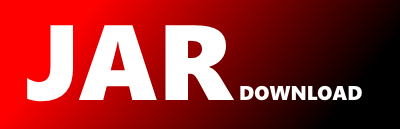
com.fortify.plugin.api.BasicVulnerabilityBuilder Maven / Gradle / Ivy
/*
* (c) Copyright 2017 Micro Focus or one of its affiliates.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.fortify.plugin.api;
import com.fortify.plugin.spi.VulnerabilityAttribute;
import java.math.BigDecimal;
import java.util.Date;
/**
* Interface that allows parser plugins to build vulnerabilities with basic set of attributes.
* Almost all methods are optional here except {@link #completeVulnerability()} that must be called when all vulnerability
* attributes are set.
*
* This is a base interface. Interfaces of the specific vulnerability builders (e.g. for static or dynamic vulnerabilities)
* must be inherited from this interface.
*
* In addition to predefined vulnerability attributes like impact, severity, priority and so on, parser plugins are allowed
* to define their own custom attributes that do not exist in SSC data model.
* First step to define custom attributes is implementing {@link com.fortify.plugin.spi.VulnerabilityAttribute} and defining
* enumeration of the attributes that plugin supports.
* Once attributes are defined, values of these attributes can be set by parser plugin by calling following methods
* of this interface:
*
* - {@link #setDecimalCustomAttributeValue(VulnerabilityAttribute vulnerabilityAttribute, BigDecimal attributeValue)}
*
- {@link #setStringCustomAttributeValue(VulnerabilityAttribute vulnerabilityAttribute, String attributeValue)}
*
- {@link #setDateCustomAttributeValue(VulnerabilityAttribute vulnerabilityAttribute, Date attributeValue)}
*
* These methods should be used for setting custom attribute values of different types. See documentation of these
* methods to get extra details.
*
* This builder does not really follow the GOF builder pattern. Build result is not returned to the client,
* but passed to the builder implementation to handle the build result (Vulnerability instance).
*
* @param Specific type of the builder.
*/
public interface BasicVulnerabilityBuilder> {
/**
* Vulnerability priority enumeration.
*/
enum Priority {
/**
* Highest level of the priority. Vulnerability marked by this priority should be reviewed / fixed ASAP.
*/
Critical,
/**
* High priority. Indicates that vulnerability is quite serious, but not critical.
*/
High,
/**
* Medium priority. Indicates that vulnerabilities are not serious and most probably cannot be used to break
* the application where they were found. Some code quality problems can be marked by medium priority.
*/
Medium,
/**
* Low priority. Is is not nice to have such vulnerabilities in the application, but it is not risky to
* deploy the application with such kinds of vulnerabilities.
*/
Low
}
/**
* Method must be called when all the attributes are set by plugin and vulnerability is ready to be processed by SSC.
* Builder is one time object that can be used for building one vulnerability.
* It is not allowed to call this function twice for the same builder instance. Once this function is called,
* build process for one vulnerability is completed and new vulnerability can be started to be built.
*/
void completeVulnerability();
/* *********************************
* General vulnerability attributes
********************************* */
/**
* Set vulnerability accuracy value. This value can be provided by analyser in scan result file or this value
* can be calculated by parser plugin using some other vulnerability attributes.
* Value of this attribute is mandatory. If analyser does not provide such value, it is recommended to set highest
* accuracy value 5.0.
* @param accuracy level of confidence that vulnerability is really vulnerability. Can take values from 1.0 to 5.0.
* 1.0 means lowest accuracy level. 5.0 means highest accuracy level.
* @return reference to this builder instance.
*/
T setAccuracy(Float accuracy);
/**
* Set type of the analyser that found this vulnerability.
* Full list of the analysers supported by Fortify can be found here {@link com.fortify.plugin.api.FortifyAnalyser}.
* Parsers can provide custom analyser name if there is no suitable analyser among the fortify analysers.
* Value of this attribute is mandatory.
* @param analyzer name of the analyser that found the vulnerability.
* @return reference to this builder instance.
*/
T setAnalyzer(String analyzer);
/**
* Set vulnerability's engine type. Engine type is very important attribute that is used by SSC to distinguish
* vulnerabilities produced by different parsers from each other. Engine type value defined in plugin.xml file
* for parser plugin and value of this attribute must be the same.
* This value must be unique across all the installed plugins.
* Value of this attribute is mandatory.
* @param engineType vulnerability's engine type.
* @return reference to this builder instance.
*/
T setEngineType(String engineType);
/**
* Set confidence for vulnerability.
* @param confidence level of confidence provided by analyser that vulnerability is true positive vulnerability
* Value must be between 1 and 5 (inclusive). 1 means lowest confidence, 5 means highest confidence.
* Value of this attribute is mandatory. If analyser does not provide such value, it is recommended to set
* average confidence value 2.5.
* @return reference to this builder instance.
*/
T setConfidence(Float confidence);
/**
* Set vulnerability priority.
* @param priority priority value. See documentation for {@link com.fortify.plugin.api.BasicVulnerabilityBuilder.Priority}
* enumeration.
* Value of this attribute is mandatory. If analyser does not provide such value, it is recommended to the parser
* developers to introduce a logic that calculates this values.
* @return reference to this builder instance.
*/
T setPriority(Priority priority);
/**
* Set the impact level of this vulnerability to the application where it was found.
* @param impact how seriously the application that contains this vulnerability will be affected if this vulnerability
* is exploited. Value must be between 1 and 5 (inclusive). Low impact means that application either is not
* be affected or consequences is quite minor. High impact indicates that consequences might be quite serious.
* Value of this attribute is mandatory. If analyser does not provide such value, it is recommended to set
* lowest, highest or some average value of this attribute depending on the type of vulnerability.
* @return reference to this builder instance.
*/
T setImpact(Float impact);
/**
* Set likelihood value.
* @param likelihood is the probability that the impact {@link com.fortify.plugin.api.BasicVulnerabilityBuilder#setImpact(Float)} will come to pass.
* Value must be between 0 and 5.0 (inclusive). 0 means lowest likelihood, 5.0 means highest likelihood.
* Value of this attribute is mandatory. If analyser does not provide such value, it is recommended to set it
* to average value 2.5.
* @return reference to this builder instance.
*/
T setLikelihood(Float likelihood);
/**
* Set vulnerability probability value.
* @param probability measures the possibility that the vulnerability will actually be exploited.
* Value must be between 0 and 5 (inclusive). 0 means lowest probability, 5 means highest probability.
* Value of this attribute is mandatory. If analyser does not provide such value, it is recommended to set it
* to average value 2.5.
* @return reference to this builder instance.
*/
T setProbability(Float probability);
/**
* Set vulnerability severity value.
* @param severity severity of the vulnerability (in scale of 1 to 5).
* Value of this attribute is mandatory. If analyser does not provide such value, it is recommended to set
* severity value to 2.5.
* @return reference to this builder instance.
* @deprecated priority should be used instead.
*/
@Deprecated
T setSeverity(Float severity);
/**
* Set primary category of the vulnerability.
* For example, Cross-Site Scripting is a primary vulnerability category that can be split into several sub categories:
* Poor Validation, External Links and so on.
* Value of this attribute is mandatory.
* @param category primary category of the vulnerability.
* @return reference to this builder instance.
*/
T setCategory(String category);
/**
* Set sub primary category of the vulnerability.
* For example, Cross-Site Scripting is a primary vulnerability category that can be split into several sub categories:
* Poor Validation, External Links and so on.
* Value of this attribute is optional if primary category does not have any sub categories.
* @param subCategory sub category of the vulnerability.
* @return reference to this builder instance.
*/
T setSubCategory(String subCategory);
/**
* Set name of the Fortify category of the vulnerability.
* Value of this attribute is optional for non Fortify parsers.>.
* SSC keeps full mapping between Fortify categories and external vulnerability categories like OWASP, CWE, FISMA,
* PCI, etc.
* If this value is provided by parser, SSC is able to map this vulnerability to external categories defined on SSC side.
* If value of this attribute is not provided, vulnerability will be processed and displayed correctly, but mapping
* to external categories will not be done.
* @param mappedCategory name of the Fortify category of the vulnerability
* @return reference to this builder instance.
*/
T setMappedCategory(String mappedCategory);
/**
* General information about vulnerability: why analyser "thinks" that this is vulnerability, some general information
* about this type of vulnerability, how can it be exploited and so on.
* Value of this attribute is optional for 3rd party parsers, but it is recommended to set it since it simplifies
* vulnerabilities audit.
* Fortify parsers can set value of this attribute to null if SSC hosts rulepacks specific to a fortify analyser that
* produced this vulnerability and the rule GUID (@link BasicVulnerabilityBuilder.setRuleGuid(String)) is passed.
* @param vulnerabilityAbstract vulnerability abstract value.
* @return reference to this builder instance.
*/
T setVulnerabilityAbstract(String vulnerabilityAbstract);
/**
* Basic information about how this vulnerability can be fixed by developers.
* Value of this attribute is optional for 3rd party parsers, but it is recommended to set it since it simplifies
* vulnerabilities audit.
* Fortify parsers can set value of this attribute to null if SSC hosts rulepacks specific to a fortify analyser that
* produced this vulnerability and the rule GUID (@link BasicVulnerabilityBuilder.setRuleGuid(String)) is passed.
* @param vulnerabilityRecommendation vulnerability recommendation value.
* @return reference to this builder instance.
*/
T setVulnerabilityRecommendation(String vulnerabilityRecommendation);
/**
* Set kingdom for vulnerability. In contrast to category {@link com.fortify.plugin.api.BasicVulnerabilityBuilder#setCategory(String)} that defines
* exact type of vulnerability, kingdom defines a general type of vulnerability.
* @param kingdom fortify specific attribute that defines a general type of vulnerability.
* List of all supported kingdoms can be found here {@link com.fortify.plugin.api.FortifyKingdom}.
* it is recommended to use this enum to get names of the kingdoms to provide them to this function.
* @return reference to this builder instance.
*/
T setKingdom(String kingdom);
/**
* Set GUID of the rule that was used for detecting this vulnerability.
* This value is very specific and it makes sense to set it only if SSC hosts rulepacks supported by analyser
* produces such types of the vulnerability.
* Value of this attribute is optional if SSC does not host analyser specific rule packs. It is currently
* implemented only for fortify parsers. 3rd party parsers can set value of this attribute to null.
* If value of this attribute is set, SSC uses it to get full information about the rule to get vulnerability abstract,
* recommendations and some other rule specific attributes when parsed issue is displayed on UI.
* @param ruleGuid GUID of the rule that was used for detecting this vulnerability.
* @return reference to this builder instance.
*/
T setRuleGuid(String ruleGuid);
/**
* Set value of decimal custom attribute. See explanation of what custom attributes means in the class documentation section.
* @param vulnerabilityAttribute attribute which value is set. null
value of this parameter is not accepted.
* @param attributeValue value of attribute. null
values can be passed.
* If value is not null, max allowed precision of the value must be {@link VulnerabilityAttribute#MAX_DECIMAL_PRECISION},
* max allowed scale must be {@link VulnerabilityAttribute#MAX_DECIMAL_SCALE}.
* Parser should not call this method if it does not produce any custom attributes of decimal type.
* @return reference to this builder instance.
* @throws IllegalArgumentException if vulnerabilityAttribute is not set, or if attributeValue did not pass validation.
*/
T setDecimalCustomAttributeValue(VulnerabilityAttribute vulnerabilityAttribute, BigDecimal attributeValue);
/**
* Set value of STRING or LONG_STRING custom attribute.
* @param vulnerabilityAttribute attribute which value is set. null
value of this parameter is not accepted.
* @param attributeValue value of attribute. null
values can be passed.
* Max allowed length is {@link VulnerabilityAttribute#MAX_STRING_LENGTH} characters for STRING values
* and {@link VulnerabilityAttribute#MAX_LONG_STRING_LENGTH} characters for LONG_STRING values.
* Parser should not call this method if it does not produce any custom attributes of string type.
* @return reference to this builder instance.
* @throws IllegalArgumentException if vulnerabilityAttribute is not set, or if attributeValue did not pass validation.
*/
T setStringCustomAttributeValue(VulnerabilityAttribute vulnerabilityAttribute, String attributeValue);
/**
* Set value of DATE custom attribute.
* @param vulnerabilityAttribute attribute which value is set. null
value of this parameter is not accepted.
* @param attributeValue value of attribute. null
values can be passed.
* Time part of Date value will be ignored when value is stored.
* Parser should not call this method if it does not produce any custom attributes of date type.
* @return reference to this builder instance.
* @throws IllegalArgumentException if vulnerabilityAttribute is not set, or if attributeValue did not pass validation.
*/
T setDateCustomAttributeValue(VulnerabilityAttribute vulnerabilityAttribute, Date attributeValue);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy