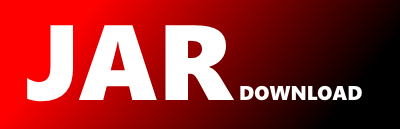
com.formkiq.vision.pdf.PDDocumentToImageTransformer Maven / Gradle / Ivy
/*
* Copyright (C) 2018 FormKiQ Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.formkiq.vision.pdf;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
import org.apache.pdfbox.contentstream.PDFStreamEngine;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.PDPage;
import com.formkiq.vision.document.DocumentImage;
/**
* {@link Function} to convert {@link PDDocument} to {@link PdfImage} by page.
*
*/
public class PDDocumentToImageTransformer extends PDFStreamEngine
implements Function>> {
/** {@link Map} {@link PdfDocumentObjects}. */
private Map tokenMap;
/**
* constructor.
* @param map {@link Map} {@link PdfDocumentObjects}
*/
public PDDocumentToImageTransformer(
final Map map) {
this.tokenMap = map;
}
@Override
public Map> apply(final PDDocument doc) {
Map> map = new HashMap<>();
try {
for (PDPage page : doc.getPages()) {
Integer pageNumber = Integer
.valueOf(doc.getPages().indexOf(page));
ImagePDFStreamEngine i = new ImagePDFStreamEngine(
this.tokenMap.get(pageNumber));
i.processPage(page);
List images = i.getImages().stream().map(s -> s)
.collect(Collectors.toList());
map.put(pageNumber, images);
}
} catch (IOException e) {
throw new RuntimeException(e);
}
return map;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy