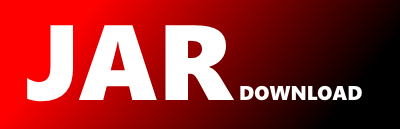
com.formkiq.vision.util.RangeUtil Maven / Gradle / Ivy
/*
* Copyright (C) 2018 FormKiQ Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.formkiq.vision.util;
import java.util.List;
import java.util.Optional;
import org.apache.commons.lang3.Range;
/**
* Helper class for {@link Range}.
*
*/
public final class RangeUtil {
/**
* private constructor.
*/
private RangeUtil() {
}
/**
* Minimum value in {@link Range}.
* @param range {@link Range} {@link List}
* @return float
*/
public static float min(final List> range) {
return range.stream().map(s -> s.getMinimum()).min(Float::compareTo)
.get().floatValue();
}
/**
* Maximum value in {@link Range}.
* @param range {@link Range} {@link List}
* @return float
*/
public static float max(final List> range) {
return range.stream().map(s -> s.getMaximum()).max(Float::compareTo)
.get().floatValue();
}
/**
* Find Overlapping Range.
* @param range {@link Range}
* @param ranges {@link List} {@link Range}
* @return {@link Range} {@link Float}
*/
public static Optional> findRange(final Range range,
final List> ranges) {
return ranges.stream().filter(r->r.isOverlappedBy(range)).findFirst();
}
/**
* Merge {@link Range}.
* @param r1 {@link Range}
* @param r2 {@link Range}
* @return {@link Range}
*/
public static Range merge(final Range r1,
final Range r2) {
return Range.between(
Float.valueOf(Math.min(r1.getMinimum().floatValue(),
r2.getMinimum().floatValue())),
Float.valueOf(Math.max(r1.getMaximum().floatValue(),
r2.getMaximum().floatValue())));
}
/**
* Change {@link Range} by delta.
* @param range {@link Range} {@link Float}
* @param lowerdelta int
* @param upperdelta int
* @return {@link Range}
*/
public static Range change(final Range range,
final int lowerdelta, final int upperdelta) {
return Range.between(
Float.valueOf(range.getMinimum().floatValue() + lowerdelta),
Float.valueOf(range.getMaximum().floatValue() + upperdelta));
}
/**
* Build {@link Range}.
* @param min float
* @param max float
* @return {@link Range}
*/
public static Range between(final float min, final float max) {
return Range.between(Float.valueOf(min), Float.valueOf(max));
}
/**
* Calculate height of {@link Range}.
* @param range {@link Range}
* @return float
*/
public static float getHeight(final Range range) {
return range.getMaximum().floatValue() - range.getMinimum().floatValue();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy