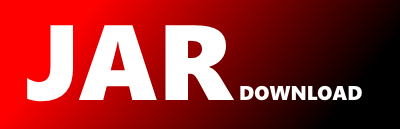
com.foursoft.vecmodel.vec120.AbstractVecCavityReferenceAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecCavityReference} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecCavityReferenceAssert, A extends VecCavityReference> extends AbstractVecExtendableElementAssert {
/**
* Creates a new {@link AbstractVecCavityReferenceAssert}
to make assertions on actual VecCavityReference.
* @param actual the VecCavityReference we want to make assertions on.
*/
protected AbstractVecCavityReferenceAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecCavityReference's componentPort is equal to the given one.
* @param componentPort the given componentPort to compare the actual VecCavityReference's componentPort to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavityReference's componentPort is not equal to the given one.
*/
public S hasComponentPort(VecComponentPort componentPort) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting componentPort of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecComponentPort actualComponentPort = actual.getComponentPort();
if (!Objects.areEqual(actualComponentPort, componentPort)) {
failWithMessage(assertjErrorMessage, actual, componentPort, actualComponentPort);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's identification is equal to the given one.
* @param identification the given identification to compare the actual VecCavityReference's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavityReference's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's integratedTerminalRole is equal to the given one.
* @param integratedTerminalRole the given integratedTerminalRole to compare the actual VecCavityReference's integratedTerminalRole to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavityReference's integratedTerminalRole is not equal to the given one.
*/
public S hasIntegratedTerminalRole(VecTerminalRole integratedTerminalRole) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting integratedTerminalRole of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecTerminalRole actualIntegratedTerminalRole = actual.getIntegratedTerminalRole();
if (!Objects.areEqual(actualIntegratedTerminalRole, integratedTerminalRole)) {
failWithMessage(assertjErrorMessage, actual, integratedTerminalRole, actualIntegratedTerminalRole);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's parentSlotReference is equal to the given one.
* @param parentSlotReference the given parentSlotReference to compare the actual VecCavityReference's parentSlotReference to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavityReference's parentSlotReference is not equal to the given one.
*/
public S hasParentSlotReference(VecSlotReference parentSlotReference) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentSlotReference of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecSlotReference actualParentSlotReference = actual.getParentSlotReference();
if (!Objects.areEqual(actualParentSlotReference, parentSlotReference)) {
failWithMessage(assertjErrorMessage, actual, parentSlotReference, actualParentSlotReference);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityCoupling contains the given VecCavityCoupling elements.
* @param refCavityCoupling the given elements that should be contained in actual VecCavityReference's refCavityCoupling.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityCoupling does not contain all given VecCavityCoupling elements.
*/
public S hasRefCavityCoupling(VecCavityCoupling... refCavityCoupling) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityCoupling varargs is not null.
if (refCavityCoupling == null) failWithMessage("Expecting refCavityCoupling parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityCoupling(), refCavityCoupling);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityCoupling contains the given VecCavityCoupling elements in Collection.
* @param refCavityCoupling the given elements that should be contained in actual VecCavityReference's refCavityCoupling.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityCoupling does not contain all given VecCavityCoupling elements.
*/
public S hasRefCavityCoupling(java.util.Collection extends VecCavityCoupling> refCavityCoupling) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityCoupling collection is not null.
if (refCavityCoupling == null) {
failWithMessage("Expecting refCavityCoupling parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityCoupling(), refCavityCoupling.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityCoupling contains only the given VecCavityCoupling elements and nothing else in whatever order.
* @param refCavityCoupling the given elements that should be contained in actual VecCavityReference's refCavityCoupling.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityCoupling does not contain all given VecCavityCoupling elements.
*/
public S hasOnlyRefCavityCoupling(VecCavityCoupling... refCavityCoupling) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityCoupling varargs is not null.
if (refCavityCoupling == null) failWithMessage("Expecting refCavityCoupling parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityCoupling(), refCavityCoupling);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityCoupling contains only the given VecCavityCoupling elements in Collection and nothing else in whatever order.
* @param refCavityCoupling the given elements that should be contained in actual VecCavityReference's refCavityCoupling.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityCoupling does not contain all given VecCavityCoupling elements.
*/
public S hasOnlyRefCavityCoupling(java.util.Collection extends VecCavityCoupling> refCavityCoupling) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityCoupling collection is not null.
if (refCavityCoupling == null) {
failWithMessage("Expecting refCavityCoupling parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityCoupling(), refCavityCoupling.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityCoupling does not contain the given VecCavityCoupling elements.
*
* @param refCavityCoupling the given elements that should not be in actual VecCavityReference's refCavityCoupling.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityCoupling contains any given VecCavityCoupling elements.
*/
public S doesNotHaveRefCavityCoupling(VecCavityCoupling... refCavityCoupling) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityCoupling varargs is not null.
if (refCavityCoupling == null) failWithMessage("Expecting refCavityCoupling parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityCoupling(), refCavityCoupling);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityCoupling does not contain the given VecCavityCoupling elements in Collection.
*
* @param refCavityCoupling the given elements that should not be in actual VecCavityReference's refCavityCoupling.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityCoupling contains any given VecCavityCoupling elements.
*/
public S doesNotHaveRefCavityCoupling(java.util.Collection extends VecCavityCoupling> refCavityCoupling) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityCoupling collection is not null.
if (refCavityCoupling == null) {
failWithMessage("Expecting refCavityCoupling parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityCoupling(), refCavityCoupling.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference has no refCavityCoupling.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityCoupling is not empty.
*/
public S hasNoRefCavityCoupling() {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCavityCoupling but had :\n <%s>";
// check
if (actual.getRefCavityCoupling().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCavityCoupling());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMounting contains the given VecCavityMounting elements.
* @param refCavityMounting the given elements that should be contained in actual VecCavityReference's refCavityMounting.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMounting does not contain all given VecCavityMounting elements.
*/
public S hasRefCavityMounting(VecCavityMounting... refCavityMounting) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMounting varargs is not null.
if (refCavityMounting == null) failWithMessage("Expecting refCavityMounting parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityMounting(), refCavityMounting);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMounting contains the given VecCavityMounting elements in Collection.
* @param refCavityMounting the given elements that should be contained in actual VecCavityReference's refCavityMounting.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMounting does not contain all given VecCavityMounting elements.
*/
public S hasRefCavityMounting(java.util.Collection extends VecCavityMounting> refCavityMounting) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMounting collection is not null.
if (refCavityMounting == null) {
failWithMessage("Expecting refCavityMounting parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityMounting(), refCavityMounting.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMounting contains only the given VecCavityMounting elements and nothing else in whatever order.
* @param refCavityMounting the given elements that should be contained in actual VecCavityReference's refCavityMounting.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMounting does not contain all given VecCavityMounting elements.
*/
public S hasOnlyRefCavityMounting(VecCavityMounting... refCavityMounting) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMounting varargs is not null.
if (refCavityMounting == null) failWithMessage("Expecting refCavityMounting parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityMounting(), refCavityMounting);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMounting contains only the given VecCavityMounting elements in Collection and nothing else in whatever order.
* @param refCavityMounting the given elements that should be contained in actual VecCavityReference's refCavityMounting.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMounting does not contain all given VecCavityMounting elements.
*/
public S hasOnlyRefCavityMounting(java.util.Collection extends VecCavityMounting> refCavityMounting) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMounting collection is not null.
if (refCavityMounting == null) {
failWithMessage("Expecting refCavityMounting parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityMounting(), refCavityMounting.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMounting does not contain the given VecCavityMounting elements.
*
* @param refCavityMounting the given elements that should not be in actual VecCavityReference's refCavityMounting.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMounting contains any given VecCavityMounting elements.
*/
public S doesNotHaveRefCavityMounting(VecCavityMounting... refCavityMounting) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMounting varargs is not null.
if (refCavityMounting == null) failWithMessage("Expecting refCavityMounting parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityMounting(), refCavityMounting);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMounting does not contain the given VecCavityMounting elements in Collection.
*
* @param refCavityMounting the given elements that should not be in actual VecCavityReference's refCavityMounting.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMounting contains any given VecCavityMounting elements.
*/
public S doesNotHaveRefCavityMounting(java.util.Collection extends VecCavityMounting> refCavityMounting) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMounting collection is not null.
if (refCavityMounting == null) {
failWithMessage("Expecting refCavityMounting parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityMounting(), refCavityMounting.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference has no refCavityMounting.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMounting is not empty.
*/
public S hasNoRefCavityMounting() {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCavityMounting but had :\n <%s>";
// check
if (actual.getRefCavityMounting().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCavityMounting());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMountingDetail contains the given VecCavityMountingDetail elements.
* @param refCavityMountingDetail the given elements that should be contained in actual VecCavityReference's refCavityMountingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMountingDetail does not contain all given VecCavityMountingDetail elements.
*/
public S hasRefCavityMountingDetail(VecCavityMountingDetail... refCavityMountingDetail) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMountingDetail varargs is not null.
if (refCavityMountingDetail == null) failWithMessage("Expecting refCavityMountingDetail parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityMountingDetail(), refCavityMountingDetail);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMountingDetail contains the given VecCavityMountingDetail elements in Collection.
* @param refCavityMountingDetail the given elements that should be contained in actual VecCavityReference's refCavityMountingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMountingDetail does not contain all given VecCavityMountingDetail elements.
*/
public S hasRefCavityMountingDetail(java.util.Collection extends VecCavityMountingDetail> refCavityMountingDetail) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMountingDetail collection is not null.
if (refCavityMountingDetail == null) {
failWithMessage("Expecting refCavityMountingDetail parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityMountingDetail(), refCavityMountingDetail.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMountingDetail contains only the given VecCavityMountingDetail elements and nothing else in whatever order.
* @param refCavityMountingDetail the given elements that should be contained in actual VecCavityReference's refCavityMountingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMountingDetail does not contain all given VecCavityMountingDetail elements.
*/
public S hasOnlyRefCavityMountingDetail(VecCavityMountingDetail... refCavityMountingDetail) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMountingDetail varargs is not null.
if (refCavityMountingDetail == null) failWithMessage("Expecting refCavityMountingDetail parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityMountingDetail(), refCavityMountingDetail);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMountingDetail contains only the given VecCavityMountingDetail elements in Collection and nothing else in whatever order.
* @param refCavityMountingDetail the given elements that should be contained in actual VecCavityReference's refCavityMountingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMountingDetail does not contain all given VecCavityMountingDetail elements.
*/
public S hasOnlyRefCavityMountingDetail(java.util.Collection extends VecCavityMountingDetail> refCavityMountingDetail) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMountingDetail collection is not null.
if (refCavityMountingDetail == null) {
failWithMessage("Expecting refCavityMountingDetail parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityMountingDetail(), refCavityMountingDetail.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMountingDetail does not contain the given VecCavityMountingDetail elements.
*
* @param refCavityMountingDetail the given elements that should not be in actual VecCavityReference's refCavityMountingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMountingDetail contains any given VecCavityMountingDetail elements.
*/
public S doesNotHaveRefCavityMountingDetail(VecCavityMountingDetail... refCavityMountingDetail) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMountingDetail varargs is not null.
if (refCavityMountingDetail == null) failWithMessage("Expecting refCavityMountingDetail parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityMountingDetail(), refCavityMountingDetail);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityMountingDetail does not contain the given VecCavityMountingDetail elements in Collection.
*
* @param refCavityMountingDetail the given elements that should not be in actual VecCavityReference's refCavityMountingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMountingDetail contains any given VecCavityMountingDetail elements.
*/
public S doesNotHaveRefCavityMountingDetail(java.util.Collection extends VecCavityMountingDetail> refCavityMountingDetail) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityMountingDetail collection is not null.
if (refCavityMountingDetail == null) {
failWithMessage("Expecting refCavityMountingDetail parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityMountingDetail(), refCavityMountingDetail.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference has no refCavityMountingDetail.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityMountingDetail is not empty.
*/
public S hasNoRefCavityMountingDetail() {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCavityMountingDetail but had :\n <%s>";
// check
if (actual.getRefCavityMountingDetail().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCavityMountingDetail());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityPlugRole contains the given VecCavityPlugRole elements.
* @param refCavityPlugRole the given elements that should be contained in actual VecCavityReference's refCavityPlugRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityPlugRole does not contain all given VecCavityPlugRole elements.
*/
public S hasRefCavityPlugRole(VecCavityPlugRole... refCavityPlugRole) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole varargs is not null.
if (refCavityPlugRole == null) failWithMessage("Expecting refCavityPlugRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityPlugRole(), refCavityPlugRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityPlugRole contains the given VecCavityPlugRole elements in Collection.
* @param refCavityPlugRole the given elements that should be contained in actual VecCavityReference's refCavityPlugRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityPlugRole does not contain all given VecCavityPlugRole elements.
*/
public S hasRefCavityPlugRole(java.util.Collection extends VecCavityPlugRole> refCavityPlugRole) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole collection is not null.
if (refCavityPlugRole == null) {
failWithMessage("Expecting refCavityPlugRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityPlugRole(), refCavityPlugRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityPlugRole contains only the given VecCavityPlugRole elements and nothing else in whatever order.
* @param refCavityPlugRole the given elements that should be contained in actual VecCavityReference's refCavityPlugRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityPlugRole does not contain all given VecCavityPlugRole elements.
*/
public S hasOnlyRefCavityPlugRole(VecCavityPlugRole... refCavityPlugRole) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole varargs is not null.
if (refCavityPlugRole == null) failWithMessage("Expecting refCavityPlugRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityPlugRole(), refCavityPlugRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityPlugRole contains only the given VecCavityPlugRole elements in Collection and nothing else in whatever order.
* @param refCavityPlugRole the given elements that should be contained in actual VecCavityReference's refCavityPlugRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityPlugRole does not contain all given VecCavityPlugRole elements.
*/
public S hasOnlyRefCavityPlugRole(java.util.Collection extends VecCavityPlugRole> refCavityPlugRole) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole collection is not null.
if (refCavityPlugRole == null) {
failWithMessage("Expecting refCavityPlugRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityPlugRole(), refCavityPlugRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityPlugRole does not contain the given VecCavityPlugRole elements.
*
* @param refCavityPlugRole the given elements that should not be in actual VecCavityReference's refCavityPlugRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityPlugRole contains any given VecCavityPlugRole elements.
*/
public S doesNotHaveRefCavityPlugRole(VecCavityPlugRole... refCavityPlugRole) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole varargs is not null.
if (refCavityPlugRole == null) failWithMessage("Expecting refCavityPlugRole parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityPlugRole(), refCavityPlugRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's refCavityPlugRole does not contain the given VecCavityPlugRole elements in Collection.
*
* @param refCavityPlugRole the given elements that should not be in actual VecCavityReference's refCavityPlugRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityPlugRole contains any given VecCavityPlugRole elements.
*/
public S doesNotHaveRefCavityPlugRole(java.util.Collection extends VecCavityPlugRole> refCavityPlugRole) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// check that given VecCavityPlugRole collection is not null.
if (refCavityPlugRole == null) {
failWithMessage("Expecting refCavityPlugRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityPlugRole(), refCavityPlugRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference has no refCavityPlugRole.
* @return this assertion object.
* @throws AssertionError if the actual VecCavityReference's refCavityPlugRole is not empty.
*/
public S hasNoRefCavityPlugRole() {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCavityPlugRole but had :\n <%s>";
// check
if (actual.getRefCavityPlugRole().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCavityPlugRole());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavityReference's referencedCavity is equal to the given one.
* @param referencedCavity the given referencedCavity to compare the actual VecCavityReference's referencedCavity to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavityReference's referencedCavity is not equal to the given one.
*/
public S hasReferencedCavity(VecCavity referencedCavity) {
// check that actual VecCavityReference we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting referencedCavity of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecCavity actualReferencedCavity = actual.getReferencedCavity();
if (!Objects.areEqual(actualReferencedCavity, referencedCavity)) {
failWithMessage(assertjErrorMessage, actual, referencedCavity, actualReferencedCavity);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy