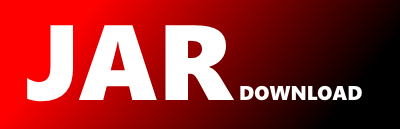
com.formkiq.vision.pdf.PDRectangleUtil Maven / Gradle / Ivy
/*
* Copyright (C) 2018 FormKiQ Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.formkiq.vision.pdf;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
import org.apache.fontbox.util.BoundingBox;
import org.apache.pdfbox.pdmodel.common.PDRectangle;
/**
* {@link PDRectangle} utilities.
*
*/
public final class PDRectangleUtil {
/**
* Do 2 {@link PDRectangle} intersect.
* @param rect1 {@link PDRectangle}
* @param rect2 {@link PDRectangle}
* @return boolean
*/
public static boolean isIntersect(final PDRectangle rect1,
final PDRectangle rect2) {
int l1x = (int) rect1.getLowerLeftX();
int l1y = (int) rect1.getUpperRightY();
int r1x = (int) rect1.getUpperRightX();
int r1y = (int) rect1.getLowerLeftY();
int l2x = (int) rect2.getLowerLeftX();
int l2y = (int) rect2.getUpperRightY();
int r2x = (int) rect2.getUpperRightX();
int r2y = (int) rect2.getLowerLeftY();
// If one rectangle is on left side of other
if (l1x > r2x || l2x > r1x) {
return false;
}
// If one rectangle is above other
if (l1y < r2y || l2y < r1y) {
return false;
}
return true;
}
/**
* Merge r1 into r2.
* @param r1 {@link PDRectangle}
* @param r2 {@link PDRectangle}
*/
public static void mergeInto(final PDRectangle r1, final PDRectangle r2) {
r1.setLowerLeftX(
Math.min(r1.getLowerLeftX(), r2.getLowerLeftX()));
r1.setLowerLeftY(
Math.min(r1.getLowerLeftY(), r2.getLowerLeftY()));
r1.setUpperRightX(
Math.max(r1.getUpperRightX(), r2.getUpperRightX()));
r1.setUpperRightY(
Math.max(r1.getUpperRightY(), r2.getUpperRightY()));
}
/**
* Remove Duplicate {@link PDRectangle}.
*
* @param list {@link List} {@link PDRectangle}
* @param delta int
* @return {@link List} {@link PDRectangle}
*/
public static List removeDuplicates(
final List list, final int delta) {
Set keys = new HashSet<>();
List results = new ArrayList<>(list.size());
for (Iterator i = list.iterator(); i.hasNext();) {
PDRectangle rect = i.next();
Collection removeKeys = generateRemoveKeys(rect, delta);
if (!removeKeys.stream().anyMatch(keys::contains)) {
results.add(rect);
keys.addAll(removeKeys);
}
}
return results;
}
/**
* Generate Remove Keys with delta.
* @param r {@link PDRectangle}
* @param delta int
* @return {@link Collection} of remove Key {@link String}.
*/
private static Collection generateRemoveKeys(final PDRectangle r,
final int delta) {
int x1 = (int) r.getLowerLeftX();
int y1 = (int) r.getLowerLeftY();
int x2 = (int) r.getUpperRightX();
int y2 = (int) r.getUpperRightY();
List list = new ArrayList<>();
for (int i = 0; i < delta; i++) {
list.add((x1 + i) + "_" + y1 + "_" + x2 + "_" + y2);
list.add((x1 + i) + "_" + (y1 + i) + "_" + x2 + "_" + y2);
list.add((x1 + i) + "_" + (y1 + i) + "_" + (x2 + i) + "_" + y2);
list.add((x1 + i) + "_" + (y1 + i) + "_" + (x2 + i) + "_"
+ (y2 + i));
list.add((x1 - i) + "_" + y1 + "_" + x2 + "_" + y2);
list.add((x1 - i) + "_" + (y1 - i) + "_" + x2 + "_" + y2);
list.add((x1 - i) + "_" + (y1 - i) + "_" + (x2 - i) + "_" + y2);
list.add((x1 - i) + "_" + (y1 - i) + "_" + (x2 - i) + "_"
+ (y2 - i));
}
list.add(x1 + "_" + y1 + "_" + x2 + "_" + y2);
return list;
}
/**
* Whether Lines Intersect at a point.
* @param r {@link PDRectangle}
* @param x double
* @param y double
* @return boolean
*/
public static boolean isIntersectionAtPoint(final PDRectangle r,
final double x, final double y) {
double x1 = r.getLowerLeftX();
double y1 = r.getLowerLeftY();
double x2 = r.getUpperRightX();
double y2 = r.getUpperRightY();
return (Math.abs(x1 - x) < 2 && Math.abs(y1 - y) < 2)
|| (Math.abs(x2 - x) < 2 && Math.abs(y1 - y) < 2)
|| (Math.abs(x2 - x) < 2 && Math.abs(y2 - y) < 2)
|| (Math.abs(x1 - x) < 2 && Math.abs(y2 - y) < 2);
}
/**
* Create {@link PDRectangle} from points.
* @param minX float
* @param minY float
* @param maxX float
* @param maxY float
* @return {@link PDRectangle}
*/
public static PDRectangle create(final float minX, final float minY,
final float maxX, final float maxY) {
return new PDRectangle(new BoundingBox(minX, minY, maxX, maxY));
}
/**
* Create {@link PDRectangle} from points.
* @param minX float
* @param minY float
* @param maxX float
* @param maxY float
* @return {@link PDRectangle}
*/
public static PDRectangle create(final double minX, final double minY,
final double maxX, final double maxY) {
return create((float) minX, (float) minY, (float) maxX, (float) maxY);
}
/**
* Create {@link PDRectangle} from points.
* @param minX float
* @param minY float
* @param maxX float
* @param maxY float
* @return {@link PDRectangle}
*/
public static PDRectangle create(final String minX, final String minY,
final String maxX, final String maxY) {
return create(Float.parseFloat(minX), Float.parseFloat(minY),
Float.parseFloat(maxX), Float.parseFloat(maxY));
}
/**
* private constructor.
*/
private PDRectangleUtil() {
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy