocks;
/** {@link Collection} {@link DocumentBlock}. */
private Collection horizontalLines;
/** {@link Collection} {@link DocumentBlock}. */
private Collection verticalLines;
/**
* constructor.
*/
public DocumentRowLayout() {
this.blocks = Collections.emptyList();
this.horizontalLines = Collections.emptyList();
this.verticalLines = Collections.emptyList();
}
/**
* constructor.
* @param documentblock {@link DocumentBlock}
*/
public DocumentRowLayout(final DocumentBlockRectangle documentblock) {
this();
setBlock(documentblock);
}
/**
* @return {@link DocumentBlockRectangle}
*/
public DocumentBlockRectangle getBlock() {
return this.block;
}
/**
* @param documentblock {@link DocumentBlock}
*/
public void setBlock(final DocumentBlockRectangle documentblock) {
this.block = documentblock;
}
/**
* @return {@link Collection} {@link DocumentRowBlock}
*/
public Collection getBlocks() {
return this.blocks;
}
/**
* @param documentblocks {@link Collection} {@link DocumentRowBlock}
*/
public void setBlocks(final Collection documentblocks) {
this.blocks = documentblocks;
}
@Override
public float getLowerLeftX() {
return this.block.getLowerLeftX();
}
@Override
public float getLowerLeftY() {
return this.block.getLowerLeftY();
}
@Override
public float getUpperRightX() {
return this.block.getUpperRightX();
}
@Override
public float getUpperRightY() {
return this.block.getUpperRightY();
}
/**
* @return {@link Collection} {@link DocumentBlockRectangle}
*/
public Collection getHorizontalLines() {
return this.horizontalLines;
}
/**
* @param lines {@link Collection} {@link DocumentBlock}
*/
public void setHorizontalLines(final Collection lines) {
if (lines.stream()
.filter(new DocumentBlockHorizontalLinePredicate().negate())
.findFirst().isPresent()) {
throw new IllegalArgumentException(
"Not all lines are Horizontal Lines.");
}
this.horizontalLines = lines;
}
/**
* @return {@link Collection} {@link DocumentBlock}
*/
public Collection getVerticalLines() {
return this.verticalLines;
}
/**
* @param lines {@link Collection} {@link DocumentBlock}
*/
public void setVerticalLines(final Collection lines) {
if (lines.stream()
.filter(new DocumentBlockVerticalLinePredicate().negate())
.findFirst().isPresent()) {
throw new IllegalArgumentException(
"Not all lines are Vertical Lines.");
}
this.verticalLines = lines;
}
@Override
public String toString() {
return this.block.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy