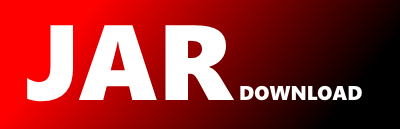
com.foursoft.vecmodel.vec120.AbstractVecComponentPortAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecComponentPort} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecComponentPortAssert, A extends VecComponentPort> extends AbstractVecConfigurableElementAssert {
/**
* Creates a new {@link AbstractVecComponentPortAssert}
to make assertions on actual VecComponentPort.
* @param actual the VecComponentPort we want to make assertions on.
*/
protected AbstractVecComponentPortAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecComponentPort's descriptions contains the given VecAbstractLocalizedString elements.
* @param descriptions the given elements that should be contained in actual VecComponentPort's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's descriptions contains the given VecAbstractLocalizedString elements in Collection.
* @param descriptions the given elements that should be contained in actual VecComponentPort's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's descriptions contains only the given VecAbstractLocalizedString elements and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecComponentPort's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's descriptions contains only the given VecAbstractLocalizedString elements in Collection and nothing else in whatever order.
* @param descriptions the given elements that should be contained in actual VecComponentPort's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's descriptions does not contain all given VecAbstractLocalizedString elements.
*/
public S hasOnlyDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's descriptions does not contain the given VecAbstractLocalizedString elements.
*
* @param descriptions the given elements that should not be in actual VecComponentPort's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(VecAbstractLocalizedString... descriptions) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString varargs is not null.
if (descriptions == null) failWithMessage("Expecting descriptions parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's descriptions does not contain the given VecAbstractLocalizedString elements in Collection.
*
* @param descriptions the given elements that should not be in actual VecComponentPort's descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's descriptions contains any given VecAbstractLocalizedString elements.
*/
public S doesNotHaveDescriptions(java.util.Collection extends VecAbstractLocalizedString> descriptions) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecAbstractLocalizedString collection is not null.
if (descriptions == null) {
failWithMessage("Expecting descriptions parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getDescriptions(), descriptions.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort has no descriptions.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's descriptions is not empty.
*/
public S hasNoDescriptions() {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have descriptions but had :\n <%s>";
// check
if (actual.getDescriptions().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getDescriptions());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's identification is equal to the given one.
* @param identification the given identification to compare the actual VecComponentPort's identification to.
* @return this assertion object.
* @throws AssertionError - if the actual VecComponentPort's identification is not equal to the given one.
*/
public S hasIdentification(String identification) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting identification of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualIdentification = actual.getIdentification();
if (!Objects.areEqual(actualIdentification, identification)) {
failWithMessage(assertjErrorMessage, actual, identification, actualIdentification);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's networkPort is equal to the given one.
* @param networkPort the given networkPort to compare the actual VecComponentPort's networkPort to.
* @return this assertion object.
* @throws AssertionError - if the actual VecComponentPort's networkPort is not equal to the given one.
*/
public S hasNetworkPort(VecNetworkPort networkPort) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting networkPort of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNetworkPort actualNetworkPort = actual.getNetworkPort();
if (!Objects.areEqual(actualNetworkPort, networkPort)) {
failWithMessage(assertjErrorMessage, actual, networkPort, actualNetworkPort);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's parentComponentConnector is equal to the given one.
* @param parentComponentConnector the given parentComponentConnector to compare the actual VecComponentPort's parentComponentConnector to.
* @return this assertion object.
* @throws AssertionError - if the actual VecComponentPort's parentComponentConnector is not equal to the given one.
*/
public S hasParentComponentConnector(VecComponentConnector parentComponentConnector) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting parentComponentConnector of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecComponentConnector actualParentComponentConnector = actual.getParentComponentConnector();
if (!Objects.areEqual(actualParentComponentConnector, parentComponentConnector)) {
failWithMessage(assertjErrorMessage, actual, parentComponentConnector, actualParentComponentConnector);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refCavityReference contains the given VecCavityReference elements.
* @param refCavityReference the given elements that should be contained in actual VecComponentPort's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refCavityReference does not contain all given VecCavityReference elements.
*/
public S hasRefCavityReference(VecCavityReference... refCavityReference) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference varargs is not null.
if (refCavityReference == null) failWithMessage("Expecting refCavityReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityReference(), refCavityReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refCavityReference contains the given VecCavityReference elements in Collection.
* @param refCavityReference the given elements that should be contained in actual VecComponentPort's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refCavityReference does not contain all given VecCavityReference elements.
*/
public S hasRefCavityReference(java.util.Collection extends VecCavityReference> refCavityReference) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference collection is not null.
if (refCavityReference == null) {
failWithMessage("Expecting refCavityReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefCavityReference(), refCavityReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refCavityReference contains only the given VecCavityReference elements and nothing else in whatever order.
* @param refCavityReference the given elements that should be contained in actual VecComponentPort's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refCavityReference does not contain all given VecCavityReference elements.
*/
public S hasOnlyRefCavityReference(VecCavityReference... refCavityReference) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference varargs is not null.
if (refCavityReference == null) failWithMessage("Expecting refCavityReference parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityReference(), refCavityReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refCavityReference contains only the given VecCavityReference elements in Collection and nothing else in whatever order.
* @param refCavityReference the given elements that should be contained in actual VecComponentPort's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refCavityReference does not contain all given VecCavityReference elements.
*/
public S hasOnlyRefCavityReference(java.util.Collection extends VecCavityReference> refCavityReference) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference collection is not null.
if (refCavityReference == null) {
failWithMessage("Expecting refCavityReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefCavityReference(), refCavityReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refCavityReference does not contain the given VecCavityReference elements.
*
* @param refCavityReference the given elements that should not be in actual VecComponentPort's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refCavityReference contains any given VecCavityReference elements.
*/
public S doesNotHaveRefCavityReference(VecCavityReference... refCavityReference) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference varargs is not null.
if (refCavityReference == null) failWithMessage("Expecting refCavityReference parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityReference(), refCavityReference);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refCavityReference does not contain the given VecCavityReference elements in Collection.
*
* @param refCavityReference the given elements that should not be in actual VecComponentPort's refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refCavityReference contains any given VecCavityReference elements.
*/
public S doesNotHaveRefCavityReference(java.util.Collection extends VecCavityReference> refCavityReference) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecCavityReference collection is not null.
if (refCavityReference == null) {
failWithMessage("Expecting refCavityReference parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefCavityReference(), refCavityReference.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort has no refCavityReference.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refCavityReference is not empty.
*/
public S hasNoRefCavityReference() {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refCavityReference but had :\n <%s>";
// check
if (actual.getRefCavityReference().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefCavityReference());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refConnectionEnd contains the given VecConnectionEnd elements.
* @param refConnectionEnd the given elements that should be contained in actual VecComponentPort's refConnectionEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refConnectionEnd does not contain all given VecConnectionEnd elements.
*/
public S hasRefConnectionEnd(VecConnectionEnd... refConnectionEnd) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd varargs is not null.
if (refConnectionEnd == null) failWithMessage("Expecting refConnectionEnd parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefConnectionEnd(), refConnectionEnd);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refConnectionEnd contains the given VecConnectionEnd elements in Collection.
* @param refConnectionEnd the given elements that should be contained in actual VecComponentPort's refConnectionEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refConnectionEnd does not contain all given VecConnectionEnd elements.
*/
public S hasRefConnectionEnd(java.util.Collection extends VecConnectionEnd> refConnectionEnd) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd collection is not null.
if (refConnectionEnd == null) {
failWithMessage("Expecting refConnectionEnd parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefConnectionEnd(), refConnectionEnd.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refConnectionEnd contains only the given VecConnectionEnd elements and nothing else in whatever order.
* @param refConnectionEnd the given elements that should be contained in actual VecComponentPort's refConnectionEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refConnectionEnd does not contain all given VecConnectionEnd elements.
*/
public S hasOnlyRefConnectionEnd(VecConnectionEnd... refConnectionEnd) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd varargs is not null.
if (refConnectionEnd == null) failWithMessage("Expecting refConnectionEnd parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefConnectionEnd(), refConnectionEnd);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refConnectionEnd contains only the given VecConnectionEnd elements in Collection and nothing else in whatever order.
* @param refConnectionEnd the given elements that should be contained in actual VecComponentPort's refConnectionEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refConnectionEnd does not contain all given VecConnectionEnd elements.
*/
public S hasOnlyRefConnectionEnd(java.util.Collection extends VecConnectionEnd> refConnectionEnd) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd collection is not null.
if (refConnectionEnd == null) {
failWithMessage("Expecting refConnectionEnd parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefConnectionEnd(), refConnectionEnd.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refConnectionEnd does not contain the given VecConnectionEnd elements.
*
* @param refConnectionEnd the given elements that should not be in actual VecComponentPort's refConnectionEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refConnectionEnd contains any given VecConnectionEnd elements.
*/
public S doesNotHaveRefConnectionEnd(VecConnectionEnd... refConnectionEnd) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd varargs is not null.
if (refConnectionEnd == null) failWithMessage("Expecting refConnectionEnd parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefConnectionEnd(), refConnectionEnd);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refConnectionEnd does not contain the given VecConnectionEnd elements in Collection.
*
* @param refConnectionEnd the given elements that should not be in actual VecComponentPort's refConnectionEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refConnectionEnd contains any given VecConnectionEnd elements.
*/
public S doesNotHaveRefConnectionEnd(java.util.Collection extends VecConnectionEnd> refConnectionEnd) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecConnectionEnd collection is not null.
if (refConnectionEnd == null) {
failWithMessage("Expecting refConnectionEnd parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefConnectionEnd(), refConnectionEnd.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort has no refConnectionEnd.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refConnectionEnd is not empty.
*/
public S hasNoRefConnectionEnd() {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refConnectionEnd but had :\n <%s>";
// check
if (actual.getRefConnectionEnd().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefConnectionEnd());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refTerminalRole contains the given VecTerminalRole elements.
* @param refTerminalRole the given elements that should be contained in actual VecComponentPort's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refTerminalRole does not contain all given VecTerminalRole elements.
*/
public S hasRefTerminalRole(VecTerminalRole... refTerminalRole) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole varargs is not null.
if (refTerminalRole == null) failWithMessage("Expecting refTerminalRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTerminalRole(), refTerminalRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refTerminalRole contains the given VecTerminalRole elements in Collection.
* @param refTerminalRole the given elements that should be contained in actual VecComponentPort's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refTerminalRole does not contain all given VecTerminalRole elements.
*/
public S hasRefTerminalRole(java.util.Collection extends VecTerminalRole> refTerminalRole) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole collection is not null.
if (refTerminalRole == null) {
failWithMessage("Expecting refTerminalRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTerminalRole(), refTerminalRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refTerminalRole contains only the given VecTerminalRole elements and nothing else in whatever order.
* @param refTerminalRole the given elements that should be contained in actual VecComponentPort's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refTerminalRole does not contain all given VecTerminalRole elements.
*/
public S hasOnlyRefTerminalRole(VecTerminalRole... refTerminalRole) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole varargs is not null.
if (refTerminalRole == null) failWithMessage("Expecting refTerminalRole parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTerminalRole(), refTerminalRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refTerminalRole contains only the given VecTerminalRole elements in Collection and nothing else in whatever order.
* @param refTerminalRole the given elements that should be contained in actual VecComponentPort's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refTerminalRole does not contain all given VecTerminalRole elements.
*/
public S hasOnlyRefTerminalRole(java.util.Collection extends VecTerminalRole> refTerminalRole) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole collection is not null.
if (refTerminalRole == null) {
failWithMessage("Expecting refTerminalRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTerminalRole(), refTerminalRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refTerminalRole does not contain the given VecTerminalRole elements.
*
* @param refTerminalRole the given elements that should not be in actual VecComponentPort's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refTerminalRole contains any given VecTerminalRole elements.
*/
public S doesNotHaveRefTerminalRole(VecTerminalRole... refTerminalRole) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole varargs is not null.
if (refTerminalRole == null) failWithMessage("Expecting refTerminalRole parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTerminalRole(), refTerminalRole);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's refTerminalRole does not contain the given VecTerminalRole elements in Collection.
*
* @param refTerminalRole the given elements that should not be in actual VecComponentPort's refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refTerminalRole contains any given VecTerminalRole elements.
*/
public S doesNotHaveRefTerminalRole(java.util.Collection extends VecTerminalRole> refTerminalRole) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalRole collection is not null.
if (refTerminalRole == null) {
failWithMessage("Expecting refTerminalRole parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTerminalRole(), refTerminalRole.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort has no refTerminalRole.
* @return this assertion object.
* @throws AssertionError if the actual VecComponentPort's refTerminalRole is not empty.
*/
public S hasNoRefTerminalRole() {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refTerminalRole but had :\n <%s>";
// check
if (actual.getRefTerminalRole().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefTerminalRole());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's signal is equal to the given one.
* @param signal the given signal to compare the actual VecComponentPort's signal to.
* @return this assertion object.
* @throws AssertionError - if the actual VecComponentPort's signal is not equal to the given one.
*/
public S hasSignal(VecSignal signal) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting signal of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecSignal actualSignal = actual.getSignal();
if (!Objects.areEqual(actualSignal, signal)) {
failWithMessage(assertjErrorMessage, actual, signal, actualSignal);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecComponentPort's signalDirection is equal to the given one.
* @param signalDirection the given signalDirection to compare the actual VecComponentPort's signalDirection to.
* @return this assertion object.
* @throws AssertionError - if the actual VecComponentPort's signalDirection is not equal to the given one.
*/
public S hasSignalDirection(VecSignalDirection signalDirection) {
// check that actual VecComponentPort we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting signalDirection of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecSignalDirection actualSignalDirection = actual.getSignalDirection();
if (!Objects.areEqual(actualSignalDirection, signalDirection)) {
failWithMessage(assertjErrorMessage, actual, signalDirection, actualSignalDirection);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy