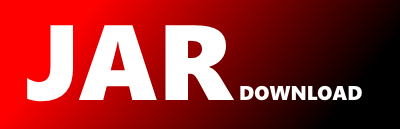
com.foursoft.vecmodel.vec120.AbstractVecCableLeadThroughReferenceAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.api.AbstractObjectAssert;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecCableLeadThroughReference} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecCableLeadThroughReferenceAssert, A extends VecCableLeadThroughReference> extends AbstractObjectAssert {
/**
* Creates a new {@link AbstractVecCableLeadThroughReferenceAssert}
to make assertions on actual VecCableLeadThroughReference.
* @param actual the VecCableLeadThroughReference we want to make assertions on.
*/
protected AbstractVecCableLeadThroughReferenceAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecCableLeadThroughReference's cableLeadThrough is equal to the given one.
* @param cableLeadThrough the given cableLeadThrough to compare the actual VecCableLeadThroughReference's cableLeadThrough to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCableLeadThroughReference's cableLeadThrough is not equal to the given one.
*/
public S hasCableLeadThrough(VecCableLeadThrough cableLeadThrough) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting cableLeadThrough of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCableLeadThrough actualCableLeadThrough = actual.getCableLeadThrough();
if (!Objects.areEqual(actualCableLeadThrough, cableLeadThrough)) {
failWithMessage(assertjErrorMessage, actual, cableLeadThrough, actualCableLeadThrough);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's identification is equal to the given one.
* @param identification the given identification to compare the actual VecCableLeadThroughReference's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCableLeadThroughReference's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's parentGrommetRole is equal to the given one.
* @param parentGrommetRole the given parentGrommetRole to compare the actual VecCableLeadThroughReference's parentGrommetRole to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCableLeadThroughReference's parentGrommetRole is not equal to the given one.
*/
public S hasParentGrommetRole(VecGrommetRole parentGrommetRole) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentGrommetRole of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecGrommetRole actualParentGrommetRole = actual.getParentGrommetRole();
if (!Objects.areEqual(actualParentGrommetRole, parentGrommetRole)) {
failWithMessage(assertjErrorMessage, actual, parentGrommetRole, actualParentGrommetRole);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedPlugs contains the given VecCavityPlugRole elements.
* @param usedPlugs the given elements that should be contained in actual VecCableLeadThroughReference's usedPlugs.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedPlugs does not contain all given VecCavityPlugRole elements.
*/
public S hasUsedPlugs(VecCavityPlugRole... usedPlugs) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole varargs is not null.
if (usedPlugs == null) failWithMessage("Expecting usedPlugs parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getUsedPlugs(), usedPlugs);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedPlugs contains the given VecCavityPlugRole elements in Collection.
* @param usedPlugs the given elements that should be contained in actual VecCableLeadThroughReference's usedPlugs.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedPlugs does not contain all given VecCavityPlugRole elements.
*/
public S hasUsedPlugs(java.util.Collection extends VecCavityPlugRole> usedPlugs) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole collection is not null.
if (usedPlugs == null) {
failWithMessage("Expecting usedPlugs parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getUsedPlugs(), usedPlugs.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedPlugs contains only the given VecCavityPlugRole elements and nothing else in whatever order.
* @param usedPlugs the given elements that should be contained in actual VecCableLeadThroughReference's usedPlugs.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedPlugs does not contain all given VecCavityPlugRole elements.
*/
public S hasOnlyUsedPlugs(VecCavityPlugRole... usedPlugs) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole varargs is not null.
if (usedPlugs == null) failWithMessage("Expecting usedPlugs parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getUsedPlugs(), usedPlugs);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedPlugs contains only the given VecCavityPlugRole elements in Collection and nothing else in whatever order.
* @param usedPlugs the given elements that should be contained in actual VecCableLeadThroughReference's usedPlugs.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedPlugs does not contain all given VecCavityPlugRole elements.
*/
public S hasOnlyUsedPlugs(java.util.Collection extends VecCavityPlugRole> usedPlugs) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole collection is not null.
if (usedPlugs == null) {
failWithMessage("Expecting usedPlugs parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getUsedPlugs(), usedPlugs.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedPlugs does not contain the given VecCavityPlugRole elements.
*
* @param usedPlugs the given elements that should not be in actual VecCableLeadThroughReference's usedPlugs.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedPlugs contains any given VecCavityPlugRole elements.
*/
public S doesNotHaveUsedPlugs(VecCavityPlugRole... usedPlugs) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole varargs is not null.
if (usedPlugs == null) failWithMessage("Expecting usedPlugs parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getUsedPlugs(), usedPlugs);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedPlugs does not contain the given VecCavityPlugRole elements in Collection.
*
* @param usedPlugs the given elements that should not be in actual VecCableLeadThroughReference's usedPlugs.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedPlugs contains any given VecCavityPlugRole elements.
*/
public S doesNotHaveUsedPlugs(java.util.Collection extends VecCavityPlugRole> usedPlugs) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole collection is not null.
if (usedPlugs == null) {
failWithMessage("Expecting usedPlugs parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getUsedPlugs(), usedPlugs.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference has no usedPlugs.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedPlugs is not empty.
*/
public S hasNoUsedPlugs() {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have usedPlugs but had :\n <%s>";
// check
if (actual.getUsedPlugs().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getUsedPlugs());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedSeals contains the given VecCavitySealRole elements.
* @param usedSeals the given elements that should be contained in actual VecCableLeadThroughReference's usedSeals.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedSeals does not contain all given VecCavitySealRole elements.
*/
public S hasUsedSeals(VecCavitySealRole... usedSeals) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavitySealRole varargs is not null.
if (usedSeals == null) failWithMessage("Expecting usedSeals parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getUsedSeals(), usedSeals);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedSeals contains the given VecCavitySealRole elements in Collection.
* @param usedSeals the given elements that should be contained in actual VecCableLeadThroughReference's usedSeals.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedSeals does not contain all given VecCavitySealRole elements.
*/
public S hasUsedSeals(java.util.Collection extends VecCavitySealRole> usedSeals) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavitySealRole collection is not null.
if (usedSeals == null) {
failWithMessage("Expecting usedSeals parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getUsedSeals(), usedSeals.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedSeals contains only the given VecCavitySealRole elements and nothing else in whatever order.
* @param usedSeals the given elements that should be contained in actual VecCableLeadThroughReference's usedSeals.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedSeals does not contain all given VecCavitySealRole elements.
*/
public S hasOnlyUsedSeals(VecCavitySealRole... usedSeals) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavitySealRole varargs is not null.
if (usedSeals == null) failWithMessage("Expecting usedSeals parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getUsedSeals(), usedSeals);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedSeals contains only the given VecCavitySealRole elements in Collection and nothing else in whatever order.
* @param usedSeals the given elements that should be contained in actual VecCableLeadThroughReference's usedSeals.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedSeals does not contain all given VecCavitySealRole elements.
*/
public S hasOnlyUsedSeals(java.util.Collection extends VecCavitySealRole> usedSeals) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavitySealRole collection is not null.
if (usedSeals == null) {
failWithMessage("Expecting usedSeals parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getUsedSeals(), usedSeals.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedSeals does not contain the given VecCavitySealRole elements.
*
* @param usedSeals the given elements that should not be in actual VecCableLeadThroughReference's usedSeals.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedSeals contains any given VecCavitySealRole elements.
*/
public S doesNotHaveUsedSeals(VecCavitySealRole... usedSeals) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavitySealRole varargs is not null.
if (usedSeals == null) failWithMessage("Expecting usedSeals parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getUsedSeals(), usedSeals);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's usedSeals does not contain the given VecCavitySealRole elements in Collection.
*
* @param usedSeals the given elements that should not be in actual VecCableLeadThroughReference's usedSeals.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedSeals contains any given VecCavitySealRole elements.
*/
public S doesNotHaveUsedSeals(java.util.Collection extends VecCavitySealRole> usedSeals) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavitySealRole collection is not null.
if (usedSeals == null) {
failWithMessage("Expecting usedSeals parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getUsedSeals(), usedSeals.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference has no usedSeals.
* @return this assertion object.
* @throws AssertionError if the actual VecCableLeadThroughReference's usedSeals is not empty.
*/
public S hasNoUsedSeals() {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have usedSeals but had :\n <%s>";
// check
if (actual.getUsedSeals().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getUsedSeals());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableLeadThroughReference's xmlId is equal to the given one.
* @param xmlId the given xmlId to compare the actual VecCableLeadThroughReference's xmlId to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCableLeadThroughReference's xmlId is not equal to the given one.
*/
public S hasXmlId(String xmlId) {
// check that actual VecCableLeadThroughReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting xmlId of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualXmlId = actual.getXmlId();
if (!Objects.areEqual(actualXmlId, xmlId)) {
failWithMessage(assertjErrorMessage, actual, xmlId, actualXmlId);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy