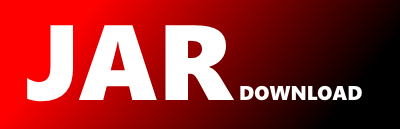
com.foursoft.vecmodel.vec120.AbstractVecBatterySpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecBatterySpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecBatterySpecificationAssert, A extends VecBatterySpecification> extends AbstractVecEEComponentSpecificationAssert {
/**
* Creates a new {@link AbstractVecBatterySpecificationAssert}
to make assertions on actual VecBatterySpecification.
* @param actual the VecBatterySpecification we want to make assertions on.
*/
protected AbstractVecBatterySpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecBatterySpecification's capacity is equal to the given one.
* @param capacity the given capacity to compare the actual VecBatterySpecification's capacity to.
* @return this assertion object.
* @throws AssertionError - if the actual VecBatterySpecification's capacity is not equal to the given one.
*/
public S hasCapacity(VecNumericalValue capacity) {
// check that actual VecBatterySpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting capacity of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualCapacity = actual.getCapacity();
if (!Objects.areEqual(actualCapacity, capacity)) {
failWithMessage(assertjErrorMessage, actual, capacity, actualCapacity);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBatterySpecification's i is equal to the given one.
* @param i the given i to compare the actual VecBatterySpecification's i to.
* @return this assertion object.
* @throws AssertionError - if the actual VecBatterySpecification's i is not equal to the given one.
*/
public S hasI(VecNumericalValue i) {
// check that actual VecBatterySpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting i of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualI = actual.getI();
if (!Objects.areEqual(actualI, i)) {
failWithMessage(assertjErrorMessage, actual, i, actualI);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBatterySpecification's iCool is equal to the given one.
* @param iCool the given iCool to compare the actual VecBatterySpecification's iCool to.
* @return this assertion object.
* @throws AssertionError - if the actual VecBatterySpecification's iCool is not equal to the given one.
*/
public S hasICool(VecNumericalValue iCool) {
// check that actual VecBatterySpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting iCool of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualICool = actual.getICool();
if (!Objects.areEqual(actualICool, iCool)) {
failWithMessage(assertjErrorMessage, actual, iCool, actualICool);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBatterySpecification's u is equal to the given one.
* @param u the given u to compare the actual VecBatterySpecification's u to.
* @return this assertion object.
* @throws AssertionError - if the actual VecBatterySpecification's u is not equal to the given one.
*/
public S hasU(VecNumericalValue u) {
// check that actual VecBatterySpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting u of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualU = actual.getU();
if (!Objects.areEqual(actualU, u)) {
failWithMessage(assertjErrorMessage, actual, u, actualU);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy