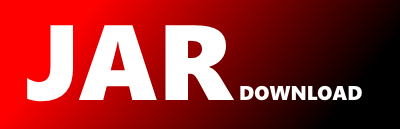
com.foursoft.vecmodel.vec113.VecContent Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.0
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2021.08.10 at 09:49:21 AM UTC
//
package com.foursoft.vecmodel.vec113;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlIDREF;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
import com.foursoft.vecmodel.vec113.visitor.Visitable;
import com.foursoft.vecmodel.vec113.visitor.Visitor;
import com.foursoft.xml.annotations.XmlBackReference;
/**
* The VecContent is the XML-Root node for any VEC-Document.
*
* Java class for VecContent complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="VecContent">
* <complexContent>
* <extension base="{http://www.prostep.org/ecad-if/2011/vec}ExtendableElement">
* <sequence>
* <element name="VecVersion" type="{http://www.prostep.org/ecad-if/2011/vec}VecVersion"/>
* <element name="GeneratingSystemName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="DateOfCreation" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="GeneratingSystemVersion" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="StandardCopyrightInformation" type="{http://www.w3.org/2001/XMLSchema}IDREF" minOccurs="0"/>
* <element name="CompliantConformanceClass" type="{http://www.prostep.org/ecad-if/2011/vec}ConformanceClass" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Contract" type="{http://www.prostep.org/ecad-if/2011/vec}Contract" maxOccurs="unbounded" minOccurs="0"/>
* <element name="CopyrightInformation" type="{http://www.prostep.org/ecad-if/2011/vec}CopyrightInformation" maxOccurs="unbounded" minOccurs="0"/>
* <element name="DocumentVersion" type="{http://www.prostep.org/ecad-if/2011/vec}DocumentVersion" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ItemHistoryEntry" type="{http://www.prostep.org/ecad-if/2011/vec}ItemHistoryEntry" maxOccurs="unbounded" minOccurs="0"/>
* <element name="PartVersion" type="{http://www.prostep.org/ecad-if/2011/vec}PartVersion" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Project" type="{http://www.prostep.org/ecad-if/2011/vec}Project" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Unit" type="{http://www.prostep.org/ecad-if/2011/vec}Unit" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "VecContent", propOrder = {
"vecVersion",
"generatingSystemName",
"dateOfCreation",
"generatingSystemVersion",
"standardCopyrightInformation",
"compliantConformanceClasses",
"contracts",
"copyrightInformations",
"documentVersions",
"itemHistoryEntries",
"partVersions",
"projects",
"units"
})
@XmlRootElement(name = "VecContent")
public class VecContent
extends VecExtendableElement
implements Serializable, Visitable
{
private final static long serialVersionUID = 1L;
/**
* Specifies the version of the VEC used for the file.
*
*/
@XmlElement(name = "VecVersion", required = true)
protected String vecVersion;
/**
* Specifies the name of the system that has generated the VEC-file.
*
*/
@XmlElement(name = "GeneratingSystemName")
protected String generatingSystemName;
/**
* Specifies the date of creation of the VEC-file.
*
*/
@XmlElement(name = "DateOfCreation")
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar dateOfCreation;
/**
* Specifies the version of the system that has generated the VEC-file.
*
*/
@XmlElement(name = "GeneratingSystemVersion")
protected String generatingSystemVersion;
/**
* References theCopyrightInformationthat is in effect for the complete content of thisVecContent. It is applied to allItemVersionsthat do not references their own individualCopyrightInformation.
*
*
*/
@XmlElement(name = "StandardCopyrightInformation", type = java.lang.Object.class)
@XmlIDREF
@XmlSchemaType(name = "IDREF")
@XmlBackReference(destinationField = "refVecContent")
protected VecCopyrightInformation standardCopyrightInformation;
@XmlElement(name = "CompliantConformanceClass")
protected List compliantConformanceClasses;
@XmlElement(name = "Contract")
protected List contracts;
@XmlElement(name = "CopyrightInformation")
protected List copyrightInformations;
@XmlElement(name = "DocumentVersion")
protected List documentVersions;
@XmlElement(name = "ItemHistoryEntry")
protected List itemHistoryEntries;
@XmlElement(name = "PartVersion")
protected List partVersions;
@XmlElement(name = "Project")
protected List projects;
@XmlElement(name = "Unit")
protected List units;
/**
* Gets the value of the vecVersion property.
*
*
Specifies the version of the VEC used for the file.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVecVersion() {
return vecVersion;
}
/**
* Sets the value of the vecVersion property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getVecVersion()
*/
public void setVecVersion(String value) {
this.vecVersion = value;
}
/**
* Gets the value of the generatingSystemName property.
*
*
Specifies the name of the system that has generated the VEC-file.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGeneratingSystemName() {
return generatingSystemName;
}
/**
* Sets the value of the generatingSystemName property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getGeneratingSystemName()
*/
public void setGeneratingSystemName(String value) {
this.generatingSystemName = value;
}
/**
* Gets the value of the dateOfCreation property.
*
*
Specifies the date of creation of the VEC-file.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getDateOfCreation() {
return dateOfCreation;
}
/**
* Sets the value of the dateOfCreation property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
* @see #getDateOfCreation()
*/
public void setDateOfCreation(XMLGregorianCalendar value) {
this.dateOfCreation = value;
}
/**
* Gets the value of the generatingSystemVersion property.
*
*
Specifies the version of the system that has generated the VEC-file.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGeneratingSystemVersion() {
return generatingSystemVersion;
}
/**
* Sets the value of the generatingSystemVersion property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getGeneratingSystemVersion()
*/
public void setGeneratingSystemVersion(String value) {
this.generatingSystemVersion = value;
}
/**
* Gets the value of the standardCopyrightInformation property.
*
*
References theCopyrightInformationthat is in effect for the complete content of thisVecContent. It is applied to allItemVersionsthat do not references their own individualCopyrightInformation.
*
*
* @return
* possible object is
* {@link Object }
*
*/
public VecCopyrightInformation getStandardCopyrightInformation() {
return standardCopyrightInformation;
}
/**
* Sets the value of the standardCopyrightInformation property.
*
* @param value
* allowed object is
* {@link Object }
*
* @see #getStandardCopyrightInformation()
*/
public void setStandardCopyrightInformation(VecCopyrightInformation value) {
this.standardCopyrightInformation = value;
}
/**
* Gets the value of the compliantConformanceClasses property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the compliantConformanceClasses property.
*
*
* For example, to add a new item, do as follows:
*
* getCompliantConformanceClasses().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VecConformanceClass }
*
*
*/
public List getCompliantConformanceClasses() {
if (compliantConformanceClasses == null) {
compliantConformanceClasses = new ArrayList();
}
return this.compliantConformanceClasses;
}
/**
* Gets the value of the contracts property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the contracts property.
*
*
* For example, to add a new item, do as follows:
*
* getContracts().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VecContract }
*
*
*/
public List getContracts() {
if (contracts == null) {
contracts = new ArrayList();
}
return this.contracts;
}
/**
* Gets the value of the copyrightInformations property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the copyrightInformations property.
*
*
* For example, to add a new item, do as follows:
*
* getCopyrightInformations().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VecCopyrightInformation }
*
*
*/
public List getCopyrightInformations() {
if (copyrightInformations == null) {
copyrightInformations = new ArrayList();
}
return this.copyrightInformations;
}
/**
* Gets the value of the documentVersions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the documentVersions property.
*
*
* For example, to add a new item, do as follows:
*
* getDocumentVersions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VecDocumentVersion }
*
*
*/
public List getDocumentVersions() {
if (documentVersions == null) {
documentVersions = new ArrayList();
}
return this.documentVersions;
}
/**
* Gets the value of the itemHistoryEntries property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the itemHistoryEntries property.
*
*
* For example, to add a new item, do as follows:
*
* getItemHistoryEntries().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VecItemHistoryEntry }
*
*
*/
public List getItemHistoryEntries() {
if (itemHistoryEntries == null) {
itemHistoryEntries = new ArrayList();
}
return this.itemHistoryEntries;
}
/**
* Gets the value of the partVersions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the partVersions property.
*
*
* For example, to add a new item, do as follows:
*
* getPartVersions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VecPartVersion }
*
*
*/
public List getPartVersions() {
if (partVersions == null) {
partVersions = new ArrayList();
}
return this.partVersions;
}
/**
* Gets the value of the projects property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the projects property.
*
*
* For example, to add a new item, do as follows:
*
* getProjects().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VecProject }
*
*
*/
public List getProjects() {
if (projects == null) {
projects = new ArrayList();
}
return this.projects;
}
/**
* Gets the value of the units property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the units property.
*
*
* For example, to add a new item, do as follows:
*
* getUnits().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link VecUnit }
*
*
*/
public List getUnits() {
if (units == null) {
units = new ArrayList();
}
return this.units;
}
publicR accept(Visitor aVisitor)
throws E
{
return aVisitor.visitVecContent(this);
}
}