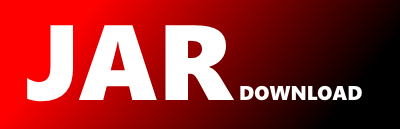
com.foursoft.vecmodel.vec120.AbstractVecCableTieSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecCableTieSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecCableTieSpecificationAssert, A extends VecCableTieSpecification> extends AbstractVecPartOrUsageRelatedSpecificationAssert {
/**
* Creates a new {@link AbstractVecCableTieSpecificationAssert}
to make assertions on actual VecCableTieSpecification.
* @param actual the VecCableTieSpecification we want to make assertions on.
*/
protected AbstractVecCableTieSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecCableTieSpecification's length is equal to the given one.
* @param length the given length to compare the actual VecCableTieSpecification's length to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCableTieSpecification's length is not equal to the given one.
*/
public S hasLength(VecNumericalValue length) {
// check that actual VecCableTieSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting length of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualLength = actual.getLength();
if (!Objects.areEqual(actualLength, length)) {
failWithMessage(assertjErrorMessage, actual, length, actualLength);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableTieSpecification's refCableTieRole contains the given VecCableTieRole elements.
* @param refCableTieRole the given elements that should be contained in actual VecCableTieSpecification's refCableTieRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCableTieSpecification's refCableTieRole does not contain all given VecCableTieRole elements.
*/
public S hasRefCableTieRole(VecCableTieRole... refCableTieRole) {
// check that actual VecCableTieSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCableTieRole varargs is not null.
if (refCableTieRole == null) failWithMessage("Expecting refCableTieRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCableTieRole(), refCableTieRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableTieSpecification's refCableTieRole contains the given VecCableTieRole elements in Collection.
* @param refCableTieRole the given elements that should be contained in actual VecCableTieSpecification's refCableTieRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCableTieSpecification's refCableTieRole does not contain all given VecCableTieRole elements.
*/
public S hasRefCableTieRole(java.util.Collection extends VecCableTieRole> refCableTieRole) {
// check that actual VecCableTieSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCableTieRole collection is not null.
if (refCableTieRole == null) {
failWithMessage("Expecting refCableTieRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCableTieRole(), refCableTieRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableTieSpecification's refCableTieRole contains only the given VecCableTieRole elements and nothing else in whatever order.
* @param refCableTieRole the given elements that should be contained in actual VecCableTieSpecification's refCableTieRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCableTieSpecification's refCableTieRole does not contain all given VecCableTieRole elements.
*/
public S hasOnlyRefCableTieRole(VecCableTieRole... refCableTieRole) {
// check that actual VecCableTieSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCableTieRole varargs is not null.
if (refCableTieRole == null) failWithMessage("Expecting refCableTieRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCableTieRole(), refCableTieRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableTieSpecification's refCableTieRole contains only the given VecCableTieRole elements in Collection and nothing else in whatever order.
* @param refCableTieRole the given elements that should be contained in actual VecCableTieSpecification's refCableTieRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCableTieSpecification's refCableTieRole does not contain all given VecCableTieRole elements.
*/
public S hasOnlyRefCableTieRole(java.util.Collection extends VecCableTieRole> refCableTieRole) {
// check that actual VecCableTieSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCableTieRole collection is not null.
if (refCableTieRole == null) {
failWithMessage("Expecting refCableTieRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCableTieRole(), refCableTieRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableTieSpecification's refCableTieRole does not contain the given VecCableTieRole elements.
*
* @param refCableTieRole the given elements that should not be in actual VecCableTieSpecification's refCableTieRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCableTieSpecification's refCableTieRole contains any given VecCableTieRole elements.
*/
public S doesNotHaveRefCableTieRole(VecCableTieRole... refCableTieRole) {
// check that actual VecCableTieSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCableTieRole varargs is not null.
if (refCableTieRole == null) failWithMessage("Expecting refCableTieRole parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCableTieRole(), refCableTieRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableTieSpecification's refCableTieRole does not contain the given VecCableTieRole elements in Collection.
*
* @param refCableTieRole the given elements that should not be in actual VecCableTieSpecification's refCableTieRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCableTieSpecification's refCableTieRole contains any given VecCableTieRole elements.
*/
public S doesNotHaveRefCableTieRole(java.util.Collection extends VecCableTieRole> refCableTieRole) {
// check that actual VecCableTieSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCableTieRole collection is not null.
if (refCableTieRole == null) {
failWithMessage("Expecting refCableTieRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCableTieRole(), refCableTieRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableTieSpecification has no refCableTieRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCableTieSpecification's refCableTieRole is not empty.
*/
public S hasNoRefCableTieRole() {
// check that actual VecCableTieSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCableTieRole but had :\n <%s>";
// check
if (actual.getRefCableTieRole().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCableTieRole());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableTieSpecification's tensioningStrength is equal to the given one.
* @param tensioningStrength the given tensioningStrength to compare the actual VecCableTieSpecification's tensioningStrength to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCableTieSpecification's tensioningStrength is not equal to the given one.
*/
public S hasTensioningStrength(VecNumericalValue tensioningStrength) {
// check that actual VecCableTieSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting tensioningStrength of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualTensioningStrength = actual.getTensioningStrength();
if (!Objects.areEqual(actualTensioningStrength, tensioningStrength)) {
failWithMessage(assertjErrorMessage, actual, tensioningStrength, actualTensioningStrength);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableTieSpecification's thickness is equal to the given one.
* @param thickness the given thickness to compare the actual VecCableTieSpecification's thickness to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCableTieSpecification's thickness is not equal to the given one.
*/
public S hasThickness(VecNumericalValue thickness) {
// check that actual VecCableTieSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting thickness of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualThickness = actual.getThickness();
if (!Objects.areEqual(actualThickness, thickness)) {
failWithMessage(assertjErrorMessage, actual, thickness, actualThickness);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCableTieSpecification's width is equal to the given one.
* @param width the given width to compare the actual VecCableTieSpecification's width to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCableTieSpecification's width is not equal to the given one.
*/
public S hasWidth(VecNumericalValue width) {
// check that actual VecCableTieSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting width of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualWidth = actual.getWidth();
if (!Objects.areEqual(actualWidth, width)) {
failWithMessage(assertjErrorMessage, actual, width, actualWidth);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy