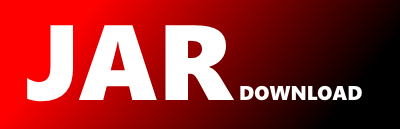
com.foursoft.vecmodel.vec120.AbstractVecBuildingBlockSpecification2DAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecBuildingBlockSpecification2D} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecBuildingBlockSpecification2DAssert, A extends VecBuildingBlockSpecification2D> extends AbstractVecSpecificationAssert {
/**
* Creates a new {@link AbstractVecBuildingBlockSpecification2DAssert}
to make assertions on actual VecBuildingBlockSpecification2D.
* @param actual the VecBuildingBlockSpecification2D we want to make assertions on.
*/
protected AbstractVecBuildingBlockSpecification2DAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's baseUnit is equal to the given one.
* @param baseUnit the given baseUnit to compare the actual VecBuildingBlockSpecification2D's baseUnit to.
* @return this assertion object.
* @throws AssertionError - if the actual VecBuildingBlockSpecification2D's baseUnit is not equal to the given one.
*/
public S hasBaseUnit(VecUnit baseUnit) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting baseUnit of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecUnit actualBaseUnit = actual.getBaseUnit();
if (!Objects.areEqual(actualBaseUnit, baseUnit)) {
failWithMessage(assertjErrorMessage, actual, baseUnit, actualBaseUnit);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's boundingBox is equal to the given one.
* @param boundingBox the given boundingBox to compare the actual VecBuildingBlockSpecification2D's boundingBox to.
* @return this assertion object.
* @throws AssertionError - if the actual VecBuildingBlockSpecification2D's boundingBox is not equal to the given one.
*/
public S hasBoundingBox(VecCartesianDimension boundingBox) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting boundingBox of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCartesianDimension actualBoundingBox = actual.getBoundingBox();
if (!Objects.areEqual(actualBoundingBox, boundingBox)) {
failWithMessage(assertjErrorMessage, actual, boundingBox, actualBoundingBox);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's cartesianPoints contains the given VecCartesianPoint2D elements.
* @param cartesianPoints the given elements that should be contained in actual VecBuildingBlockSpecification2D's cartesianPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's cartesianPoints does not contain all given VecCartesianPoint2D elements.
*/
public S hasCartesianPoints(VecCartesianPoint2D... cartesianPoints) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecCartesianPoint2D varargs is not null.
if (cartesianPoints == null) failWithMessage("Expecting cartesianPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getCartesianPoints(), cartesianPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's cartesianPoints contains the given VecCartesianPoint2D elements in Collection.
* @param cartesianPoints the given elements that should be contained in actual VecBuildingBlockSpecification2D's cartesianPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's cartesianPoints does not contain all given VecCartesianPoint2D elements.
*/
public S hasCartesianPoints(java.util.Collection extends VecCartesianPoint2D> cartesianPoints) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecCartesianPoint2D collection is not null.
if (cartesianPoints == null) {
failWithMessage("Expecting cartesianPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getCartesianPoints(), cartesianPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's cartesianPoints contains only the given VecCartesianPoint2D elements and nothing else in whatever order.
* @param cartesianPoints the given elements that should be contained in actual VecBuildingBlockSpecification2D's cartesianPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's cartesianPoints does not contain all given VecCartesianPoint2D elements.
*/
public S hasOnlyCartesianPoints(VecCartesianPoint2D... cartesianPoints) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecCartesianPoint2D varargs is not null.
if (cartesianPoints == null) failWithMessage("Expecting cartesianPoints parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getCartesianPoints(), cartesianPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's cartesianPoints contains only the given VecCartesianPoint2D elements in Collection and nothing else in whatever order.
* @param cartesianPoints the given elements that should be contained in actual VecBuildingBlockSpecification2D's cartesianPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's cartesianPoints does not contain all given VecCartesianPoint2D elements.
*/
public S hasOnlyCartesianPoints(java.util.Collection extends VecCartesianPoint2D> cartesianPoints) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecCartesianPoint2D collection is not null.
if (cartesianPoints == null) {
failWithMessage("Expecting cartesianPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getCartesianPoints(), cartesianPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's cartesianPoints does not contain the given VecCartesianPoint2D elements.
*
* @param cartesianPoints the given elements that should not be in actual VecBuildingBlockSpecification2D's cartesianPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's cartesianPoints contains any given VecCartesianPoint2D elements.
*/
public S doesNotHaveCartesianPoints(VecCartesianPoint2D... cartesianPoints) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecCartesianPoint2D varargs is not null.
if (cartesianPoints == null) failWithMessage("Expecting cartesianPoints parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getCartesianPoints(), cartesianPoints);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's cartesianPoints does not contain the given VecCartesianPoint2D elements in Collection.
*
* @param cartesianPoints the given elements that should not be in actual VecBuildingBlockSpecification2D's cartesianPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's cartesianPoints contains any given VecCartesianPoint2D elements.
*/
public S doesNotHaveCartesianPoints(java.util.Collection extends VecCartesianPoint2D> cartesianPoints) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecCartesianPoint2D collection is not null.
if (cartesianPoints == null) {
failWithMessage("Expecting cartesianPoints parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getCartesianPoints(), cartesianPoints.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D has no cartesianPoints.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's cartesianPoints is not empty.
*/
public S hasNoCartesianPoints() {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have cartesianPoints but had :\n <%s>";
// check
if (actual.getCartesianPoints().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getCartesianPoints());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometryNodes contains the given VecGeometryNode2D elements.
* @param geometryNodes the given elements that should be contained in actual VecBuildingBlockSpecification2D's geometryNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometryNodes does not contain all given VecGeometryNode2D elements.
*/
public S hasGeometryNodes(VecGeometryNode2D... geometryNodes) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode2D varargs is not null.
if (geometryNodes == null) failWithMessage("Expecting geometryNodes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getGeometryNodes(), geometryNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometryNodes contains the given VecGeometryNode2D elements in Collection.
* @param geometryNodes the given elements that should be contained in actual VecBuildingBlockSpecification2D's geometryNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometryNodes does not contain all given VecGeometryNode2D elements.
*/
public S hasGeometryNodes(java.util.Collection extends VecGeometryNode2D> geometryNodes) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode2D collection is not null.
if (geometryNodes == null) {
failWithMessage("Expecting geometryNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getGeometryNodes(), geometryNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometryNodes contains only the given VecGeometryNode2D elements and nothing else in whatever order.
* @param geometryNodes the given elements that should be contained in actual VecBuildingBlockSpecification2D's geometryNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometryNodes does not contain all given VecGeometryNode2D elements.
*/
public S hasOnlyGeometryNodes(VecGeometryNode2D... geometryNodes) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode2D varargs is not null.
if (geometryNodes == null) failWithMessage("Expecting geometryNodes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getGeometryNodes(), geometryNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometryNodes contains only the given VecGeometryNode2D elements in Collection and nothing else in whatever order.
* @param geometryNodes the given elements that should be contained in actual VecBuildingBlockSpecification2D's geometryNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometryNodes does not contain all given VecGeometryNode2D elements.
*/
public S hasOnlyGeometryNodes(java.util.Collection extends VecGeometryNode2D> geometryNodes) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode2D collection is not null.
if (geometryNodes == null) {
failWithMessage("Expecting geometryNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getGeometryNodes(), geometryNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometryNodes does not contain the given VecGeometryNode2D elements.
*
* @param geometryNodes the given elements that should not be in actual VecBuildingBlockSpecification2D's geometryNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometryNodes contains any given VecGeometryNode2D elements.
*/
public S doesNotHaveGeometryNodes(VecGeometryNode2D... geometryNodes) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode2D varargs is not null.
if (geometryNodes == null) failWithMessage("Expecting geometryNodes parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getGeometryNodes(), geometryNodes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometryNodes does not contain the given VecGeometryNode2D elements in Collection.
*
* @param geometryNodes the given elements that should not be in actual VecBuildingBlockSpecification2D's geometryNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometryNodes contains any given VecGeometryNode2D elements.
*/
public S doesNotHaveGeometryNodes(java.util.Collection extends VecGeometryNode2D> geometryNodes) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometryNode2D collection is not null.
if (geometryNodes == null) {
failWithMessage("Expecting geometryNodes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getGeometryNodes(), geometryNodes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D has no geometryNodes.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometryNodes is not empty.
*/
public S hasNoGeometryNodes() {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have geometryNodes but had :\n <%s>";
// check
if (actual.getGeometryNodes().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getGeometryNodes());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometrySegments contains the given VecGeometrySegment2D elements.
* @param geometrySegments the given elements that should be contained in actual VecBuildingBlockSpecification2D's geometrySegments.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometrySegments does not contain all given VecGeometrySegment2D elements.
*/
public S hasGeometrySegments(VecGeometrySegment2D... geometrySegments) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment2D varargs is not null.
if (geometrySegments == null) failWithMessage("Expecting geometrySegments parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getGeometrySegments(), geometrySegments);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometrySegments contains the given VecGeometrySegment2D elements in Collection.
* @param geometrySegments the given elements that should be contained in actual VecBuildingBlockSpecification2D's geometrySegments.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometrySegments does not contain all given VecGeometrySegment2D elements.
*/
public S hasGeometrySegments(java.util.Collection extends VecGeometrySegment2D> geometrySegments) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment2D collection is not null.
if (geometrySegments == null) {
failWithMessage("Expecting geometrySegments parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getGeometrySegments(), geometrySegments.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometrySegments contains only the given VecGeometrySegment2D elements and nothing else in whatever order.
* @param geometrySegments the given elements that should be contained in actual VecBuildingBlockSpecification2D's geometrySegments.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometrySegments does not contain all given VecGeometrySegment2D elements.
*/
public S hasOnlyGeometrySegments(VecGeometrySegment2D... geometrySegments) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment2D varargs is not null.
if (geometrySegments == null) failWithMessage("Expecting geometrySegments parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getGeometrySegments(), geometrySegments);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometrySegments contains only the given VecGeometrySegment2D elements in Collection and nothing else in whatever order.
* @param geometrySegments the given elements that should be contained in actual VecBuildingBlockSpecification2D's geometrySegments.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometrySegments does not contain all given VecGeometrySegment2D elements.
*/
public S hasOnlyGeometrySegments(java.util.Collection extends VecGeometrySegment2D> geometrySegments) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment2D collection is not null.
if (geometrySegments == null) {
failWithMessage("Expecting geometrySegments parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getGeometrySegments(), geometrySegments.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometrySegments does not contain the given VecGeometrySegment2D elements.
*
* @param geometrySegments the given elements that should not be in actual VecBuildingBlockSpecification2D's geometrySegments.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometrySegments contains any given VecGeometrySegment2D elements.
*/
public S doesNotHaveGeometrySegments(VecGeometrySegment2D... geometrySegments) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment2D varargs is not null.
if (geometrySegments == null) failWithMessage("Expecting geometrySegments parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getGeometrySegments(), geometrySegments);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's geometrySegments does not contain the given VecGeometrySegment2D elements in Collection.
*
* @param geometrySegments the given elements that should not be in actual VecBuildingBlockSpecification2D's geometrySegments.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometrySegments contains any given VecGeometrySegment2D elements.
*/
public S doesNotHaveGeometrySegments(java.util.Collection extends VecGeometrySegment2D> geometrySegments) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecGeometrySegment2D collection is not null.
if (geometrySegments == null) {
failWithMessage("Expecting geometrySegments parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getGeometrySegments(), geometrySegments.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D has no geometrySegments.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's geometrySegments is not empty.
*/
public S hasNoGeometrySegments() {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have geometrySegments but had :\n <%s>";
// check
if (actual.getGeometrySegments().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getGeometrySegments());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's placedElementViewItems contains the given VecOccurrenceOrUsageViewItem2D elements.
* @param placedElementViewItems the given elements that should be contained in actual VecBuildingBlockSpecification2D's placedElementViewItems.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's placedElementViewItems does not contain all given VecOccurrenceOrUsageViewItem2D elements.
*/
public S hasPlacedElementViewItems(VecOccurrenceOrUsageViewItem2D... placedElementViewItems) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsageViewItem2D varargs is not null.
if (placedElementViewItems == null) failWithMessage("Expecting placedElementViewItems parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPlacedElementViewItems(), placedElementViewItems);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's placedElementViewItems contains the given VecOccurrenceOrUsageViewItem2D elements in Collection.
* @param placedElementViewItems the given elements that should be contained in actual VecBuildingBlockSpecification2D's placedElementViewItems.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's placedElementViewItems does not contain all given VecOccurrenceOrUsageViewItem2D elements.
*/
public S hasPlacedElementViewItems(java.util.Collection extends VecOccurrenceOrUsageViewItem2D> placedElementViewItems) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsageViewItem2D collection is not null.
if (placedElementViewItems == null) {
failWithMessage("Expecting placedElementViewItems parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPlacedElementViewItems(), placedElementViewItems.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's placedElementViewItems contains only the given VecOccurrenceOrUsageViewItem2D elements and nothing else in whatever order.
* @param placedElementViewItems the given elements that should be contained in actual VecBuildingBlockSpecification2D's placedElementViewItems.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's placedElementViewItems does not contain all given VecOccurrenceOrUsageViewItem2D elements.
*/
public S hasOnlyPlacedElementViewItems(VecOccurrenceOrUsageViewItem2D... placedElementViewItems) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsageViewItem2D varargs is not null.
if (placedElementViewItems == null) failWithMessage("Expecting placedElementViewItems parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPlacedElementViewItems(), placedElementViewItems);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's placedElementViewItems contains only the given VecOccurrenceOrUsageViewItem2D elements in Collection and nothing else in whatever order.
* @param placedElementViewItems the given elements that should be contained in actual VecBuildingBlockSpecification2D's placedElementViewItems.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's placedElementViewItems does not contain all given VecOccurrenceOrUsageViewItem2D elements.
*/
public S hasOnlyPlacedElementViewItems(java.util.Collection extends VecOccurrenceOrUsageViewItem2D> placedElementViewItems) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsageViewItem2D collection is not null.
if (placedElementViewItems == null) {
failWithMessage("Expecting placedElementViewItems parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPlacedElementViewItems(), placedElementViewItems.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's placedElementViewItems does not contain the given VecOccurrenceOrUsageViewItem2D elements.
*
* @param placedElementViewItems the given elements that should not be in actual VecBuildingBlockSpecification2D's placedElementViewItems.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's placedElementViewItems contains any given VecOccurrenceOrUsageViewItem2D elements.
*/
public S doesNotHavePlacedElementViewItems(VecOccurrenceOrUsageViewItem2D... placedElementViewItems) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsageViewItem2D varargs is not null.
if (placedElementViewItems == null) failWithMessage("Expecting placedElementViewItems parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPlacedElementViewItems(), placedElementViewItems);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's placedElementViewItems does not contain the given VecOccurrenceOrUsageViewItem2D elements in Collection.
*
* @param placedElementViewItems the given elements that should not be in actual VecBuildingBlockSpecification2D's placedElementViewItems.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's placedElementViewItems contains any given VecOccurrenceOrUsageViewItem2D elements.
*/
public S doesNotHavePlacedElementViewItems(java.util.Collection extends VecOccurrenceOrUsageViewItem2D> placedElementViewItems) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecOccurrenceOrUsageViewItem2D collection is not null.
if (placedElementViewItems == null) {
failWithMessage("Expecting placedElementViewItems parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPlacedElementViewItems(), placedElementViewItems.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D has no placedElementViewItems.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's placedElementViewItems is not empty.
*/
public S hasNoPlacedElementViewItems() {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have placedElementViewItems but had :\n <%s>";
// check
if (actual.getPlacedElementViewItems().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getPlacedElementViewItems());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D contains the given VecBuildingBlockPositioning2D elements.
* @param refBuildingBlockPositioning2D the given elements that should be contained in actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D does not contain all given VecBuildingBlockPositioning2D elements.
*/
public S hasRefBuildingBlockPositioning2D(VecBuildingBlockPositioning2D... refBuildingBlockPositioning2D) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockPositioning2D varargs is not null.
if (refBuildingBlockPositioning2D == null) failWithMessage("Expecting refBuildingBlockPositioning2D parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefBuildingBlockPositioning2D(), refBuildingBlockPositioning2D);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D contains the given VecBuildingBlockPositioning2D elements in Collection.
* @param refBuildingBlockPositioning2D the given elements that should be contained in actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D does not contain all given VecBuildingBlockPositioning2D elements.
*/
public S hasRefBuildingBlockPositioning2D(java.util.Collection extends VecBuildingBlockPositioning2D> refBuildingBlockPositioning2D) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockPositioning2D collection is not null.
if (refBuildingBlockPositioning2D == null) {
failWithMessage("Expecting refBuildingBlockPositioning2D parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefBuildingBlockPositioning2D(), refBuildingBlockPositioning2D.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D contains only the given VecBuildingBlockPositioning2D elements and nothing else in whatever order.
* @param refBuildingBlockPositioning2D the given elements that should be contained in actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D does not contain all given VecBuildingBlockPositioning2D elements.
*/
public S hasOnlyRefBuildingBlockPositioning2D(VecBuildingBlockPositioning2D... refBuildingBlockPositioning2D) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockPositioning2D varargs is not null.
if (refBuildingBlockPositioning2D == null) failWithMessage("Expecting refBuildingBlockPositioning2D parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefBuildingBlockPositioning2D(), refBuildingBlockPositioning2D);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D contains only the given VecBuildingBlockPositioning2D elements in Collection and nothing else in whatever order.
* @param refBuildingBlockPositioning2D the given elements that should be contained in actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D does not contain all given VecBuildingBlockPositioning2D elements.
*/
public S hasOnlyRefBuildingBlockPositioning2D(java.util.Collection extends VecBuildingBlockPositioning2D> refBuildingBlockPositioning2D) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockPositioning2D collection is not null.
if (refBuildingBlockPositioning2D == null) {
failWithMessage("Expecting refBuildingBlockPositioning2D parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefBuildingBlockPositioning2D(), refBuildingBlockPositioning2D.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D does not contain the given VecBuildingBlockPositioning2D elements.
*
* @param refBuildingBlockPositioning2D the given elements that should not be in actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D contains any given VecBuildingBlockPositioning2D elements.
*/
public S doesNotHaveRefBuildingBlockPositioning2D(VecBuildingBlockPositioning2D... refBuildingBlockPositioning2D) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockPositioning2D varargs is not null.
if (refBuildingBlockPositioning2D == null) failWithMessage("Expecting refBuildingBlockPositioning2D parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefBuildingBlockPositioning2D(), refBuildingBlockPositioning2D);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D does not contain the given VecBuildingBlockPositioning2D elements in Collection.
*
* @param refBuildingBlockPositioning2D the given elements that should not be in actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D contains any given VecBuildingBlockPositioning2D elements.
*/
public S doesNotHaveRefBuildingBlockPositioning2D(java.util.Collection extends VecBuildingBlockPositioning2D> refBuildingBlockPositioning2D) {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// check that given VecBuildingBlockPositioning2D collection is not null.
if (refBuildingBlockPositioning2D == null) {
failWithMessage("Expecting refBuildingBlockPositioning2D parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefBuildingBlockPositioning2D(), refBuildingBlockPositioning2D.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecBuildingBlockSpecification2D has no refBuildingBlockPositioning2D.
* @return this assertion object.
* @throws AssertionError if the actual VecBuildingBlockSpecification2D's refBuildingBlockPositioning2D is not empty.
*/
public S hasNoRefBuildingBlockPositioning2D() {
// check that actual VecBuildingBlockSpecification2D we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refBuildingBlockPositioning2D but had :\n <%s>";
// check
if (actual.getRefBuildingBlockPositioning2D().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefBuildingBlockPositioning2D());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy