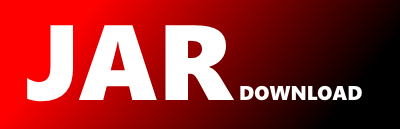
com.formkiq.vision.pdf.ImagePDFStreamEngine Maven / Gradle / Ivy
/*
* Copyright (C) 2018 FormKiQ Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.formkiq.vision.pdf;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.imageio.ImageIO;
import org.apache.commons.lang3.Range;
import org.apache.pdfbox.contentstream.PDFStreamEngine;
import org.apache.pdfbox.contentstream.operator.Operator;
import org.apache.pdfbox.cos.COSBase;
import org.apache.pdfbox.cos.COSName;
import org.apache.pdfbox.pdmodel.common.PDRectangle;
import org.apache.pdfbox.pdmodel.graphics.PDXObject;
import org.apache.pdfbox.pdmodel.graphics.form.PDFormXObject;
import org.apache.pdfbox.pdmodel.graphics.image.PDImageXObject;
/**
* {@link PDFStreamEngine} for Images.
*
*/
public class ImagePDFStreamEngine extends PDFStreamEngine {
/** {@link PdfDocumentObjects}. */
private PdfDocumentObjects tokenResult;
/** {@link List} {@link PdfImage}. */
private List images;
/**
* constructor.
* @param result {@link PdfDocumentObjects}
*/
public ImagePDFStreamEngine(final PdfDocumentObjects result) {
this.images = new ArrayList<>();
this.tokenResult = result;
}
/**
* This is used to handle an operation.
*
* @param operator The operation to perform.
* @param operands The list of arguments.
*
* @throws IOException If there is an error processing the operation.
*/
@Override
protected void processOperator(final Operator operator,
final List operands) throws IOException {
String operation = operator.getName();
if ("Do".equals(operation)) {
COSName objectName = (COSName) operands.get(0);
PDXObject xobject = getResources().getXObject(objectName);
if (xobject instanceof PDImageXObject) {
PDImageXObject image = (PDImageXObject) xobject;
int imageWidth = image.getWidth();
int imageHeight = image.getHeight();
PDRectangle imageRect = this.tokenResult
.getObjectRectangle(objectName.getName());
PdfImage pdfImage = new PdfImage();
pdfImage.setImageName(objectName.getName());
pdfImage.setImage(toBytes(image, pdfImage.getImagetype()));
pdfImage.setWidth(imageWidth);
pdfImage.setHeight(imageHeight);
pdfImage.setX(Range.between(
Float.valueOf(imageRect.getUpperRightX()),
Float.valueOf(
imageRect.getUpperRightX() + imageWidth)));
pdfImage.setY(Range.between(
Float.valueOf(imageRect.getUpperRightY()),
Float.valueOf(
imageRect.getUpperRightY() + imageHeight)));
this.images.add(pdfImage);
} else if (xobject instanceof PDFormXObject) {
PDFormXObject form = (PDFormXObject) xobject;
showForm(form);
}
} else {
super.processOperator(operator, operands);
}
}
/**
* Convert {@link PDImageXObject} to byte[].
* @param image {@link PDImageXObject}
* @param imageType {@link String}
* @return byte[]
*/
private byte[] toBytes(final PDImageXObject image, final String imageType) {
ByteArrayOutputStream bytes = new ByteArrayOutputStream();
try {
ImageIO.write(image.getImage(), imageType, bytes);
} catch (IOException e) {
throw new RuntimeException(e);
}
return bytes.toByteArray();
}
/**
* @return {@link List} {@link PdfImage}
*/
public List getImages() {
return this.images;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy