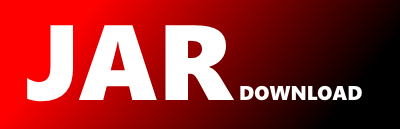
com.foursoft.vecmodel.vec120.AbstractVecCavitySpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecCavitySpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecCavitySpecificationAssert, A extends VecCavitySpecification> extends AbstractVecSpecificationAssert {
/**
* Creates a new {@link AbstractVecCavitySpecificationAssert}
to make assertions on actual VecCavitySpecification.
* @param actual the VecCavitySpecification we want to make assertions on.
*/
protected AbstractVecCavitySpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecCavitySpecification's angles contains the given VecNumericalValue elements.
* @param angles the given elements that should be contained in actual VecCavitySpecification's angles.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's angles does not contain all given VecNumericalValue elements.
*/
public S hasAngles(VecNumericalValue... angles) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNumericalValue varargs is not null.
if (angles == null) failWithMessage("Expecting angles parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAngles(), angles);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's angles contains the given VecNumericalValue elements in Collection.
* @param angles the given elements that should be contained in actual VecCavitySpecification's angles.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's angles does not contain all given VecNumericalValue elements.
*/
public S hasAngles(java.util.Collection extends VecNumericalValue> angles) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNumericalValue collection is not null.
if (angles == null) {
failWithMessage("Expecting angles parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getAngles(), angles.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's angles contains only the given VecNumericalValue elements and nothing else in whatever order.
* @param angles the given elements that should be contained in actual VecCavitySpecification's angles.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's angles does not contain all given VecNumericalValue elements.
*/
public S hasOnlyAngles(VecNumericalValue... angles) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNumericalValue varargs is not null.
if (angles == null) failWithMessage("Expecting angles parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAngles(), angles);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's angles contains only the given VecNumericalValue elements in Collection and nothing else in whatever order.
* @param angles the given elements that should be contained in actual VecCavitySpecification's angles.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's angles does not contain all given VecNumericalValue elements.
*/
public S hasOnlyAngles(java.util.Collection extends VecNumericalValue> angles) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNumericalValue collection is not null.
if (angles == null) {
failWithMessage("Expecting angles parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getAngles(), angles.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's angles does not contain the given VecNumericalValue elements.
*
* @param angles the given elements that should not be in actual VecCavitySpecification's angles.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's angles contains any given VecNumericalValue elements.
*/
public S doesNotHaveAngles(VecNumericalValue... angles) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNumericalValue varargs is not null.
if (angles == null) failWithMessage("Expecting angles parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAngles(), angles);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's angles does not contain the given VecNumericalValue elements in Collection.
*
* @param angles the given elements that should not be in actual VecCavitySpecification's angles.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's angles contains any given VecNumericalValue elements.
*/
public S doesNotHaveAngles(java.util.Collection extends VecNumericalValue> angles) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecNumericalValue collection is not null.
if (angles == null) {
failWithMessage("Expecting angles parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getAngles(), angles.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification has no angles.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's angles is not empty.
*/
public S hasNoAngles() {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have angles but had :\n <%s>";
// check
if (actual.getAngles().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getAngles());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's cavityDesign is equal to the given one.
* @param cavityDesign the given cavityDesign to compare the actual VecCavitySpecification's cavityDesign to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavitySpecification's cavityDesign is not equal to the given one.
*/
public S hasCavityDesign(String cavityDesign) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting cavityDesign of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualCavityDesign = actual.getCavityDesign();
if (!Objects.areEqual(actualCavityDesign, cavityDesign)) {
failWithMessage(assertjErrorMessage, actual, cavityDesign, actualCavityDesign);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's cavityDimension is equal to the given one.
* @param cavityDimension the given cavityDimension to compare the actual VecCavitySpecification's cavityDimension to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavitySpecification's cavityDimension is not equal to the given one.
*/
public S hasCavityDimension(VecSize cavityDimension) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting cavityDimension of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecSize actualCavityDimension = actual.getCavityDimension();
if (!Objects.areEqual(actualCavityDimension, cavityDimension)) {
failWithMessage(assertjErrorMessage, actual, cavityDimension, actualCavityDimension);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's compatibleTerminalTypes contains the given VecTerminalType elements.
* @param compatibleTerminalTypes the given elements that should be contained in actual VecCavitySpecification's compatibleTerminalTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's compatibleTerminalTypes does not contain all given VecTerminalType elements.
*/
public S hasCompatibleTerminalTypes(VecTerminalType... compatibleTerminalTypes) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalType varargs is not null.
if (compatibleTerminalTypes == null) failWithMessage("Expecting compatibleTerminalTypes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getCompatibleTerminalTypes(), compatibleTerminalTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's compatibleTerminalTypes contains the given VecTerminalType elements in Collection.
* @param compatibleTerminalTypes the given elements that should be contained in actual VecCavitySpecification's compatibleTerminalTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's compatibleTerminalTypes does not contain all given VecTerminalType elements.
*/
public S hasCompatibleTerminalTypes(java.util.Collection extends VecTerminalType> compatibleTerminalTypes) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalType collection is not null.
if (compatibleTerminalTypes == null) {
failWithMessage("Expecting compatibleTerminalTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getCompatibleTerminalTypes(), compatibleTerminalTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's compatibleTerminalTypes contains only the given VecTerminalType elements and nothing else in whatever order.
* @param compatibleTerminalTypes the given elements that should be contained in actual VecCavitySpecification's compatibleTerminalTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's compatibleTerminalTypes does not contain all given VecTerminalType elements.
*/
public S hasOnlyCompatibleTerminalTypes(VecTerminalType... compatibleTerminalTypes) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalType varargs is not null.
if (compatibleTerminalTypes == null) failWithMessage("Expecting compatibleTerminalTypes parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getCompatibleTerminalTypes(), compatibleTerminalTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's compatibleTerminalTypes contains only the given VecTerminalType elements in Collection and nothing else in whatever order.
* @param compatibleTerminalTypes the given elements that should be contained in actual VecCavitySpecification's compatibleTerminalTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's compatibleTerminalTypes does not contain all given VecTerminalType elements.
*/
public S hasOnlyCompatibleTerminalTypes(java.util.Collection extends VecTerminalType> compatibleTerminalTypes) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalType collection is not null.
if (compatibleTerminalTypes == null) {
failWithMessage("Expecting compatibleTerminalTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getCompatibleTerminalTypes(), compatibleTerminalTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's compatibleTerminalTypes does not contain the given VecTerminalType elements.
*
* @param compatibleTerminalTypes the given elements that should not be in actual VecCavitySpecification's compatibleTerminalTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's compatibleTerminalTypes contains any given VecTerminalType elements.
*/
public S doesNotHaveCompatibleTerminalTypes(VecTerminalType... compatibleTerminalTypes) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalType varargs is not null.
if (compatibleTerminalTypes == null) failWithMessage("Expecting compatibleTerminalTypes parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getCompatibleTerminalTypes(), compatibleTerminalTypes);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's compatibleTerminalTypes does not contain the given VecTerminalType elements in Collection.
*
* @param compatibleTerminalTypes the given elements that should not be in actual VecCavitySpecification's compatibleTerminalTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's compatibleTerminalTypes contains any given VecTerminalType elements.
*/
public S doesNotHaveCompatibleTerminalTypes(java.util.Collection extends VecTerminalType> compatibleTerminalTypes) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalType collection is not null.
if (compatibleTerminalTypes == null) {
failWithMessage("Expecting compatibleTerminalTypes parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getCompatibleTerminalTypes(), compatibleTerminalTypes.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification has no compatibleTerminalTypes.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's compatibleTerminalTypes is not empty.
*/
public S hasNoCompatibleTerminalTypes() {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have compatibleTerminalTypes but had :\n <%s>";
// check
if (actual.getCompatibleTerminalTypes().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getCompatibleTerminalTypes());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's geometry is equal to the given one.
* @param geometry the given geometry to compare the actual VecCavitySpecification's geometry to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavitySpecification's geometry is not equal to the given one.
*/
public S hasGeometry(String geometry) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting geometry of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualGeometry = actual.getGeometry();
if (!Objects.areEqual(actualGeometry, geometry)) {
failWithMessage(assertjErrorMessage, actual, geometry, actualGeometry);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's maxWireElementOutsideDiameter is equal to the given one.
* @param maxWireElementOutsideDiameter the given maxWireElementOutsideDiameter to compare the actual VecCavitySpecification's maxWireElementOutsideDiameter to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavitySpecification's maxWireElementOutsideDiameter is not equal to the given one.
*/
public S hasMaxWireElementOutsideDiameter(VecNumericalValue maxWireElementOutsideDiameter) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting maxWireElementOutsideDiameter of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualMaxWireElementOutsideDiameter = actual.getMaxWireElementOutsideDiameter();
if (!Objects.areEqual(actualMaxWireElementOutsideDiameter, maxWireElementOutsideDiameter)) {
failWithMessage(assertjErrorMessage, actual, maxWireElementOutsideDiameter, actualMaxWireElementOutsideDiameter);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's minWireElementOutsideDiameter is equal to the given one.
* @param minWireElementOutsideDiameter the given minWireElementOutsideDiameter to compare the actual VecCavitySpecification's minWireElementOutsideDiameter to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavitySpecification's minWireElementOutsideDiameter is not equal to the given one.
*/
public S hasMinWireElementOutsideDiameter(VecNumericalValue minWireElementOutsideDiameter) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting minWireElementOutsideDiameter of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualMinWireElementOutsideDiameter = actual.getMinWireElementOutsideDiameter();
if (!Objects.areEqual(actualMinWireElementOutsideDiameter, minWireElementOutsideDiameter)) {
failWithMessage(assertjErrorMessage, actual, minWireElementOutsideDiameter, actualMinWireElementOutsideDiameter);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's primaryLockingType is equal to the given one.
* @param primaryLockingType the given primaryLockingType to compare the actual VecCavitySpecification's primaryLockingType to.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavitySpecification's primaryLockingType is not equal to the given one.
*/
public S hasPrimaryLockingType(String primaryLockingType) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting primaryLockingType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualPrimaryLockingType = actual.getPrimaryLockingType();
if (!Objects.areEqual(actualPrimaryLockingType, primaryLockingType)) {
failWithMessage(assertjErrorMessage, actual, primaryLockingType, actualPrimaryLockingType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's refCavity contains the given VecCavity elements.
* @param refCavity the given elements that should be contained in actual VecCavitySpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's refCavity does not contain all given VecCavity elements.
*/
public S hasRefCavity(VecCavity... refCavity) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity varargs is not null.
if (refCavity == null) failWithMessage("Expecting refCavity parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavity(), refCavity);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's refCavity contains the given VecCavity elements in Collection.
* @param refCavity the given elements that should be contained in actual VecCavitySpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's refCavity does not contain all given VecCavity elements.
*/
public S hasRefCavity(java.util.Collection extends VecCavity> refCavity) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity collection is not null.
if (refCavity == null) {
failWithMessage("Expecting refCavity parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavity(), refCavity.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's refCavity contains only the given VecCavity elements and nothing else in whatever order.
* @param refCavity the given elements that should be contained in actual VecCavitySpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's refCavity does not contain all given VecCavity elements.
*/
public S hasOnlyRefCavity(VecCavity... refCavity) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity varargs is not null.
if (refCavity == null) failWithMessage("Expecting refCavity parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavity(), refCavity);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's refCavity contains only the given VecCavity elements in Collection and nothing else in whatever order.
* @param refCavity the given elements that should be contained in actual VecCavitySpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's refCavity does not contain all given VecCavity elements.
*/
public S hasOnlyRefCavity(java.util.Collection extends VecCavity> refCavity) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity collection is not null.
if (refCavity == null) {
failWithMessage("Expecting refCavity parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavity(), refCavity.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's refCavity does not contain the given VecCavity elements.
*
* @param refCavity the given elements that should not be in actual VecCavitySpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's refCavity contains any given VecCavity elements.
*/
public S doesNotHaveRefCavity(VecCavity... refCavity) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity varargs is not null.
if (refCavity == null) failWithMessage("Expecting refCavity parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavity(), refCavity);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification's refCavity does not contain the given VecCavity elements in Collection.
*
* @param refCavity the given elements that should not be in actual VecCavitySpecification's refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's refCavity contains any given VecCavity elements.
*/
public S doesNotHaveRefCavity(java.util.Collection extends VecCavity> refCavity) {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// check that given VecCavity collection is not null.
if (refCavity == null) {
failWithMessage("Expecting refCavity parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavity(), refCavity.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification has no refCavity.
* @return this assertion object.
* @throws AssertionError if the actual VecCavitySpecification's refCavity is not empty.
*/
public S hasNoRefCavity() {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCavity but had :\n <%s>";
// check
if (actual.getRefCavity().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCavity());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification is sealable.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavitySpecification is not sealable.
*/
public S isSealable() {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.FALSE, actual.isSealable())) {
failWithMessage("\nExpecting that actual VecCavitySpecification is sealable but is not.");
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCavitySpecification is not sealable.
* @return this assertion object.
* @throws AssertionError - if the actual VecCavitySpecification is sealable.
*/
public S isNotSealable() {
// check that actual VecCavitySpecification we want to make assertions on is not null.
isNotNull();
// null safe check
if (Objects.areEqual(Boolean.TRUE, actual.isSealable())) {
failWithMessage("\nExpecting that actual VecCavitySpecification is not sealable but is.");
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy