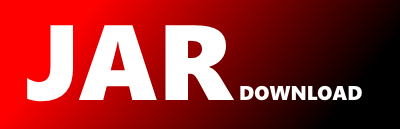
org.postgresql.core.v3.SimpleQuery Maven / Gradle / Ivy
/*-------------------------------------------------------------------------
*
* Copyright (c) 2004-2011, PostgreSQL Global Development Group
* Copyright (c) 2004, Open Cloud Limited.
*
*
*-------------------------------------------------------------------------
*/
package org.postgresql.core.v3;
import org.postgresql.core.*;
import java.lang.ref.PhantomReference;
/**
* V3 Query implementation for a single-statement query.
* This also holds the state of any associated server-side
* named statement. We use a PhantomReference managed by
* the QueryExecutor to handle statement cleanup.
*
* @author Oliver Jowett ([email protected])
*/
class SimpleQuery implements V3Query {
SimpleQuery(String[] fragments, ProtocolConnectionImpl protoConnection)
{
this.fragments = fragments;
this.protoConnection = protoConnection;
}
public ParameterList createParameterList() {
if (fragments.length == 1)
return NO_PARAMETERS;
return new SimpleParameterList(fragments.length - 1, protoConnection);
}
public String toString(ParameterList parameters) {
StringBuffer sbuf = new StringBuffer(fragments[0]);
for (int i = 1; i < fragments.length; ++i)
{
if (parameters == null)
sbuf.append('?');
else
sbuf.append(parameters.toString(i));
sbuf.append(fragments[i]);
}
return sbuf.toString();
}
public String toString() {
return toString(null);
}
public void close() {
unprepare();
}
//
// V3Query
//
public SimpleQuery[] getSubqueries() {
return null;
}
//
// Implementation guts
//
String[] getFragments() {
return fragments;
}
void setStatementName(String statementName) {
this.statementName = statementName;
this.encodedStatementName = Utils.encodeUTF8(statementName);
}
void setStatementTypes(int[] paramTypes) {
this.preparedTypes = paramTypes;
}
int[] getStatementTypes() {
return preparedTypes;
}
String getStatementName() {
return statementName;
}
boolean isPreparedFor(int[] paramTypes) {
if (statementName == null)
return false; // Not prepared.
// Check for compatible types.
for (int i = 0; i < paramTypes.length; ++i)
if (paramTypes[i] != Oid.UNSPECIFIED && paramTypes[i] != preparedTypes[i])
return false;
return true;
}
boolean hasUnresolvedTypes() {
if (preparedTypes == null)
return true;
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy