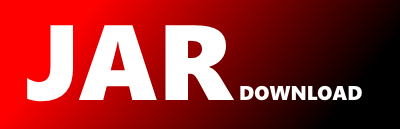
com.formkiq.vision.document.DocumentBlock Maven / Gradle / Ivy
/*
* Copyright (C) 2018 FormKiQ Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.formkiq.vision.document;
import static java.lang.Math.abs;
import java.io.Serializable;
/**
* Document Block.
*
*/
public class DocumentBlock implements DocumentBlockRectangle, Serializable {
/** serialVersionUID. */
private static final long serialVersionUID = 4708996570546973347L;
/** Lower Left X. */
private float lowerLeftX;
/** Lower Left Y. */
private float lowerLeftY;
/** Upper Right X. */
private float upperRightX;
/** Upper Right Y. */
private float upperRightY;
/**
* constructor.
*/
public DocumentBlock() {
}
/**
* constructor.
* @param rect {@link DocumentBlockRectangle}
*/
public DocumentBlock(final DocumentBlockRectangle rect) {
this(rect.getLowerLeftX(), rect.getLowerLeftY(), rect.getUpperRightX(),
rect.getUpperRightY());
}
/**
* constructor.
* @param lowerX float
* @param lowerY float
* @param upperX float
* @param upperY float
*/
public DocumentBlock(final float lowerX, final float lowerY,
final float upperX, final float upperY) {
this();
setLowerLeftX(lowerX);
setLowerLeftY(lowerY);
setUpperRightX(upperX);
setUpperRightY(upperY);
}
/**
* constructor.
* @param lowerX float
* @param lowerY float
* @param upperX float
* @param upperY float
*/
public DocumentBlock(final String lowerX, final String lowerY,
final String upperX, final String upperY) {
this();
setLowerLeftX(Float.valueOf(lowerX).floatValue());
setLowerLeftY(Float.valueOf(lowerY).floatValue());
setUpperRightX(Float.valueOf(upperX).floatValue());
setUpperRightY(Float.valueOf(upperY).floatValue());
}
/**
* @return float
*/
@Override
public float getLowerLeftX() {
return this.lowerLeftX;
}
/**
* @param x float
*/
public void setLowerLeftX(final float x) {
this.lowerLeftX = x;
}
/**
* @return float
*/
@Override
public float getLowerLeftY() {
return this.lowerLeftY;
}
/**
* @param y float
*/
public void setLowerLeftY(final float y) {
this.lowerLeftY = y;
}
/**
* @return float
*/
@Override
public float getUpperRightX() {
return this.upperRightX;
}
/**
* @param x float
*/
public void setUpperRightX(final float x) {
this.upperRightX = x;
}
/**
* @return float
*/
@Override
public float getUpperRightY() {
return this.upperRightY;
}
/**
* @param y float
*/
public void setUpperRightY(final float y) {
this.upperRightY = y;
}
/**
* This will get the height of this rectangle as calculated by
* upperRightY - lowerLeftY.
*
* @return The height of this rectangle.
*/
public float getHeight() {
return getUpperRightY() - getLowerLeftY();
}
/**
* This will get the width of this rectangle as calculated by
* upperRightX - lowerLeftX.
*
* @return The width of this rectangle.
*/
public float getWidth() {
return getUpperRightX() - getLowerLeftX();
}
@Override
public String toString() {
return "[" + getLowerLeftX() + "," + getLowerLeftY() + ","
+ getUpperRightX() + "," + getUpperRightY() + "]";
}
/**
* Block Area.
* @return float
*/
public float getArea() {
return getWidth() * getHeight();
}
/**
* Is float equal.
* @param r0 float
* @param r1 float
* @return boolean
*/
public static boolean isEquals(final float r0, final float r1) {
return abs(r0 - r1) < 2;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy