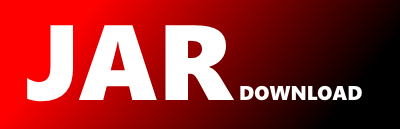
com.formkiq.vision.pdf.PdfText Maven / Gradle / Ivy
/*
* Copyright (C) 2017 FormKiQ Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.formkiq.vision.pdf;
import org.apache.pdfbox.pdmodel.common.PDRectangle;
import com.formkiq.vision.document.DocumentText;
/**
* Holder class for Text Strings in PDF.
*
*/
public class PdfText implements DocumentText {
/** {@link String}. */
private String text;
/** Float Size in PT. */
private float fontSize;
/** Font Name. */
private String fontName;
/** {@link PDRectangle}. */
private PDRectangle rectangle;
/**
* default constructor.
*/
public PdfText() {
}
/**
* @return {@link String}
*/
public String getText() {
return this.text;
}
/**
* @param s {@link String}
*/
public void setText(final String s) {
this.text = s;
}
/**
* @return float
*/
public float getFontSize() {
return this.fontSize;
}
/**
* @param size float
*/
public void setFontSize(final float size) {
this.fontSize = size;
}
@Override
public String toString() {
return "text=" + this.text + ",rectangle=" + this.rectangle
+ ",fontsize=" + this.fontSize;
}
/**
* @return {@link String}
*/
public String getFontName() {
return this.fontName;
}
/**
* @param name {@link String}
*/
public void setFontName(final String name) {
this.fontName = name;
}
@Override
public float getLowerLeftX() {
return this.rectangle.getLowerLeftX();
}
@Override
public float getLowerLeftY() {
return this.rectangle.getLowerLeftY();
}
@Override
public float getUpperRightX() {
return this.rectangle.getUpperRightX();
}
@Override
public float getUpperRightY() {
return this.rectangle.getUpperRightY();
}
/**
* @return {@link PDRectangle}
*/
public PDRectangle getRectangle() {
return this.rectangle;
}
/**
* @param rect {@link PDRectangle}
*/
public void setRectangle(final PDRectangle rect) {
this.rectangle = rect;
}
@Override
public void setUpperRightX(final float x) {
this.rectangle.setUpperRightX(x);
}
@Override
public void setLowerLeftY(final float y) {
this.rectangle.setLowerLeftY(y);
}
@Override
public void setLowerLeftX(final float x) {
this.rectangle.setLowerLeftX(x);
}
@Override
public void setUpperRightY(final float y) {
this.rectangle.setUpperRightY(y);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy