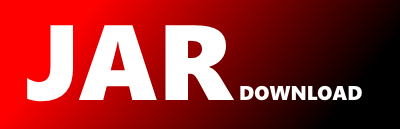
com.foursoft.vecmodel.vec120.AbstractVecCartesianVector3DAssert Maven / Gradle / Ivy
Show all versions of vec120-assertions Show documentation
package com.foursoft.vecmodel.vec120;
import org.assertj.core.api.Assertions;
/**
* Abstract base class for {@link VecCartesianVector3D} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecCartesianVector3DAssert, A extends VecCartesianVector3D> extends AbstractVecCartesianVectorAssert {
/**
* Creates a new {@link AbstractVecCartesianVector3DAssert}
to make assertions on actual VecCartesianVector3D.
* @param actual the VecCartesianVector3D we want to make assertions on.
*/
protected AbstractVecCartesianVector3DAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecCartesianVector3D's x is equal to the given one.
* @param x the given x to compare the actual VecCartesianVector3D's x to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCartesianVector3D's x is not equal to the given one.
*/
public S hasX(double x) {
// check that actual VecCartesianVector3D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting x of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check value for x
double actualX = actual.getX();
if (actualX != x) {
failWithMessage(assertjErrorMessage, actual, x, actualX);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCartesianVector3D's x is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param x the value to compare the actual VecCartesianVector3D's x to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecCartesianVector3D's x is not close enough to the given value.
*/
public S hasXCloseTo(double x, double assertjOffset) {
// check that actual VecCartesianVector3D we want to make assertions on is not null.
isNotNull();
double actualX = actual.getX();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting x:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualX, x, assertjOffset, Math.abs(x - actualX));
// check
Assertions.assertThat(actualX).overridingErrorMessage(assertjErrorMessage).isCloseTo(x, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCartesianVector3D's y is equal to the given one.
* @param y the given y to compare the actual VecCartesianVector3D's y to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCartesianVector3D's y is not equal to the given one.
*/
public S hasY(double y) {
// check that actual VecCartesianVector3D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting y of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check value for y
double actualY = actual.getY();
if (actualY != y) {
failWithMessage(assertjErrorMessage, actual, y, actualY);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCartesianVector3D's y is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param y the value to compare the actual VecCartesianVector3D's y to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecCartesianVector3D's y is not close enough to the given value.
*/
public S hasYCloseTo(double y, double assertjOffset) {
// check that actual VecCartesianVector3D we want to make assertions on is not null.
isNotNull();
double actualY = actual.getY();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting y:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualY, y, assertjOffset, Math.abs(y - actualY));
// check
Assertions.assertThat(actualY).overridingErrorMessage(assertjErrorMessage).isCloseTo(y, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCartesianVector3D's z is equal to the given one.
* @param z the given z to compare the actual VecCartesianVector3D's z to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCartesianVector3D's z is not equal to the given one.
*/
public S hasZ(double z) {
// check that actual VecCartesianVector3D we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting z of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check value for z
double actualZ = actual.getZ();
if (actualZ != z) {
failWithMessage(assertjErrorMessage, actual, z, actualZ);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCartesianVector3D's z is close to the given value by less than the given offset.
*
* If difference is equal to the offset value, assertion is considered successful.
* @param z the value to compare the actual VecCartesianVector3D's z to.
* @param assertjOffset the given offset.
* @return this assertion object.
* @throws AssertionError - if the actual VecCartesianVector3D's z is not close enough to the given value.
*/
public S hasZCloseTo(double z, double assertjOffset) {
// check that actual VecCartesianVector3D we want to make assertions on is not null.
isNotNull();
double actualZ = actual.getZ();
// overrides the default error message with a more explicit one
String assertjErrorMessage = String.format("\nExpecting z:\n <%s>\nto be close to:\n <%s>\nby less than <%s> but difference was <%s>",
actualZ, z, assertjOffset, Math.abs(z - actualZ));
// check
Assertions.assertThat(actualZ).overridingErrorMessage(assertjErrorMessage).isCloseTo(z, Assertions.within(assertjOffset));
// return the current assertion for method chaining
return myself;
}
}