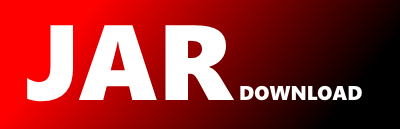
com.foursoft.vecmodel.vec120.AbstractVecCompositionSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
/**
* Abstract base class for {@link VecCompositionSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecCompositionSpecificationAssert, A extends VecCompositionSpecification> extends AbstractVecSpecificationAssert {
/**
* Creates a new {@link AbstractVecCompositionSpecificationAssert}
to make assertions on actual VecCompositionSpecification.
* @param actual the VecCompositionSpecification we want to make assertions on.
*/
protected AbstractVecCompositionSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecCompositionSpecification's components contains the given VecPartOccurrence elements.
* @param components the given elements that should be contained in actual VecCompositionSpecification's components.
* @return this assertion object.
* @throws AssertionError if the actual VecCompositionSpecification's components does not contain all given VecPartOccurrence elements.
*/
public S hasComponents(VecPartOccurrence... components) {
// check that actual VecCompositionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (components == null) failWithMessage("Expecting components parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getComponents(), components);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCompositionSpecification's components contains the given VecPartOccurrence elements in Collection.
* @param components the given elements that should be contained in actual VecCompositionSpecification's components.
* @return this assertion object.
* @throws AssertionError if the actual VecCompositionSpecification's components does not contain all given VecPartOccurrence elements.
*/
public S hasComponents(java.util.Collection extends VecPartOccurrence> components) {
// check that actual VecCompositionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (components == null) {
failWithMessage("Expecting components parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getComponents(), components.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCompositionSpecification's components contains only the given VecPartOccurrence elements and nothing else in whatever order.
* @param components the given elements that should be contained in actual VecCompositionSpecification's components.
* @return this assertion object.
* @throws AssertionError if the actual VecCompositionSpecification's components does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyComponents(VecPartOccurrence... components) {
// check that actual VecCompositionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (components == null) failWithMessage("Expecting components parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getComponents(), components);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCompositionSpecification's components contains only the given VecPartOccurrence elements in Collection and nothing else in whatever order.
* @param components the given elements that should be contained in actual VecCompositionSpecification's components.
* @return this assertion object.
* @throws AssertionError if the actual VecCompositionSpecification's components does not contain all given VecPartOccurrence elements.
*/
public S hasOnlyComponents(java.util.Collection extends VecPartOccurrence> components) {
// check that actual VecCompositionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (components == null) {
failWithMessage("Expecting components parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getComponents(), components.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCompositionSpecification's components does not contain the given VecPartOccurrence elements.
*
* @param components the given elements that should not be in actual VecCompositionSpecification's components.
* @return this assertion object.
* @throws AssertionError if the actual VecCompositionSpecification's components contains any given VecPartOccurrence elements.
*/
public S doesNotHaveComponents(VecPartOccurrence... components) {
// check that actual VecCompositionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence varargs is not null.
if (components == null) failWithMessage("Expecting components parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getComponents(), components);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCompositionSpecification's components does not contain the given VecPartOccurrence elements in Collection.
*
* @param components the given elements that should not be in actual VecCompositionSpecification's components.
* @return this assertion object.
* @throws AssertionError if the actual VecCompositionSpecification's components contains any given VecPartOccurrence elements.
*/
public S doesNotHaveComponents(java.util.Collection extends VecPartOccurrence> components) {
// check that actual VecCompositionSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecPartOccurrence collection is not null.
if (components == null) {
failWithMessage("Expecting components parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getComponents(), components.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecCompositionSpecification has no components.
* @return this assertion object.
* @throws AssertionError if the actual VecCompositionSpecification's components is not empty.
*/
public S hasNoComponents() {
// check that actual VecCompositionSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have components but had :\n <%s>";
// check
if (actual.getComponents().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getComponents());
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy