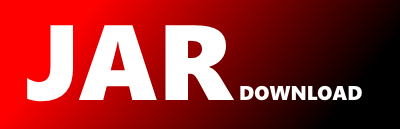
com.foursoft.vecmodel.vec120.AbstractVecCavityAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecCavity} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecCavityAssert, A extends VecCavity> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecCavityAssert}
to make assertions on actual VecCavity.
* @param actual the VecCavity we want to make assertions on.
*/
protected AbstractVecCavityAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecCavity is available.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavity is not available.
*/
public S isAvailable() {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.FALSE, actual.isAvailable())) {
failWithMessage("\nExpecting that actual VecCavity is available but is not.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity is not available.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavity is available.
*/
public S isNotAvailable() {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.TRUE, actual.isAvailable())) {
failWithMessage("\nExpecting that actual VecCavity is not available but is.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's cavityNumber is equal to the given one.
* @param cavityNumber the given cavityNumber to compare the actual VecCavity's cavityNumber to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavity's cavityNumber is not equal to the given one.
*/
public S hasCavityNumber(String cavityNumber) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting cavityNumber of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualCavityNumber = actual.getCavityNumber();
if (!Objects.areEqual(actualCavityNumber, cavityNumber)) {
failWithMessage(assertjErrorMessage, actual, cavityNumber, actualCavityNumber);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's cavitySpecification is equal to the given one.
* @param cavitySpecification the given cavitySpecification to compare the actual VecCavity's cavitySpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavity's cavitySpecification is not equal to the given one.
*/
public S hasCavitySpecification(VecCavitySpecification cavitySpecification) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting cavitySpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCavitySpecification actualCavitySpecification = actual.getCavitySpecification();
if (!Objects.areEqual(actualCavitySpecification, cavitySpecification)) {
failWithMessage(assertjErrorMessage, actual, cavitySpecification, actualCavitySpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity is has integrated terminal.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavity is not has integrated terminal.
*/
public S isHasIntegratedTerminal() {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.FALSE, actual.isHasIntegratedTerminal())) {
failWithMessage("\nExpecting that actual VecCavity is has integrated terminal but is not.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity is not has integrated terminal.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavity is has integrated terminal.
*/
public S isNotHasIntegratedTerminal() {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.TRUE, actual.isHasIntegratedTerminal())) {
failWithMessage("\nExpecting that actual VecCavity is not has integrated terminal but is.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's integratedTerminalSpecification is equal to the given one.
* @param integratedTerminalSpecification the given integratedTerminalSpecification to compare the actual VecCavity's integratedTerminalSpecification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavity's integratedTerminalSpecification is not equal to the given one.
*/
public S hasIntegratedTerminalSpecification(VecTerminalSpecification integratedTerminalSpecification) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting integratedTerminalSpecification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTerminalSpecification actualIntegratedTerminalSpecification = actual.getIntegratedTerminalSpecification();
if (!Objects.areEqual(actualIntegratedTerminalSpecification, integratedTerminalSpecification)) {
failWithMessage(assertjErrorMessage, actual, integratedTerminalSpecification, actualIntegratedTerminalSpecification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's parentSlot is equal to the given one.
* @param parentSlot the given parentSlot to compare the actual VecCavity's parentSlot to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavity's parentSlot is not equal to the given one.
*/
public S hasParentSlot(VecSlot parentSlot) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentSlot of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecSlot actualParentSlot = actual.getParentSlot();
if (!Objects.areEqual(actualParentSlot, parentSlot)) {
failWithMessage(assertjErrorMessage, actual, parentSlot, actualParentSlot);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityAddOn contains the given VecCavityAddOn elements.
* @param refCavityAddOn the given elements that should be contained in actual VecCavity's refCavityAddOn.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityAddOn does not contain all given VecCavityAddOn elements.
*/
public S hasRefCavityAddOn(VecCavityAddOn... refCavityAddOn) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityAddOn varargs is not null.
if (refCavityAddOn == null) failWithMessage("Expecting refCavityAddOn parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityAddOn(), refCavityAddOn);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityAddOn contains the given VecCavityAddOn elements in Collection.
* @param refCavityAddOn the given elements that should be contained in actual VecCavity's refCavityAddOn.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityAddOn does not contain all given VecCavityAddOn elements.
*/
public S hasRefCavityAddOn(java.util.Collection extends VecCavityAddOn> refCavityAddOn) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityAddOn collection is not null.
if (refCavityAddOn == null) {
failWithMessage("Expecting refCavityAddOn parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityAddOn(), refCavityAddOn.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityAddOn contains only the given VecCavityAddOn elements and nothing else in whatever order.
* @param refCavityAddOn the given elements that should be contained in actual VecCavity's refCavityAddOn.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityAddOn does not contain all given VecCavityAddOn elements.
*/
public S hasOnlyRefCavityAddOn(VecCavityAddOn... refCavityAddOn) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityAddOn varargs is not null.
if (refCavityAddOn == null) failWithMessage("Expecting refCavityAddOn parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityAddOn(), refCavityAddOn);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityAddOn contains only the given VecCavityAddOn elements in Collection and nothing else in whatever order.
* @param refCavityAddOn the given elements that should be contained in actual VecCavity's refCavityAddOn.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityAddOn does not contain all given VecCavityAddOn elements.
*/
public S hasOnlyRefCavityAddOn(java.util.Collection extends VecCavityAddOn> refCavityAddOn) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityAddOn collection is not null.
if (refCavityAddOn == null) {
failWithMessage("Expecting refCavityAddOn parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityAddOn(), refCavityAddOn.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityAddOn does not contain the given VecCavityAddOn elements.
*
* @param refCavityAddOn the given elements that should not be in actual VecCavity's refCavityAddOn.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityAddOn contains any given VecCavityAddOn elements.
*/
public S doesNotHaveRefCavityAddOn(VecCavityAddOn... refCavityAddOn) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityAddOn varargs is not null.
if (refCavityAddOn == null) failWithMessage("Expecting refCavityAddOn parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityAddOn(), refCavityAddOn);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityAddOn does not contain the given VecCavityAddOn elements in Collection.
*
* @param refCavityAddOn the given elements that should not be in actual VecCavity's refCavityAddOn.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityAddOn contains any given VecCavityAddOn elements.
*/
public S doesNotHaveRefCavityAddOn(java.util.Collection extends VecCavityAddOn> refCavityAddOn) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityAddOn collection is not null.
if (refCavityAddOn == null) {
failWithMessage("Expecting refCavityAddOn parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityAddOn(), refCavityAddOn.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity has no refCavityAddOn.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityAddOn is not empty.
*/
public S hasNoRefCavityAddOn() {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCavityAddOn but had :\n <%s>";
// check
if (actual.getRefCavityAddOn().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCavityAddOn());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityReference contains the given VecCavityReference elements.
* @param refCavityReference the given elements that should be contained in actual VecCavity's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityReference does not contain all given VecCavityReference elements.
*/
public S hasRefCavityReference(VecCavityReference... refCavityReference) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference varargs is not null.
if (refCavityReference == null) failWithMessage("Expecting refCavityReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityReference(), refCavityReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityReference contains the given VecCavityReference elements in Collection.
* @param refCavityReference the given elements that should be contained in actual VecCavity's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityReference does not contain all given VecCavityReference elements.
*/
public S hasRefCavityReference(java.util.Collection extends VecCavityReference> refCavityReference) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference collection is not null.
if (refCavityReference == null) {
failWithMessage("Expecting refCavityReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityReference(), refCavityReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityReference contains only the given VecCavityReference elements and nothing else in whatever order.
* @param refCavityReference the given elements that should be contained in actual VecCavity's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityReference does not contain all given VecCavityReference elements.
*/
public S hasOnlyRefCavityReference(VecCavityReference... refCavityReference) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference varargs is not null.
if (refCavityReference == null) failWithMessage("Expecting refCavityReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityReference(), refCavityReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityReference contains only the given VecCavityReference elements in Collection and nothing else in whatever order.
* @param refCavityReference the given elements that should be contained in actual VecCavity's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityReference does not contain all given VecCavityReference elements.
*/
public S hasOnlyRefCavityReference(java.util.Collection extends VecCavityReference> refCavityReference) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference collection is not null.
if (refCavityReference == null) {
failWithMessage("Expecting refCavityReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityReference(), refCavityReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityReference does not contain the given VecCavityReference elements.
*
* @param refCavityReference the given elements that should not be in actual VecCavity's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityReference contains any given VecCavityReference elements.
*/
public S doesNotHaveRefCavityReference(VecCavityReference... refCavityReference) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference varargs is not null.
if (refCavityReference == null) failWithMessage("Expecting refCavityReference parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityReference(), refCavityReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refCavityReference does not contain the given VecCavityReference elements in Collection.
*
* @param refCavityReference the given elements that should not be in actual VecCavity's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityReference contains any given VecCavityReference elements.
*/
public S doesNotHaveRefCavityReference(java.util.Collection extends VecCavityReference> refCavityReference) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference collection is not null.
if (refCavityReference == null) {
failWithMessage("Expecting refCavityReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityReference(), refCavityReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity has no refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refCavityReference is not empty.
*/
public S hasNoRefCavityReference() {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCavityReference but had :\n <%s>";
// check
if (actual.getRefCavityReference().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCavityReference());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refOpenCavitiesAssignment contains the given VecOpenCavitiesAssignment elements.
* @param refOpenCavitiesAssignment the given elements that should be contained in actual VecCavity's refOpenCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refOpenCavitiesAssignment does not contain all given VecOpenCavitiesAssignment elements.
*/
public S hasRefOpenCavitiesAssignment(VecOpenCavitiesAssignment... refOpenCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecOpenCavitiesAssignment varargs is not null.
if (refOpenCavitiesAssignment == null) failWithMessage("Expecting refOpenCavitiesAssignment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefOpenCavitiesAssignment(), refOpenCavitiesAssignment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refOpenCavitiesAssignment contains the given VecOpenCavitiesAssignment elements in Collection.
* @param refOpenCavitiesAssignment the given elements that should be contained in actual VecCavity's refOpenCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refOpenCavitiesAssignment does not contain all given VecOpenCavitiesAssignment elements.
*/
public S hasRefOpenCavitiesAssignment(java.util.Collection extends VecOpenCavitiesAssignment> refOpenCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecOpenCavitiesAssignment collection is not null.
if (refOpenCavitiesAssignment == null) {
failWithMessage("Expecting refOpenCavitiesAssignment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefOpenCavitiesAssignment(), refOpenCavitiesAssignment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refOpenCavitiesAssignment contains only the given VecOpenCavitiesAssignment elements and nothing else in whatever order.
* @param refOpenCavitiesAssignment the given elements that should be contained in actual VecCavity's refOpenCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refOpenCavitiesAssignment does not contain all given VecOpenCavitiesAssignment elements.
*/
public S hasOnlyRefOpenCavitiesAssignment(VecOpenCavitiesAssignment... refOpenCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecOpenCavitiesAssignment varargs is not null.
if (refOpenCavitiesAssignment == null) failWithMessage("Expecting refOpenCavitiesAssignment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefOpenCavitiesAssignment(), refOpenCavitiesAssignment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refOpenCavitiesAssignment contains only the given VecOpenCavitiesAssignment elements in Collection and nothing else in whatever order.
* @param refOpenCavitiesAssignment the given elements that should be contained in actual VecCavity's refOpenCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refOpenCavitiesAssignment does not contain all given VecOpenCavitiesAssignment elements.
*/
public S hasOnlyRefOpenCavitiesAssignment(java.util.Collection extends VecOpenCavitiesAssignment> refOpenCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecOpenCavitiesAssignment collection is not null.
if (refOpenCavitiesAssignment == null) {
failWithMessage("Expecting refOpenCavitiesAssignment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefOpenCavitiesAssignment(), refOpenCavitiesAssignment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refOpenCavitiesAssignment does not contain the given VecOpenCavitiesAssignment elements.
*
* @param refOpenCavitiesAssignment the given elements that should not be in actual VecCavity's refOpenCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refOpenCavitiesAssignment contains any given VecOpenCavitiesAssignment elements.
*/
public S doesNotHaveRefOpenCavitiesAssignment(VecOpenCavitiesAssignment... refOpenCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecOpenCavitiesAssignment varargs is not null.
if (refOpenCavitiesAssignment == null) failWithMessage("Expecting refOpenCavitiesAssignment parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefOpenCavitiesAssignment(), refOpenCavitiesAssignment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refOpenCavitiesAssignment does not contain the given VecOpenCavitiesAssignment elements in Collection.
*
* @param refOpenCavitiesAssignment the given elements that should not be in actual VecCavity's refOpenCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refOpenCavitiesAssignment contains any given VecOpenCavitiesAssignment elements.
*/
public S doesNotHaveRefOpenCavitiesAssignment(java.util.Collection extends VecOpenCavitiesAssignment> refOpenCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecOpenCavitiesAssignment collection is not null.
if (refOpenCavitiesAssignment == null) {
failWithMessage("Expecting refOpenCavitiesAssignment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefOpenCavitiesAssignment(), refOpenCavitiesAssignment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity has no refOpenCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refOpenCavitiesAssignment is not empty.
*/
public S hasNoRefOpenCavitiesAssignment() {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refOpenCavitiesAssignment but had :\n <%s>";
// check
if (actual.getRefOpenCavitiesAssignment().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefOpenCavitiesAssignment());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refPinComponent contains the given VecPinComponent elements.
* @param refPinComponent the given elements that should be contained in actual VecCavity's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refPinComponent does not contain all given VecPinComponent elements.
*/
public S hasRefPinComponent(VecPinComponent... refPinComponent) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent varargs is not null.
if (refPinComponent == null) failWithMessage("Expecting refPinComponent parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPinComponent(), refPinComponent);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refPinComponent contains the given VecPinComponent elements in Collection.
* @param refPinComponent the given elements that should be contained in actual VecCavity's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refPinComponent does not contain all given VecPinComponent elements.
*/
public S hasRefPinComponent(java.util.Collection extends VecPinComponent> refPinComponent) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent collection is not null.
if (refPinComponent == null) {
failWithMessage("Expecting refPinComponent parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefPinComponent(), refPinComponent.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refPinComponent contains only the given VecPinComponent elements and nothing else in whatever order.
* @param refPinComponent the given elements that should be contained in actual VecCavity's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refPinComponent does not contain all given VecPinComponent elements.
*/
public S hasOnlyRefPinComponent(VecPinComponent... refPinComponent) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent varargs is not null.
if (refPinComponent == null) failWithMessage("Expecting refPinComponent parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPinComponent(), refPinComponent);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refPinComponent contains only the given VecPinComponent elements in Collection and nothing else in whatever order.
* @param refPinComponent the given elements that should be contained in actual VecCavity's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refPinComponent does not contain all given VecPinComponent elements.
*/
public S hasOnlyRefPinComponent(java.util.Collection extends VecPinComponent> refPinComponent) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent collection is not null.
if (refPinComponent == null) {
failWithMessage("Expecting refPinComponent parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefPinComponent(), refPinComponent.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refPinComponent does not contain the given VecPinComponent elements.
*
* @param refPinComponent the given elements that should not be in actual VecCavity's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refPinComponent contains any given VecPinComponent elements.
*/
public S doesNotHaveRefPinComponent(VecPinComponent... refPinComponent) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent varargs is not null.
if (refPinComponent == null) failWithMessage("Expecting refPinComponent parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPinComponent(), refPinComponent);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refPinComponent does not contain the given VecPinComponent elements in Collection.
*
* @param refPinComponent the given elements that should not be in actual VecCavity's refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refPinComponent contains any given VecPinComponent elements.
*/
public S doesNotHaveRefPinComponent(java.util.Collection extends VecPinComponent> refPinComponent) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecPinComponent collection is not null.
if (refPinComponent == null) {
failWithMessage("Expecting refPinComponent parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefPinComponent(), refPinComponent.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity has no refPinComponent.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refPinComponent is not empty.
*/
public S hasNoRefPinComponent() {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refPinComponent but had :\n <%s>";
// check
if (actual.getRefPinComponent().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefPinComponent());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSealedCavitiesAssignment contains the given VecSealedCavitiesAssignment elements.
* @param refSealedCavitiesAssignment the given elements that should be contained in actual VecCavity's refSealedCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSealedCavitiesAssignment does not contain all given VecSealedCavitiesAssignment elements.
*/
public S hasRefSealedCavitiesAssignment(VecSealedCavitiesAssignment... refSealedCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSealedCavitiesAssignment varargs is not null.
if (refSealedCavitiesAssignment == null) failWithMessage("Expecting refSealedCavitiesAssignment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSealedCavitiesAssignment(), refSealedCavitiesAssignment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSealedCavitiesAssignment contains the given VecSealedCavitiesAssignment elements in Collection.
* @param refSealedCavitiesAssignment the given elements that should be contained in actual VecCavity's refSealedCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSealedCavitiesAssignment does not contain all given VecSealedCavitiesAssignment elements.
*/
public S hasRefSealedCavitiesAssignment(java.util.Collection extends VecSealedCavitiesAssignment> refSealedCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSealedCavitiesAssignment collection is not null.
if (refSealedCavitiesAssignment == null) {
failWithMessage("Expecting refSealedCavitiesAssignment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSealedCavitiesAssignment(), refSealedCavitiesAssignment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSealedCavitiesAssignment contains only the given VecSealedCavitiesAssignment elements and nothing else in whatever order.
* @param refSealedCavitiesAssignment the given elements that should be contained in actual VecCavity's refSealedCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSealedCavitiesAssignment does not contain all given VecSealedCavitiesAssignment elements.
*/
public S hasOnlyRefSealedCavitiesAssignment(VecSealedCavitiesAssignment... refSealedCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSealedCavitiesAssignment varargs is not null.
if (refSealedCavitiesAssignment == null) failWithMessage("Expecting refSealedCavitiesAssignment parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSealedCavitiesAssignment(), refSealedCavitiesAssignment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSealedCavitiesAssignment contains only the given VecSealedCavitiesAssignment elements in Collection and nothing else in whatever order.
* @param refSealedCavitiesAssignment the given elements that should be contained in actual VecCavity's refSealedCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSealedCavitiesAssignment does not contain all given VecSealedCavitiesAssignment elements.
*/
public S hasOnlyRefSealedCavitiesAssignment(java.util.Collection extends VecSealedCavitiesAssignment> refSealedCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSealedCavitiesAssignment collection is not null.
if (refSealedCavitiesAssignment == null) {
failWithMessage("Expecting refSealedCavitiesAssignment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSealedCavitiesAssignment(), refSealedCavitiesAssignment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSealedCavitiesAssignment does not contain the given VecSealedCavitiesAssignment elements.
*
* @param refSealedCavitiesAssignment the given elements that should not be in actual VecCavity's refSealedCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSealedCavitiesAssignment contains any given VecSealedCavitiesAssignment elements.
*/
public S doesNotHaveRefSealedCavitiesAssignment(VecSealedCavitiesAssignment... refSealedCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSealedCavitiesAssignment varargs is not null.
if (refSealedCavitiesAssignment == null) failWithMessage("Expecting refSealedCavitiesAssignment parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSealedCavitiesAssignment(), refSealedCavitiesAssignment);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSealedCavitiesAssignment does not contain the given VecSealedCavitiesAssignment elements in Collection.
*
* @param refSealedCavitiesAssignment the given elements that should not be in actual VecCavity's refSealedCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSealedCavitiesAssignment contains any given VecSealedCavitiesAssignment elements.
*/
public S doesNotHaveRefSealedCavitiesAssignment(java.util.Collection extends VecSealedCavitiesAssignment> refSealedCavitiesAssignment) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSealedCavitiesAssignment collection is not null.
if (refSealedCavitiesAssignment == null) {
failWithMessage("Expecting refSealedCavitiesAssignment parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSealedCavitiesAssignment(), refSealedCavitiesAssignment.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity has no refSealedCavitiesAssignment.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSealedCavitiesAssignment is not empty.
*/
public S hasNoRefSealedCavitiesAssignment() {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refSealedCavitiesAssignment but had :\n <%s>";
// check
if (actual.getRefSealedCavitiesAssignment().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefSealedCavitiesAssignment());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSegmentConnectionPoint contains the given VecSegmentConnectionPoint elements.
* @param refSegmentConnectionPoint the given elements that should be contained in actual VecCavity's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSegmentConnectionPoint does not contain all given VecSegmentConnectionPoint elements.
*/
public S hasRefSegmentConnectionPoint(VecSegmentConnectionPoint... refSegmentConnectionPoint) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint varargs is not null.
if (refSegmentConnectionPoint == null) failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSegmentConnectionPoint contains the given VecSegmentConnectionPoint elements in Collection.
* @param refSegmentConnectionPoint the given elements that should be contained in actual VecCavity's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSegmentConnectionPoint does not contain all given VecSegmentConnectionPoint elements.
*/
public S hasRefSegmentConnectionPoint(java.util.Collection extends VecSegmentConnectionPoint> refSegmentConnectionPoint) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint collection is not null.
if (refSegmentConnectionPoint == null) {
failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSegmentConnectionPoint contains only the given VecSegmentConnectionPoint elements and nothing else in whatever order.
* @param refSegmentConnectionPoint the given elements that should be contained in actual VecCavity's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSegmentConnectionPoint does not contain all given VecSegmentConnectionPoint elements.
*/
public S hasOnlyRefSegmentConnectionPoint(VecSegmentConnectionPoint... refSegmentConnectionPoint) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint varargs is not null.
if (refSegmentConnectionPoint == null) failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSegmentConnectionPoint contains only the given VecSegmentConnectionPoint elements in Collection and nothing else in whatever order.
* @param refSegmentConnectionPoint the given elements that should be contained in actual VecCavity's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSegmentConnectionPoint does not contain all given VecSegmentConnectionPoint elements.
*/
public S hasOnlyRefSegmentConnectionPoint(java.util.Collection extends VecSegmentConnectionPoint> refSegmentConnectionPoint) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint collection is not null.
if (refSegmentConnectionPoint == null) {
failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSegmentConnectionPoint does not contain the given VecSegmentConnectionPoint elements.
*
* @param refSegmentConnectionPoint the given elements that should not be in actual VecCavity's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSegmentConnectionPoint contains any given VecSegmentConnectionPoint elements.
*/
public S doesNotHaveRefSegmentConnectionPoint(VecSegmentConnectionPoint... refSegmentConnectionPoint) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint varargs is not null.
if (refSegmentConnectionPoint == null) failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity's refSegmentConnectionPoint does not contain the given VecSegmentConnectionPoint elements in Collection.
*
* @param refSegmentConnectionPoint the given elements that should not be in actual VecCavity's refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSegmentConnectionPoint contains any given VecSegmentConnectionPoint elements.
*/
public S doesNotHaveRefSegmentConnectionPoint(java.util.Collection extends VecSegmentConnectionPoint> refSegmentConnectionPoint) {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// check that given VecSegmentConnectionPoint collection is not null.
if (refSegmentConnectionPoint == null) {
failWithMessage("Expecting refSegmentConnectionPoint parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSegmentConnectionPoint(), refSegmentConnectionPoint.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavity has no refSegmentConnectionPoint.
* @return this assertion object.
* @throws AssertionError if the actual VecCavity's refSegmentConnectionPoint is not empty.
*/
public S hasNoRefSegmentConnectionPoint() {
// check that actual VecCavity we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refSegmentConnectionPoint but had :\n <%s>";
// check
if (actual.getRefSegmentConnectionPoint().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefSegmentConnectionPoint());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy