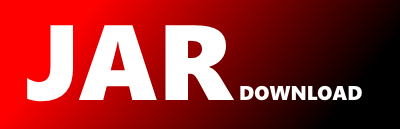
com.formkiq.vision.predicate.DocumentBlockContainsPredicate Maven / Gradle / Ivy
/*
* Copyright (C) 2018 FormKiQ Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.formkiq.vision.predicate;
import java.util.List;
import java.util.function.Predicate;
import org.apache.commons.lang3.Range;
import com.formkiq.vision.document.DocumentBlock;
import com.formkiq.vision.document.DocumentBlockRectangle;
import com.formkiq.vision.util.RangeUtil;
/**
* {@link Predicate} for checking whether the {@link DocumentBlock} is contained
* within the {@link List} of {@link DocumentBlock}.
*
*/
public class DocumentBlockContainsPredicate
implements Predicate {
/** {@link DocumentBlock}. */
private DocumentBlockRectangle block;
/**
* constructor.
* @param docblock {@link DocumentBlockRectangle}
*/
public DocumentBlockContainsPredicate(
final DocumentBlockRectangle docblock) {
this.block = docblock;
}
/**
* constructor.
* @param docblock {@link DocumentBlockRectangle}
* @param delta int
*/
public DocumentBlockContainsPredicate(final DocumentBlockRectangle docblock,
final int delta) {
this(new DocumentBlock(docblock.getLowerLeftX() - delta,
docblock.getLowerLeftY() - delta,
docblock.getUpperRightX() + delta,
docblock.getUpperRightY() + delta));
}
@Override
public boolean test(final DocumentBlockRectangle t) {
return contains(this.block, t);
}
/**
* Whether {@link DocumentBlockRectangle} contains another
* {@link DocumentBlockRectangle}.
*
* @param parent {@link DocumentBlockRectangle}
* @param child {@link DocumentBlockRectangle}
* @param delta int
* @return boolean
*/
public static boolean contains(final DocumentBlockRectangle parent,
final DocumentBlockRectangle child, final int delta) {
return contains(new DocumentBlock(parent.getLowerLeftX() - delta,
parent.getLowerLeftY() - delta, parent.getUpperRightX() + delta,
parent.getUpperRightY() + delta), child);
}
/**
* Whether {@link DocumentBlockRectangle} contains another
* {@link DocumentBlockRectangle}.
*
* @param parent {@link DocumentBlockRectangle}
* @param child {@link DocumentBlockRectangle}
* @return boolean
*/
public static boolean contains(final DocumentBlockRectangle parent,
final DocumentBlockRectangle child) {
return parent != null && child != null
&& (int) parent.getLowerLeftX() <= (int) child.getLowerLeftX()
&& (int) parent.getUpperRightX() >= (int) child.getUpperRightX()
&& (int) parent.getLowerLeftY() <= (int) child.getLowerLeftY()
&& (int) parent.getUpperRightY() >= (int) child
.getUpperRightY();
}
/**
* Whether {@link DocumentBlockRectangle} overlaps with each other.
* @param r0 {@link DocumentBlockRectangle}
* @param r1 {@link DocumentBlockRectangle}
* @return boolean
*/
public static boolean overlaps(final DocumentBlockRectangle r0,
final DocumentBlockRectangle r1) {
Range x0 = RangeUtil.between(r0.getLowerLeftX(), r0.getUpperRightX());
Range y0 = RangeUtil.between(r0.getLowerLeftY(), r0.getUpperRightY());
Range x1 = RangeUtil.between(r1.getLowerLeftX(), r1.getUpperRightX());
Range y1 = RangeUtil.between(r1.getLowerLeftY(), r1.getUpperRightY());
return x0.isOverlappedBy(x1) && y0.isOverlappedBy(y1);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy