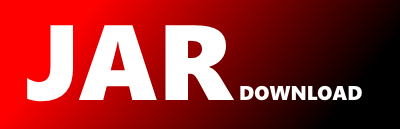
com.foursoft.vecmodel.vec120.AbstractVecConductorSpecificationAssert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vec120-assertions Show documentation
Show all versions of vec120-assertions Show documentation
The Assertions Library for VEC version 1.2.0.
package com.foursoft.vecmodel.vec120;
import org.assertj.core.internal.Iterables;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link VecConductorSpecification} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractVecConductorSpecificationAssert, A extends VecConductorSpecification> extends AbstractVecSpecificationAssert {
/**
* Creates a new {@link AbstractVecConductorSpecificationAssert}
to make assertions on actual VecConductorSpecification.
* @param actual the VecConductorSpecification we want to make assertions on.
*/
protected AbstractVecConductorSpecificationAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual VecConductorSpecification's crossSectionArea is equal to the given one.
* @param crossSectionArea the given crossSectionArea to compare the actual VecConductorSpecification's crossSectionArea to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConductorSpecification's crossSectionArea is not equal to the given one.
*/
public S hasCrossSectionArea(VecNumericalValue crossSectionArea) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting crossSectionArea of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualCrossSectionArea = actual.getCrossSectionArea();
if (!Objects.areEqual(actualCrossSectionArea, crossSectionArea)) {
failWithMessage(assertjErrorMessage, actual, crossSectionArea, actualCrossSectionArea);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's currentInformations contains the given VecConductorCurrentInformation elements.
* @param currentInformations the given elements that should be contained in actual VecConductorSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's currentInformations does not contain all given VecConductorCurrentInformation elements.
*/
public S hasCurrentInformations(VecConductorCurrentInformation... currentInformations) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorCurrentInformation varargs is not null.
if (currentInformations == null) failWithMessage("Expecting currentInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getCurrentInformations(), currentInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's currentInformations contains the given VecConductorCurrentInformation elements in Collection.
* @param currentInformations the given elements that should be contained in actual VecConductorSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's currentInformations does not contain all given VecConductorCurrentInformation elements.
*/
public S hasCurrentInformations(java.util.Collection extends VecConductorCurrentInformation> currentInformations) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorCurrentInformation collection is not null.
if (currentInformations == null) {
failWithMessage("Expecting currentInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getCurrentInformations(), currentInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's currentInformations contains only the given VecConductorCurrentInformation elements and nothing else in whatever order.
* @param currentInformations the given elements that should be contained in actual VecConductorSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's currentInformations does not contain all given VecConductorCurrentInformation elements.
*/
public S hasOnlyCurrentInformations(VecConductorCurrentInformation... currentInformations) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorCurrentInformation varargs is not null.
if (currentInformations == null) failWithMessage("Expecting currentInformations parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getCurrentInformations(), currentInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's currentInformations contains only the given VecConductorCurrentInformation elements in Collection and nothing else in whatever order.
* @param currentInformations the given elements that should be contained in actual VecConductorSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's currentInformations does not contain all given VecConductorCurrentInformation elements.
*/
public S hasOnlyCurrentInformations(java.util.Collection extends VecConductorCurrentInformation> currentInformations) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorCurrentInformation collection is not null.
if (currentInformations == null) {
failWithMessage("Expecting currentInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getCurrentInformations(), currentInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's currentInformations does not contain the given VecConductorCurrentInformation elements.
*
* @param currentInformations the given elements that should not be in actual VecConductorSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's currentInformations contains any given VecConductorCurrentInformation elements.
*/
public S doesNotHaveCurrentInformations(VecConductorCurrentInformation... currentInformations) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorCurrentInformation varargs is not null.
if (currentInformations == null) failWithMessage("Expecting currentInformations parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getCurrentInformations(), currentInformations);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's currentInformations does not contain the given VecConductorCurrentInformation elements in Collection.
*
* @param currentInformations the given elements that should not be in actual VecConductorSpecification's currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's currentInformations contains any given VecConductorCurrentInformation elements.
*/
public S doesNotHaveCurrentInformations(java.util.Collection extends VecConductorCurrentInformation> currentInformations) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecConductorCurrentInformation collection is not null.
if (currentInformations == null) {
failWithMessage("Expecting currentInformations parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getCurrentInformations(), currentInformations.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification has no currentInformations.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's currentInformations is not empty.
*/
public S hasNoCurrentInformations() {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have currentInformations but had :\n <%s>";
// check
if (actual.getCurrentInformations().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getCurrentInformations());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's massInformation is equal to the given one.
* @param massInformation the given massInformation to compare the actual VecConductorSpecification's massInformation to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConductorSpecification's massInformation is not equal to the given one.
*/
public S hasMassInformation(VecMassInformation massInformation) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting massInformation of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecMassInformation actualMassInformation = actual.getMassInformation();
if (!Objects.areEqual(actualMassInformation, massInformation)) {
failWithMessage(assertjErrorMessage, actual, massInformation, actualMassInformation);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's materials contains the given VecMaterial elements.
* @param materials the given elements that should be contained in actual VecConductorSpecification's materials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's materials does not contain all given VecMaterial elements.
*/
public S hasMaterials(VecMaterial... materials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (materials == null) failWithMessage("Expecting materials parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getMaterials(), materials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's materials contains the given VecMaterial elements in Collection.
* @param materials the given elements that should be contained in actual VecConductorSpecification's materials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's materials does not contain all given VecMaterial elements.
*/
public S hasMaterials(java.util.Collection extends VecMaterial> materials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (materials == null) {
failWithMessage("Expecting materials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getMaterials(), materials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's materials contains only the given VecMaterial elements and nothing else in whatever order.
* @param materials the given elements that should be contained in actual VecConductorSpecification's materials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's materials does not contain all given VecMaterial elements.
*/
public S hasOnlyMaterials(VecMaterial... materials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (materials == null) failWithMessage("Expecting materials parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getMaterials(), materials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's materials contains only the given VecMaterial elements in Collection and nothing else in whatever order.
* @param materials the given elements that should be contained in actual VecConductorSpecification's materials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's materials does not contain all given VecMaterial elements.
*/
public S hasOnlyMaterials(java.util.Collection extends VecMaterial> materials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (materials == null) {
failWithMessage("Expecting materials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getMaterials(), materials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's materials does not contain the given VecMaterial elements.
*
* @param materials the given elements that should not be in actual VecConductorSpecification's materials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's materials contains any given VecMaterial elements.
*/
public S doesNotHaveMaterials(VecMaterial... materials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (materials == null) failWithMessage("Expecting materials parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getMaterials(), materials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's materials does not contain the given VecMaterial elements in Collection.
*
* @param materials the given elements that should not be in actual VecConductorSpecification's materials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's materials contains any given VecMaterial elements.
*/
public S doesNotHaveMaterials(java.util.Collection extends VecMaterial> materials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (materials == null) {
failWithMessage("Expecting materials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getMaterials(), materials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification has no materials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's materials is not empty.
*/
public S hasNoMaterials() {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have materials but had :\n <%s>";
// check
if (actual.getMaterials().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getMaterials());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's numberOfStrands is equal to the given one.
* @param numberOfStrands the given numberOfStrands to compare the actual VecConductorSpecification's numberOfStrands to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConductorSpecification's numberOfStrands is not equal to the given one.
*/
public S hasNumberOfStrands(VecNumericalValue numberOfStrands) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting numberOfStrands of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualNumberOfStrands = actual.getNumberOfStrands();
if (!Objects.areEqual(actualNumberOfStrands, numberOfStrands)) {
failWithMessage(assertjErrorMessage, actual, numberOfStrands, actualNumberOfStrands);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's platingMaterials contains the given VecMaterial elements.
* @param platingMaterials the given elements that should be contained in actual VecConductorSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's platingMaterials does not contain all given VecMaterial elements.
*/
public S hasPlatingMaterials(VecMaterial... platingMaterials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (platingMaterials == null) failWithMessage("Expecting platingMaterials parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPlatingMaterials(), platingMaterials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's platingMaterials contains the given VecMaterial elements in Collection.
* @param platingMaterials the given elements that should be contained in actual VecConductorSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's platingMaterials does not contain all given VecMaterial elements.
*/
public S hasPlatingMaterials(java.util.Collection extends VecMaterial> platingMaterials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (platingMaterials == null) {
failWithMessage("Expecting platingMaterials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getPlatingMaterials(), platingMaterials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's platingMaterials contains only the given VecMaterial elements and nothing else in whatever order.
* @param platingMaterials the given elements that should be contained in actual VecConductorSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's platingMaterials does not contain all given VecMaterial elements.
*/
public S hasOnlyPlatingMaterials(VecMaterial... platingMaterials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (platingMaterials == null) failWithMessage("Expecting platingMaterials parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPlatingMaterials(), platingMaterials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's platingMaterials contains only the given VecMaterial elements in Collection and nothing else in whatever order.
* @param platingMaterials the given elements that should be contained in actual VecConductorSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's platingMaterials does not contain all given VecMaterial elements.
*/
public S hasOnlyPlatingMaterials(java.util.Collection extends VecMaterial> platingMaterials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (platingMaterials == null) {
failWithMessage("Expecting platingMaterials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getPlatingMaterials(), platingMaterials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's platingMaterials does not contain the given VecMaterial elements.
*
* @param platingMaterials the given elements that should not be in actual VecConductorSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's platingMaterials contains any given VecMaterial elements.
*/
public S doesNotHavePlatingMaterials(VecMaterial... platingMaterials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial varargs is not null.
if (platingMaterials == null) failWithMessage("Expecting platingMaterials parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPlatingMaterials(), platingMaterials);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's platingMaterials does not contain the given VecMaterial elements in Collection.
*
* @param platingMaterials the given elements that should not be in actual VecConductorSpecification's platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's platingMaterials contains any given VecMaterial elements.
*/
public S doesNotHavePlatingMaterials(java.util.Collection extends VecMaterial> platingMaterials) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecMaterial collection is not null.
if (platingMaterials == null) {
failWithMessage("Expecting platingMaterials parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getPlatingMaterials(), platingMaterials.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification has no platingMaterials.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's platingMaterials is not empty.
*/
public S hasNoPlatingMaterials() {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have platingMaterials but had :\n <%s>";
// check
if (actual.getPlatingMaterials().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getPlatingMaterials());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refInternalComponentConnection contains the given VecInternalComponentConnection elements.
* @param refInternalComponentConnection the given elements that should be contained in actual VecConductorSpecification's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refInternalComponentConnection does not contain all given VecInternalComponentConnection elements.
*/
public S hasRefInternalComponentConnection(VecInternalComponentConnection... refInternalComponentConnection) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection varargs is not null.
if (refInternalComponentConnection == null) failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refInternalComponentConnection contains the given VecInternalComponentConnection elements in Collection.
* @param refInternalComponentConnection the given elements that should be contained in actual VecConductorSpecification's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refInternalComponentConnection does not contain all given VecInternalComponentConnection elements.
*/
public S hasRefInternalComponentConnection(java.util.Collection extends VecInternalComponentConnection> refInternalComponentConnection) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection collection is not null.
if (refInternalComponentConnection == null) {
failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refInternalComponentConnection contains only the given VecInternalComponentConnection elements and nothing else in whatever order.
* @param refInternalComponentConnection the given elements that should be contained in actual VecConductorSpecification's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refInternalComponentConnection does not contain all given VecInternalComponentConnection elements.
*/
public S hasOnlyRefInternalComponentConnection(VecInternalComponentConnection... refInternalComponentConnection) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection varargs is not null.
if (refInternalComponentConnection == null) failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refInternalComponentConnection contains only the given VecInternalComponentConnection elements in Collection and nothing else in whatever order.
* @param refInternalComponentConnection the given elements that should be contained in actual VecConductorSpecification's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refInternalComponentConnection does not contain all given VecInternalComponentConnection elements.
*/
public S hasOnlyRefInternalComponentConnection(java.util.Collection extends VecInternalComponentConnection> refInternalComponentConnection) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection collection is not null.
if (refInternalComponentConnection == null) {
failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refInternalComponentConnection does not contain the given VecInternalComponentConnection elements.
*
* @param refInternalComponentConnection the given elements that should not be in actual VecConductorSpecification's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refInternalComponentConnection contains any given VecInternalComponentConnection elements.
*/
public S doesNotHaveRefInternalComponentConnection(VecInternalComponentConnection... refInternalComponentConnection) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection varargs is not null.
if (refInternalComponentConnection == null) failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refInternalComponentConnection does not contain the given VecInternalComponentConnection elements in Collection.
*
* @param refInternalComponentConnection the given elements that should not be in actual VecConductorSpecification's refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refInternalComponentConnection contains any given VecInternalComponentConnection elements.
*/
public S doesNotHaveRefInternalComponentConnection(java.util.Collection extends VecInternalComponentConnection> refInternalComponentConnection) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecInternalComponentConnection collection is not null.
if (refInternalComponentConnection == null) {
failWithMessage("Expecting refInternalComponentConnection parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefInternalComponentConnection(), refInternalComponentConnection.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification has no refInternalComponentConnection.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refInternalComponentConnection is not empty.
*/
public S hasNoRefInternalComponentConnection() {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refInternalComponentConnection but had :\n <%s>";
// check
if (actual.getRefInternalComponentConnection().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefInternalComponentConnection());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refSignal contains the given VecSignal elements.
* @param refSignal the given elements that should be contained in actual VecConductorSpecification's refSignal.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refSignal does not contain all given VecSignal elements.
*/
public S hasRefSignal(VecSignal... refSignal) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSignal varargs is not null.
if (refSignal == null) failWithMessage("Expecting refSignal parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSignal(), refSignal);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refSignal contains the given VecSignal elements in Collection.
* @param refSignal the given elements that should be contained in actual VecConductorSpecification's refSignal.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refSignal does not contain all given VecSignal elements.
*/
public S hasRefSignal(java.util.Collection extends VecSignal> refSignal) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSignal collection is not null.
if (refSignal == null) {
failWithMessage("Expecting refSignal parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefSignal(), refSignal.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refSignal contains only the given VecSignal elements and nothing else in whatever order.
* @param refSignal the given elements that should be contained in actual VecConductorSpecification's refSignal.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refSignal does not contain all given VecSignal elements.
*/
public S hasOnlyRefSignal(VecSignal... refSignal) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSignal varargs is not null.
if (refSignal == null) failWithMessage("Expecting refSignal parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSignal(), refSignal);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refSignal contains only the given VecSignal elements in Collection and nothing else in whatever order.
* @param refSignal the given elements that should be contained in actual VecConductorSpecification's refSignal.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refSignal does not contain all given VecSignal elements.
*/
public S hasOnlyRefSignal(java.util.Collection extends VecSignal> refSignal) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSignal collection is not null.
if (refSignal == null) {
failWithMessage("Expecting refSignal parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefSignal(), refSignal.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refSignal does not contain the given VecSignal elements.
*
* @param refSignal the given elements that should not be in actual VecConductorSpecification's refSignal.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refSignal contains any given VecSignal elements.
*/
public S doesNotHaveRefSignal(VecSignal... refSignal) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSignal varargs is not null.
if (refSignal == null) failWithMessage("Expecting refSignal parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSignal(), refSignal);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refSignal does not contain the given VecSignal elements in Collection.
*
* @param refSignal the given elements that should not be in actual VecConductorSpecification's refSignal.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refSignal contains any given VecSignal elements.
*/
public S doesNotHaveRefSignal(java.util.Collection extends VecSignal> refSignal) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecSignal collection is not null.
if (refSignal == null) {
failWithMessage("Expecting refSignal parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefSignal(), refSignal.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification has no refSignal.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refSignal is not empty.
*/
public S hasNoRefSignal() {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refSignal but had :\n <%s>";
// check
if (actual.getRefSignal().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefSignal());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refTerminalPairing contains the given VecTerminalPairing elements.
* @param refTerminalPairing the given elements that should be contained in actual VecConductorSpecification's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refTerminalPairing does not contain all given VecTerminalPairing elements.
*/
public S hasRefTerminalPairing(VecTerminalPairing... refTerminalPairing) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing varargs is not null.
if (refTerminalPairing == null) failWithMessage("Expecting refTerminalPairing parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTerminalPairing(), refTerminalPairing);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refTerminalPairing contains the given VecTerminalPairing elements in Collection.
* @param refTerminalPairing the given elements that should be contained in actual VecConductorSpecification's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refTerminalPairing does not contain all given VecTerminalPairing elements.
*/
public S hasRefTerminalPairing(java.util.Collection extends VecTerminalPairing> refTerminalPairing) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing collection is not null.
if (refTerminalPairing == null) {
failWithMessage("Expecting refTerminalPairing parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefTerminalPairing(), refTerminalPairing.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refTerminalPairing contains only the given VecTerminalPairing elements and nothing else in whatever order.
* @param refTerminalPairing the given elements that should be contained in actual VecConductorSpecification's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refTerminalPairing does not contain all given VecTerminalPairing elements.
*/
public S hasOnlyRefTerminalPairing(VecTerminalPairing... refTerminalPairing) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing varargs is not null.
if (refTerminalPairing == null) failWithMessage("Expecting refTerminalPairing parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTerminalPairing(), refTerminalPairing);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refTerminalPairing contains only the given VecTerminalPairing elements in Collection and nothing else in whatever order.
* @param refTerminalPairing the given elements that should be contained in actual VecConductorSpecification's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refTerminalPairing does not contain all given VecTerminalPairing elements.
*/
public S hasOnlyRefTerminalPairing(java.util.Collection extends VecTerminalPairing> refTerminalPairing) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing collection is not null.
if (refTerminalPairing == null) {
failWithMessage("Expecting refTerminalPairing parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefTerminalPairing(), refTerminalPairing.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refTerminalPairing does not contain the given VecTerminalPairing elements.
*
* @param refTerminalPairing the given elements that should not be in actual VecConductorSpecification's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refTerminalPairing contains any given VecTerminalPairing elements.
*/
public S doesNotHaveRefTerminalPairing(VecTerminalPairing... refTerminalPairing) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing varargs is not null.
if (refTerminalPairing == null) failWithMessage("Expecting refTerminalPairing parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTerminalPairing(), refTerminalPairing);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refTerminalPairing does not contain the given VecTerminalPairing elements in Collection.
*
* @param refTerminalPairing the given elements that should not be in actual VecConductorSpecification's refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refTerminalPairing contains any given VecTerminalPairing elements.
*/
public S doesNotHaveRefTerminalPairing(java.util.Collection extends VecTerminalPairing> refTerminalPairing) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecTerminalPairing collection is not null.
if (refTerminalPairing == null) {
failWithMessage("Expecting refTerminalPairing parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefTerminalPairing(), refTerminalPairing.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification has no refTerminalPairing.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refTerminalPairing is not empty.
*/
public S hasNoRefTerminalPairing() {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refTerminalPairing but had :\n <%s>";
// check
if (actual.getRefTerminalPairing().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefTerminalPairing());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refWireElementSpecification contains the given VecWireElementSpecification elements.
* @param refWireElementSpecification the given elements that should be contained in actual VecConductorSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasRefWireElementSpecification(VecWireElementSpecification... refWireElementSpecification) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (refWireElementSpecification == null) failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElementSpecification(), refWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refWireElementSpecification contains the given VecWireElementSpecification elements in Collection.
* @param refWireElementSpecification the given elements that should be contained in actual VecConductorSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasRefWireElementSpecification(java.util.Collection extends VecWireElementSpecification> refWireElementSpecification) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (refWireElementSpecification == null) {
failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContains(info, actual.getRefWireElementSpecification(), refWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refWireElementSpecification contains only the given VecWireElementSpecification elements and nothing else in whatever order.
* @param refWireElementSpecification the given elements that should be contained in actual VecConductorSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasOnlyRefWireElementSpecification(VecWireElementSpecification... refWireElementSpecification) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (refWireElementSpecification == null) failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElementSpecification(), refWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refWireElementSpecification contains only the given VecWireElementSpecification elements in Collection and nothing else in whatever order.
* @param refWireElementSpecification the given elements that should be contained in actual VecConductorSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refWireElementSpecification does not contain all given VecWireElementSpecification elements.
*/
public S hasOnlyRefWireElementSpecification(java.util.Collection extends VecWireElementSpecification> refWireElementSpecification) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (refWireElementSpecification == null) {
failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message, to set another message call: info.overridingErrorMessage("my error message");
Iterables.instance().assertContainsOnly(info, actual.getRefWireElementSpecification(), refWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refWireElementSpecification does not contain the given VecWireElementSpecification elements.
*
* @param refWireElementSpecification the given elements that should not be in actual VecConductorSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refWireElementSpecification contains any given VecWireElementSpecification elements.
*/
public S doesNotHaveRefWireElementSpecification(VecWireElementSpecification... refWireElementSpecification) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification varargs is not null.
if (refWireElementSpecification == null) failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElementSpecification(), refWireElementSpecification);
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's refWireElementSpecification does not contain the given VecWireElementSpecification elements in Collection.
*
* @param refWireElementSpecification the given elements that should not be in actual VecConductorSpecification's refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refWireElementSpecification contains any given VecWireElementSpecification elements.
*/
public S doesNotHaveRefWireElementSpecification(java.util.Collection extends VecWireElementSpecification> refWireElementSpecification) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// check that given VecWireElementSpecification collection is not null.
if (refWireElementSpecification == null) {
failWithMessage("Expecting refWireElementSpecification parameter not to be null.");
return myself; // to fool Eclipse "Null pointer access" warning on toArray.
}
// check with standard error message (use overridingErrorMessage before contains to set your own message).
Iterables.instance().assertDoesNotContain(info, actual.getRefWireElementSpecification(), refWireElementSpecification.toArray());
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification has no refWireElementSpecification.
* @return this assertion object.
* @throws AssertionError if the actual VecConductorSpecification's refWireElementSpecification is not empty.
*/
public S hasNoRefWireElementSpecification() {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// we override the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting :\n <%s>\nnot to have refWireElementSpecification but had :\n <%s>";
// check
if (actual.getRefWireElementSpecification().iterator().hasNext()) {
failWithMessage(assertjErrorMessage, actual, actual.getRefWireElementSpecification());
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's resistance is equal to the given one.
* @param resistance the given resistance to compare the actual VecConductorSpecification's resistance to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConductorSpecification's resistance is not equal to the given one.
*/
public S hasResistance(VecNumericalValue resistance) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting resistance of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualResistance = actual.getResistance();
if (!Objects.areEqual(actualResistance, resistance)) {
failWithMessage(assertjErrorMessage, actual, resistance, actualResistance);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's strandDiameter is equal to the given one.
* @param strandDiameter the given strandDiameter to compare the actual VecConductorSpecification's strandDiameter to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConductorSpecification's strandDiameter is not equal to the given one.
*/
public S hasStrandDiameter(VecNumericalValue strandDiameter) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting strandDiameter of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualStrandDiameter = actual.getStrandDiameter();
if (!Objects.areEqual(actualStrandDiameter, strandDiameter)) {
failWithMessage(assertjErrorMessage, actual, strandDiameter, actualStrandDiameter);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's structure is equal to the given one.
* @param structure the given structure to compare the actual VecConductorSpecification's structure to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConductorSpecification's structure is not equal to the given one.
*/
public S hasStructure(String structure) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting structure of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualStructure = actual.getStructure();
if (!Objects.areEqual(actualStructure, structure)) {
failWithMessage(assertjErrorMessage, actual, structure, actualStructure);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's type is equal to the given one.
* @param type the given type to compare the actual VecConductorSpecification's type to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConductorSpecification's type is not equal to the given one.
*/
public S hasType(String type) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting type of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualType = actual.getType();
if (!Objects.areEqual(actualType, type)) {
failWithMessage(assertjErrorMessage, actual, type, actualType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual VecConductorSpecification's voltageRange is equal to the given one.
* @param voltageRange the given voltageRange to compare the actual VecConductorSpecification's voltageRange to.
* @return this assertion object.
* @throws AssertionError - if the actual VecConductorSpecification's voltageRange is not equal to the given one.
*/
public S hasVoltageRange(VecNumericalValue voltageRange) {
// check that actual VecConductorSpecification we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting voltageRange of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
VecNumericalValue actualVoltageRange = actual.getVoltageRange();
if (!Objects.areEqual(actualVoltageRange, voltageRange)) {
failWithMessage(assertjErrorMessage, actual, voltageRange, actualVoltageRange);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy