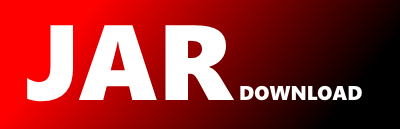
com.foursoft.vecmodel.vec120.AbstractVecApplicationConstraintAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecApplicationConstraint} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecApplicationConstraintAssert, A extends VecApplicationConstraint> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecApplicationConstraintAssert}
to make assertions on actual VecApplicationConstraint.
* @param actual the VecApplicationConstraint we want to make assertions on.
*/
protected AbstractVecApplicationConstraintAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecApplicationConstraint's baseInclusion is equal to the given one.
* @param baseInclusion the given baseInclusion to compare the actual VecApplicationConstraint's baseInclusion to.
* @return this assertion object.
* @throws AssertionError - if the actual VecApplicationConstraint's baseInclusion is not equal to the given one.
*/
public S hasBaseInclusion(VecApplicationConstraint baseInclusion) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting baseInclusion of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecApplicationConstraint actualBaseInclusion = actual.getBaseInclusion();
if (!Objects.areEqual(actualBaseInclusion, baseInclusion)) {
failWithMessage(assertjErrorMessage, actual, baseInclusion, actualBaseInclusion);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's fromDate is equal to the given one.
* @param fromDate the given fromDate to compare the actual VecApplicationConstraint's fromDate to.
* @return this assertion object.
* @throws AssertionError - if the actual VecApplicationConstraint's fromDate is not equal to the given one.
*/
public S hasFromDate(javax.xml.datatype.XMLGregorianCalendar fromDate) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting fromDate of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
javax.xml.datatype.XMLGregorianCalendar actualFromDate = actual.getFromDate();
if (!Objects.areEqual(actualFromDate, fromDate)) {
failWithMessage(assertjErrorMessage, actual, fromDate, actualFromDate);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's fromSerialNumber is equal to the given one.
* @param fromSerialNumber the given fromSerialNumber to compare the actual VecApplicationConstraint's fromSerialNumber to.
* @return this assertion object.
* @throws AssertionError - if the actual VecApplicationConstraint's fromSerialNumber is not equal to the given one.
*/
public S hasFromSerialNumber(String fromSerialNumber) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting fromSerialNumber of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualFromSerialNumber = actual.getFromSerialNumber();
if (!Objects.areEqual(actualFromSerialNumber, fromSerialNumber)) {
failWithMessage(assertjErrorMessage, actual, fromSerialNumber, actualFromSerialNumber);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's parentApplicationConstraintSpecification is equal to the given one.
* @param parentApplicationConstraintSpecification the given parentApplicationConstraintSpecification to compare the actual VecApplicationConstraint's parentApplicationConstraintSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecApplicationConstraint's parentApplicationConstraintSpecification is not equal to the given one.
*/
public S hasParentApplicationConstraintSpecification(VecApplicationConstraintSpecification parentApplicationConstraintSpecification) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentApplicationConstraintSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecApplicationConstraintSpecification actualParentApplicationConstraintSpecification = actual.getParentApplicationConstraintSpecification();
if (!Objects.areEqual(actualParentApplicationConstraintSpecification, parentApplicationConstraintSpecification)) {
failWithMessage(assertjErrorMessage, actual, parentApplicationConstraintSpecification, actualParentApplicationConstraintSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's project contains the given VecProject elements.
* @param project the given elements that should be contained in actual VecApplicationConstraint's project.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's project does not contain all given VecProject elements.
*/
public S hasProject(VecProject... project) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecProject varargs is not null.
if (project == null) failWithMessage("Expecting project parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getProject(), project);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's project contains the given VecProject elements in Collection.
* @param project the given elements that should be contained in actual VecApplicationConstraint's project.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's project does not contain all given VecProject elements.
*/
public S hasProject(java.util.Collection extends VecProject> project) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecProject collection is not null.
if (project == null) {
failWithMessage("Expecting project parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getProject(), project.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's project contains only the given VecProject elements and nothing else in whatever order.
* @param project the given elements that should be contained in actual VecApplicationConstraint's project.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's project does not contain all given VecProject elements.
*/
public S hasOnlyProject(VecProject... project) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecProject varargs is not null.
if (project == null) failWithMessage("Expecting project parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getProject(), project);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's project contains only the given VecProject elements in Collection and nothing else in whatever order.
* @param project the given elements that should be contained in actual VecApplicationConstraint's project.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's project does not contain all given VecProject elements.
*/
public S hasOnlyProject(java.util.Collection extends VecProject> project) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecProject collection is not null.
if (project == null) {
failWithMessage("Expecting project parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getProject(), project.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's project does not contain the given VecProject elements.
*
* @param project the given elements that should not be in actual VecApplicationConstraint's project.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's project contains any given VecProject elements.
*/
public S doesNotHaveProject(VecProject... project) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecProject varargs is not null.
if (project == null) failWithMessage("Expecting project parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getProject(), project);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's project does not contain the given VecProject elements in Collection.
*
* @param project the given elements that should not be in actual VecApplicationConstraint's project.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's project contains any given VecProject elements.
*/
public S doesNotHaveProject(java.util.Collection extends VecProject> project) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecProject collection is not null.
if (project == null) {
failWithMessage("Expecting project parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getProject(), project.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint has no project.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's project is not empty.
*/
public S hasNoProject() {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have project but had :\n <%s>";
// check
if (actual.getProject().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getProject());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's projectPhases contains the given String elements.
* @param projectPhases the given elements that should be contained in actual VecApplicationConstraint's projectPhases.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's projectPhases does not contain all given String elements.
*/
public S hasProjectPhases(String... projectPhases) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given String varargs is not null.
if (projectPhases == null) failWithMessage("Expecting projectPhases parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getProjectPhases(), projectPhases);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's projectPhases contains the given String elements in Collection.
* @param projectPhases the given elements that should be contained in actual VecApplicationConstraint's projectPhases.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's projectPhases does not contain all given String elements.
*/
public S hasProjectPhases(java.util.Collection extends String> projectPhases) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given String collection is not null.
if (projectPhases == null) {
failWithMessage("Expecting projectPhases parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getProjectPhases(), projectPhases.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's projectPhases contains only the given String elements and nothing else in whatever order.
* @param projectPhases the given elements that should be contained in actual VecApplicationConstraint's projectPhases.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's projectPhases does not contain all given String elements.
*/
public S hasOnlyProjectPhases(String... projectPhases) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given String varargs is not null.
if (projectPhases == null) failWithMessage("Expecting projectPhases parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getProjectPhases(), projectPhases);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's projectPhases contains only the given String elements in Collection and nothing else in whatever order.
* @param projectPhases the given elements that should be contained in actual VecApplicationConstraint's projectPhases.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's projectPhases does not contain all given String elements.
*/
public S hasOnlyProjectPhases(java.util.Collection extends String> projectPhases) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given String collection is not null.
if (projectPhases == null) {
failWithMessage("Expecting projectPhases parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getProjectPhases(), projectPhases.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's projectPhases does not contain the given String elements.
*
* @param projectPhases the given elements that should not be in actual VecApplicationConstraint's projectPhases.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's projectPhases contains any given String elements.
*/
public S doesNotHaveProjectPhases(String... projectPhases) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given String varargs is not null.
if (projectPhases == null) failWithMessage("Expecting projectPhases parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getProjectPhases(), projectPhases);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's projectPhases does not contain the given String elements in Collection.
*
* @param projectPhases the given elements that should not be in actual VecApplicationConstraint's projectPhases.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's projectPhases contains any given String elements.
*/
public S doesNotHaveProjectPhases(java.util.Collection extends String> projectPhases) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given String collection is not null.
if (projectPhases == null) {
failWithMessage("Expecting projectPhases parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getProjectPhases(), projectPhases.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint has no projectPhases.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's projectPhases is not empty.
*/
public S hasNoProjectPhases() {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have projectPhases but had :\n <%s>";
// check
if (actual.getProjectPhases().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getProjectPhases());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refApplicationConstraint contains the given VecApplicationConstraint elements.
* @param refApplicationConstraint the given elements that should be contained in actual VecApplicationConstraint's refApplicationConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refApplicationConstraint does not contain all given VecApplicationConstraint elements.
*/
public S hasRefApplicationConstraint(VecApplicationConstraint... refApplicationConstraint) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecApplicationConstraint varargs is not null.
if (refApplicationConstraint == null) failWithMessage("Expecting refApplicationConstraint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefApplicationConstraint(), refApplicationConstraint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refApplicationConstraint contains the given VecApplicationConstraint elements in Collection.
* @param refApplicationConstraint the given elements that should be contained in actual VecApplicationConstraint's refApplicationConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refApplicationConstraint does not contain all given VecApplicationConstraint elements.
*/
public S hasRefApplicationConstraint(java.util.Collection extends VecApplicationConstraint> refApplicationConstraint) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecApplicationConstraint collection is not null.
if (refApplicationConstraint == null) {
failWithMessage("Expecting refApplicationConstraint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefApplicationConstraint(), refApplicationConstraint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refApplicationConstraint contains only the given VecApplicationConstraint elements and nothing else in whatever order.
* @param refApplicationConstraint the given elements that should be contained in actual VecApplicationConstraint's refApplicationConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refApplicationConstraint does not contain all given VecApplicationConstraint elements.
*/
public S hasOnlyRefApplicationConstraint(VecApplicationConstraint... refApplicationConstraint) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecApplicationConstraint varargs is not null.
if (refApplicationConstraint == null) failWithMessage("Expecting refApplicationConstraint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefApplicationConstraint(), refApplicationConstraint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refApplicationConstraint contains only the given VecApplicationConstraint elements in Collection and nothing else in whatever order.
* @param refApplicationConstraint the given elements that should be contained in actual VecApplicationConstraint's refApplicationConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refApplicationConstraint does not contain all given VecApplicationConstraint elements.
*/
public S hasOnlyRefApplicationConstraint(java.util.Collection extends VecApplicationConstraint> refApplicationConstraint) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecApplicationConstraint collection is not null.
if (refApplicationConstraint == null) {
failWithMessage("Expecting refApplicationConstraint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefApplicationConstraint(), refApplicationConstraint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refApplicationConstraint does not contain the given VecApplicationConstraint elements.
*
* @param refApplicationConstraint the given elements that should not be in actual VecApplicationConstraint's refApplicationConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refApplicationConstraint contains any given VecApplicationConstraint elements.
*/
public S doesNotHaveRefApplicationConstraint(VecApplicationConstraint... refApplicationConstraint) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecApplicationConstraint varargs is not null.
if (refApplicationConstraint == null) failWithMessage("Expecting refApplicationConstraint parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefApplicationConstraint(), refApplicationConstraint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refApplicationConstraint does not contain the given VecApplicationConstraint elements in Collection.
*
* @param refApplicationConstraint the given elements that should not be in actual VecApplicationConstraint's refApplicationConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refApplicationConstraint contains any given VecApplicationConstraint elements.
*/
public S doesNotHaveRefApplicationConstraint(java.util.Collection extends VecApplicationConstraint> refApplicationConstraint) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecApplicationConstraint collection is not null.
if (refApplicationConstraint == null) {
failWithMessage("Expecting refApplicationConstraint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefApplicationConstraint(), refApplicationConstraint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint has no refApplicationConstraint.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refApplicationConstraint is not empty.
*/
public S hasNoRefApplicationConstraint() {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refApplicationConstraint but had :\n <%s>";
// check
if (actual.getRefApplicationConstraint().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefApplicationConstraint());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refConfigurableElement contains the given VecConfigurableElement elements.
* @param refConfigurableElement the given elements that should be contained in actual VecApplicationConstraint's refConfigurableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refConfigurableElement does not contain all given VecConfigurableElement elements.
*/
public S hasRefConfigurableElement(VecConfigurableElement... refConfigurableElement) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecConfigurableElement varargs is not null.
if (refConfigurableElement == null) failWithMessage("Expecting refConfigurableElement parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefConfigurableElement(), refConfigurableElement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refConfigurableElement contains the given VecConfigurableElement elements in Collection.
* @param refConfigurableElement the given elements that should be contained in actual VecApplicationConstraint's refConfigurableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refConfigurableElement does not contain all given VecConfigurableElement elements.
*/
public S hasRefConfigurableElement(java.util.Collection extends VecConfigurableElement> refConfigurableElement) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecConfigurableElement collection is not null.
if (refConfigurableElement == null) {
failWithMessage("Expecting refConfigurableElement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefConfigurableElement(), refConfigurableElement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refConfigurableElement contains only the given VecConfigurableElement elements and nothing else in whatever order.
* @param refConfigurableElement the given elements that should be contained in actual VecApplicationConstraint's refConfigurableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refConfigurableElement does not contain all given VecConfigurableElement elements.
*/
public S hasOnlyRefConfigurableElement(VecConfigurableElement... refConfigurableElement) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecConfigurableElement varargs is not null.
if (refConfigurableElement == null) failWithMessage("Expecting refConfigurableElement parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefConfigurableElement(), refConfigurableElement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refConfigurableElement contains only the given VecConfigurableElement elements in Collection and nothing else in whatever order.
* @param refConfigurableElement the given elements that should be contained in actual VecApplicationConstraint's refConfigurableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refConfigurableElement does not contain all given VecConfigurableElement elements.
*/
public S hasOnlyRefConfigurableElement(java.util.Collection extends VecConfigurableElement> refConfigurableElement) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecConfigurableElement collection is not null.
if (refConfigurableElement == null) {
failWithMessage("Expecting refConfigurableElement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefConfigurableElement(), refConfigurableElement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refConfigurableElement does not contain the given VecConfigurableElement elements.
*
* @param refConfigurableElement the given elements that should not be in actual VecApplicationConstraint's refConfigurableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refConfigurableElement contains any given VecConfigurableElement elements.
*/
public S doesNotHaveRefConfigurableElement(VecConfigurableElement... refConfigurableElement) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecConfigurableElement varargs is not null.
if (refConfigurableElement == null) failWithMessage("Expecting refConfigurableElement parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefConfigurableElement(), refConfigurableElement);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's refConfigurableElement does not contain the given VecConfigurableElement elements in Collection.
*
* @param refConfigurableElement the given elements that should not be in actual VecApplicationConstraint's refConfigurableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refConfigurableElement contains any given VecConfigurableElement elements.
*/
public S doesNotHaveRefConfigurableElement(java.util.Collection extends VecConfigurableElement> refConfigurableElement) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// check that given VecConfigurableElement collection is not null.
if (refConfigurableElement == null) {
failWithMessage("Expecting refConfigurableElement parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefConfigurableElement(), refConfigurableElement.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint has no refConfigurableElement.
* @return this assertion object.
* @throws AssertionError if the actual VecApplicationConstraint's refConfigurableElement is not empty.
*/
public S hasNoRefConfigurableElement() {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refConfigurableElement but had :\n <%s>";
// check
if (actual.getRefConfigurableElement().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefConfigurableElement());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's toDate is equal to the given one.
* @param toDate the given toDate to compare the actual VecApplicationConstraint's toDate to.
* @return this assertion object.
* @throws AssertionError - if the actual VecApplicationConstraint's toDate is not equal to the given one.
*/
public S hasToDate(javax.xml.datatype.XMLGregorianCalendar toDate) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting toDate of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
javax.xml.datatype.XMLGregorianCalendar actualToDate = actual.getToDate();
if (!Objects.areEqual(actualToDate, toDate)) {
failWithMessage(assertjErrorMessage, actual, toDate, actualToDate);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's toSerialNumber is equal to the given one.
* @param toSerialNumber the given toSerialNumber to compare the actual VecApplicationConstraint's toSerialNumber to.
* @return this assertion object.
* @throws AssertionError - if the actual VecApplicationConstraint's toSerialNumber is not equal to the given one.
*/
public S hasToSerialNumber(String toSerialNumber) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting toSerialNumber of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualToSerialNumber = actual.getToSerialNumber();
if (!Objects.areEqual(actualToSerialNumber, toSerialNumber)) {
failWithMessage(assertjErrorMessage, actual, toSerialNumber, actualToSerialNumber);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecApplicationConstraint's type is equal to the given one.
* @param type the given type to compare the actual VecApplicationConstraint's type to.
* @return this assertion object.
* @throws AssertionError - if the actual VecApplicationConstraint's type is not equal to the given one.
*/
public S hasType(VecApplicationConstraintType type) {
// check that actual VecApplicationConstraint we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting type of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecApplicationConstraintType actualType = actual.getType();
if (!Objects.areEqual(actualType, type)) {
failWithMessage(assertjErrorMessage, actual, type, actualType);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy